Welcome! Today we’ll dive into a powerful feature of the C# programming language that is gaining increasing attention from game developers and programmers alike— the C# ‘Span’. This often underappreciated tool has profound implications on your projects, particularly in memory management, and can add a new level of sophistication to your coding proficiency. Let’s explore its utility and potential!
Table of contents
Understanding ‘Span’ in C#
The ‘Span’ in C# is a new type introduced in C# 7.2 that provides a range of benefits, particularly in memory management for your code. It represents a contiguous region of arbitrary memory, which can transform how you manage memory in your games, leading to leaner, faster, and smoother running code.
The Power of ‘Span’
Span can significantly increase a program’s performance, especially in game development where memory management is key. With it, we can drastically reduce the amount of memory allocations and memory copies, improving overall program speed and reducing latency.
Why Learn About ‘Span’?
Understanding and effectively using ‘Span’ can be a real game changer, raising your game development skillset to advanced levels. Despite its power, it’s often overlooked by beginners. But remember, familiarizing yourself with such concepts provides you with profound insights into memory management—a critical aspect in coding and game development.
Moreover, it’s a great addition to your problem-solving toolkit, empowering you to further optimize your code’s performance in ways that you’ve never thought possible.
Creating a Span
Creating ‘Span’ in C# is straightforward. Here’s a simple example:
// Creating a span of integers int[] myArray = new int[5] {1, 2, 3, 4, 5}; Span<int> mySpan = myArray;
With this, you’ve created a ‘Span’ of integers. The ‘Span’ is now essentially a ‘view’ into your array.
Manipulating a Span
Elements inside your Span object can be accessed the same way as a regular array:
int firstElement = mySpan[0]; // Output: 1
You can also modify the elements, and the changes are reflected in the original array:
mySpan[0] = 10; Console.WriteLine(myArray[0]); // Output: 10
Slicing a Span
One of the unique features of Span is ‘slicing’. This means creating a new Span from a range of the original Span:
Span<int> slicedSpan = mySpan.Slice(start: 1, length: 2); // slicedSpan now represents a view onto the 2nd and 3rd elements of myArray
Checking the Length of a Span
Just like arrays, you can also check the length of a Span:
int spanLength = mySpan.Length; // Output: 5
As you can see from these examples, working with Span is similar to working with regular arrays in C#, but provides more powerful capabilities.
Working with Span and Strings
One major advantage ‘Span’ holds is its compatibility with strings. With ‘Span’, you can manipulate and extract sections of the string without creating new string objects, therefore reducing memory load:
//Define string string str = "Learning Span with Zenva"; Span<char> spanChar = str.AsSpan(); //Output: "Learning Span" Console.WriteLine(spanChar.Slice(start: 0, length: 14));
Iterating Over a Span
Similar to arrays, you can iterate over ‘Span’ using a foreach loop:
foreach (var item in mySpan) { Console.WriteLine(item); }
Converting Array to Span and Vice Versa
Converting an array to a ‘Span’ is also quite simple, as you can assign an array directly to a ‘Span’. Converting a ‘Span’ back to an array is straightforward as well:
int[] numbersArray = new[] { 1, 2, 3, 4, 5 }; Span<int> numbersSpan = numbersArray; //From Span back to Array int[] newArray = numbersSpan.ToArray();
Remember, by doing so you’re duplicating all elements in the ‘Span’ and creating a new array in memory.
Clearing a Span
The Clear method can help delete all the elements of the ‘Span’ by setting them to zero:
numbersSpan.Clear(); foreach (int num in numbersSpan) { //Output: 0 Console.WriteLine(num); }
It’s important to note that the Clear method changes the original array.
Working with Multi-Dimensional Span
A significant limitation of ‘Span’ is that it doesn’t support multi-dimensional arrays. However, for such cases, Memory<T> can be used that provides much of the same functionality of Span and supports multi-dimensional arrays.
With the knowledge of ‘Span’, you are now equipped with a powerful tool in your C# programming journey, paving the way towards more efficient, performant, and low-latency games and applications. Happy coding!
Span and ReadOnlySpan
You might have noticed so far, the ‘Span’ objects let us modify the original array or string. But, what if we want an immutable version to ensure data integrity? Here’s where ReadOnlySpan comes in:
ReadOnlySpan<int> readOnlySpan = myArray; readOnlySpan[0] = 10; // This line would result in an error
As the name suggests, a ‘ReadOnlySpan’ offers a view of the data that cannot be modified.
Extracting Elements Using Index
Extracting elements from a Span utilizes the same indexing system as arrays:
int number = mySpan[0]; // Output: 1
Mutable References and Span
Unlike arrays, a Span provides a mutable reference to the elements, which means you’re virtually accessing the original data. Any modification to the elements of the Span reflects back:
mySpan[0] = 100; Console.WriteLine(myArray[0]); // Output: 100
Reversing a Span
Span also comes with a Reverse method. Here’s how to use it:
mySpan.Reverse(); foreach (var item in mySpan) { Console.WriteLine(item); } // Outputs: 5, 4, 3, 2, 1
Remember, this method also modifies the original array or string.
Finding an Element’s Index in a Span
The IndexOf method can be used to find an element’s index within the Span:
int index = mySpan.IndexOf(3); Console.WriteLine(index); // Output: 2
As you can see, working with Span in C# can make your coding simpler, more effective, and far more efficient with regards to memory use. The hands-on examples in these sections should help you get started, but remember, practice is key to mastery!
Where to go next?
Learning never ends! Now that you have a solid understanding of the C# ‘Span’ utility and the change it can bring to your projects, it’s time to take the next step on your journey.
You may consider diving into our Unity Game Development Mini-Degree program—an extensive collection of courses on Unity, a versatile game engine popular among triple-A and indie developers alike. It caters to an array of topics from understanding game mechanics, animations, and audio effects to assisting you in creating cross-platform games in 2D, 3D as well as AR and VR.
For a broader overview of what we offer, you might also want to explore our full lineup of Unity courses. Whether you’re just getting started or fine-tuning your skills, Zenva’s offerings are designed to guide learners from beginner to professional, with self-paced, project-centered lessons, quizzes, and coding challenges that not only teach, but reinforce learning.
Our ultimate goal? To empower you to publish your own games and build a strong portfolio, thus setting the stage for unlimited possibilities in the gaming industry!
Conclusion
It’s exciting to think about how much potential remains out there in the game development world, just waiting to be discovered. As we’ve shown in this tutorial, understanding tools like the C# ‘Span’ and how to use them can make a significant difference in the performance and efficiency of your projects. It might not be the most straightforward concept to grasp for beginners, but once understood, it can be a real asset to your programming skills.
At Zenva, we are committed to providing high-quality, accessible education for anyone who aspires to learn game development, programming and beyond. Our Unity Game Development Mini-Degree is designed to not only teach you coding, but also to guide you on your journey towards becoming an accomplished game developer. No matter your current level, there’s always a new skill waiting to be mastered!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
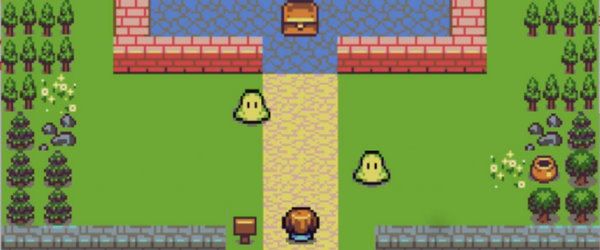
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.