Table of contents
Understanding the ‘For Each’ Loop in C#
For anyone interested in diving into the world of game development, getting a solid understanding of programming concepts is a must, and one such essential concept is the ‘For Each’ loop in C#. Used to iterate through items in a collection, ‘For Each’ in C# is incredibly useful when dealing with large datasets or when you’re looking to perform an operation on every element within a group.
What is the ‘For Each’ Loop?
The ‘For Each’ loop, as mentioned earlier, allows us to iterate through every element in a collection. Collections can be anything from an array, a list, or any other type of data collection in C#.
Why Should I Learn ‘For Each’ in C#?
It simplifies your code and makes it more readable. It automatically takes care of the iteration process for you. ‘For Each’ in C# is more efficient and offers fewer chances for error, since it removes the need to manage the loop’s index counter manually.
Starting with ‘For Each’: A Basic Example
Let’s tackle a simple scenario. Suppose you have an array of integers and you want to print each number. In C#, using a ‘For Each’ loop could look like this:
int[] numbers = {1, 2, 3, 4, 5}; foreach(int num in numbers) { Console.WriteLine(num); }
Here, ‘foreach’ is the command for the loop, ‘int num’ declares a variable to hold the current collection element, ‘in’ specifies the collection to iterate, and ‘numbers’ is the array we’re iterating over.
Moving Forward: Diving Deeper
‘For Each’ isn’t just for arrays. You can use it on any collection of data including Lists, Queues, Stacks, or even Dictionaries. Let’s see how ‘For Each’ handles a List of strings:
List<string> names = new List<string>{"John", "Jane", "Steve"}; foreach(string name in names) { Console.WriteLine(name); }
No matter the collection type, ‘For Each’ in C# handles the element retrieval for you, simplifying your code and refining your program’s readability.
Curious About How ‘For Each’ Works Under the Hood?
Did you know that ‘For Each’ actually uses the ‘IEnumerator’ interface in the background? It calls the ‘MoveNext()’ method to iterate through the collection, and ‘Current’ property to get the current element. While you don’t necessarily need to know this to use ‘For Each’, it’s worth understanding what’s going on behind the scenes.
Going Further: Exploring C# with Zenva
If you’re eager to learn more about C#, especially around its application in game development, we recommend our Unity Game Development Mini-Degree. Whether you’re at the start of your coding journey or looking to expand your expertise, our Unity Mini-Degree will help you master C# and Unity, taking you from a beginner to an advanced game developer.
With this comprehensive curriculum, you’ll gain practical skills by creating functional games, mastering Unity’s UI system, and learning important programming principles – all guided by a hands-on, engaging approach.
Find out more about our Unity Mini-Degree here.
Conclusion
Understanding the ‘For Each’ loop in C# is a key step in your programming journey. Its ability to simplify your code, enhance readability and efficiency, make it a valuable concept to understand and apply. In this tutorial, we explored what ‘For Each’ in C# is, why it’s important to learn, how to use it, and how it operates under the hood.
As a programmer and game developer, your learning journey never stops. Continue to explore, learn, and grow your skills. If you’re passionate about game development, especially with C#, don’t hesitate to check out our Unity Mini-Degree. It’s designed to help you succeed, regardless of your current skill level. With Zenva, you’re in competent hands to navigate the wonderful world of programming and game development. Happy coding!
C# ‘For Each’ Loop with an Array of Strings
An array of strings is another common type of collection ‘For Each’ can be used with. Let’s look at an example where we are iterating over an array of student names.
string[] students = {"John", "Sally", "Tom"}; foreach (string student in students) { Console.WriteLine(student); }
In this example, the ‘For Each’ loop iterates through each student name in the students array, and then uses the WriteLine function to print each name to the console.
Using ‘For Each’ with Multidimensional Arrays
Yes, ‘For Each’ can be used with multidimensional arrays too. Here is an example of a ‘For Each’ loop used with a 2D array:
int[,] numbers2D = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; foreach(int num in numbers2D) { Console.WriteLine(num); }
This code will print out each number in the 2D array.
‘For Each’ with Lists in C#
‘For Each’ can also be used with Lists. Let’s look at how to create a list of integers, then print all even numbers from the list.
List<int> numbersList = new List<int>{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; foreach(int num in numbersList) { if (num % 2 == 0) { Console.WriteLine(num); } }
Here, our ‘For Each’ loop goes through the list, and the ‘if’ statement check if each number is even (i.e., divisible by 2 with no remainder). If it is, it gets printed to the console.
‘For Each’ with Dictionaries in C#
‘For Each’ can be used to iterate through dictionary items as well. Here is an example:
Dictionary<string, int> fruitCounts = new Dictionary<string, int> { {"Apples", 5}, {"Oranges", 7}, {"Pears", 3} }; foreach(KeyValuePair<string, int> entry in fruitCounts) { Console.WriteLine($"We have {entry.Value} {entry.Key}"); }
In this case, we’re looping through a dictionary with string keys (the names of fruits) and integer values (the quantity of each fruit). The ‘For Each’ retrieves each KeyValuePair object from the dictionary, which we can then access with its Value and Key properties.
‘For Each’ with Custom Object Collections
‘For Each’ can also be used with more complex collections, like those comprising custom objects. Let’s see this with an example using a simple ‘Student’ class:
public class Student { public string Name { get; set; } public int Grade { get; set; } } List<Student> students = new List<Student> { new Student { Name = "John", Grade = 85 }, new Student { Name = "Sally", Grade = 90 }, new Student { Name = "Tom", Grade = 78 } }; foreach (Student student in students) { Console.WriteLine($"Name: {student.Name}, Grade: {student.Grade}"); }
In this ‘For Each’ loop, we iterate through a list of custom Student objects. The loop prints the name and grade of each student in the list.
‘For Each’ with Jagged Arrays
A jagged array is an array whose elements are arrays. The arrays that make up the elements can be of different sizes and dimensions. Here is an example of ‘For Each’ used with a jagged array:
int[][] numbersJagged = new int[][] { new int[] {1, 2, 3}, new int[] {4, 5, 6, 7, 8}, new int[] {9, 10} }; foreach (int[] arr in numbersJagged) { foreach(int num in arr) { Console.WriteLine(num); } }
This code will print out each number in the jagged array. Note that we used a nested ‘For Each’ to iterate through the sub-arrays.
‘For Each’ with Queues and Stacks
Collections like Queues and Stacks can also be iterated over with ‘For Each’. Here are quick examples for each.
Queue<int> numbersQueue = new Queue<int>(); numbersQueue.Enqueue(1); numbersQueue.Enqueue(2); numbersQueue.Enqueue(3); foreach (int num in numbersQueue) { Console.WriteLine(num); }
Stack<int> numbersStack = new Stack<int>(); numbersStack.Push(1); numbersStack.Push(2); numbersStack.Push(3); foreach (int num in numbersStack) { Console.WriteLine(num); }
The first ‘For Each’ iterates over a queue of integers and prints them, while the second does the same for a stack.
‘For Each’ with Sets
Let’s not forget about sets. Sets, such as the HashSet or SortedSet, can be also be worked with using ‘For Each’. Here’s a quick example with a HashSet:
HashSet<string> fruitsSet = new HashSet<string> {"apple", "banana", "orange"}; foreach (string fruit in fruitsSet) { Console.WriteLine(fruit); }
In the loop above, we’re printing all the fruit names from our HashSet. With these examples, we see that ‘For Each’ in C# is extremely versatile and adaptable to various collection types. Your programming chore becomes easier, and the readability of your code improves significantly.
‘For Each’ with Linked Lists
C# also provides a LinkedList collection. Here’s a ‘For Each’ example with a LinkedList:
LinkedList<int> numbersLinkedList = new LinkedList<int>(); numbersLinkedList.AddLast(1); numbersLinkedList.AddLast(2); numbersLinkedList.AddLast(3); foreach (int num in numbersLinkedList) { Console.WriteLine(num); }
This ‘For Each’ loop prints each number from the linked list.
‘For Each’ with Enumerations
Enumerations (enums) in C# represent a specific set of named constants. You can also iterate over these constants with a ‘For Each’ loop.
enum DaysOfWeek {Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday}; foreach (DaysOfWeek day in Enum.GetValues(typeof(DaysOfWeek))) { Console.WriteLine(day); }
Here, we have an enumeration for the days of the week. The ‘For Each’ loop fetches each day from the enumeration and prints it.
‘For Each’ with Strings
You can use a ‘For Each’ loop to iterate over each character of a string. Here’s an example:
string greeting = "Hello, World!"; foreach (char ch in greeting) { Console.WriteLine(ch); }
In our ‘For Each’ loop, every character in our quoted string will be printed, including spaces and punctuation.
‘For Each’ Loop Control
Remember that you can control the flow within a ‘For Each’ loop using ‘break’ and ‘continue’. ‘break’ allows you to exit the loop completely, while ‘continue’ causes the loop to skip the remainder of the current iteration and start the next one right away. Here’s how you might use them:
List<int> numbersControlList = new List<int>{1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; foreach (int num in numbersControlList) { if (num == 5) { break; // Exit the loop completely when num equals 5 } if (num == 3) { continue; // Skip the current iteration when num equals 3 } Console.WriteLine(num); }
In the example above, the ‘For Each’ loop will print numbers from 1 to 4. When it reaches the number 5, it breaks the loop completely and stops execution. The number 3 gets skipped due to the ‘continue’ statement.
Navigating Nested Collections
Navigating nested collections or complex object hierarchies often requires nested ‘For Each’ loops. Suppose you have a list of students, each with a list of assigned grades. To print each student’s grades, you would need nested ‘For Each’ loops:
public class Student { public string Name { get; set; } public List<int> Grades { get; set; } = new List<int>(); } List<Student> students = new List<Student> { new Student { Name = "John", Grades = new List<int>{85, 86, 90} }, new Student { Name = "Sally", Grades = new List<int>{78, 80, 94} }, new Student { Name = "Tom" , Grades = new List<int>{88, 91, 75} } }; foreach (Student student in students) { Console.WriteLine($"Name: {student.Name}"); foreach (int grade in student.Grades) { Console.WriteLine($"\tGrade: {grade}"); } }
The outer ‘For Each’ iterates through the list of students, while the inner ‘For Each’ iterates through each student’s list of grades.
How to Keep Learning
Learning is a continuous journey, and now that you’ve grasped the concept of ‘For Each’ in C#, it’s essential to delve deeper into the world of programming and game development.
With Zenva, you can transition from a beginner to a professional coder, leveraging our broad range of courses that include programming, game development, and AI. Our content spans the needs of all learners, whether you’re just starting off or looking to further enhance your skills. What’s more, we offer over 250 supported courses to aid in propelling your career forward.
Delve Deeper with Unity
For those fond of game development, our Unity Game Development Mini-Degree is a comprehensive set of courses designed to equip you with the skills necessary to build cross-platform games using Unity. Unity is a powerful game engine, employed by both AAA and indie developers worldwide, and offers interesting career opportunities. Our courses are flexible, accessible 24/7, and come with practical coding exercises, quizzes, and challenges to reinforce your learning. Completing these courses can position you to publish your own games, secure employment, and even start your venture.
Check out our broad Unity collection for more. Keep growing, keep learning, and let your coding journey with Zenva open doors to innumerable possibilities.
Conclusion
Your journey of mastering programming in C# and becoming a skilled game developer has just started with understanding the ‘For Each’ loop. The key is to keep practicing, keep building, and understand that the learning process never ends. Whether you are iterating over arrays, lists, or custom collections, ‘For Each’ is a pivotal tool that will always remain useful and advantageous.
Learn more, achieve more. Check out our comprehensive Unity Game Development Mini-Degree to build your proficiency in Unity, C#, and game development. Visit us at Zenva today, and let’s turn that spark of learning into a roaring flame of achievement. We’re here to help you create, build, and realize your dreams in coding and game development.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
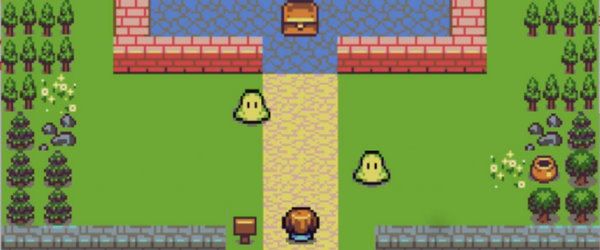
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.