In today’s digital world, becoming proficient in a programming language is equivalent to wielding a powerful tool. When it comes to choice, the C# language stands above many, especially in creating video games. In this tutorial, we’ll explore an integral aspect of C# programming— the wait command. Let’s dive right into it!
Table of contents
What is C# Wait?
The C# ‘wait’ is a command used to delay execution or hold a process for certain periods in a program. It enables the handling of multiple processes and adds a new depth to game mechanics when used appropriately.
Why Should You Learn It?
Mastering the ‘wait’ command in C# opens up new horizons in the realm of game development. It offers a way to create delays in game mechanics, thereby providing programmers with an improved measure of control over game events.
From delaying enemy spawns to timed power-ups, the practical applications of the ‘wait’ command in games are vast and powerful. Learning how to use this feature efficiently will certainly give your games a professional touch.
What Is It For?
The ‘wait’ command primarily serves to introduce pauses or breaks within a program flow. For game developers, this command can be used to control the timing of events, character actions, or even the commencement of a new game level.
Overall, the C# ‘wait’ command is invaluable for creating more dynamic and engaging games, making it a must-learn for all aspiring game developers.
Using Thread.Sleep() to Pause Execution
The most common way to introduce a wait command in C# is to use the `Thread.Sleep()` method, which belongs to the `System.Threading` namespace. This method pauses the execution of the current thread for a specified number of milliseconds.
using System.Threading; class Program { static void Main() { Console.WriteLine("Hello, World!"); Thread.Sleep(2000); // Pauses the program for 2000 milliseconds (or 2 seconds) Console.WriteLine("Goodbye, World!"); } }
Here, “Hello, World!” is first printed to the console. The program then suspends for 2 seconds before printing “Goodbye, World!”.
Using async and await for Asynchronous Delays
Asynchronous programming is yet another way to introduce delays in C#. Using the `async` and `await` keywords, we can execute a task asynchronously.
using System.Threading.Tasks; class Program { static async Task Main() { Console.WriteLine("Hello, World!"); await Task.Delay(2000); // Pauses the program for 2000 milliseconds (or 2 seconds) Console.WriteLine("Goodbye, World!"); } }
This program has the same effect as the earlier example, but it uses the asynchronous method, Task.Delay(), instead of Thread.Sleep(). This is ideal for scenarios where other tasks can be performed while waiting as it does not block the current thread.
Interval Timing with Timer Class
You can also make use of the Timer class in the System.Timers namespace to create repeatable delays at a set interval. The Elapsed event can be raised at this interval.
using System.Timers; class Program { static void Main() { Timer timer = new Timer(2000); // Create a timer that signals the Elapsed event every 2000 milliseconds (2 seconds) timer.Elapsed += OnTimedEvent; // Add event handler for Elapsed event timer.Start(); // Start the timer Console.ReadLine(); // Prevents the program from closing immediately } private static void OnTimedEvent(Object source, ElapsedEventArgs e) { Console.WriteLine("Elapsed event raised at {0}", e.SignalTime); } }
In this example, an event is raised every 2 seconds that prints the time of the event to the console.
Pausing for User Input
Sometimes, we might want to pause the program until the user provides some input. For this purpose, we can use the Console.ReadKey() method.
class Program { static void Main() { Console.WriteLine("Press any key to continue..."); Console.ReadKey(); // Pause until a key is pressed Console.WriteLine("Continuing execution..."); } }
In this scenario, the program prints “Press any key to continue…” and then waits for the user to press a key before printing “Continuing execution…”.
Implementing Countdown
We can also use the `Thread.Sleep()` method to create countdown timers. Let’s see how we can implement a simple countdown.
using System.Threading; class Program { static void Main() { for (int i = 5; i > 0; i--) { Console.WriteLine(i); // Prints the current countdown number Thread.Sleep(1000); // Wait for one second } Console.WriteLine("Go!"); } }
This will start a countdown from 5 to 1, waiting for one second between each number, and finally printing “Go!”.
Creating Delayed Tasks Using Task.Delay()
Similar to Thread.Sleep(), you can also utilize Task.Delay() to create delayed tasks in an asynchronous manner. We demonstrate this below.
using System.Threading.Tasks; class Program { static async Task Main() { Console.WriteLine(await DelayedMessage("Hello after 2 seconds", 2)); } static async Task DelayedMessage(string message, int delayInSeconds) { await Task.Delay(delayInSeconds * 1000); return message; } }
This will create an async task that waits 2 seconds using Task.Delay() and then returns a string message.
Using Stopwatch for Exact Timing
Another beneficial class for controlling timing in C# program is Stopwatch from the System.Diagnostics namespace which can be used to time a section of code.
using System.Diagnostics; class Program { static void Main() { Stopwatch stopwatch = new Stopwatch(); stopwatch.Start(); // Start stopwatch // Run lengthy task for (int i = 0; i < 1000000; i++) { // Perform operations } stopwatch.Stop(); // Stop stopwatch Console.WriteLine("Time elapsed: {0}ms", stopwatch.ElapsedMilliseconds); } }
This code measures the time it takes to execute a loop a million times, showing just how precise the Stopwatch class can be.
Using Timers With Unity Game Engine
In Unity, C#’s ‘wait’ command can be extremely useful in implementing advanced game mechanics. The Unity API offers a more efficient alternative, with its WaitForSeconds real-time and WaitForSecondsRealtime commands that integrate well with its update cycles.
using UnityEngine; public class TimerExample : MonoBehaviour { void Start() { StartCoroutine(WaitAndPrint()); } IEnumerator WaitAndPrint() { yield return new WaitForSeconds(5); print("After 5 seconds"); } }
This Unity-specific code shows the use of `WaitForSeconds` in a coroutine to print a message after waiting for five seconds.
Multiple Async Tasks with Different Delays
Making use of async-await, we can run multiple tasks simultaneously each with different delay times. Let’s take a look.
using System.Threading.Tasks; class Program { static async Task Main() { await Task.WhenAll(new List<Task<T>>() { Task.Delay(2000).ContinueWith((_) => Console.WriteLine("Task 1 complete")), Task.Delay(5000).ContinueWith((_) => Console.WriteLine("Task 2 complete")), Task.Delay(1000).ContinueWith((_) => Console.WriteLine("Task 3 complete")) }); } }
This program makes use of multiple Task.Delay() calls to simultaneously run three tasks with different delays, each printing a message when complete.
Pausing Execution Until a Certain Time
We can also delay execution until a certain future time rather than for a certain number of milliseconds.
using System; class Program { static void Main() { DateTime currentTime = DateTime.Now; DateTime desiredTime = currentTime.AddSeconds(2); // Set the desired time to current time plus 2 seconds while (DateTime.Now < desiredTime) { // Do nothing until the desired time } Console.WriteLine("2 seconds has elapsed"); } }
In the above code, we leverage DateTime to delay console output by 2 seconds.
Delay Execution Until All Tasks Complete
We might need to pause a program until several tasks have completed. This can be achieved elegantly with the Task.WhenAll() method.
using System.Threading.Tasks; class Program { static async Task Main() { var tasks = new Task[3]; tasks[0] = Task.Run(() => { /* perform task 0 operations */ }); tasks[1] = Task.Run(() => { /* perform task 1 operations */ }); tasks[2] = Task.Run(() => { /* perform task 2 operations */ }); await Task.WhenAll(tasks); // Pause until all tasks are complete Console.WriteLine("All tasks are complete"); } }
In this program, Task.WhenAll() will asynchronously wait until all tasks are complete before continuing to execute the subsequent lines of code.
Continue Your Learning Journey with Zenva
If you’ve relished delving into the C# ‘wait’ command and its applications in game development, now is the perfect time to take your skills to new heights. Here at Zenva, we offer a comprehensive range of over 250 courses in programming, game development, and AI to take you from beginner to professional.
One of our most sought-after collections is the Unity Game Development Mini-Degree. This all-encompassing program doesn’t solely focus on C# wait, but it offers a deep dive into using Unity, a popular game engine highly cherished in the industry for its capabilities in creating 2D, 3D, AR, and VR games. With modules covering game mechanics, animations, audio effects, and more, it equips learners with the tools needed to create immersive and interactive game experiences whether you’re a beginner or an experienced developer.
For a more extensive selection on Unity courses, visit our Unity. The pathway to mastering game development and scripting languages could be just a click away. Begin multiplying the effects of your coding efforts by applying what you learned and more from the best at Zenva Academy. Don’t just learn to code, learn to create wonders.
Conclusion
Mastering the C# ‘wait’ command and its application is an essential stepping stone on your journey to becoming an adept game developer. Not only it makes your games feel more polished, but it also opens the door for advancements in your game mechanics. Whether it’s controlling the flow of game events, setting timed tasks, or managing multiple processes, the ‘wait’ command empowers you with an invaluable tool to refine your game design skills.
So, why wait? Explore Zenva’s Unity Game Development Mini-Degree and countless other handy resources we provide to help you improve your prowess in game development and beyond. Remember, the language of code is the brush that paints vibrant, interactive worlds. Begin your journey with Zenva and start building your dream games today.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
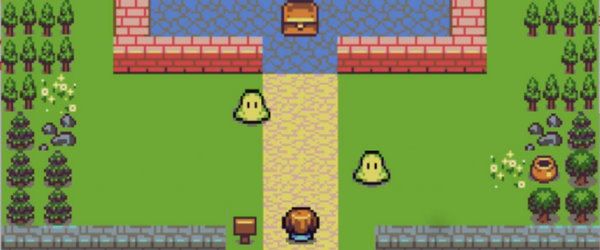
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.