Welcome to a rich and immersive learning experience about C# programming. In this multi-part tutorial, we will venture into the heart of C# code examples around simple game mechanics and analogies. It’s a journey that will be engaging and valuable for both the novice coders and those with some programming experience under their belt.
The motive behind this tutorial is simple: to make C# more accessible, understandable and practical for you. So, whether you are starting your coding journey from scratch or looking to level up your current skills, this comprehensive tutorial is where you need to be.
Table of contents
So, what is C#?
C# pronounced as ‘C-Sharp’, is a general-purpose, object-oriented programming language. Developed by Microsoft, it is used for creating a range of applications, including console applications, robust Windows desktop applications, web services, and games.
But why should I learn it?
Learning C# is an excellent investment for a number of reasons.
- Firstly, C# is one of the most popular programming languages worldwide. Many businesses, especially in the field of game development, choose C# due to its simplicity and flexibility.
- Secondly, considering the gaming industry, C# is the primary language used in Unity – one of the world’s leading real-time game engines.
- Last but certainly not least, learning C# opens many doors to lucrative job opportunities in multiple sectors, from tech to entertainment and beyond.
What can I use it for?
As an extremely versatile language, C# is predominantly used for building professional-grade desktop applications and games. Also, given its role in .NET framework, it’s extensively used to develop Windows applications and web services.
Setting Up Your Environment
To start playing with C#, you’ll need a text editor or an integrated development environment (IDE) where you can write and run your code.
We recommend using Visual Studio for a seamless experience. Here’s how you can get it set up:
- Visit the Visual Studio Download page, and grab the latest community edition, which is free for learning and small project purposes.
- Once the download is complete, initiate the installation process. You will be prompted to select workloads for your project. Check the box that says .NET desktop development.
- Click Install and let Visual Studio do its thing. Once the installation is complete, you’re set to start coding in C#!
A Simple Console Application in C#
Now that you have your development environment, let’s create a new console application.
//Open Visual Studio and select "Create a new project". //In the search box, type "Console" and choose "Console App (.NET Core)" as the template. //Name your project, choose the location and click "Create"!
Your new C# project comes with a template code. Let’s replace it with this:
using System; namespace HelloWorld { class Program { static void Main(string[] args) { Console.WriteLine("Hello Zenva!"); } } }
Press the Start button on your IDE (or F5 on your keyboard) to run the application. Your console should display the message “Hello Zenva!”.
The Basics of C# Syntax
Let’s dive into some of the basics of C# syntax:
Variables and Types
In C#, data can be stored in various types. To declare a variable, you must specify its type:
string name = "Zenva"; int age = 2020; bool isBest = true;
Control Structures
C# includes several control structures such as if-else, switch, and loops. Here are some examples:
// IF-ELSE int temperature = 34; if (temperature < 30) { Console.WriteLine("It's cold."); } else { Console.WriteLine("It's hot."); } // SWITCH string fruit = "Apple"; switch (fruit) { case "Apple": Console.WriteLine("It's an apple."); break; case "Banana": Console.WriteLine("It's a banana."); break; default: Console.WriteLine("It's a mystery fruit."); break; } // WHILE LOOP int i = 1; while (i <= 5) { Console.WriteLine(i); i++; }
Functions
A function is a block of code that performs a specific task. A simple function could look like this:
void SayHello() { Console.WriteLine("Hello Zenva!"); }
To call this function, you simply need to use its name followed by parentheses:
SayHello(); // Outputs "Hello Zenva!"
Functions can also take parameters and return values. Here is an example of a function that takes two numbers, adds them, and returns the result:
int Add(int num1, int num2) { int result = num1 + num2; return result; }
Here’s how you can call this function:
int sum = Add(5, 7); // sum will be 12
Arrays
An array is a special variable that can hold multiple values of the same type. To create an array, you need to declare a variable with square brackets and assign it a new array instance of a certain size:
int[] numbers = new int[5]; // An array of integers with a length of 5
You can set or access the values in an array using an index:
numbers[0] = 10; // Set the first value int firstValue = numbers[0]; // Get the first value
Classes and Objects
C# is an object-oriented programming language, which means it uses classes and objects. A class is a blueprint for an object, and an object is an instance of a class. Here’s a simple class definition:
class Dog { public string name; public int age; public void Bark() { Console.WriteLine("Woof!"); } }
To create an object of this class, you need to use the new keyword:
Dog myDog = new Dog();
Once an object is created, you can access its members using the dot operator:
myDog.name = "Rex"; myDog.age = 5; myDog.Bark(); // Outputs "Woof!"
Data Structures: Lists and Dictionaries
While arrays are useful, they have limitations – their size is fixed. In C#, we use Lists and Dictionaries as more flexible counterparts.
A List is a collection of items, similar to an array but with a dynamic size. Here’s how you can create and use a List:
// Create a new list List<string> names = new List<string>(); // Add items to the list names.Add("Peter"); names.Add("Paul"); names.Add("Mary"); // Remove an item from the list names.Remove("Paul"); // Count the items in the list int count = names.Count; // count will be 2
A Dictionary is a collection of key-value pairs. Here’s an example of how to use a Dictionary:
// Create a new dictionary Dictionary<string, int> ages = new Dictionary<string, int>(); // Add items to the dictionary ages.Add("Peter", 30); ages.Add("Paul", 35); // Remove an item from the dictionary ages.Remove("Peter"); // Get a value from the dictionary int paulAge = ages["Paul"]; // paulAge will be 35
Working with Files
The System.IO namespace in C# has various classes that help you work with files. Here’s how you can create a new file and write text to it:
using System.IO; string path = @"C:\Temp\MyFile.txt"; // Write text to the file File.WriteAllText(path, "Hello Zenva!"); // Read text from the file string text = File.ReadAllText(path);
We’ve also included a common operation, reading from a file, in the code block above!
Exception Handling
When an error occurs during program execution, C# throws an exception. When an unhandled exception is thrown, your program stops running. However, you can handle exceptions using try-catch blocks. Here’s how:
try { int result = 10 / 0; } catch (DivideByZeroException ex) { Console.WriteLine(ex.Message); // Outputs "Attempted to divide by zero." }
In the catch block, you can provide a tailored message to the user, log the error or perhaps try an alternative approach to solve the problem.
These were some basic – yet powerful – elements of C#. Once mastered, you’ll be on your way to becoming a fearsome C# programmer who can navigate complex software or game development with ease.
We believe in making learning an interactive, fun and enriching experience. We can’t wait to see how you use these C# basics to build some fantastic applications! Happy coding, and stay tuned for more tutorials.
Where to Go Next: Continuing Your Journey
Having conquered the basics of C# programming, it’s natural to wonder, “Where do I go next?”. An exciting continuation of your journey could be delving into the world of game development with Unity. This is where C# shines the brightest, and where your potential is just waiting to be unlocked.
To ease your next steps, we invite you to explore our Unity Game Development Mini-Degree. A comprehensive collection of courses, it takes you from the fundamentals of Unity to in-depth topics like AI, animations, storytelling and more. Once you complete these courses, you’ll have a strong portfolio of Unity games and projects under your belt.
But, if you’re looking for a wider ocean to swim in, check out our more extensive selection of courses on Unity. Whatever route you choose, Zenva offers over 250 courses that take you from beginner to professional, empowering you to learn coding, create games and earn certificates along the way. Keep progressing, keep learning and you’ll soon realize the world is an open book of programming for you!
Conclusion
Whether you come from a non-coding background or are a seasoned programmer looking to expand your skills, C# is a fantastic language to add to your arsenal. It is a key player in the software and game development sectors and serves as the magic wand in creating some of the most exciting, immersive games in Unity.
With Zenva, you’re never alone on your learning journey. Our industry-leading content empowers you to rise above challenges and achieve your goals. Ready to significantly upgrade your skills and open a world of possibilities? Take our Unity Game Development Mini-Degree now, and let’s turn your programming dreams into reality!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
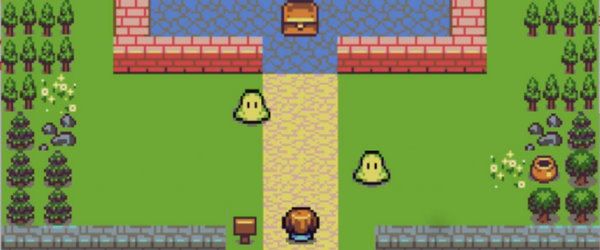
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.