Table of contents
What is ‘C# foreach’?
‘Foreach’ is a loop construct found in C#. Much like the ‘for’ loop, it allows you to iterate through a collection or an array. However, ‘foreach’ offers a simpler and more readable syntax, making it easier to work with, especially for beginners.
What is it used for?
A ‘foreach’ loop in C# is used when you want to go through each element in an array or collection. Imagine you’re coding a game where each avatar collects coins. With ‘foreach’, you can easily go through each avatar and add or deduct coins based on their performance!
Why should you learn it?
Learning ‘foreach’ in C# has several benefits:
- Streamlines your coding process – eliminating the need to keep track of the index in an array.
- Makes you code more readable and cleaner for other developers to understand.
- In gaming, it can be used to perform actions on every item in a player’s inventory or every enemy in a level.
In this tutorial, we aim to present ‘C# foreach’ in an engaging, accessible way, packed with real-world examples that breathe life into coding concepts. Ready to start? Let’s get coding!
Creating a Simple foreach Loop
The ‘foreach’ loop in C# allows you to iterate over elements in an array or collection. Let’s start with a basic example of creating a ‘foreach’ loop to print all elements of an array.
string[] fruits = {"apple", "banana", "cherry"}; foreach (string fruit in fruits) { Console.WriteLine(fruit); }
In the code snippet above, the ‘foreach’ loop is used to go through all the elements of the ‘fruits’ array and print each one to the console.
Using foreach with Lists
‘Foreach’ doesn’t just work with arrays, you can use it with any collection type. Here’s how you can use ‘foreach’ with a List in C#.
List<string> fruits = new List<string>() {"apple", "banana", "cherry"}; foreach (string fruit in fruits) { Console.WriteLine(fruit); }
In this case, ‘foreach’ is used to iterate over the elements of the List ‘fruits’.
Working with Complex Types
‘Foreach’ isn’t limited to simple data types, you can also use it with complex types. Here’s an example of how to use ‘foreach’ with a List of custom objects.
public class Fruit { public string Name { get; set; } public string Color { get; set; } } List<Fruit> fruits = new List<Fruit>() { new Fruit(){Name="apple", Color="red"}, new Fruit(){Name="banana", Color="yellow"}, new Fruit(){Name="cherry", Color="red"} }; foreach (Fruit fruit in fruits) { Console.WriteLine($"Name: {fruit.Name}, Color: {fruit.Color}"); }
The loop iterates over the ‘fruits’ List, and for each Fruit object, it prints the name and the color properties.
foreach in Multidimensional arrays
Let’s take a look at how to use the ‘foreach’ loop with a multidimensional array in C#.
int[,] numbers = { { 1, 2, 3 }, { 4, 5, 6 }, { 7, 8, 9 } }; foreach (int number in numbers) { Console.Write(number + " "); }
In this case, ‘foreach’ is used to iterate over all elements of a multidimensional array.
Breaking Out of a foreach Loop
While ‘foreach’ is designed to go through each item in a collection, there might be cases where you want to break out of the loop before it completes. For example, you may want to stop the loop once a specific item is discovered. Here’s how you can do it.
string[] fruits = {"apple", "banana", "cherry"}; foreach (string fruit in fruits) { if (fruit == "banana") { break; // Stops the loop } Console.WriteLine(fruit); }
The loop breaks and stops printing once it encounters the element “banana” in the array.
Using Multiple foreach Loops
In certain cases, you may need to use multiple ‘foreach’ loops. Let’s assume you have a list of lists (a common scenario in game development for a map grid or a multi-story level), you would first iterate over the outer list, then run another ‘foreach’ loop for each inner list.
List<List<string>> mapGrid = new List<List<string>>() { new List<string>() { "grass", "grass", "water" }, new List<string>() { "tree", "grass", "grass" }, new List<string>() { "water", "water", "grass" } }; foreach (List<string> mapRow in mapGrid) { foreach (string mapCell in mapRow) { Console.Write(mapCell + " "); } Console.WriteLine(); }
This will correctly print out the contents of each list within the ‘mapGrid’ list.
Continuing a foreach Loop
The ‘continue’ keyword can be used in a ‘foreach’ loop to skip the current iteration and move on to the next item in the loop. Below is a simple example of a ‘foreach’ loop using ‘continue’ to bypass an element.
string[] fruits = {"apple", "banana", "cherry"}; foreach (string fruit in fruits) { if (fruit == "banana") { continue; // Skips the loop on encountering "banana" } Console.WriteLine(fruit); }
This loop will print all elements in the array except for “banana”.
Combining foreach with if-else logic
‘Foreach’ can also be combined with if-else logical statements. This is useful when you need to perform different actions based on the element value. Here is a ‘foreach’ loop combined with some simple logic:
string[] fruits = {"apple", "banana", "cherry"}; foreach (string fruit in fruits) { if (fruit == "banana") { Console.WriteLine(fruit + " is yellow"); } else { Console.WriteLine(fruit + " is not yellow"); } }
This code will print a different message based on whether the fruit is a banana or not.
Adding Elements to a Collection using foreach Loop
It’s essential to note that ‘foreach’ is not suitable for modifying a collection you are iterating over. Adding elements to a collection within a ‘foreach’ loop will result in an InvalidOperationException runtime error.
Here’s how an unsuccessful attempt to add an element to a collection using ‘foreach’ would look:
List<string> fruits = new List<string>() {"apple", "banana", "cherry"}; foreach (string fruit in fruits) { if (fruit == "banana") { fruits.Add("mango"); // This will cause an error } Console.WriteLine(fruit); }
An Alternative Way of Modifying a Collection using for Loop
If you must modify a collection while you iterate over it, use a ‘for’ loop instead.
Here is an example on how to add a new element to an array using ‘for’ loop:
string[] fruits = { "apple", "banana", "cherry" }; for (int i = 0; i < fruits.Length; i++) { if (fruits[i] == "banana") { Array.Resize(ref fruits, fruits.Length + 1); fruits[fruits.Length - 1] = "mango"; } Console.WriteLine(fruits[i]); }
Using foreach on Dictionary
You can use ‘foreach’ to iterate through both keys and values in a Dictionary. Here’s how:
Dictionary<string, string> fruitColors = new Dictionary<string, string>() { { "apple", "red" }, { "banana", "yellow" }, { "cherry", "red" } }; foreach (KeyValuePair<string, string> entry in fruitColors) { Console.WriteLine($"The {entry.Key} is {entry.Value}"); }
In this snippet, ‘foreach’ is used to print both the fruit and its associated color from a dictionary.
Using foreach on Collections implementing IEnumerable Interface
The ‘foreach’ loop can also iterate over any custom collection type that implements the IEnumerable interface. Here’s how:
public class FruitCollection : IEnumerable { private List<string> _fruits = new List<string>() { "apple", "banana", "cherry" }; public IEnumerator GetEnumerator() => _fruits.GetEnumerator(); } FruitCollection fruitCollection = new FruitCollection(); foreach (string fruit in fruitCollection) { Console.WriteLine(fruit); }
In this code, ‘FruitCollection’ is a custom class that implements ‘IEnumerable’. ‘foreach’ is then used to iterate over instances of this class.
What’s the Next Step?
You’ve now got a foundation of knowledge in ‘foreach’ in C#! But remember, learning is a lifelong journey, especially in the ever-evolving world of tech and game development. The key to success here is consistency and continuous learning.
At Zenva, you can keep enhancing your skills and dive deeper into the realm of game development with our Unity Game Development Mini-Degree. This comprehensive collection of courses offers extensive insight into how you can leverage Unity – one of the world’s most well-known game engines – to create your own games. Covering a range of topics such as game mechanics, audio effects, animations, and more, you’ll learn by doing with our project-based curriculum. Uniity’s application spans beyond just gaming, it’s used in various other industries such as automotive, healthcare, education and more, so your learning can open numerous doors in the tech industry.
For a wider selection of courses, you can also browse through our Unity collection. We offer over 250 supported courses and by learning with us, you’ll join a community of learners who have used their newfound skills to boost their careers. Remember, with Zenva, you can go from beginner to professional. So don’t stop here. Continue learning, continue creating, and keep that dev flame alive!
Conclusion
Understanding ‘foreach’ in C# empowers you to write cleaner, more readable code – an invaluable skill in game development and beyond. Whether you’re making your own games with Unity or building applications with C#, loops are an essential aspect of programming.
Here at Zenva, we continue to provide high-quality content, tutorials and courses to keep the learning journey fascinating. We believe in transforming beginners into professionals, one concept at a time. So, keep exploring, keep learning, and remember – the only way to get better at coding is to code!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
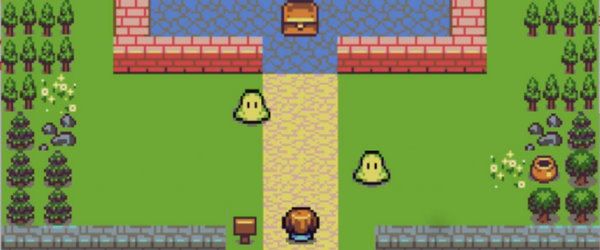
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.