Welcome to this comprehensive tutorial on how we can convert XML files into C# data structures. This often-overlooked skill is an extremely useful tool in every developer’s arsenal, particularly when building data-intensive applications or games. Let’s dive right in and learn the ins and outs of XML to C#.
Table of contents
What is XML to C# Conversion?
XML – Extensible Markup Language – is commonly used when data needs to be shared or stored. With its structured and organized format, it allows us to store valuable pieces of data and deliver it across platforms. Azure data solutions, Android mobile applications, and even game development scenarios heavily utilize XML.
On the other hand, C# (pronounced as C Sharp) is an object-oriented, type-safe, simple, versatile and powerful programming language developed by Microsoft. It is used in a ton of applications itself, mainly focusing on Windows application, game development using Unity, and many others.
Why Should We Learn It?
Learning the conversion process from XML to C# is critical for a couple of reasons:
- It allows us to extract XML data and use it within in our application, powering game mechanics, user interfaces, and system integrations.
- It introduces us to working with data – a critical part of modern application development and a stepping stone towards other areas such as databases or network communications.
Armed with the knowledge and ability to convert XML to C#, we enhance our all-around developer skills and take our projects to the next level.
Basic XML to C# Conversion
We’ll start with the basics – converting a simple XML file into C#. Here’s an example of an XML file:
<Course> <ID>1</ID> <Name>Intro to C#</Name> </Course>
First, we need to prepare the C# data structure equivalent. Here’s how you can create it:
public class Course { public int ID { get; set; } public string Name { get; set; } }
Now let’s write a piece of code that will deserialize the XML data into the C# data structure:
XmlSerializer serializer = new XmlSerializer(typeof(Course)); StreamReader reader = new StreamReader("course.xml"); Course course = (Course)serializer.Deserialize(reader); reader.Close();
Once completed, we have transferred our XML data into a C# object known as ‘course’. This conversion allows us to interact with the XML data meaningfully within our application.
XML to C# Conversion with an XML Document
What if our XML file has more complexity? Suppose we have a list of courses each having their ‘ID’ and ‘Name’? Here’s how such an XML file could look:
<Courses> <Course> <ID>1</ID> <Name>Intro to C#</Name> </Course> <Course> <ID>2</ID> <Name>Mastering XML</Name> </Course> </Courses>
We need to alter our C# data structure to accommodate a list of courses. Here’s how this can be done:
public class Course { public int ID { get; set; } public string Name { get; set; } } public class Courses { public Course[] Course { get; set; } }
The deserialization code remains similar as the earlier scenario:
XmlSerializer serializer = new XmlSerializer(typeof(Courses)); StreamReader reader = new StreamReader("courses.xml"); Courses courses = (Courses)serializer.Deserialize(reader); reader.Close();
We have now achieved our goal – converted a list of XML courses data into a C# object.
Handling Attributes in XML to C# Conversion
XML data can also contain attributes within its tags. Here is an XML structure reflecting such data:
<Course ID="1"> <Name>Intro to C#</Name> </Course>
To handle this attribute in C#, we need to slightly modify our class structure:
public class Course { [XmlAttribute] public int ID { get; set; } public string Name { get; set; } }
Here, the [XmlAttribute]
informs the deserializer that this element is an attribute. The deserialization code remains identical to the previous examples.
XML to C# with XML Namespaces
Sometimes, dealing with XML namespaces can be complicated for beginners. Here we have an XML file containing namespaces:
<Course xmlns="http://www.zenva.com"> <ID>1</ID> <Name>Intro to C#</Name> </Course>
We need to acknowledge the XML namespaces while creating our C# classes:
[XmlRoot(Namespace = "http://www.zenva.com")] public class Course { public int ID { get; set; } public string Name { get; set; } }
In the above code, the [XmlRoot]
attribute is notifying the deserializer about the root of the XML document and the corresponding XML namespace. This allows seamless conversion of our XML file with a namespace into the C# data structure.
Conclusion
As we have seen, converting XML to C# data structures is a powerful way to handle data in many project scenarios. While there are more complexities that can come up while parsing XML files, the basics taught here will give you a strong starting point.
By grasping these fundamentals, we at Zenva encourage you to experiment, build, and enhance your coding journey. Remember, practice is key to mastering anything – so roll up your sleeves, fire up your IDE, and start practicing!
Advanced XML to C# Conversion Scenarios
Let’s venture into some more complex XML structures and their counterparts in C#. Understanding these scenarios will equip you to handle diverse data structures that may come your way.
XML to C# Conversion with Child Elements
XML files can also contain child elements within the tags. For instance:
<Course> <ID>1</ID> <Name>Intro to C#</Name> <Chapter>Getting Started</Chapter> <Chapter>Basics</Chapter> </Course>
To map the XML structure to C#, we will create two classes:
public class Course { public int ID { get; set; } public string Name { get; set; } [XmlElement("Chapter")] public List Chapters { get; set; } }
By using the [XmlElement]
attribute tagging on the list of chapter strings, we advise the deserializer that these elements within the XML should be mapped to the ‘Chapters’ list in the C# structure.
XML with Different Names for Elements and Attributes
XML code may not always align with the C# class property names. In such a case, utilize the [XmlElement]
and [XmlAttribute]
tags to help align XML and C# structures:
<Course> <id>1</id> <title>Intro to C#</title> </Course>
Here, the C# class structure can be aligned as follows:
public class Course { [XmlElement("id")] public int ID { get; set; } [XmlElement("title")] public string Name { get; set; } }
The process of aligning names between XML and C# using these attributes is known as ‘name mapping’.
Ignoring Elements in XML to C# Conversion
There could be instances where you might not require all the data present in an XML document. For instance, in the below XML, we might not need the ‘Duration’ information:
<Course> <ID>1</ID> <Name>Intro to C#</Name> <Duration>30</Duration> </Course>
We can ignore this in our C# class using the [XmlIgnore]
attribute:
public class Course { public int ID { get; set; } public string Name { get; set; } [XmlIgnore] public int Duration { get; set; } }
The deserializer will now ignore the ‘Duration’ from the XML data while converting to the C# object.
In this part of our tutorial, we covered advanced scenarios and dug deeper into transforming XML data into C# objects.
These problem-solving strategies can be widely applied in many application and game development scenarios. Stay tuned for more informative tutorials! As we always say at Zenva, happy coding!
What’s Next?
Well done on reaching this far! Our journey into learning how to convert XML to C# data structures has surely opened up new possibilities for better managing and utilizing data within applications.
If you’re looking to go beyond, why not broaden your skills and learn how to create games? We encourage you to check out our Unity Game Development Mini-Degree. This comprehensive collection of courses covers game development with Unity, one of the most widely-used and powerful game engines. You’ll dive deep into various aspects of game development, including game mechanics, animation, and audio effects. It offers adaptability for both beginners and more experienced developers.
Or if you want to explore a broader collection, head onto our Unity courses incorporating an expansive selection of learning materials and resources. At Zenva, whether you’re a beginner or a professional wanting to update your skills, we have a coding solution for you. So why wait? Let’s continue learning, continue coding, and achieve your ultimate career goals!
Conclusion
Transforming XML data into usable C# objects opens multiple avenues for developers. It helps to power up game mechanics, system integrations, and user interfaces. With the fundamentals in hand, the road ahead is clear to tackle complex scenarios and larger data sets. The knowledge gained allows us to respond flexibly and effectively to various challenges in our coding journey.
At Zenva, we believe in empowering individuals with the tools they need to create, innovate and bring their thoughts to life, one line of code at a time. Our Unity Game Development Mini-Degree is one such step toward achieving that goal. Stick with us to stay updated on the latest trends in coding, game creation, and much more! Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
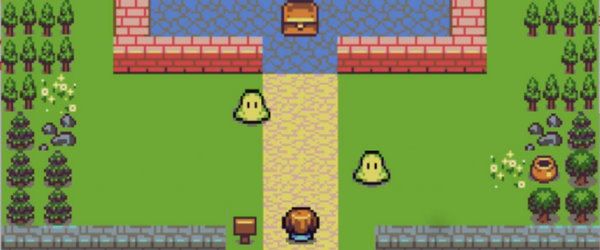
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.