Welcome to another Zenva tutorial, where we aim to make programming concepts as understandable as possible, enriching your journey in the coding world. Today, we dive into a very useful feature in C#, the “any” method.
Table of contents
Unlocking the any() Method
The “any” method is a built-in functionality in the C# LINQ (Language Integrated Query) library. LINQ offers a plethora of operations to deal with collections of data in a quick, clean, and efficient manner.
Why Use the any() Method?
With “any”, you can check whether any elements in a collection meet a specific condition. It returns a boolean value – “true” if at least one element in the collection satisfies the provided condition, and “false” if otherwise. This eliminates a lot of manual processes, saving coding time, achieving cleaner code, and enhancing the efficiency of your program.
How Does It Boost My Game Development Skills?
Imagine you’re developing a role-playing game where characters have inventories. Using the “any” method, you can easily check if any of your characters possesses a particular item, all in one simple line of code.
Why Should I Learn It?
Every programmer aims to write code that’s clean, efficient and easy to maintain. Learning how to use built-in methods such as “any” elevates your code to that professional level. Plus, it’s a beneficial tool for game design, making inventory checks, player movements, and other similar feature implementations a breeze.
Let’s put these words into action in the next section by exploring some beginner-friendly code examples.
The Basics of Using the any() Method
The generic syntax for using the any() method in C# is as follows:
collectionName.any(Boolean condition)
Let’s apply this syntax to an example:
List<int> listOfNumbers = new List<int>() { 1, 2, 3, 4, 5 }; bool containsEvenNumber = listOfNumbers.Any(number => number % 2 == 0); Console.WriteLine(containsEvenNumber); // Outputs: "True"
In the code snippet above, we created a list of integers and we use any() method to check if there’s an even integer in the list. Yes, there is, hence it returns “true”.
Applying the any() Method in Game Development
Here’s a more realistic game development example, involving a list of characters and their inventory that contains a specific item.
class Character { public string Name { get; set; } public List<string> Inventory { get; set; } } List<Character> characterList = new List<Character>() { new Character() { Name = "Hero", Inventory = new List<string>() { "Sword", "Shield" }}, new Character() { Name = "Mage", Inventory = new List<string>() { "Staff", "Potion" }} }; bool containsSword = characterList.Any(character => character.Inventory.Contains("Sword")); Console.WriteLine(containsSword); // Outputs: "True"
In this example, the characters in the game have an inventory of items. The “any” method helps to quickly check if any character has a “Sword” in their inventory, which makes it effortless to implement a quick inventory check for any item during gameplay.
Diving Deeper into the any() Method
You can also use any() without providing any condition, just to check if the collection has any element at all:
List<int> listOfNumbers = new List<int>(); bool hasElements = listOfNumbers.Any(); Console.WriteLine(hasElements); // Outputs: "False"
In this example, the list is empty, so the any() call returns “false”. This is quite useful to either affirm the existence of items in a collection or managing scenarios where you might encounter an empty collection.
any() Method with Different Data Types
The beauty of the any() method is that it’s not bounded by any specific data type. Let’s explore its usage with some different data types.
String Array
Checking if a string array contains any empty string:
string[] nameArray = { "John", "Marry", "", "David" }; bool containsEmptyString = nameArray.Any(name => string.IsNullOrEmpty(name)); Console.WriteLine(containsEmptyString); // Outputs: "True"
In this scenario, the any() method verifies if there’s any empty string in the array and found one, hence the output is “true”.
Dictionary
With a Dictionary, you can check for the existence of a specific key-value pair:
Dictionary<string, int> fruitBasket = new Dictionary<string, int>() { {"apple", 5}, {"banana", 7}, {"pear", 3}}; bool containsMoreThan5Bananas = fruitBasket.Any(basket => basket.Key == "banana" && basket.Value > 5); Console.WriteLine(containsMoreThan5Bananas); // Outputs: "True"
Essentially, it confirms that there are more than 5 bananas in the fruit basket, hence returning “true”.
Dealing with Complex Cases
When dealing with more complex cases, using any() method properly is vital. Consider the case of a game where characters have different skill levels in different areas:
class Character { public string Name { get; set; } public Dictionary<string, int> Skills { get; set; } } List<Character> characterList = new List<Character>() { new Character() { Name = "Hero", Skills = new Dictionary<string, int>() { { "Strength", 10 }, { "Speed", 8 }}}, new Character() { Name = "Mage", Skills = new Dictionary<string, int>() { { "Magic", 10 }, { "Strength", 3 }}} }; bool anyCharacterHasHighStrength = characterList.Any(character => character.Skills.Any(skill => skill.Key == "Strength" && skill.Value > 8)); Console.WriteLine(anyCharacterHasHighStrength); // Outputs: "True"
In the above example, it recognizes that the “Hero” character has a strength value of over 8, so the “any” condition is true.
Conclusion
The any() method in C# brings substantial value in handling the simplest to the most complex collection data types. From ensuring cleaner code to optimizing the functionality of your programs, integrating the any() method into your code is definitely a skill worth mastering.
At Zenva, we are passionate about making coding as accessible and digestible as possible. We hope this tutorial has shed light on the any() method in C# and its practical applications, particularly in game design. Stick with us as we continue to unravel more inspiring and beneficial programming concepts, and keep coding!
Enhancing Your Programming Skills with any() Method
Understanding the any() method and its applications is one thing, putting it to use across different scenarios is another. Let’s further immerse ourselves in more examples to solidify our understanding and application of the any() method.
Lists of Class Instances
Imagine you’re building a game and you want to ascertain if any character has achieved a certain level. Here’s how that’s done:
class Character { public string Name { get; set; } public int Level { get; set; } } List<Character> characterList = new List<Character>() { new Character() { Name = "Hero", Level = 3}, new Character() { Name = "Mage", Level = 2} }; bool hasLevel3Character = characterList.Any(character => character.Level == 3); Console.WriteLine(hasLevel3Character); // Outputs: "True"
This code makes a quick inventory check to see if any of the characters has reached level 3. The result is true, hence confirming that a character(s) has achieved that level.
Integrating Negation
The any() method can be combined with a negation operator to check if no elements in a collection meet a specific condition. Let’s apply this with a List of integers:
List<int> listOfNumbers = new List<int>() { 1, 2, 3, 4, 5 }; bool containsNoZero = !listOfNumbers.Any(number => number == 0); Console.WriteLine(containsNoZero); // Outputs: "True"
This example checks whether the list of numbers doesn’t contain any zeros. Since there are no zeros in the list, the output is “true”.
any() with Structures
The any() method can seamlessly be used with custom structures as well. Review this example involving a simple structure representing a person:
struct Person { public string FirstName; public int Age; } List<Person> peopleList = new List<Person>() { new Person() { FirstName = "John", Age = 25}, new Person() { FirstName = "Steven", Age = 30} }; bool containsPersonNamedJohn = peopleList.Any(person => person.FirstName == "John"); Console.WriteLine(containsPersonNamedJohn); // Outputs: "True"
This bit of code checks if any person in the list is named “John”. So yes, there is a person named “John”, and it outputs “true”.
any() with Jagged Arrays
Nested arrays or jagged arrays can benefit significantly from the any method. Take this example:
int[][] jaggedArray = new int[][] { new int[] {1, 2, 3, 4}, new int[] {5, 6, 7, 8} }; bool containsNumber4 = jaggedArray.Any(innerArray => innerArray.Any(number => number == 4)); Console.WriteLine(containsNumber4); // Outputs: "True"
This checks if any number in the jagged array equals 4. By confirming that, it outputs “true”.
any() with 2D Arrays
Let’s take a look at another complex usage of the any() method with a two-dimensional array:
int[,] array2D = new int[2, 2] { { 1, 2 }, { 3, 4 } }; bool containsNumber4 = Enumerable.Range(0, array2D.GetLength(0)).Any(i => Enumerable.Range(0, array2D.GetLength(1)).Any(j => array2D[i, j] == 4)); Console.WriteLine(containsNumber4); // Outputs: "True"
This code first generates a range for each dimension of the 2D array and then uses any() to check if the number 4 is present anywhere in the array. It finds the number 4 and thus returns “true”.
Much like a swiss army knife, the any() method is a diverse tool that simplifies otherwise complex operations in C# programming. By providing more efficient, cleaner coding practices, it makes our code easier to read, understand and maintain – all crucial qualities to enhance your programming journey.
We hope these examples better illustrate the versatility and adaptability of the any() method in C#, and showcases its practical uses in various game development scenarios. Keep exploring these functionalities and more with Zenva as we continually simplify the world of coding.
Spread Your Coding Wings Further
Having grasped the power of the any() method and its applications in C#, it’s time to delve deeper and explore the endless possibilities that coding provides. One of the best ways to proceed is to arm yourself with the ability to create games, the key to which lies in mastering Unity, a leading game development engine.
We, at Zenva, invite you to enroll in our Unity Game Development Mini-Degree. This comprehensive collection of courses is designed to provide a solid introduction to Unity and its use in game development, offering a hands-on, project-based way of learning. It delves into varying aspects of game mechanics, cinematic cutscenes, audio effects, and AI. Not only does this suit beginners, but it also caters to experienced developers aiming to further their Unity prowess.
With Unity under your belt, the gaming world is your oyster, opening up numerous lucrative opportunities in various industries. There’s a broader range of courses to check out in our Unity Collection as well. At Zenva, our aim is to enhance your coding journey from beginner to professional, offering over 250 supported courses. Now, all it takes is for you to take the leap and keep learning!
Conclusion
Demystifying the any() method in C# creates a space where the seemingly complex becomes powerfully simple. Its multiple coding applications, ranging from cleaner code to more efficient programs, constitute an indispensable arsenal for growth and professional progression in game development and beyond.
Let’s continue this learning journey together, to strengthen our skills, broaden our knowledge, and fulfil our endless potential. Our Unity Game Development Mini-Degree awaits you, with world-class content geared towards giving you a head-start in the ever-evolving world of game development. The future of coding unfolds her wings before you, and with Zenva, you’re poised to take flight.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
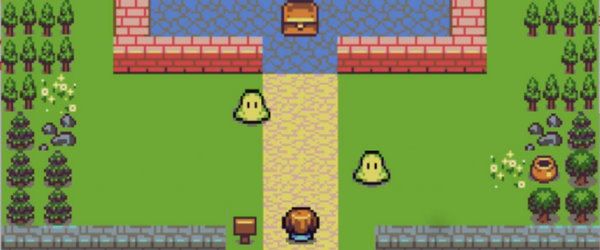
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.