Welcome to our tutorial on literals in programming—entrance tickets to the vast amusement park of coding, where each ride and attraction operates on the values you provide. Just as tokens represent a set number of rides, literals represent fixed values in code––without them, programs would lack the fundamental data to perform operations or make decisions. This makes understanding literals a core skill for both aspiring and experienced developers, across any language or platform. By mastering this concept, you’ll be able to input data into your programs and understand the constants that you’ll use in variables and expressions.
Table of contents
What Are Literals?
Literals are the notations for representing fixed values directly in your source code. They are the raw values that are built into the syntax of programming languages and are used to initialize variables or constants. Stepping through the garden of programming, think of literals as the seeds sown into the soil—germinating into the variables that grow and change throughout your code’s logic.
Why Are Literals Important?
In the landscape of programming, literals are like the bedrock upon which more complex expressions and data manipulations are built. They are the starting points and references that give meaning and context to the computations that programs perform. Learning about literals is crucial because:
– They help create more readable and maintainable code.
– They are used to assign values that do not change (constants) or to start a variable’s life with an initial value.
– They enable developers to quickly understand what kind of data a program will work with.
What Are They Used For?
From the coordinates in a video game to the initial scores in a scoreboard app, literals set the stage for the action to unfold. They are involved in:
– Assigning values to variables
– Defining constant values that serve as configuration or reference points
– Being passed as arguments to functions and methods
– Setting up arrays, lists, and other data structures with initial values
Join us as we navigate through the concept of literals, detailing their types and uses with engaging examples. This journey is meant for all—from those just starting their coding adventure to seasoned programmers polishing their foundational knowledge. Let’s unleash the power of literals and elevate our programming prowess together!
Types of Literals in Programming
In programming, different literals correspond to various types of data. Whether you’re working with whole numbers, precise measurements, text, or logical conditions, choosing the right literal is key to providing clear instructions to the computer. Let’s explore some fundamental literal types with examples to illuminate their implementation.
Numeric Literals can be further divided into integers and floating-point literals.
// Integer literal int myAge = 30; // Floating-point literal double piValue = 3.14159;
String Literals represent sequences of characters and are often enclosed in quotes.
// String literal String greeting = "Hello, Zenva students!";
Boolean Literals are the simplest form, representing true or false values.
// Boolean literal for a condition check boolean isGameRunning = true; // Boolean literal for a feature toggle boolean isFeatureEnabled = false;
Character Literals are single characters surrounded by single quotes. They’re different from strings, which are sequences of characters.
// Character literal char initialLetter = 'Z';
Null Literal is used in many programming languages to represent the absence of a value or a non-existent object.
// Null literal String noValueYet = null;
Array Literals are used to initialize arrays. They are enclosed in braces and separate elements with commas.
// Array literal with integer values int[] numberArray = {1, 2, 3, 4, 5}; // Array literal with string values String[] colorArray = {"red", "green", "blue"};
Understanding these types of literals will help you write clearer and more explicit code. They are the constants, the dependable elements in your programming toolbox that keep your code grounded and functional.
Literal Values in Conditional Statements and Loops
Literals are also commonly used in conditional statements and loops. Here, they can define the conditions under which certain blocks of code execute, or they determine the number of iterations that loops perform.
// Using boolean literals in an if statement if (true) { System.out.println("This will always print."); } // Using a numeric literal in a loop for (int i = 0; i < 10; i++) { System.out.println("This will print 10 times."); }
Literals allow you to quickly alter the behavior of these statements during the early stages of development without needing to declare and initialize variables.
As we continue, you’ll see more tailored examples that incorporate a variety of literal types within programming constructs. This will not only cement your understanding of literals but also their practical applications in real-world scenarios. Let’s keep building the foundation to write elegant and efficient code!
Practical Applications of Literals
Diving deeper into the world of code, we find that literals are essential ingredients in many programming recipes. From declaring default values to specifying parameters for method calls, their presence is ubiquitous. Here are some practical examples that illustrate the varied uses of literals.
Setting Default Values
When defining variables, we can assign default values using literals, providing a clear starting point for later operations.
// Default integer value int defaultScore = 0; // Default floating-point value double temperature = 98.6;
Control Structures
Literals are indispensable when creating control structures such as conditional statements and loops. They define the explicit conditions and limits within these structures.
// Conditional statements with literals if (userInput == 1) { System.out.println("Option 1 selected."); } // For loop with a numeric literal in its condition for (int counter = 0; counter < 5; counter++) { System.out.println("Loop iteration " + counter); }
Calculations
For calculations, literals can be used to specify values that are to be operated on. It’s a direct way to insert numbers or other data into mathematical expressions or business logic.
// Simple arithmetic with literals int total = 50 + 25; // Expected: 75 // Using literals in a more complex expression double area = 3.14 * (radius * radius); // Assuming 'radius' is a previously declared variable
Function and Method Arguments
When invoking functions or methods, literals provide the actual parameters. These are the concrete values your methods will operate on.
// Method call with string literal for logging logMessage("Starting the application..."); // Function call with literals as arguments for calculation double distance = calculateDistance(0.0, 0.0, 5.0, 5.0);
Array and Object Initialization
When creating data structures or objects, literals can be used to set initial values.
// Initializing an array of numbers int[] fibonacciSeries = {0, 1, 1, 2, 3, 5, 8, 13, 21, 34}; // Creating an object with literals for property values Person newEmployee = new Person("Jane", "Doe");
Best Practices with Literal Values
While literals are incredibly useful, they should be employed with forethought and care. Here are some best practices to consider when using literals in your code.
Avoid Magic Numbers
Literal values in the middle of code, commonly known as “magic numbers” (or strings), can make understanding the code’s purpose difficult. Instead, use named constants to clarify their meaning.
// Instead of magic numbers: double circumference = 2 * 3.14159 * radius; // Use a named constant for the literal value: final double PI = 3.14159; double circumference = 2 * PI * radius;
Use Enumerations for Sets of Related Constants
When dealing with a set of related constant values, consider using an enumeration. This groups the literals in a meaningful way, increasing readability and maintainability.
enum Direction { NORTH, SOUTH, EAST, WEST } // Usage Direction myDirection = Direction.NORTH;
Documentation Comments
Always document the purpose of literals, especially if their meaning isn’t immediately clear from the context. Comments become essential in understanding why a particular value is used.
// Good documentation for a literal final int MAX_LOGIN_ATTEMPTS = 5; // Maximum allowed attempts before locking the account
Literals may seem simple, but their correct application is powerful. Follow these practices to keep your code clear and understandable. Remember, each literal value you place is like carefully choosing the bricks for your programming foundation—one that supports the elegant architectures you’ll build as a proficient developer!Certainly! Let’s delve deeper into the world of literals with more code examples and their implications. As we progress, you’ll get a better sense of how to use literals wisely and strategically to make your code both clean and effective.
When it comes to control structure, having a clear understanding of when to use literals can bring about a tremendous difference in code clarity. For instance, consider how we apply literals within a “switch” statement.
// Switch case with literals int statusCode = 404; switch (statusCode) { case 200: System.out.println("OK"); break; case 404: System.out.println("Not Found"); break; case 500: System.out.println("Server Error"); break; default: System.out.println("Unknown Status"); break; }
Here, literals serve to match specific cases within the switch, simplifying the structure and implementing a mapped response easily.
Moreover, when initializing complex data structures, such as dictionaries or maps, literals provide a straightforward syntax for setting up key-value pairs:
// Dictionary initialization with literals Map<String, Integer> phoneBook = new HashMap<>(); phoneBook.put("Alice", 5551234); phoneBook.put("Bob", 5559876); phoneBook.put("Charlie", 5556789);
Utilizing literals for method calls showcases their convenience as you can pass values directly without prior variable declarations:
// Method call with literals as arguments displayMessage("Welcome to Zenva!", true);
This direct approach, however, should be used judiciously, as overuse can lead to less readable code if the context isn’t clear.
Let’s also consider how literals can be employed in object-oriented programming when constructing objects:
// Object construction with literals Rectangle box = new Rectangle(5, 10); // Width and height are provided directly
Literal values can be used to instantiate objects with predefined states. This is common in creating configuration objects or when setting up initial test data.
Boolean literals are particularly interesting to look at, especially in control flows. Let’s observe this in a “do-while” loop:
// Conventional do-while loop do { performAction(); } while (shouldContinue()); // do-while loop with a boolean literal do { performOneTimeSetup(); } while (false);
In the second example, the loop will execute exactly once, due to the literal `false` in the condition. This can be particularly useful for emphasizing a single-time execution in the context of an iterative control structure.
Lastly, let’s look at an example of string concatenation with literals – a common operation in many applications:
// String concatenation with literals String fullName = "John" + " " + "Doe"; System.out.println("Full name: " + fullName);
In this case, the ‘+’ operator is used to concatenate string literals, forming a new string without any need for intermediate variables.
Through these examples, the versatility and necessity of literals in programming become evident. Their correct and judicious use can enhance your code’s legibility, efficiency, and overall quality. Nonetheless, remember to always balance their use with good coding practices, striking the right harmony between ease of understanding and efficient coding.
Continue Your Coding Journey
Diving into literals is just the beginning! As your understanding of programming grows, you’ll find there are endlessly fascinating concepts to explore and skills to master. If you’re eager to take the next step on your learning pathway, we’ve got you covered.
We encourage you to check out our Python Mini-Degree, a treasure trove of knowledge that aims to solidify your programming foundations and advance your skills. Python, renowned for being a beginner-friendly yet powerful language, opens doors to various domains: whether it’s game development, data science, or web applications, Python is a valuable asset in your toolkit.
For a broader horizon of opportunities, delve into our extensive collection of Programming Courses. Each course is designed to offer practical, step-by-step guidance, empowering you to build projects that showcase your newfound expertise. Our flexible, 24/7 accessible courses support you from your first line of code to creating sophisticated projects that you’ll be proud to share.
Your adventure in programming awaits, and we’re thrilled to be a part of it!
Conclusion
As we wrap up our exploration of literals, we’ve seen them as the building blocks of programming languages—the immutable values that give precision and clarity to our code. Whether you’re just starting out or looking to refine your skills, a solid grasp on using literals is a cornerstone for any programmer. The best way to cement this knowledge is through practice and continuously challenging yourself with new projects.
And remember, if you’re looking for a path to enhance your programming prowess, our Python Mini-Degree is the ideal next step. It’s more than just a course; it’s a comprehensive learning experience designed to support you every step of the way. So why wait? Join us at Zenva Academy and take your coding abilities from budding to brilliant. We look forward to being part of your journey towards becoming a coding maestro!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
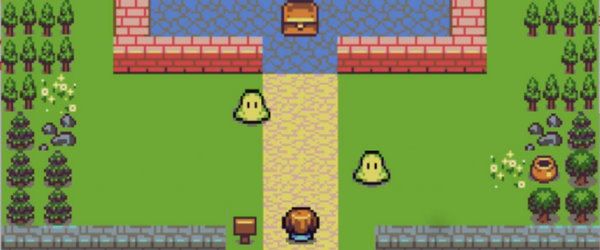
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.