Understanding the hierarchy of operations is akin to learning the rules of a game: it helps to ensure that we achieve the correct result every time. Much like in a strategy game where the player must understand the order of actions to optimize their moves, programming also requires a solid grasp of which operations take precedence to guarantee that our code behaves as intended. Embarking on the adventure of operator precedence will unlock a new level of control and precision in your code, leading to more robust and error-free programs.
What is Operator Precedence?
Table of contents
What is Operator Precedence?
Operator precedence is the rule that defines the order in which different operations are processed by a computer program. In the same way that in mathematics multiplication is performed before addition, programming languages also decide which operations to execute first based on a predefined hierarchy. This not only includes arithmetic operations but also logical, relational, and other types of operations that you might encounter while coding.
What is it for?
Understanding operator precedence is crucial for writing correct and efficient code. If you misunderstand the order in which operations are performed, you could end up with unexpected results, which leads to bugs in your program. Knowing the precedence helps you predict the outcome of your expressions, making debugging and development smoother and faster.
Why Should I Learn It?
Learning about operator precedence is an essential step for any programmer. It ensures that you can read and write code effectively, anticipate how an expression will be evaluated, and avoid common errors. Even though different programming languages might have slight variations in their rules, the core concepts remain similar, making this knowledge highly transferable and a fundamental part of your programming skill set.
Basic Arithmetic Operations
Let’s start with basic arithmetic operations which form the bedrock of operator precedence. These include addition (+), subtraction (-), multiplication (*), and division (/).
// Example 1: Without considering precedence console.log(10 + 5 * 2); // Outputs 20, not 30 because * has higher precedence than + // Example 2: Using parentheses to change order console.log((10 + 5) * 2); // Outputs 30 because parentheses take the highest precedence
Without proper understanding, one might expect the first example to output 30, calculating from left to right. However, multiplication takes precedence over addition, resulting in 10 + (5 * 2), which is 20.
// Example 3: Division over addition console.log(20 + 25 / 5); // Outputs 25, not 9, division is performed first // Example 4: Subtraction and multiplication console.log(50 - 5 * 6); // Outputs 20, not 270, * before -
In the third example, the division is performed before the addition, leading to 20 + (25 / 5), not (20 + 25) / 5 as one might erroneously assume without knowledge of operator precedence. Similarly, multiplication is executed before subtraction in the fourth example.
Combining Arithmetic with Assignment Operations
Assignment operators are also important and often mixed with arithmetic operations. The equal sign (=) is the most common assignment operator.
// Example 5: Combining addition with assignment let a = 10; a += 5; // Equivalent to a = a + 5 console.log(a); // Outputs 15 // Example 6: Combining multiplication with assignment let b = 5; b *= 2; // Equivalent to b = b * 2 console.log(b); // Outputs 10
These compound assignment operators help shorten your code by combining an arithmetic operation with assignment in one go. In the above examples, a += 5
is a more concise way of writing a = a + 5
, and likewise, b *= 2
is shorthand for b = b * 2
.
Logical Operators and Their Precedence
Moving onto logical operators, these include AND (&&), OR (||), and NOT (!) operators that are widely used in conditions and control flows.
// Example 7: AND takes precedence over OR console.log(false || true && false); // Outputs false, AND is evaluated first // Example 8: Using parentheses with logical operators console.log((false || true) && false); // Outputs false, changes evaluation order
Without brackets, in the seventh example, the &&
operator takes precedence over ||
, whereas in the eighth example, the addition of parentheses alters the order of evaluation. This is crucial in control flow logic to yield correct results.
// Example 9: Not operator has highest precedence console.log(!true || false); // Outputs false because !true is evaluated first // Example 10: Combination with logical and comparison operators console.log(5 > 3 && 2 || 4 3 && 2 is truthy
In the ninth example, the NOT operator (!) inverts the truth value before any other logical operation takes place. Meanwhile, the tenth example combines logical AND, OR, and comparison operators, showcasing the importance of understanding precedence to parse the expression correctly.
Relational Operators Precedence
Finally, relational operators include greater than (>), less than (<), equal to (==), and not equal to (!=). These operators are typically used in comparing two values.
// Example 11: Greater than and equal to console.log(3 > 2 == 1); // Outputs true because 3 > 2 is true (1) and true == 1 in JavaScript // Example 12: Not equal takes precedence over logical OR let c = 5, d = 10; console.log(c != 6 || d > 10); // Outputs true, c != 6 is evaluated first
In the eleventh example, comparison operators are evaluated from left to right since they have the same level of precedence, where 3 > 2
yields true
, which is equivalent to 1
in JavaScript. In the final example, c != 6
is evaluated separately from d > 10
due to precedence rules.
With these practical operator precedence examples, we are paving the way to more complex and powerful code, ensuring that our fellow programmers understand the underpinnings of logic within a programming context. This knowledge is indispensable for bug-free and predictable code. Stay with us as we dive into more advanced scenarios in the next part of this tutorial series.
As we delve further into the intricacies of operator precedence, it’s essential to comprehend that certain operations, though less common in everyday coding, play a pivotal role in specific scenarios. These involve bitwise operators, the ternary operator, and the type comparison operator typeof
.
Bitwise Operators
Bitwise operators such as AND (&), OR (|), XOR (^), NOT (~), and shifts (<>, >>>) operate on the binary representations of integers. They can be used for efficient mathematical computations, digital signal processing, and managing permissions in low-level programming.
// Example 13: Bitwise AND precedence console.log((5 & 1) === 0); // Outputs false because 5 & 1 evaluates to 1 // Example 14: Bitwise OR and shifts let e = 4; // In binary: 100 console.log(e >> 1 | 2); // Outputs 2, because 100 shifted right is 10 (2 in decimal)
The thirteenth example introduces bitwise AND, which compares each bit of the numbers and returns a new number. Bitwise operations are often used with comparison operators, like in this example where it’s evaluated before the strict equals operator ===
.
In the fourteenth example, we perform a right bitwise shift on the number 4 (which is 100 in binary), resulting in 10 in binary (or 2 in decimal). This result is then combined with 2 using a bitwise OR, which evaluates to 2, showcasing how these operators interact with each other.
Ternary Operator
The ternary operator is a concise way to perform an if-else statement. It takes three operands and is often used for inline conditional evaluations.
// Example 15: Ternary operator basic usage let age = 20; let beverage = age >= 21 ? "Beer" : "Juice"; console.log(beverage); // Outputs "Juice" // Example 16: Nested ternary operators let score = 85; let grade = score > 90 ? "A" : score > 75 ? "B" : "C"; console.log(grade); // Outputs "B"
The fifteenth example shows a simple use of the ternary operator, deciding the value of beverage
based on the condition age >= 21
. The sixteenth example introduces a nested ternary, which, while powerful, can be difficult to read and should be used with care.
The ‘typeof’ Operator
The typeof
operator is used to determine the type of a JavaScript variable or expression. Its result is always a string that names the type.
// Example 17: Basic typeof usage let f = "Hello, world!"; console.log(typeof f); // Outputs "string" // Example 18: typeof with different types let g = 42; console.log(typeof g === "number"); // Outputs true
In the seventeenth example, we use typeof
to verify that f
is a string. The eighteenth example demonstrates comparing the type of g
directly with a type string “number”, which is a common way to use typeof
for type checking in JavaScript.
It’s pivotal to recognize that while operator precedence determines how an expression is processed, relying excessively on implicit precedence rules can lead to code that is hard to read and maintain. It’s often recommended to use parentheses to make the intended order of operations explicit, which not only clarifies the code for others but also helps prevent unintended consequences arising from complex precedence rules.
With a solid understanding of operator precedence under your belt, you’re well-equipped to navigate the majority of expressions you’ll encounter. As always, practice is key, so consider experimenting with these examples and creating your own to master the subtle art of operator precedence.
Continuing our exploration of operator precedence, let’s shift the spotlight onto some advanced scenarios that can often trip up even experienced programmers. Paying close attention to these examples will not only reinforce your understanding but also fine-tune your coding skills to catch potential bugs.
Short-circuit evaluation with logical operators can lead to different code paths being taken, which is often a source of confusion but can be used to our advantage when understood correctly.
// Example 19: Short-circuit evaluation with logical OR let h; let i = h || "default value"; console.log(i); // Outputs "default value" because h is undefined // Example 20: Logical AND used for conditional execution let j = 1; j > 0 && console.log('Positive number'); // Logs "Positive number" since j > 0 is true
In the nineteenth example, the logical OR operator returns the first truthy operand, which is why i
gets assigned a “default value” when h
is undefined. Similarly, in the twentieth example, the second expression is only logged if the first condition is true due to the logical AND operator.
Grouping expressions using parentheses is an effective way to ensure that operations are performed in the order you intend.
// Example 21: Grouping with parentheses let k = 3 + 4 * (2 - 1); console.log(k); // Outputs 7, parentheses change the natural precedence // Example 22: Nested parentheses let l = (4 + (3 - 1)) * ((2 * 2) + 1); console.log(l); // Outputs 25, multiple groupings clarify the order
In the twenty-first example, multiplication would naturally take precedence, but parentheses force the addition inside them to occur first. The twenty-second example uses nested parentheses to resolve complex expressions in a step-by-step manner, much like solving a multi-layered puzzle.
Another area where precedence rules apply is in the combination of increment/decrement operators (++/–) with other operations.
// Example 23: Post-increment precedence let m = 5; let n = m++ + 2; // Post-increment; m is incremented after this line console.log(n); // Outputs 7, m was 5 during this operation // Example 24: Pre-increment precedence let o = 5; let p = ++o + 2; // Pre-increment; o is incremented before this line console.log(p); // Outputs 8, o was 6 during this operation
Example 23 demonstrates the use of the post-increment operator, where m
is used in its original form in the expression before being incremented. Contrastingly, in example 24, the pre-increment leads to o
being incremented before being used in its respective expression.
Finally, let’s dive into the comma operator, which allows the evaluation of multiple expressions, where each expression is evaluated but only the result of the last one is returned.
// Example 25: Comma operator usage let q = (2, 3, 5); // Only the last number is assigned to q console.log(q); // Outputs 5 // Example 26: Using comma operator in a for-loop for (let r = 1, s = 1; r <= 5; r++, s++) { console.log(r * s); // Outputs the multiplication of r and s }
In the twenty-fifth example, the comma operator evaluates each number, but only the value 5 (the final one) is assigned to q
. The twenty-sixth example is where the comma operator is commonly found: in the header of a for-loop to allow for multiple expressions to be initialized and updated.
Mastering these advanced operator precedence rules can significantly enhance your problem-solving skills in programming. It’s important not just to learn the rules but to apply them through practice and code-writing. Observing their effects firsthand not only anchors the concepts but enables you to write more deliberate and expressive code. As you continue to evolve as a programmer, a deep understanding of operator precedence will undoubtedly become a tool as indispensable as any programming language’s core syntax.
Where to Go Next in Your Programming Journey
Mastering operator precedence is a fantastic step in your journey as a programmer, but there’s always more to learn and explore! We encourage you to continue building on this foundation by delving deeper into programming languages and concepts. Python, with its clear syntax and powerful libraries, is an excellent next step. Dive into our Python Mini-Degree and embrace the world of opportunities that Python programming has to offer.
Whether you’re starting as a beginner or looking to polish advanced skills, our Python Mini-Degree provides you with hands-on projects, from creating simple arcade games to more complex applications. The flexibility and breadth of our courses not only fit your schedule but also your curiosity and career aspirations. And the beauty of Python’s popularity? It opens doors to numerous careers in web development, data analysis, artificial intelligence, and more.
If you seek broader horizons beyond Python, take a look at our extensive range of Programming courses. With over 250 expertly crafted courses, we cover a wide scope of topics and skills that can help propel your career and allow you to turn your creative ideas into reality. Continue your learning adventure with us, and join the ranks of Zenva alumni who’ve gone on to publish their games, launch websites, and achieve their professional goals.
Conclusion
Embarking on the journey of understanding operator precedence is more than a lesson in coding; it’s about honing the ability to think logically and systematically. Like meticulously planning the moves in a chess game, each step in programming can have profound implications on the outcome. The confidence you’ll gain from mastering this aspect of programming will be your beacon as you navigate through more complex code. Keep this momentum going; take the skills you’ve cultivated to new heights with our Python Mini-Degree and watch as doors to incredible opportunities begin to unlock right before your eyes.
Remember, the world of coding is vast and filled with endless possibilities. Whether you dream of creating games, developing applications, or automating tasks, continued learning is the key to turning those dreams into digital realities. Let your understanding of operator precedence be the firm foundation upon which you build a robust and versatile programming knowledge. Join us at Zenva, and let’s write the future of technology together—one line of code at a time.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
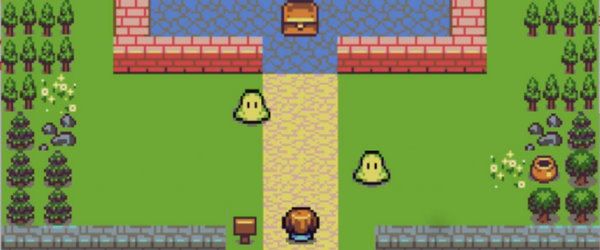
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.