Welcome to our tutorial on local variables, a fundamental concept that stands at the very core of programming. Whether you’re just starting out your journey in computer science or you’re an experienced coder refining your knowledge base, understanding local variables is essential. These variables are the building blocks of our code — aiding in holding information, passing data and controlling the flow of our applications. Throughout this tutorial, we’ll delve into what local variables are, how they work, and why they’re an indispensable tool for any programmer.
Table of contents
What Are Local Variables?
Local variables are variables that are declared inside a function or a block and can only be accessed within that function or block. They provide a way to store data temporarily for use within a specific context.
What Are Local Variables For?
Local variables serve a few key purposes:
– They help manage data by keeping it contained within a context where it’s relevant.
– They contribute to the readability and maintainability of code by making sure variables are only used where necessary.
– They enhance a program’s performance by reducing the scope of variables and therefore the memory footprint.
Why Should I Learn About Local Variables?
Learning about local variables is crucial because:
– They are used in almost every programming language, making this knowledge highly transferable.
– Understanding local variables is vital for writing efficient and error-free code.
– They play a pivotal role in the concept of ‘scope’, which is fundamental to the structuring and understanding of any program.
With this foundation in place, we’ll now jump into some hands-on examples to get a closer look at how local variables function in a programming environment.
Declaring and Using Local Variables in Functions
Local variables defined within a function are only accessible within that function, which makes them private and secure. Here’s an example in Python:
def calculate_area(width, height): area = width * height return area print(calculate_area(4, 5))
And in JavaScript, it looks like this:
function calculateArea(width, height) { let area = width * height; return area; } console.log(calculateArea(4, 5));
Notice how `area` is a local variable to the `calculate_area` and `calculateArea` functions in Python and JavaScript, respectively. You cannot access the variable `area` outside of these functions.
Local Variables and Control Structures
Local variables also play a critical role within control structures like loops and conditionals.
Here’s an example with a for loop in C++:
for (int i = 0; i < 10; i++) { cout << i << endl; } // 'i' is not accessible here
And within an if-statement in Ruby:
if user.is_active greeting_message = "Welcome back, #{user.name}!" puts greeting_message end # 'greeting_message' is not accessible here
In both examples, `i` and `greeting_message` are local to their blocks and cannot be used outside.
Function Parameters as Local Variables
When you pass parameters to a function, they are treated as local variables within that function.
In this Java method:
public void printSum(int a, int b) { int sum = a + b; System.out.println("The sum is: " + sum); }
The parameters `a` and `b` are local variables inside the `printSum` method.
Similarly, in Go:
func printSum(a int, b int) { sum := a + b fmt.Println("The sum is: ", sum) }
Both `a` and `b` are local to the `printSum` function.
Temporary Variables in Loops and Algorithm Implementations
Using temporary local variables in loops or during algorithm implementations can greatly simplify problem solving.
Consider a simple Python function to reverse a list:
def reverse_list(my_list): for i in range(len(my_list) // 2): temp = my_list[i] my_list[i] = my_list[-i-1] my_list[-i-1] = temp return my_list print(reverse_list([1, 2, 3, 4, 5]))
The ‘temp’ variable is a local variable employed to swap elements temporarily.
Similarly, in a sorting algorithm in C:
void bubbleSort(int arr[], int n) { for (int i = 0; i < n-1; i++) for (int j = 0; j arr[j+1]) { int temp = arr[j]; arr[j] = arr[j+1]; arr[j+1] = temp; } }
Here, `temp` is again used as a temporary local variable for swapping values in an array. Remember, the use of local variables helps to keep these temporary exchanges self-contained within the function or loop scope.Local variables are typically used within functions to hold temporary state and computation results. Let’s explore more contexts where local variables shine through additional code examples:
Counter Variables in Loops
A common use of local variables is as counters in loops. Here’s a classic for loop in C#:
for (int counter = 0; counter < 10; counter++) { Console.WriteLine(counter); }
The variable `counter` is only accessible within the loop, and it governs the number of times the loop will execute.
Short-lived Variables in Single-use Cases
Consider a situation where you might want to store a value for just a single use:
// Swift func greetUser(user: String) { let greeting = "Hello, \(user)!" print(greeting) } greetUser(user: "Alice")
The `greeting` variable only exists during the execution of `greetUser`. It’s briefly used to store a formatted string and then no longer needed.
Local Variables in Conditional Statements
Similar to loops, local variables are essential within conditional blocks. For example, in Java:
if (isValidUser) { String welcomeMessage = "Welcome to the system!"; System.out.println(welcomeMessage); }
`welcomeMessage` is a local variable inside the `if` block, scoped to that condition only.
Recursive Function Calls
Local variables in recursive calls illustrate how each function call has its own set of local variables. A factorial function in Python demonstrates this:
def factorial(n): if n == 1: return 1 else: return n * factorial(n - 1) print(factorial(5))
Each call to the `factorial` function creates a new local variable `n`, separate from `n` in other calls.
Working with Multiple Local Variables
It’s common to use several local variables to hold various pieces of data:
// PHP function processOrder($itemId, $quantity) { $itemPrice = getItemPrice($itemId); $orderTotal = $itemPrice * $quantity; $tax = calculateTax($orderTotal); $totalWithTax = $orderTotal + $tax; return $totalWithTax; }
In the PHP function `processOrder`, `itemPrice`, `orderTotal`, `tax`, and `totalWithTax` are all local variables that help calculate the final order cost.
Indexing in For-Each Loops
Sometimes, you might want to combine local variables with for-each loops to keep track of the index:
for (let [index, value] of ['a', 'b', 'c'].entries()) { console.log(`Index: ${index}, Value: ${value}`); }
In JavaScript, `.entries()` can be used to create an iterator that provides both the index and value, which are used as local variables within the loop.
These examples are but a glimpse into the vast world of local variables, highlighting their versatility and convenience in different programming scenarios. Local variables are indispensable for creating clear and concise code, and as we can see, they’re utilized in countless ways across various languages and contexts. Understanding and effectively using local variables will undoubtedly improve your programming skills and your ability to communicate with the computer efficiently.Local variables truly shine when utilized within specialized functions to perform specific tasks. Let’s continue exploring their use through various code examples in different languages:
Using Local Variables to Track State
When dealing with a function that tracks an internal state, local variables are indispensable. Consider this JavaScript function that filters out even numbers from an array:
function filterEvenNumbers(numbers) { const result = []; for (const number of numbers) { if (number % 2 === 0) { result.push(number); } } return result; } console.log(filterEvenNumbers([1, 2, 3, 4, 5]));
The `result` array is a local variable that collects the filtered values throughout the iteration.
Moving on to a more mathematical problem, here’s how you can use local variables to calculate Fibonacci numbers in Java:
public int fibonacci(int n) { if (n 1) { int sum = a + b; a = b; b = sum; } return b; }
Within the `fibonacci` method, `a` and `b` are local variables representing the last two numbers of the Fibonacci sequence being calculated.
Iteration with Local Variables
Iteration is a common scenario where local variables are frequently used. Here’s a Python example that computes the factorial of a number using an iterative approach:
def factorial_iterative(n): result = 1 for i in range(2, n + 1): result *= i return result print(factorial_iterative(5))
The `result` variable is used to accumulate the product of all integers up to `n`.
Local Variables in Exception Handling
Handling exceptions is another programming area where local variables are essential. For example, in C#:
try { int dividend = 50; int divisor = 0; int quotient = dividend / divisor; Console.WriteLine(quotient); } catch (DivideByZeroException e) { string message = "Attempted divide by zero."; Console.WriteLine(message); }
The `dividend`, `divisor`, and `quotient` variables are local to the try block, and the `message` variable is local to the catch block.
Refactoring with Local Variables
You can use local variables to refactor and improve your code. Here’s an example of breaking down a complex expression into more readable parts using local variables in Ruby:
def discount_price(original_price, discount_percentage) discount_amount = original_price * (discount_percentage / 100.0) price_after_discount = original_price - discount_amount final_price = price_after_discount.round(2) return final_price end puts discount_price(100, 15)
Each step of the calculation uses a local variable, improving the readability and maintainability of the function.
Local variables are a powerful feature in any programmer’s toolkit, acting as both placeholders and manipulators of data within the boundaries of our functions and blocks. They offer clarity by encapsulating logic and aid in debugging by limiting the scope of variables. Through these examples, we can appreciate their role in creating efficient and organized code that is easier to understand and maintain. Remember, coding is not just about getting the job done; it’s about doing it in a way that stands the test of time and teamwork, and that’s where good practices like using local variables appropriately make all the difference.
Where to Go Next in Your Programming Journey
You’ve taken some crucial steps in mastering the fundamentals of programming, and we believe that a strong foundation will set you up for success in any coding endeavor you choose to pursue. As you move forward, we encourage you to keep challenging yourself and expanding your knowledge.
For those interested in diving deeper into the world of Python and building upon what you’ve learned, consider exploring our Python Mini-Degree. This comprehensive collection is meticulously crafted to guide you through Python’s versatile landscape, where you can go from basic coding principles to creating your own games and applications. It’s perfect for budding programmers eager to harness Python’s potential in various cutting-edge fields.
If you’re looking to broaden your skills across a wider range of programming topics, take a look at our full Programming courses. With over 250 supported courses, you can propel your journey from beginner to professional at your own pace, ensuring a well-rounded skill set ready for the industry.
Embrace the path of continuous learning with Zenva, and let us be your partner in coding, game development, and beyond. Whether you’ve just started or are seeking to polish your skills further, we’re here to support you with high-quality content that can take your career to new heights.
Conclusion
Understanding local variables is akin to learning the art of wielding the building blocks of code with precision and intent. As you continue to practice and apply these concepts, you’ll find your programs becoming more logical, more efficient, and notably easier to debug. Through the various scenarios and languages we’ve delved into today, it’s clear that the principles of local variables transcend syntax and form the backbone of good programming practices.
We at Zenva are excited to accompany you as you craft your own path in the vast universe of coding. Whether you’re looking to deepen your understanding of a specific language, venture into the realms of game development, or build functional and intricate applications, our doors are open, and our resources are at your service. Leap into your next challenge with confidence, backed by the strong foundations you’ve cemented today, and let us guide you through the next chapter of your coding adventure. 🚀
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
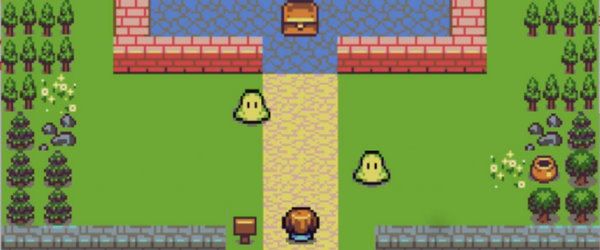
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.