In the dynamic world of programming, where change is the only constant, one key practice stands as a cornerstone for maintaining clean, efficient, and sustainable code: code refactoring. As we embark on this tutorial, we invite learners of all levels to join us in exploring the transformative power of refactoring—whether you’re just starting your coding journey or you’re an experienced coder looking to polish your craft.
Table of contents
What is Code Refactoring?
Code refactoring is the process of restructuring existing computer code without changing its external behavior. It’s akin to cleaning up your workspace, organizing your desk, and labeling your files—not to do different work, but to make the work you’re already doing more efficient and accessible. With refactoring, you can expect your code to become cleaner, easier to understand, and more maintainable.
What is it for?
Think of refactoring as tuning a musical instrument before a concert; you’re not changing the melodies you’ll play, but you’re ensuring that each note comes out clear and your performance is smooth. Similarly, refactoring enhances your code’s performance by:
- Improving code readability and structure
- Reducing complexity and duplication
- Identifying and fixing latent or potential bugs
- Enabling easier updates and feature additions in the future
Why Should I Learn It?
Learning to refactor code is an investment in your future as a programmer. It helps you write code that’s built to last and easily adaptable to change, which is crucial given the fast-paced nature of technology. Moreover, it’s a highly sought-after skill among employers, as clean code is easier to work with, reduces onboarding time for new developers, and ultimately saves time and money.
Refactoring is not just about making your code “look nice”—it’s about ensuring your codebase is a well-oiled machine that can grow and evolve with your project’s needs. Let’s dive in and discover the techniques and reasons behind this essential practice, using Python as our language of choice to crystallize the concepts of code refactoring in your developer toolkit.
Discovering Bad Smells in Code
Before we dive into refactoring patterns, it’s crucial to identify the “bad smells” in code. These issues hint at possible improvements and lead us to specific refactoring actions. Below are examples of common bad smells:
# Duplicate Code: The same code structure appears in multiple places. def calculate_area(radius): return 3.14 * radius * radius # Later in the same codebase... def print_area(radius): area = 3.14 * radius * radius print(f"The area is: {area}")
Now, let’s see what long method smells like, which is when a block of code tries to do too much.
# Long Method: A method that has grown too long and is doing too much. def process_data(data): # lots of code to validate data # ... # lots of code to process data # ... # lots of code to format results # ... return results
Large Class is another smell indicating that a class may have too many responsibilities.
# Large Class: A class that is trying to do too much. class Employee: def __init__(self, name, address, date_joined): self.name = name self.address = address self.date_joined = date_joined # Methods related to employee's personal details # ... # Methods related to employee's work records # ... # Methods related to employee's benefit plans # ...
Next, are some instances of how code can violate the DRY (Don’t Repeat Yourself) principle, leading to unnecessary repetition.
Refactoring Techniques
Recognizing bad smells is the first step. Now, let’s rectify them with some refactoring techniques:
Removing duplicate code by extracting a common method:
# Before Refactoring def calculate_area(radius): return 3.14 * radius * radius def print_area(radius): area = 3.14 * radius * radius print(f"The area is: {area}") # After Refactoring def calculate_area(radius): return 3.14 * radius * radius def print_area(radius): area = calculate_area(radius) print(f"The area is: {area}")
Now, let’s break down a long method into smaller, more manageable pieces.
# Before Refactoring def process_data(data): # lots of code return results # After Refactoring def validate_data(data): # code to validate data return validated_data def process_data(validated_data): # code to process data return processed_data def format_results(processed_data): # code to format data return results # Now, our main method looks cleaner def main(data): validated = validate_data(data) processed = process_data(validated) results = format_results(processed) return results
For a large class, we may need to apply the Extract Class refactoring technique.
# Before Refactoring class Employee: # Employee has too many responsibilities # After Refactoring class PersonalDetails: # Handles personal details of an employee class WorkRecords: # Handles work history of an employee class BenefitPlans: # Handles benefit plans of an employee # Now, Employee class can be more focused class Employee: def __init__(self, name, personal_details, work_records, benefits): self.name = name self.personal_details = personal_details self.work_records = work_records self.benefit_plans = benefits
By applying these techniques, we not only improve the readability of the code but also make future maintenance and extension more manageable. It’s a meticulous process of transforming code for the better without altering its functionality.
Continuing our journey into code refactoring, we will explore more techniques that can turn a cluttered codebase into an efficient, scalable foundation for any application. Whether you’re working with Python or any other programming language, these principles are universally applicable.
One common issue that requires refactoring is the “Switch Statements” smell. Often, complex switch (or if-else chains) can be replaced with polymorphism for a cleaner design.
# Before Refactoring class Bird: def get_speed(self, bird_type): if bird_type == "EuropeanSwallow": return "Average speed" elif bird_type == "AfricanSwallow": return "Slightly higher average speed" elif bird_type == "NorwegianBlueParrot": return "Not flying" # additional cases # After Refactoring class Bird: def get_speed(self): pass class EuropeanSwallow(Bird): def get_speed(self): return "Average speed" class AfricanSwallow(Bird): def get_speed(self): return "Slightly higher average speed" class NorwegianBlueParrot(Bird): def get_speed(self): return "Not flying"
Another technique is method extraction, where a piece of code that does a specific job is moved into its method.
# Before Refactoring def print_owed_amount(customer): ow_ammount = customer.get_owed_amount() print(f"Amount owed: {ow_ammount}") # After Refactoring def get_owed_statement(customer): return f"Amount owed: {customer.get_owed_amount()}" def print_owed_amount(customer): print(get_owed_statement(customer))
Refactoring temporal coupling between method calls is important for the flexibility of code, where the sequence of method calls is mandatory for the proper execution of operations.
# Before Refactoring class DataProcessor: def __init__(self): self.data_loaded = False def load_data(self): # Loading data logic self.data_loaded = True def process_data(self): if not self.data_loaded: raise Exception("Data is not loaded yet.") # Process data logic # After Refactoring class DataProcessor: def __init__(self): self.data = self.load_data() def load_data(self): # Loading data logic return data def process_data(self, data): # Process data logic
The Inline Method is another valuable refactoring technique, where a method’s body is less obvious than its name, we can replace calls to the method with the method’s content itself and delete the method altogether.
# Before Refactoring class Order: def get_base_price(self): return self.base_price def calculate_discount(self): base_price = self.get_base_price() if base_price > 1000: return base_price * 0.95 return base_price * 0.98 # After Refactoring class Order: def calculate_discount(self): if self.base_price > 1000: return self.base_price * 0.95 return self.base_price * 0.98
Lastly, the Replace Temp with Query technique helps reduce temporary variables and enable better code understanding and reuse by turning the temp into a private method.
# Before Refactoring def calculate_total(): base_price = quantity * item_price if base_price > 1000: return base_price * 0.95 return base_price * 0.98 # After Refactoring def calculate_base_price(): return quantity * item_price def calculate_total(): if calculate_base_price() > 1000: return calculate_base_price() * 0.95 return calculate_base_price() * 0.98
Remember, refactoring is an art that demands thoughtful consideration of the current code and an understanding of what the future requirements might be. Each refactoring step should be done in such a manner that the system never stops working—the end goal always is to improve the design of the software while it’s being written, not afterward. By mastering these techniques, you’ll be well on your way to becoming a more proficient and agile software developer.
Advancing further into the domain of code refactoring, we will delve into some additional patterns that enhance the clarity, performance, and longevity of code. Engaging with these examples will broaden your understanding and equip you with a multifaceted set of tools for tackling various coding scenarios that may necessitate refactoring.
One pattern we may encounter is the “Replace Magic Number with Symbolic Constant.” This helps to clarify the meaning of numbers within the code, making it self-documenting.
# Before Refactoring def calculate_circumference(radius): return 2 * 3.14159 * radius # What is 3.14159? # After Refactoring PI = 3.14159 def calculate_circumference(radius): return 2 * PI * radius # Much clearer! PI is defined as a constant.
Consolidating conditional expressions which are complex or duplicate throughout multiple parts of the code is another area that can benefit from refactoring:
# Before Refactoring if age > 65 or age 65 or age < 21 and not is_employed if is_eligible_for_discount(age, is_employed): discount = 10
Introducing Assertion is a refactoring technique to make the expected behavior of the code more explicit, thus preventing future modifications from introducing errors.
# Before Refactoring def get_person_age(person): return person.age # After Refactoring def get_person_age(person): assert person is not None, "person should not be None" return person.age
Another pattern is “Replace Type Code with Subclasses,” which can aid in making the code more maintainable and readable when dealing with an object that can have one of several types.
# Before Refactoring class Employee: def __init__(self, employee_type): self.employee_type = employee_type # After Refactoring class Employee: pass class Manager(Employee): pass class Engineer(Employee): pass
The Inline Class refactoring technique can be used when a class isn’t doing enough to justify its existence. By moving all its features into another class, we can simplify the overall design:
# Before Refactoring class Contact: def __init__(self, email): self.email = email class Person: def __init__(self, name, contact): self.name = name self.contact = contact # After Refactoring class Person: def __init__(self, name, email): self.name = name self.email = email
The Refactor Method Object pattern comes in handy when a method has too many parameters and local variables, making it challenging to maintain:
# Before Refactoring def calculate_payment(date, amount, employee): # complex logic using date, amount and employee # After Refactoring class PaymentCalculator: def __init__(date, amount, employee): self.date = date self.amount = amount self.employee = employee def calculate(): # complex logic that was inside the calculate_payment method
Lastly, let’s see the “Parameterize Method” refactoring method which you would use when several methods perform similar actions that are only slightly different.
# Before Refactoring def five_percent_raise(employee): employee.raise_salary(1.05) def ten_percent_raise(employee): employee.raise_salary(1.10) # After Refactoring def give_raise(employee, percentage): employee.raise_salary(1 + percentage / 100)
Each of these refactoring examples serves to polish the code, making it not just a functioning set of instructions but a readable and maintainable document. The ability to refactor effectively significantly enhances the quality of a project, ensuring that the software can easily adapt to new requirements and saving valuable time in the long run. Adopting these practices strengthens your status not just as a coder, but as a true craftsman of your trade.
Where to Go Next in Your Coding Journey
Embarking on the quest of learning to program, especially in the diverse and powerful language of Python, is an exhilarating challenge. You’ve made significant strides by understanding the value of code refactoring, but the path ahead is abundant with opportunities to sharpen your skills further and expand your knowledge.
To continue your development journey, we highly recommend exploring our Python Mini-Degree. It’s a curated collection of courses designed to take you from beginner to professional, covering a wide range of topics from coding basics to game and app development utilizing Python’s most popular frameworks. This Mini-Degree is perfect for both absolute beginners or experienced programmers looking to deepen their expertise and create a portfolio of impressive projects.
For a more expansive dive into different programming realms, our Programming courses offer over 250 courses across game development, artificial intelligence, and more. With Zenva, learning is flexible and always at your pace, ensuring knowledge is not just acquired but thoroughly understood and retained. Create games, master algorithms, and get ready to transform your curiosity into a burgeoning career.
Conclusion
In conclusion, code refactoring is a vital yet intricate aspect of the programming lifecycle that truly separates good code from great. It’s a continuous process of improvement, fostering robust and dynamic development ecosystems. By delving into the practices we’ve discussed, you’re not only preparing your code for the challenges of today but future-proofing it for the innovations of tomorrow. Take pride as you refactor, for you’re not merely writing code; you’re crafting the very infrastructure that powers technology.
Your journey doesn’t end here. We encourage you to continue refining your programming skills and learning with us at Zenva. The Python Mini-Degree awaits, promising a world of knowledge from the fundamentals to advanced concepts that will empower you to code, create, and innovate. Embrace the journey, flex your problem-solving muscles, and remember, we are here to support you at every loop, function, and line of code along the way.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
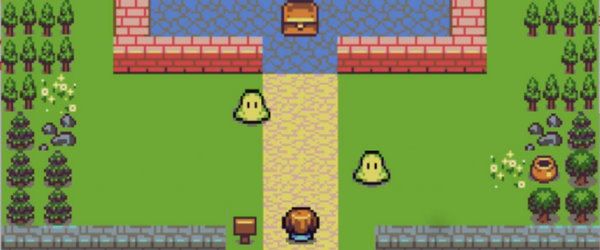
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.