Understanding the mechanism behind pseudo-random number generation is a fundamental concept in programming that can unlock doors to a universe of functionality, from simple games to complex simulations. Embark on this intriguing journey with us as you’ll see that the patterns behind randomness are not just a matter of chance but a crafty dance of algorithms designed to mimic unpredictability. By delving into this tutorial, you’ll gain a skill set that is both practical and fascinating, which can serve as a cornerstone for your programming arsenal.
Table of contents
What Is Pseudo-Random Number Generation?
Pseudo-random number generation (PRNG) refers to the process by which a computer program generates numbers that appear random, but are actually produced by a deterministic and repeatable process. The ‘pseudo’ in pseudo-random suggests that while these numbers seem haphazard, they are ultimately governed by an initial value known as a seed.
What Is PRNG Used For?
The uses of PRNG stretch far and wide. They are essential in fields such as cryptography, simulations, gaming, statistical sampling, and anywhere else where unbiased randomness is needed. Imagine rolling dice in a board game or shuffling cards in a virtual deck—such actions rely on PRNG.
Why Should I Learn It?
Understanding PRNG enhances your capability to implement features that require randomness in your programs. It’s a crucial skill for any developer, touching on core programming concepts like algorithmic thinking and software design. Learning about PRNG provides insight into how systems manage to produce sequences that appear unpredictable, opening the door to critically understanding and utilizing randomness in your projects.
Generating Random Numbers in JavaScript
JavaScript provides built-in methods to generate pseudo-random numbers. The Math.random() function is the key to starting our exploration. It generates a floating-point, pseudo-random number in the range from 0 (inclusive) to 1 (exclusive). Let’s see how it works.
// Generating a basic random number with JavaScript var randomNumber = Math.random(); console.log(randomNumber);
This will log a number like 0.123456789 in the console. Now, what if we want to control the range of our random numbers? We can scale and adjust the range as needed.
// Generating a random number between 0 and 10 var randomUpToTen = Math.random() * 10; console.log(randomUpToTen); // Generating a random integer between 0 and 10 var randomIntegerUpToTen = Math.floor(Math.random() * 11); console.log(randomIntegerUpToTen); // Generating a random integer between 1 and 10 var randomIntegerOneToTen = Math.floor(Math.random() * 10) + 1; console.log(randomIntegerOneToTen);
These snippets above produce a random floating-point number up to 10, a random integer up to 10, and a random integer between 1 and 10, respectively.
Using a Seed in PRNG
JavaScript’s Math.random() doesn’t allow us to set a seed directly. However, we can implement our own seeded PRNG for consistent results across sessions. Here’s an example of a simple seed-based PRNG using the linear congruential generator algorithm:
// A simple linear congruential generator function LCG(seed) { const a = 1664525; const c = 1013904223; const m = Math.pow(2, 32); return function() { seed = (a * seed + c) % m; return seed / m; }; } var seed = Date.now(); // Using the current timestamp as a seed var rng = LCG(seed); console.log(rng()); console.log(rng());
The above code defines a function that returns a new function, a closure, which calculates a new number each time it’s called based on the previous one.
Randomizing Array Elements
Randomizing or shuffling array elements is a common task that’s often performed in games and simulations. We can use the Fisher-Yates (aka Knuth) shuffle to achieve this:
// Function to perform the Fisher-Yates (Knuth) shuffle function shuffleArray(array) { for (let i = array.length - 1; i > 0; i--) { const j = Math.floor(Math.random() * (i + 1)); // random index from 0 to i [array[i], array[j]] = [array[j], array[i]]; // swap elements } return array; } var myArray = [1, 2, 3, 4, 5]; console.log(shuffleArray(myArray));
Each call to shuffleArray() will rearrange the elements in a seemingly random order.
Selecting Random Elements from an Array
Sometimes we do not want to shuffle the whole array, but just pick one or several random elements from it. Here’s how we can do that:
// Picking a single random element from an array function pickRandomElement(arr) { var index = Math.floor(Math.random() * arr.length); return arr[index]; } // Picking 'n' random elements from an array function pickNRandomElements(arr, n) { let choices = [...arr]; let results = []; for (let i = 0; i < n; i++) { let index = Math.floor(Math.random() * choices.length); results.push(choices[index]); choices.splice(index, 1); // remove the chosen element } return results; } var colors = ['red', 'green', 'blue', 'yellow', 'purple']; console.log(pickRandomElement(colors)); // Example output: 'blue' console.log(pickNRandomElements(colors, 3)); // Example output: ['yellow', 'red', 'green']
The first function returns a single random item from the array. The second function returns ‘n’ unique random items, ensuring no repetitions.
In these examples, we’ve covered basic random number generation, a seeded PRNG implementation, array shuffling, and random element selection—paving the way for you to integrate randomness into your JavaScript applications!
Randomly Generating Colors
Now that we’ve mastered selecting random elements and shuffling arrays, let’s dive into a colorful example. Generating random colors can be quite useful, especially when you’re trying to create eye-catching designs or visuals in JavaScript. Here’s how to generate a random RGB color:
// Generating a random RGB color function randomColor() { var r = Math.floor(Math.random() * 256); // Random between 0-255 var g = Math.floor(Math.random() * 256); // Random between 0-255 var b = Math.floor(Math.random() * 256); // Random between 0-255 return 'rgb(' + r + ',' + g + ',' + b + ')'; } console.log(randomColor());
This code generates a string in the format `rgb(r,g,b)`, with `r`, `g`, and `b` representing red, green, and blue components, respectively.
Creating Random Shapes
In web development and game design, creating random shapes can make the user experience more dynamic. The following example demonstrates how to create random circles with different radii and positions on an HTML canvas:
// Assuming we have a canvas with id="myCanvas" var canvas = document.getElementById('myCanvas'); var ctx = canvas.getContext('2d'); function drawRandomCircle() { var x = Math.random() * canvas.width; var y = Math.random() * canvas.height; var radius = Math.random() * 40; // max radius of 40 ctx.beginPath(); ctx.arc(x, y, radius, 0, 2 * Math.PI, false); ctx.fillStyle = randomColor(); ctx.fill(); } // Draw 5 random circles for(let i = 0; i < 5; i++) { drawRandomCircle(); }
The drawRandomCircle function creates a circle at a random position within the canvas boundaries with a randomly assigned color and radius.
Generating Random Positions
Moving elements around randomly can create an intriguing visual effect. Here’s an example of how you might randomly position an HTML element on the screen:
// Randomly position an element within the viewport function randomPosition(element) { var x = Math.random() * (window.innerWidth - element.clientWidth); var y = Math.random() * (window.innerHeight - element.clientHeight); element.style.position = 'absolute'; element.style.left = x + 'px'; element.style.top = y + 'px'; } var myElement = document.getElementById('myElement'); randomPosition(myElement);
This function takes an HTMLElement as an argument and positions it at a random location within the viewport, taking into account the element’s size.
Random Angles for Movement
In animations and games, you often need to move objects at random angles. Here’s a simple way to generate a random angle and move an object in that direction:
// Generate a random angle in radians function randomAngle() { return Math.random() * 2 * Math.PI; } // Move an object at a random angle function moveAtRandomAngle(object, speed) { var angle = randomAngle(); object.x += Math.cos(angle) * speed; object.y += Math.sin(angle) * speed; } // Assuming we have an object with x and y properties var myObject = { x: 100, y: 100 }; moveAtRandomAngle(myObject, 5); console.log(myObject);
The above function will update the given object’s `x` and `y` properties, moving it at a random angle based on the specified speed.
With an array of various random techniques at your disposal, you can begin to craft programs that exhibit complex, naturalistic behaviors and styles. The random elements integrated into your applications will elevate the dynamism and keep your users engaged with fresh, unexpected experiences.
Let’s continue enhancing our toolkit with more ways to employ randomness in our projects. We’ll delve into generating random speeds, directions, text, and even simulating random growth patterns. These examples build on the knowledge we’ve already gathered and push the boundaries of what we can achieve with pseudo-randomness in programming.
Random speeds can be useful for creating animations or simulating movement. Below is an example of how to assign a random speed to an object within a certain range.
// Assigning a random speed between minSpeed and maxSpeed function randomSpeed(minSpeed, maxSpeed) { return Math.random() * (maxSpeed - minSpeed) + minSpeed; } // Example usage var minSpeed = 2; var maxSpeed = 10; var myObjectSpeed = randomSpeed(minSpeed, maxSpeed); console.log("Random Speed: " + myObjectSpeed);
Randomly directing movement can also be interesting, especially when simulating wandering characters or objects in a game. Here’s a way to change an object’s direction randomly:
// Randomly changing an object's direction function changeDirection(object, speed, angleRange) { var angle = Math.random() * angleRange - angleRange / 2; // Angle deviation object.velX = Math.cos(angle) * speed; object.velY = Math.sin(angle) * speed; } // Assuming object has velX and velY properties var myObject = { velX: 0, velY: 0 }; changeDirection(myObject, myObjectSpeed, Math.PI / 4); // 45-degree range console.log(myObject);
Generating random text is another useful scenario, often used in captcha systems or for generating unique IDs and keys.
// Generating a random string of alphanumeric characters function generateRandomString(length) { var characters = 'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789'; var result = ''; for (var i = 0; i < length; i++) { result += characters.charAt(Math.floor(Math.random() * characters.length)); } return result; } // Example usage var randomString = generateRandomString(10); // Generate a string of length 10 console.log("Random String: " + randomString);
Simulating growth patterns, such as those seen in natural phenomena or simulations, can be achieved by combining random angles and distances. Here’s a basic example:
// Simulating a random growth pattern starting from (x, y) function growRandomly(ctx, startX, startY, steps, stepLength) { ctx.beginPath(); ctx.moveTo(startX, startY); for (var i = 0; i < steps; i++) { startX += Math.cos(randomAngle()) * stepLength; startY += Math.sin(randomAngle()) * stepLength; ctx.lineTo(startX, startY); } ctx.strokeStyle = randomColor(); ctx.stroke(); } var canvas = document.getElementById('growthCanvas'); var ctx = canvas.getContext('2d'); growRandomly(ctx, canvas.width / 2, canvas.height / 2, 10, 20);
Another compelling use is generating sounds with random properties; for instance, generating a random tone can add an element of unpredictability to an application’s audio feedback.
// Generating a random tone frequency within a given range function randomTone(minFrequency, maxFrequency) { return Math.random() * (maxFrequency - minFrequency) + minFrequency; } // Assuming we have an audio context and oscillator var audioContext = new (window.AudioContext || window.webkitAudioContext)(); var oscillator = audioContext.createOscillator(); oscillator.frequency.value = randomTone(200, 2000); // Random frequency between 200Hz and 2000Hz oscillator.type = 'sine'; // Pure tone oscillator.connect(audioContext.destination); oscillator.start();
Understanding and implementing randomness in coding is like harnessing the dynamic forces of chance within the controlled environment of algorithms. It allows the creation of more engaging, lifelike programs and applications. With practice, these random functions will not only be tools in your coding repertoire but also gateways to numerous possibilities, enhancing everything from user interfaces to game mechanics.
Where to Go Next with Your Coding Journey
Embarking on your programming journey through the fascinating realm of randomness is just the beginning. If you’re eager to expand your skills further, especially in one of the most versatile and high-demand programming languages, our Python Mini-Degree is the perfect next step. With courses ranging from the basics to complex algorithms, and even game and app development, you’ll find ample opportunities to grow your coding expertise.
The Python Mini-Degree encompasses project-based learning that will aid you in not just understanding concepts, but also applying them practically. Whether you’re a beginner or someone looking to deepen your understanding of Python and its applications, our curated courses will guide you through each learning stage at your own pace. Explore our Python Mini-Degree and take your coding skills to new heights.
And for those who wish to dive into a broader range of programming languages and technologies, we invite you to peruse our comprehensive selection of Programming courses. Enhance your proficiency and unlock new opportunities in your professional and personal coding projects. At Zenva, we’re committed to helping you transform your passion for technology into a tangible skill set that can propel your career forward.
Conclusion
Unveiling the intricacies of pseudo-random number generation is a testament to the beautiful complexity of programming. By harnessing this foundational principle, you’ve added a versatile tool to your developer’s kit—one that breathes life into games, simulations, and any application where the element of surprise adds that spark of vitality. Through practice and exploration, every random number, color, or movement you generate not only serves a purpose but also weaves a thread in the rich tapestry of your coding creations.
Remember, this is merely one step in a lifelong adventure of learning and discovery. Continue building, creating, and expanding your horizons with our Python Mini-Degree—a gateway to mastering Python and beyond. Whether it’s creating algorithms or designing your next big game, let each challenge fuel your journey, and may your code reflect the boundless potential of your imagination.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
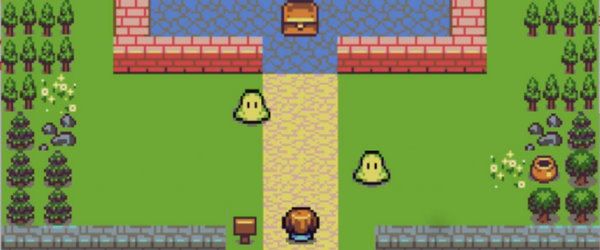
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.