Python is a versatile language that many developers use to process and analyze data. But what happens when that data is large, and exists in a structured format such as SQL? When working with larger databases, it can often be impractical (or even impossible) to load all data into memory. That’s where interfacing directly with SQL through Python comes into play.
Table of contents
What is SQL?
SQL (Structured Query Language) is a programming language specifically designed to handle data stored in a Relational Database Management System (RDBMS), or for stream processing in a Relational Data Stream Management System (RDSMS). Simply put, SQL is perfect for managing complex data with multiple relationships.
What is Python SQL Programming
Python SQL programming involves using Python to interface and execute SQL commands in an RDBMS. By using Python’s standard database API (DB-API), it’s possible to interact with a variety of different database systems, both relational and non-relational.
Why Learn to Use Python with SQL?
There are multitudes of reasons to learn how to use Python with SQL. Here are few reasons why it can be beneficial:
- Data stored in SQL databases is inherently relational, being able to leverage the Python’s ability to perform operations on these relationships can yield powerful insights.
- Python’s standard database API simplifies the process of interacting with your database, reducing the need for complex SQL queries.
- Learning Python SQL programming can expand your skill set and job prospects, as knowledge of both languages is highly sought after in the world of data analysis and big data.
In this tutorial, we’ll be diving into some practical examples that demonstrate how to use Python to work with large SQL databases. Stay tuned!
Setting up the Python Environment for SQL
Before we begin with the code examples, it’s essential to set up the Python environment for SQL. For this guide, we’ll use ‘sqlite3’ module which is included in the Python’s Standard Library.
# Importing the sqlite3 module import sqlite3 # Establishing a connection connection = sqlite3.connect('my_database.db') # Creating a cursor object cursor = connection.cursor()
Creating Tables with Python and SQL
Using Python, we can execute SQL commands to create tables in our database. Let’s create a simple table to store employee records:
# SQL command to create a table sql_command = """CREATE TABLE employee ( employee_id INTEGER PRIMARY KEY, full_name VARCHAR(20), age INTEGER, department VARCHAR(10));""" # Execute the command cursor.execute(sql_command)
Inserting Data into Tables
Let’s now add some data into our employee table.
# SQL command to insert data sql_command = """INSERT INTO employee VALUES (01, "John Doe", 29, "Marketing");""" cursor.execute(sql_command) # Commit your changes connection.commit()
Fetching and Displaying Data
We can retrieve data from our tables using Python as well. Let’s fetch some data from our table.
# SQL command to fetch data sql_command = """SELECT * FROM employee;""" # Execute and Fetch data cursor.execute(sql_command) records = cursor.fetchall() # Display the records for record in records: print(record)
Before finishing, always remember to close the connection. This can be done using the ‘close’ function.
# Close the connection connection.close()
This completes the first part of our Python and SQL tutorial. In the next part, we’ll delve deeper into the usage of SQL and Python together, such as querying databases, data manipulation, and more. Stay tuned!
Querying Specific Data
We’re not always interested in all the data in a table. In such cases, we can use the WHERE clause to filter the results. For example, let’s find all the employees that are from the Marketing department:
# Establish the Connection connection = sqlite3.connect('my_database.db') cursor = connection.cursor() # SQL command to fetch specific data sql_command = """SELECT * FROM employee WHERE department = 'Marketing';""" # Execute and Fetch data cursor.execute(sql_command) records = cursor.fetchall() # Display the records for record in records: print(record) # Close the connection connection.close()
Updating Records
Record updates are essential for any database. Let’s update the department of one employee using Python:
# Establish the Connection connection = sqlite3.connect('my_database.db') cursor = connection.cursor() # SQL command to update a record sql_command = """UPDATE employee SET department = 'Sales' WHERE employee_id = 01;""" # Execute and commit the changes cursor.execute(sql_command) connection.commit() # Close the connection connection.close()
Deleting Records
Just like updating, deletion of records is a common task when dealing with databases. Let’s delete a record from our employee table:
# Establish the Connection connection = sqlite3.connect('my_database.db') cursor = connection.cursor() # SQL command to delete a record sql_command = """DELETE FROM employee WHERE employee_id = 01;""" # Execute and commit the changes cursor.execute(sql_command) connection.commit() # Close the connection connection.close()
Using Aggregate Functions
Aggregate functions perform calculations on a set of values and return a single value. Common SQL aggregate functions include COUNT, SUM, AVG, MAX, and MIN. Let’s use the AVG function to find the average age of employees in our table:
# Establish the Connection connection = sqlite3.connect('my_database.db') cursor = connection.cursor() # SQL command to find the average employee age sql_command = """SELECT AVG(age) FROM employee;""" # Execute and Fetch data cursor.execute(sql_command) average_age = cursor.fetchall() # Display the result print("Average Age of Employees: ", average_age[0][0]) # Close the connection connection.close()
With these Python SQL programming concepts, you should now be able to create, manage, and manipulate a SQL database using Python. In the next tutorial, we will discuss some more advanced SQL operations that can be performed using Python. Stay tuned!
Moving Forward with Python and SQL
You’ve now got a foundational understanding of Python SQL programming – you’re able to create, manage, and manipulate SQL databases using Python. But your journey doesn’t stop here. The sphere of Python and SQL is vast and offers limitless opportunities for continued learning and improvement.
If Python has sparked your interest and you’re ready to level up your skills, we invite you to check out our comprehensive Python Mini-Degree program. This program offers a collection of courses covering various topics such as coding basics, algorithms, object-oriented programming, and even game and app development. From building your games and apps to creating AI chatbots, the mini-degree ends up fueling your passion for Python and transforming it into useful, practical skills.
If you’re seeking a more broad repository, you can browse our collection of Python courses. With over 250 supported courses and beginner to professional level content, you can continue to master Python at your pace, on your terms.
At Zenva, our passion is to help individuals across the globe to gain the skills they need to boost their careers and make their dreams come true. As you continue learning with us, we aim to provide you with the knowledge and guidance you need to go from beginner to professional. Whether you want to start your own business, land your dream job, or simply expand your horizons, we’re here to support your growth every step of the way.
Conclusion
With the power of Python and SQL at your fingertips, you’re opening the door to a world of potential. You’ve conquered the basics, grasped the framework, and are ready to venture further into the endless possibilities that this skill set enables. But remember, this is only the beginning of the journey.
We’re inviting you to take the next step with us at Zenva and propel your skills to new frontiers. Our comprehensive Python Mini-Degree program and Python courses are specifically designed to guide you along your learning journey and to empower you to conquer the digital world. With each lesson, you’ll transform your curious flame into a roaring fire of knowledge, skill, and confidence. Unleash your potential with Zenva today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
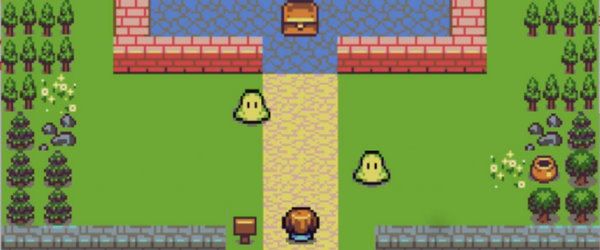
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.