Welcome to this beginner-friendly guide on Python’s “try-except” handling! We’ll unpack this important concept in a way that’s both easy to understand and immediately applicable to your Python projects. Let’s dive in.
Table of contents
What is Python’s ‘try-except’?
In Python, ‘try-except’ is a mechanism for handling exceptions. It helps us to write a safer and more robust code, which is better prepared for unexpected circumstances.
So, why is this important? Well, errors and exceptions are almost inevitable in any coding process. Thus, understanding how to handle these exceptions is a crucial part of becoming a successful coder.
– It allows us to prevent our program from crashing when an exception occurs.
– It enables us to fix issues and debug more easily.
– It’s an essential skill for any Python developer, and its importance cannot be overstated!
Making your code robust against potential errors means fewer headaches down the line, and a smoother experience for users. In essence, the function of the ‘try-except’ block is kind of like a safety net for your digital acrobatics, making sure nothing goes awry mid-action!
Stay with us to learn how to use this key Python tool effectively! Your skills will level up in no time. Don’t worry if you are new to coding, we’ve got your back. This powerful tool is something that coders at every stage of their journey should master. Let the journey begin!
Basic Syntax of ‘try-except’
Here’s the basic syntax:
try: # Code that might raise an exception except ExceptionType: # Code to handle the exception
Within the ‘try’ block, you place the code that might raise an exception. If no exception is raised, the ‘try’ block completes as expected and the code within the ‘except’ block is skipped. If an exception is thrown, the rest of the ‘try’ block is skipped, and the ‘except’ block runs instead.
Simple ‘try-except’ example
try: print(1/0) except ZeroDivisionError: print("You can't divide by zero!")
In this code, we attempt to divide by zero, which throws a ZeroDivisionError. Because we’ve set up a ‘try-except’ block, this error is caught and our custom error message is printed out.
Handling Multiple Errors
You can also catch and handle multiple different types of exceptions with multiple ‘except’ blocks:
try: # Some code except ZeroDivisionError: # Handle a division by zero except TypeError: # Handle trying to process incompatible data types except: # An optional 'catch all' for any other types of exceptions
This is particularly useful when your ‘try’ block contains code that could potentially raise more than one type of exception.
‘try-except’ with ‘else’
Additionally, Python allows an ‘else’ clause on the ‘try-except’ block which will be executed if the ‘try’ clause does not raise an exception.
try: print("Hello, World!") except: print("Something went wrong!") else: print("Nothing went wrong!")
In this case, because printing “Hello, World!” does not raise an exception, “Nothing went wrong!” would be printed out.
‘try-except’ with ‘finally’
Python also supports a ‘finally’ clause, which is a block of code that will be executed no matter if an exception is raised or not. This is really handy for cleanup tasks, such as closing a file.
try: print("Hello, World!") except: print("Something went wrong!") finally: print("Executing final clean up code.")
In the above example, whether an exception is raised or not, “Executing final clean up code.” will always be printed.
Raising Exceptions
Sometimes, we actually want to cause an exception when a certain condition is met, rather than trying to prevent one. In Python, you can manually raise exceptions using the ‘raise’ keyword.
x = 10 if x > 5: raise Exception('x should not exceed 5. The value of x was: {}'.format(x))
This can be a powerful tool when used in conjunction with ‘try-except’. You can raise an exception when something goes wrong and then catch it and handle it in an ‘except’ block.
‘try-except’ with custom exception classes
In Python, you can define custom exceptions by creating a new class. This new class should derive from the built-in Exception class.
class CustomError(Exception): pass try: raise CustomError except CustomError: print("A custom exception occurred.")
Here, we define CustomError as a new type of Exception. We then raise and catch this exception within a ‘try-except’ block.
Nested ‘try-except’ blocks
In Python, ‘try-except’ blocks can also be nested within one another. This can be a powerful tool for catching and handling exceptions at multiple levels of your code.
try: # Outer try block try: # Inner try block except InnerException: # Handle inner exception except OuterException: # Handle outer exception
In this way, Python’s ‘try-except’ handling can be incredibly flexible, allowing you to precisely control how your program reacts to various potential exceptions at different levels of execution.
Where To Go Next?
You’ve explored the basics of Python’s ‘try-except’ handling, you’ve seen it in action, and now you’re probably wondering: what’s next?
Python Mini-Degree
At Zenva, we believe in continuing the learning journey. That’s why we offer more comprehensive courses like the Python Mini-Degree.
This collection of courses goes beyond the basics, delving into broader areas of Python programming like object-oriented programming, game and app development, and even coding basics and algorithms. You’ll also learn to work with popular Python libraries and frameworks such as Pygame, Tkinter, Kivy, and PySimpleGUI.
Here’s what you can expect from our Python Mini-Degree:
- Courses designed for beginners and intermediate learners.
- Projects that let you apply your learning directly by creating arcade games, a medical diagnosis bot, a to-do list app, and more!
- Flexibility with 24/7 access to the courses
- Instructors who are certified and experienced in programming and teaching.
By the end of these courses, you’ll be ready to build a portfolio of Python projects and prepare yourself for a career in programming. Python is ubiquitous in various industries, including data science, AI, and robotics. The knowledge you’ll acquire is both versatile and highly sought-after.
Intermediate Python Learning
If you’re past the basics and looking for a challenge, we’ve got you covered. Check out our intermediate Python courses for a more advanced learning experience.
Remember: learning, just like coding, is a journey, not a destination. So whether you’re a beginner or an intermediate learner, we at Zenva are here to support you every step of the way in your coding journey. Let’s keep exploring the opportunities in Python together!
Conclusion
Today, we’ve discovered the power and versatility of Python’s ‘try-except’ handling. We’ve seen how it can enhance your coding skills, increase the robustness of your programs and truly skyrocket your development workflow.
The incredible world of Python awaits you with its endless opportunities! Keep exploring, keep learning, and keep pushing your boundaries. Remember, at Zenva, we’re on this journey with you. So, when you’re ready to take the next big step in your coding journey, join us for our comprehensive Python Mini-Degree. Until then, happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
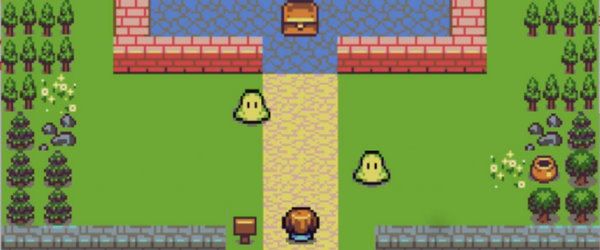
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.