Welcome to the exciting world of Python programming! This tutorial will take you through the Python pprint module – a tool that makes your life a bit easier when working with complex data structures. It’s a small but powerful tool that has a significant impact on how you read, understand, and debug your code.
Table of contents
What is the Python pprint module?
The pprint module in Python is a built-in module used for pretty-printing. In the world of programming, “pretty-printing” refers to displaying data structures like dictionaries, lists, and nested lists in a well-organized and more readable format.
Why Should I Learn pprint?
Aside from making your code output organized and neat, the pprint module is valuable for debugging complex applications. It allows you to read and understand your data structures at a glance, which ultimately leads to more effective and efficient debugging.
Even for experienced coders, pprint can be handy, especially when working with complicated projects or large datasets. It’s an incredibly useful tool that no Python programmer should be without.
Let’s begin our journey with Python’s pprint module. We’ll start from the basics and gradually move to more intricate examples.
Importing the pprint module
The first step is to import the pprint module in Python. Here’s how:
import pprint
Now, let’s print a simple dictionary using pprint.
import pprint data = {'name': 'John', 'age': 30, 'city': 'New York'} pprint.pprint(data)
Running the above code will output:
{'age': 30, 'city': 'New York', 'name': 'John'}
Working with Nested Lists
Where pprint truly shines is when working with nested lists. Let’s see it in action:
import pprint nested_list = [['apple','orange','grapes'],['carrot','beans','potato'],['dog','cat','giraffe']] pprint.pprint(nested_list, width=30)
The width parameter adjusts the width of the output, allowing you to display more data comfortably.
Customizing Indentation
We can also customize the indentation using the indent parameter.
import pprint data = {'name': 'John', 'age': 30, 'city': 'New York'} pprint.pprint(data, indent=4)
The above code enhances readability by adding indentation.
Handling Depth in Nested Structures
For very deep data structures, we can limit the nesting level using the depth parameter.
import pprint nested_list = [['apple','orange','grapes'],['carrot','beans','potato'],['dog','cat','giraffe']] pprint.pprint(nested_list, depth=1)
This will only print up to the first nesting level, handy when dealing with deeply nested structures.
Sorting Keys in Dictionaries
The sort_dicts parameter can be handy when you want to sort a dictionary by its keys. By default, it’s set to True. Here’s how it’s done:
import pprint data = {'banana': 3, 'apple': 4, 'pear': 1, 'orange': 2} pprint.pprint(data, sort_dicts=False)
The code keeps the original order of the dictionary keys and doesn’t sort them.
Compact Printing
The compact parameter helps to save space by fitting more data on each output line.
import pprint data = {'banana': 3, 'apple': 4, 'pear': 1, 'orange': 2} pprint.pprint(data, compact=True)
Running this code will display the data in a compact manner that uses the space more efficiently.
Using pprint for Printing JSON Data
pprint can also come in handy when you’re working with JSON data. JSON data often takes the form of complex, nested structures that can be hard to follow. Here’s an example:
import pprint import json json_data = '{"name": "John", "age": 30, "city": "New York", "pets": ["dog", "cat"]}' json_parsed = json.loads(json_data) pprint.pprint(json_parsed)
The code above parses a JSON data string and then uses pprint to print it in a well-organized format.
Printing Objects with pprint
We’re not just limited to the basic data types. With pprint, we can also print instances of a class!
import pprint class Person(object): def __init__(self, name, age): self.name = name self.age = age john = Person('John', 30) pprint.pprint(john.__dict__)
Here, we’ve printed out the attributes of an instance of the class Person by accessing its __dict__ attribute, which returns a dictionary of the instance’s attributes.
Where to Go Next
Mastering the pprint module is just the beginning of your Python journey. There’s a whole universe of knowledge waiting to be explored.
Check out our Python Mini-Degree. It’s a comprehensive collection of courses that cover various aspects of Python programming, from coding basics and algorithms to object-oriented programming, game development, and app development.
You’ll learn by creating real-world apps, games, and algorithms through step-by-step projects. Our curriculum is designed for both beginners and experienced programmers and can be accessed 24/7 on any device.
Python is a widely-used programming language, and mastering it can open up opportunities in numerous fields like data science, game development, and robotics. Our courses come equipped with interactive lessons, quizzes, and certificates upon completion. Whether you’re seeking a career change, your dream job, or kickstarting your own business, our courses can help you achieve your goals.
In addition to the Python Mini-Degree, we offer a wider collection of Python-focused courses. Check out our comprehensive list of Python Courses to continue bolstering your Python prowess.
Conclusion
Python is a versatile language with a rich selection of modules, and the pprint module is one such treasure. Learning how to use pprint effectively is an invaluable skill that can drastically enhance readability and debugging in your Python applications.
Continue to explore Python and its countless capabilities with us at Zenva. You can accelerate your learning journey with our Python Mini-Degree. With Zenva, you’re not just learning, you’re creating, building, and mastering. Join us as we continue to shape the future through code!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
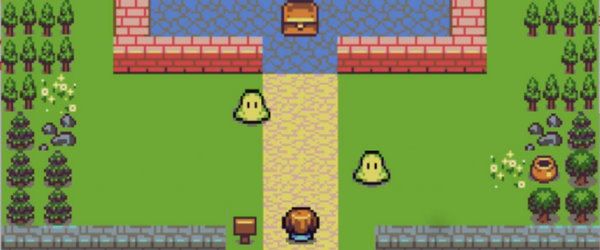
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.