Today, we invite you to delve into an integral aspect of Python programming – connecting to MySQL databases using the MySQLdb module. This opens up immense possibilities to build applications with robust database functionalities. Whether you are beginner or a seasoned coder, this comprehensive guide will equip you with the necessary know-how to leverage MySQL databases in your Python projects.
Table of contents
What is MySQLdb?
MySQLdb is a powerful third-party module in Python that allows interaction with MySQL databases. This interface enables seamless data exchange between your Python programs and MySQL databases, making it crucial for any data-handling application.
Why Use MySQLdb?
Python’s MySQLdb module offers several compelling features:
- It supports a multitude of SQL data types.
- Simultaneously handles multiple connections to different databases.
- Provides Python-compatible data conversion routines.
Learning MySQLdb connection in Python has become indispensable for Python developers, particularly those working on applications involving data storage, manipulation, and retrieval.
How Does MySQLdb Work?
MySQLdb essentially builds a bridge between your Python programs and MySQL databases. It translates Python method calls into SQL commands, executes them, and conveys the results back to your program.
Connecting to MySQL Database with Python
Before you even create or manipulate your data, you need to establish a connection with your MySQL database using Python. In general, connecting to the MySQL database involves four key steps:
- Importing the MySQLdb module
- Specifying your database details
- Establishing the connection
- Creating a cursor object
Here’s a basic example illustrating these steps:
import MySQLdb db = MySQLdb.connect(host="localhost", user="username", password = "password", db="database_name") cursor = db.cursor() print("Connected to MySQL database")
In the above code, replace `username`, `password`, and `database_name` with your specific MySQL credentials.
Executing SQL Queries with Python
Once connected, you can use the `cursor.execute()` method to execute SQL queries:
cursor.execute("SELECT VERSION()") data = cursor.fetchone() print("Database version : %s " % data)
On execution, the script will output the version of your MySQL database.
Creating Tables with Python
Let’s dive in further and create a table in our database:
cursor.execute("DROP TABLE IF EXISTS EMPLOYEE") sql = '''CREATE TABLE EMPLOYEE( FIRST_NAME CHAR(20) NOT NULL, LAST_NAME CHAR(20), AGE INT, SEX CHAR(1), INCOME FLOAT)''' cursor.execute(sql)
This script first drops the `EMPLOYEE` table if it exists, then goes on to create it.
Inserting Data into Tables with Python
Now, let’s learn how to insert data into a table:
sql = """INSERT INTO EMPLOYEE(FIRST_NAME, LAST_NAME, AGE, SEX, INCOME) VALUES ('John', 'Doe', 30, 'M', 7000)""" try: cursor.execute(sql) db.commit() except: db.rollback()
Always remember to use `commit()` method to save the changes and `rollback()` method to revert in any event of an error.
Inserting Data Dynamically
Often, you will need to insert data dynamically, i.e., the data to be inserted wouldn’t be hard-coded as in the previous example. The MySQLdb interface provides an effective and safe way to handle such situations.
sql = "INSERT INTO EMPLOYEE(FIRST_NAME, LAST_NAME, AGE, SEX, INCOME) VALUES (%s, %s, %s, %s, %s)" data = ('Jane', 'Doe', 25, 'F', 8000) try: cursor.execute(sql, data) db.commit() except: db.rollback()
Here, the data to be inserted is kept separate from the query string, thus preventing SQL injection attacks.
Selecting Data from Tables
To select data from a table, you can use the `SELECT` statement. Below is an example:
sql = "SELECT * FROM EMPLOYEE \ WHERE INCOME > '%d'" % (1000) try: cursor.execute(sql) results = cursor.fetchall() for row in results: fname = row[0] lname = row[1] age = row[2] sex = row[3] income = row[4] print("fname=%s,lname=%s,age=%d,sex=%s, income=%d" % \ (fname, lname, age, sex, income )) except: print("Error: unable to fetch data")
Updating Data in Tables
You can use the `UPDATE` statement to modify data in your tables.
sql = "UPDATE EMPLOYEE SET AGE = AGE + 1 WHERE SEX = '%c'" % ('M') try: cursor.execute(sql) db.commit() except: db.rollback()
The above code will increment the age of all male employees by 1.
Deleting Data from Tables
Lastly, to delete data from your tables, you can use the `DELETE` statement.
sql = "DELETE FROM EMPLOYEE WHERE AGE > '%d'" % (20) try: cursor.execute(sql) db.commit() except: db.rollback()
With all these examples, you should now be equipped with a robust foundational knowledge of interacting with MySQL databases using the MySQLdb interface in Python. It’s time to start building applications with sturdy database functionalities!
Where to Go Next?
Congratulations on completing this guide! You are now well-versed in communicating with MySQL databases using the MySQLdb interface in Python. The journey has just begun, though, and there is still so much more to explore. We encourage you to keep moving forward, building and honing your skills, and applying what you’ve learned in new and challenging projects.
We provide an array of resources to guide you on your journey. One avenue you could explore is our comprehensive Python Mini-Degree. The Python Mini-Degree is a collection of crash courses designed to immerse you in the versatile world of Python programming. You will journey through key aspects of Python, including coding basics, understanding algorithms, and exploring object-oriented programming. But it doesn’t stop there!
In-context, project-based learning is at the heart of our teaching methodology. As you progress, you’ll get to create your own games, algorithms, and real-world applications. With all the fascinating projects you’ll undertake, you’ll also have the perfect content for a standout Python portfolio to showcase your skills to prospective employers.
Python is a buzzword in the tech industry, particularly in data science domains. Our Python Mini-Degree is tailored to suit learners at various stages of their learning journey, from beginners to more experienced programmers. The program offers flexibility and accessibility, designed for seamless navigation on any device.
The teaching modules include live coding lessons and quizzes to ensure a thorough grasp of the subject matter. At the conclusion of each course, you will be awarded a certificate of completion.
You can also explore our vast collection of Python courses that cover a wide array of subjects in Python programming. These additional resources will bolster your skillset, presenting you with even more opportunities to excel.
Conclusion
Connecting to MySQL databases using Python’s MySQLdb module is an essential skill for any budding or experienced Python programmer. It allows you to leverage the strengths of programming and database management – a powerful combination in our data-centric world.
We at Zenva are committed to helping you advance in your learning journey. Our Python Mini-Degree is an excellent starting point. You can build on the knowledge you’ve gained here and take your Python programming skills to the next level. We look forward to helping you become even more competent and confident in Python!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
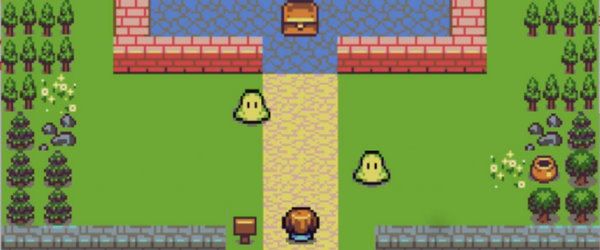
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.