Welcome to this insightful and super engaging tutorial on Python’s list data type. Whether you’re just kick-starting your coding journey or are a seasoned programmer, our comprehensive guide will surely give you a fresh perspective on Python lists.
Table of contents
Understanding Python Lists
Python lists are a type of data structure that allow storage of multiple items in a single variable. These items, also known as elements, can be of diverse types, like numbers, strings, even other lists, and more. Python lists are undoubtedly one of the most versatile data structures used universally in coding environments.
Python lists hold a special place in the realm of coding because they’re:
– Easy to use and understand – making them perfect even for absolute beginners.
– Extremely adaptable – lists can hold different types of data simultaneously.
– Dynamically resizable – can adjust their size on-the-fly, unlike arrays in some languages.
Having a good grasp of Python lists is therefore integral to becoming efficient at Python coding.
Python even allows you to manipulate lists in various ways such as finding the length of the list, accessing elements, slicing, and more. These operations help provide greater control over the data, making Python programming both powerful and flexible.
Now that you’re aware of ‘what’ Python lists are, ‘why’ you should learn them, and ‘what’ you can do with them, let’s dive into some coding tutorials to bring these concepts to life.
Our coding examples will be centered around simple gaming scenarios for added fun and better understanding. Let’s get our hands ‘code-dirty’!
Python Lists: Getting Started
Let’s start by creating our very first Python List.
# Creating a simple Python list monsters = ['Goblin', 'Dragon', 'Zombie'] print(monsters)
In the above code, we’ve created a Python list monsters containing three elements. We then print the list to the console.
Accessing Elements
You can access individual elements in a Python list using their index.
# Accessing elements in Python list print(monsters[0]) # Output: Goblin print(monsters[2]) # Output: Zombie
In Python, index is zero-based. So, the first element is at index 0, the second at index 1, and so on.
Modifying Elements
Python lists are mutable, meaning, you can change their elements.
# Modifying a Python list monsters[1] = 'Werewolf' print(monsters) # Output: ['Goblin', 'Werewolf', 'Zombie']
Here, we’ve replaced the second element ‘Dragon’ with ‘Werewolf’.
Adding and Removing Elements
Python provides methods like append() and remove() to add and remove elements.
# Adding and Removing elements from Python lists monsters.append('Vampire') # Adding element print(monsters) # Output: ['Goblin', 'Werewolf', 'Zombie', 'Vampire'] monsters.remove('Zombie') # Removing element print(monsters) # Output: ['Goblin', 'Werewolf', 'Vampire']
In the above code, we first add ‘Vampire’ to our list, and then remove ‘Zombie’.
These are just basics and Python lists can do a lot more. In the next section, we’ll explore more advanced list operations.
Finding the Length of the List
You can find the size of a Python list using the len() function.
# Finding the length of a Python list print(len(monsters)) # Output: 3
This tell us that there are three monsters in our list.
Slicing a List
Slicing allows you to get a subset of the list.
# Slicing a Python list print(monsters[1:3]) # Output: ['Werewolf', 'Vampire']
The above code will print a subset of the list from index 1 to 2. The end index in Python slicing is exclusive.
Sorting a List
Python provides the sort() method to sort the elements in a list.
# Sorting a Python list monsters.sort() print(monsters) # Output: ['Goblin', 'Vampire', 'Werewolf']
Sort() will sort the elements in increasing order of their values. Here, the monsters are sorted alphabetically.
Nested Lists
A Python list can even contain other lists.
# Creating a nested Python list worlds = [['Earth', 'Mars', 'Venus'], ['Middle-earth', 'Narnia']] print(worlds[1][0]) # Output: Middle-earth
We’ve created a list worlds that has two lists as its elements. Each list contains names of imaginary or real worlds. We can access ‘Middle-earth’ using its indices.
Iterating Over a List
You can use a for loop to iterate over the elements in a list.
# Iterating over a Python list for monster in monsters: print(monster)
This will print each monster one by one.
Python lists are powerful and mastering them is paramount to using Python effectively. We encourage you to play around with the above examples and create some of your own to gain a hands-on understanding. Happy coding!
Where To Go Next?
You’ve made a great start by learning about Python lists. However, the Python programming universe is vast and full of exciting opportunities for you to continue exploring.
A great place to keep going is our Python Mini-Degree. It’s our most comprehensive collection of Python courses. You get the opportunity to consolidate and enhance your Python skills through exciting hands-on projects. By the end of the mini-degree, you’ll have an impressive portfolio featuring games, real-world apps, and algorithms all created by none other than you!
This plethora of Python goodness covers a multitude of topics like coding basics, data science applications, object-oriented programming, and even game and app development. The knowledge you gain will certainly open doors to a myriad of industry segments such as data science, AI, and game development. All our courses are flexibly designed to allow 24/7 access, so you can learn at your own pace, at any place.
Upon successful completion of your courses, we provide certificates, which many of our students have used to land jobs or even start their own businesses.
Furthermore, we have an expansive collection of Python courses that cater to every Python enthusiast’s needs. Be sure to check out our full range of Python Courses for a broader perspective on what you can do with Python.
Your learning journey has only just started. There’s so much more to explore, and we can’t wait to see you grow your skills with Zenva. Continue your Python adventure today and keep on coding!
Conclusion
With Python lists, you’ve unlocked a powerful tool to efficiently manage and organize your data during coding. The journey you’ve embarked upon today paints but a small portion of the canvas that encapsulates the magic world of Python programming. But remember, every grand masterpiece begins with a single stroke.
As you delve deeper into Python, don’t forget to check out our extensive range of courses, including our comprehensive Python Mini-Degree. It’s been designed to fuel your coding journey with a multitude of engaging, real-world projects and interactive learning. Keep exploring, keep learning, and remember, every line of code takes you one step closer to your coding masterpiece. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
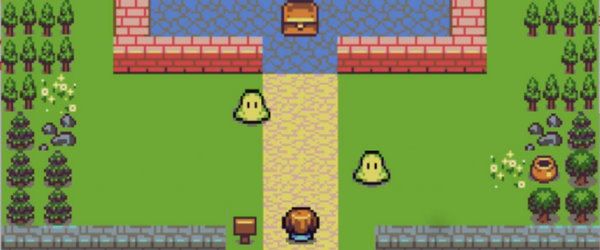
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.