When you start to dig into Python, you swiftly discover it’s packed with useful tools that can simplify your coding life. One such tool is the Counter class from the Collections module.
Table of contents
What are Python Counters in Collections?
Python Counters are part of the collections module, which provides alternatives to general-purpose containers like dictionaries, lists, tuples, and sets. More specifically, Counters are a subclass of the dictionary and are designed to count hashable objects, which makes them invaluable when dealing with a large volume of data that needs quantifying.
Why Learn Python Counters?
Python Counters can simplify complicated counting tasks, especially with larger data sets. By using the Counter class, you’re able to count items in a list or occurances of specific characters in a string, and more, with a simplistic, easy-to-understand syntax.
Python Counters are highly prized in data management, making them an asset in fields like data analysis, machine learning, and game mechanics. So, whether you’re exploring big data or designing an epic game battle system, Counters are an essential tool to have in your Python toolkit.
End of part 1. Remember, your work should be in HTML format.
How to Use Python Counters: Basic Examples
Let’s start with a basic example of using the Counter class to count the number of occurrences in a simple list.
from collections import Counter my_list = ['apple', 'banana', 'apple', 'pear', 'apple', 'banana', 'pear', 'apple'] count = Counter(my_list) print(count)
You should see the following output:
Counter({'apple': 4, 'banana': 2, 'pear': 2})
Each unique object in the list has a corresponding count of its occurrences.
So, what happens if we apply the Counter to a string instead of a list?
from collections import Counter my_string = "I love to code with Python!" count = Counter(my_string) print(count)
Here’s the output:
Counter({' ': 5, 'o': 4, 'I': 1, 'l': 1, 'v': 1, 'e': 1, 't': 2, 'c': 1, 'd': 1, 'w': 1, 'i': 1, 'h': 1, 'P': 1, 'y': 1, 't': 1, 'h': 1, 'n': 1, '!': 1})
End of part 2.
Digging Deeper: Advanced Python Counter Examples
Now that you’ve got the basics down, let’s explore some of the more advanced features Python Counters offer.
Counter Arithmetic
Python Counters support addition, subtraction, intersection, and union operations.
from collections import Counter counter1 = Counter(['apple', 'banana']) counter2 = Counter(['apple', 'pear']) # Addition result = counter1 + counter2 print("Addition: ", result) # Subtraction result = counter1 - counter2 print("Subtraction: ", result) # Intersection result = counter1 & counter2 print("Intersection: ", result) # Union result = counter1 | counter2 print("Union: ", result)
Sorting Items In A Counter
You can use the Counter’s methods combined with Python’s sorted() function to sort items.
from collections import Counter count = Counter('banana') # Sorting items sorted_items = sorted(count.items(), key=lambda x: x[1], reverse=True) # Display sorted items for i in sorted_items: print(i)
Most Common Elements
The most_common() method allows you to retrieve the elements that occur the most often.
from collections import Counter # Creating a new Counter count = Counter('abcdefgabcabcabc') # Three most common elements print(count.most_common(3))
Working with Unseen Keys
A regular dictionary hits you with a KeyError if you try to access a non-existent key. Counter, however, returns a zero count.
from collections import Counter count = Counter('abcdefgabcabcabc') print(count['z'])
Python Counters offer an efficient and straightforward way of handling counting challenges. Its additional features make it especially valuable for operating with large quantities of hashable objects. As part of the Collections module, it’s just one of the conveniences Python offers to make your coding life a tad bit easier. Start implementing it in your Python applications today!
Where to Go Next to Advance Your Python Journey?
If you found the power and functionality of Python Counters exciting and want to deepen your understanding of Python, we at Zenva have something that’s just right for you! Our all-inclusive Python Programming Mini-Degree is a comprehensive collection of Python-focused courses to take you from beginner coder to capable Python programmer.
This collection of courses explores a wide range of Python applications including coding basics, algorithms, object-oriented programming, and even game and app development. Thus, providing you a vast ocean of opportunities to build your own games, applications, and tackle real-world projects.
Our learners have used the skills they acquire from our courses to publish games, land jobs, and start businesses. Python is a highly sought-after skill in the job market, especially in data science. This makes our Python Programming Mini-Degree an essential tool to jumpstart or advance your career in tech.
In addition to the Mini-Degree, we offer a wider collection of standalone Python courses tailor-made for specific learning needs.
Now that you’ve ignited your Python journey with Python Counters, it’s time to further spark your love for Python with us at Zenva! Happy coding!
Conclusion
Grappling with Python’s collections module and the Counter class doesn’t have to be a hair-puller. As we’ve demonstrated, understanding the core concept followed by real-world examples can turn this seemingly tough nut into an exquisite delicacy. Now you’re armed with a fresh quiver of Python tools, ready to conquer any counting challenge you encounter in your coding journey!
Whether you’re a beginner setting your first steps in the world of Python or an experienced programmer looking to fine-tune your skills, our Python Programming Mini-Degree encompasses all you need to soar you to new heights. Python’s charm lies in its simplicity and versatility. Let’s conquer it, one Python Counter at a time, together at Zenva!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
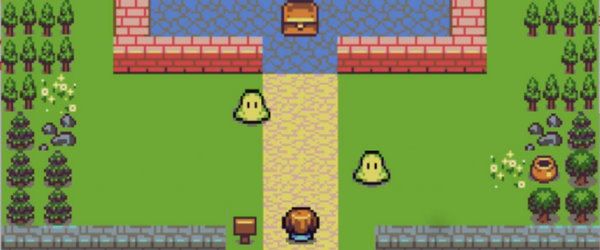
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.