Welcome fellow coders and gaming enthusiasts! Today, we’re diving into an exciting and useful Python library known as ‘numba’. Whether you’re nurturing your beginner coding enthusiasm or an experienced programmer looking for opportunities to level up your skills, this tutorial is a perfect match for you.
Table of contents
What is Numba?
Numba, simply put, is a Python library that can dramatically speed up your data-heavy and computationally intense Python applications. It does this by dynamically compiling parts of your code to machine language, making it closer to the speed of execution that a language like C++ would offer.
Irrespective of your project targets, if you’re dealing with large amounts of data, computation heavy tasks, or things that require serious performance- think data analysis or graphics- Numba can speed up your code execution like magic.
Although Python is a favourite among many coders for its readability and simplicity, as a higher-level language it can often run slower compared to languages closer to machine code, like C. Here’s where Numba comes to your rescue, letting you keep all the advantages of Python, while supercharging your code’s execution speed. With big data and high performance computing pervading across domains- gaming, AI, data science- mastering Numba can be an amazing addition to your developer toolkit.
So, are you ready to supercharge your Python skills with Numba? Let’s delve right in! We have an array of simple yet illustrative examples ahead, which will allow you to grasp the essence of this powerful tool in no time.
Getting Started with Numba
Before we proceed with code, we need to ensure that Numba is installed. If not, you can easily install it using pip.
pip install numba
Do make sure you’re using Python version 3.7 or later so everything runs smoothly and you can make the best out of what Numba has to offer!
A Simple Numba Function
First, let’s create a simple Python function and use Numba to compile it. You will see how easy it is to use Numba.
import numba @numba.jit def addition
Using the Numba JIT Compiler
The first step to using Numba is annotating your function with the @numba.jit decorator. Here is a simple function that adds together two numbers:
import numba @numba.jit def addition(a, b): return a + b print(addition(5, 10))
As you can see, there is nothing too complex about the function itself, but the @numba.jit decorator tells Numba to compile this function using the JIT compiler.
Speed Comparison
Now, let’s actually compare the speed of our compiled function to the standard Python function:
import numba import time # Normal Python function def python_addition(a, b): return a + b # Numba function @numba.jit def numba_addition(a, b): return a + b # Time the execution of both functions start = time.time() python_addition(5, 10) print("Python Time: ", time.time() - start) start = time.time() numba_addition(5, 10) print("Numba Time: ", time.time() - start)
In this example, even though the time difference might be very small, but for larger, more complex operations, Numba can result in significant speed improvements.
Adding More Complexity
Until now, our function was pretty simple. Let’s take this up a notch and see how numba deals with more complex functions:
import numba # Numba function @numba.jit def numba_complex(a, b): result = 0 for i in range(1000): result += a**2 + b**3 return result print(numba_complex(5, 10))
Although the operation seems complex, Numba has no problem in executing it, and with a lower time of execution.
Using Numba with NumPy
Numba works very well with NumPy, the hugely popular Python library for numerical computations. Let’s see an example:
import numba import numpy as np # Numba function @numba.jit def numba_array(arr): return np.sum(np.sqrt(arr)) # Create a large array arr = np.arange(1000000) print(numba_array(arr))
Here, Numba compiles the function and optimizes it to work with large NumPy arrays. The result is faster execution speed and improved performance.
Where to Go Next
Congratulations! You’ve taken the first steps to supercharging your Python code with Numba. Of course, there’s much more to explore in this powerful library.
Moreover, learning to code is a journey, with each step building upon the previous one. If you’re passionate about Python or looking to step into the exciting careers in coding, game development, or AI, our Python courses are an excellent next step.
In fact, we highly recommend checking out our Python Mini-Degree. This is much more than just a course. It’s a comprehensive program that guides you from the basics of Python programming, all the way through creating your own apps, games, and AI chatbots!
This Mini-Degree program has been carefully curated to not just teach, but to provide an interactive, project-based learning where you’ll create your own projects. This hands-on approach to learning results in a deeper understanding and more importantly, leaves you with a professional portfolio, showcasing your skills to future employers.
Our Python Mini-Degree is a practical, results-oriented program suitable for learners at every level. Regardless of your experience, you’ll have access to expert mentors ready to answer your questions and guide you along the way. We believe in providing content that keeps up with industry developments, ensuring that our learners are always at the forefront.
Conclusion
Exploring Python and its expansive universe of libraries like Numba is a rewarding adventure that keeps unfolding with each new skill you acquire. The beauty of Python entrusts you with a powerful tool to handle anything from simple scripting to advanced machine learning applications, while libraries like Numba help you to optimize your code for performance.
So, why wait? Multiply your Python superpowers with our Python Mini-Degree today! Let’s continue our journey in the vast world of Python together, with the only limit being your own creativity. Remember, every line of code you write brings you one step closer to where you want to be. Start your journey with Zenva today and let’s see where your code takes you!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
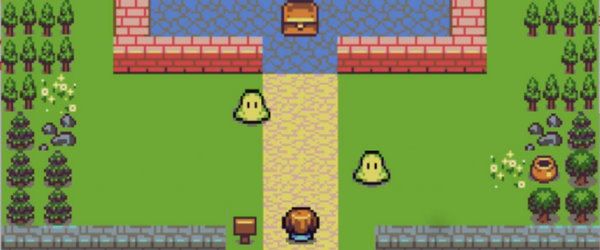
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.