In the rich world of programming, mastering the solid blocks is of paramount importance no matter your skill level. If you’re an aspirant or a proficient coder, understanding the ropes of the C# language is instrumental. In this enlightening tutorial, we focus on one such construct of C#, the this keyword. We aim to give you a clear, demonstrative understanding of how it works and how to apply it effectively in your coding journey.
Table of contents
What is ‘this’ in C#?
The this keyword in C# is a reference variable pointing to the current object in a method or a constructor. It helps to distinguish between class variables (fields) and method parameters when the naming is identical.
What is ‘this’ Used for in C#?
The this keyword in C# has some distinct use cases:
- It’s used for referring to instance variables of the same class.
- Possess the ability to pass the current object as a parameter to other methods.
- Often used to declare indexers.
Why Should I Learn ‘this’ in C#?
Are you pondering why learning the this keyword is essential in C#? Here is why:
- It improves the readability of your code by distinguishing between instance variables and local variables.
- The this keyword plays a vital role in implementing certain design patterns such as the builder design pattern, factory pattern, etc.
- Getting a handle on the this keyword propels you closer to mastering C#, especially for game development in Unity, where C# is extensively used.
Stay tuned to delve deep into the workings of the this keyword through illustrative examples, explanation and more!
Using ‘this’ to Refer to Instance Variables
A common usage of the this keyword is to clarify which variables belong to an instance of a class when local variables of the same name are present. It’s more like self-resolution within the class.
public class Clock { int hours; public void SetTime(int hours) { this.hours = hours; } }
In the code above, this.hours refers to the class’s instance variable, while hours uses the method’s parameter.
Using ‘this’ to Pass Current Object to Other Methods
Another handy use case for the this keyword is to pass the current object as a parameter to other methods. This pattern is commonly seen in event handlers and callback functions.
class Program { void LogProgramDetails(Program program) { Console.WriteLine(program.ToString()); } void RunProgram() { // Pass current object to other method LogProgramDetails(this); } }
In the example above, the LogProgramDetails method takes an instance of Program as a parameter. Inside RunProgram, we’re passing the current Program instance using the this keyword.
Using ‘this’ to Declare Indexers
One interesting aspect of C# is that it provides an elegant way to work with arrays and collections through indexers. The this keyword is handy when used to declare indexers in a class.
public class MyClass { private string[] array = new string[5]; // Indexer declaration public string this[int index] { get { return array[index]; } set { array[index] = value; } } }
Here, we utilized the this keyword to add an indexer to our class. This allows objects of MyClass to be accessed using [ ] index notation, just like an array!
Using ‘this’ in Extension Methods
C# allows the creation of extension methods – adding extra methods to existing types, without modifying the original type. In extension methods, the this keyword is used to denote the type being extended.
public static class StringExtensions { public static string FirstThreeLetters(this String str) { return str.Substring(0, 3); } }
In this code, we extended the System.String class by adding a FirstThreeLetters method – this simply returns the first three letters from any string.
Digging Deeper into ‘this’ Keyword
Let’s delve further into the diverse applications of the this keyword, which make our code easier to understand and our classes more versatile.
Chaining Constructors with ‘this’
In C#, you can use the this keyword to invoke one constructor from another within the same class. This is termed as constructor chaining.
public class Circle { private double radius; //Constructor with no parameter public Circle() : this(1.0) { } //Constructor with a parameter public Circle(double radius) { this.radius = radius; } }
In the code, we use the this keyword to invoke the one-parameter constructor from the no-parameter constructor. This allows us to set a default value for the radius if no arguments are provided.
‘this’ as Return Value
It is possible to use this as a return value from a method to permit method chaining.
public class Box { private int width; public Box SetWidth(int width) { this.width = width; return this; } } Box box = new Box(); box.SetWidth(10);
Here, we’ve written a method that updates the width of a Box object and returns this, referring to the own updated object.
Using ‘this’ in Properties
The this keyword is used in properties to refer to the current instance.
public class Student { private string name; public string Name { get { return this.name; } set { this.name = value; } } }
In the example above, we are using this to clarify that we are referring to the instance variables when getting and setting the value of the Name property.
‘this’ in Delegates
Finally, we consider the this keyword in the context of delegates. A delegate is a type-safe function pointer, guiding which method to call.
public class Animator { public delegate void AnimationDelegate(); public event AnimationDelegate PerformAnimation; public void StartAnimation() { this.PerformAnimation?.Invoke(); } }
In this case, we have used the this keyword to call the delegate method within our class.Great! Let’s dive deeper into using the this keyword in C#, detailing how it can make your code more readable and versatile.
Implementing Interface Members Explicitly with ‘this’
When a class implements an interface, the interface’s members can be implemented explicitly using the this keyword. This is especially useful when a class could implement multiple interfaces with conflicting member names.
public interface IShape { void Draw(); } public class Circle : IShape { void IShape.Draw() { Console.WriteLine("Drawing circle."); } }
Although this isn’t used directly, we’re employing explicit interface implementation which works similarly. When you call Draw() on an IShape object, it’ll know exactly which Draw() method you’re referring to.
‘this’ in Anonymous Types and Lambdas
The this keyword shines in the concise syntax of anonymous types and lambda expressions. It helps to access containing type’s members within these compact constructs.
public class Calculator { private int x = 10; public void Compute() { var multiplyByTwo = new Func(y => this.x * 2); Console.WriteLine(multiplyByTwo(5)); // Output: 20 } }
In this example, we used this inside a lambda to reference a field of the Calculator class.
‘this’ in Exception Handling
Exception handling is a crucial part of any application. The this keyword becomes handy when extending classes with standard exception handling methods.
public static class ExceptionExtensions { public static string FullMessage(this Exception ex) { if (ex.InnerException == null) return ex.Message; else return ex.Message + " --> " + ex.InnerException.FullMessage(); } }
In this extension, this is used to access the Exception instance. Now, all exceptions thrown in your application can use the FullMessage() method to print a detailed message including any inner exceptions.
‘this’ in Asynchronous Programming
If you’re into modern C# programming, you can’t escape the asynchronous programming paradigm. The this keyword becomes handy when extending classes related to asynchronous operations.
public static class TaskExtensions { // This method ignores the exception if the task fails. public static async void IgnoreException(this Task task) { try { await task; } catch { /* Ignore the exception */ } } }
Here, this is used on the Task object as we’re extending it. We’ve defined an IgnoreException() method that swallows any exceptions that a task may throw.
These are some ways on how the this keyword can be beneficially leveraged in diverse aspects of the C# language. Wrapping up, it’s clear how mastering this can take you a step forward in crafting efficient, maintainable and well-structured code.
Where to Go Next
Great work! You’ve taken a significant step forward in your coding journey by mastering the this keyword in C#. But the learning doesn’t stop here! If you’re interested in progressing with Unity, where C# is extensively used, our comprehensive Unity Game Development Mini-Degree is waiting for you.
Our Unity course will enrich you with game mechanics, cinematic cutscenes, audio effects, custom game assets, procedural maps, enemy AI, and animation. Completing these courses can aid in building a portfolio of Unity games and projects. Remember, Unity is not only ruling the game industry, but it’s also being adopted in non-game industries like architecture, film, education, and healthcare.
For an even broader collection, feel free to explore our Unity courses. In the world of coding, the journey never ends, and with Zenva, you can confidently stride from beginner to professional. Ready to keep learning? Happy coding!
Conclusion
Knowing your C# fundamentals can provide a strong platform for diving into game design or systems programming. Equipped with a better understanding of the this keyword, you’re one step closer to becoming a C# maestro. By no stretch is C# limited to games. C# also finds extensive use in designing and coding software for the web, mobile, and enterprise environments.
So, are you ready to deepen your knowledge and become proficient in coding? Check out our comprehensive Unity Game Development Mini-Degree. Becoming a part of Zenva, you’re signing up for more than just online courses, you’re entering a community of doers, creators, and ever-learners. Remember, there’s no limit to how far you can soar with the right guidance!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
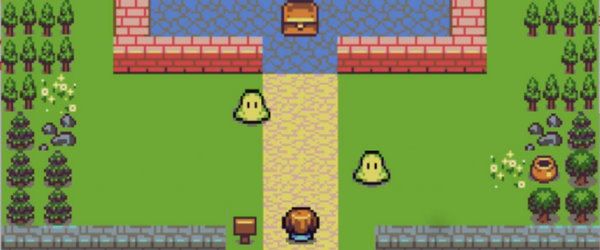
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.