Welcome to our deep dive into the world of GDScript static typing! If you’ve been keeping pace with the modern field of game development, you already know that static typing plays an increasing role for efficient and seamless coding practices. In this extensive tutorial, we’ll harness the power of static typing in GDScript to build a stronger foundation for your coding skills.
Table of contents
What is GDScript Static Typing?
GDScript Static Typing is a versatile tool we can use to define variable types in our code. Static typing may sound daunting for beginners, but fear not -remember, every guru was once a beginner. As we dive in, you’ll find that static typing not only makes your code cleaner but also less prone to errors.
Why Should I Learn GDScript Static Typing?
Static typing is all about clarity and precision. The more information you provide about a function or an object in your code, the more efficient and bug-free your coding becomes. Here are some reasons to learn GDScript static typing:
- Code Understanding: When your codebase grows, it becomes tough to keep track of variable types. Static typing helps you remember what kind of values each variable represents.
- Efficiency: Code debugging becomes faster since the interpreter throws errors during compile-time itself if a variable is assigned a different datatype than specified.
- Performance: GDScript can optimize your code when the datatype is known, resulting in better performance in your games.
Now that we understand the importance of GDScript static typing, let’s get our hands dirty with some code! Get ready to write code that’s clean, efficient, and intuitive! Stay tuned as we dive deeper into GDScript static typing.
Understanding the Basics of GDScript Static Typing
Mastering static typing in GDScript requires understanding its elementary parts. Let’s begin with defining variables using static typing.
var health: int = 10
Where “health” is the variable name, “int” is the datatype, and “10” is the assigned value. This line defines “health” as an integer with a value of 10.
Let’s apply static typing to an array.
var items: Array = []
Here “items” is an empty Array. You can only add ‘Array’ type items to “items”.
Static Typing with Functions
Static typing can also be used with function parameters and return types for precision.
For example, defining a function using static typing would look like this:
func add(a: int, b: int) -> int: return a + b
The function ‘add’ takes two integer arguments, ‘a’ and ‘b’, and returns their sum, which would also be an integer.
Type Inference
When variables are initialized immediately, GDScript can infer their types without needing explicit declaration. This is called Type Inference and can be used like this:
var health := 10
This variable ‘health’ would be an integer type because of the assigned value “10”. The colon equals sign (:=) is the operator for type inference.
Optional Typing
Sometimes, we might not know the type of a variable when we declare it. We can use ‘null’ or ‘Variant’ (the most general type in GDScript) in such cases.
For example:
var player = null var item: Variant
In these cases, the ‘player’ variable is set to ‘null’ and ‘item’ is a Variant type. As we proceed with our game development, these variables can hold any data type.
We have now covered the basics of static typing in GDScript. It’s time to put your gained knowledge into practice and make your code more efficient and bug-free!
Soft and Hard Static Typing
GDScript supports both soft and hard static typing. Soft typing warns us when a variable is re-assigned a value of a different type, whereas hard typing can prevent that from happening.
Soft typing is the default type, and its variables can be re-assigned to any type:
var health: int = 10 health = "Healthy" # This is completely fine and valid
However, if we use the hard typing denoted by `:=`, we can initialize using an inferred type which cannot be changed later:
var health := 10 health = "Healthy" # This will throw an error
Static Typing and Object-Oriented Programming
Static typing offers advantages in Object Oriented Programming as well. Here’s how you can define a custom datatype using a script:
class_name Player var name: String var health: int
And use it in our static typing:
var player := Player.new()
Here we can see that we are initializing a new object of the ‘Player’ class and assigning it to the `player` variable. This propels our code towards clarity and makes it bug-free.
Function Overloading with Static Typing
With static typing, you can have multiple functions with the same name and different parameters. This is called function overloading:
func attack(enemy: Enemy): print("Attack an enemy") func attack(enemy: Enemy, weapon: Weapon): print("Attack an enemy with a weapon")
In the code above, the ‘attack’ function is overloaded – it has two definitions with different parameters.
Conclusion
At Zenva, we strive to empower coders to reach their maximum potential. Starting with the basics, we explored the value and functionality of static typing in GDScript, and shared numerous implementation examples. With static typing, sturdy, less error-prone, and efficient code is now right at your fingertips. So take this knowledge, apply it in your exploration of game development, and stay tuned for more enriching tutorials!
Safe Lines in GDScript Static Typing
In GDScript, variables that have been defined with a static type can’t trigger type errors. This assurance is made for variables accessed directly, but not for those accessed dynamically (through get, set, call).
A line of code in GDScript where no type error can occur is called a “safe line”. To mark expressions which can introduce type errors, the safe keyword is used:
var variant_type = 30 var safe_string: String = "Hello, Zenva!" safe_string = variant_type # Unsafe assignment
In this example, the last line is considered unsafe and would trigger an error in GDScript because it violates the rules of static typing.
Static Typing with Arrays
Arrays can also benefit from static typing. An array can be declared having a particular type of elements like so:
var int_array: Array[int] = [1, 2, 3, 4] var string_array: Array[String] = ["apple", "banana", "pear"]
In these examples, ‘int_array’ can only contain integers, while ‘string_array’ can only contain strings. Any attempt to assign a different value would result in a type error.
Handling Nil
When working with static typing, handling null (or Nil in GDScript) becomes important. We define an object as Nil, if we want to allow that our variable could be null. Take a look at this example:
var player: Player = null player.health = 30 # This results in an error
In this case, as player is ‘null’, trying to assign a value to ‘health’ will generate a null instance error. With typing, we are forced to consider the possibility of null objects and handle them correctly:
if player != null: player.health = 30 # No error because we checked for null
This way, typing prevents nor from calling methods or accessing properties of null instances, which could crash the game.
GDScript’s Type Hierarchy
In GDScript, a variable of a given type can also accept any value of a subtype, thanks to the concept of “inheritance”. This is best illustrated with an example:
var node: Node = Button.new() # Button is a subclass of Node
In this code snippet, even though we declared ‘node’ as type ‘Node’, we can assign it a new ‘Button’ instance because ‘Button’ is a subclass of ‘Node’. This is a major feature of GDScript’s type hierarchy and promotes flexibility in the code.
That’s the depth of versatility that learning GDScript static typing can offer. Keep practicing these concepts and you’ll soon get the hang of static typing in GDScript.
Continuing Your Learning Journey
Acquiring new skills is not a destination but a journey, and with the power of GDScript static typing now in your arsenal, you’re more equipped than ever before to delve deeper into the world of game development!
At Zenva, we are dedicated to providing high-quality, accessible avenues for driven learners like you. Whether you’re just starting out or strengthening existing abilities, we have numerous courses tailored to suit your needs, taking you from beginner to professional.
Our Godot Game Development Mini-Degree is a compact yet comprehensive collection of online courses designed to help you master the Godot 4 engine. With it, learn to render graphics, use GDScript, create gameplay control flow, combat systems, UI design, and much more, all at your own pace.
If you’re ready to further explore the expanse of Godot, you can check out our broader collection of Godot courses. Embark on this enriching journey with Zenva today and catapult your coding skills to the next level!
Conclusion
Steeping yourself in the retrospect of what we’ve explored in this comprehensive tutorial, it’s clear how GDScript static typing can enhance the efficiency and reliability of your game code. Armed with this potent tool, you’re now prepared to create robust, optimized, and bug-free games!
Your learning journey doesn’t stop here, Zenva has knowledge vistas waiting to be explored and mastered. So why wait? Embark on our Godot Game Development Mini-Degree and turn your game development dreams into reality. Continue your journey with us and find your coding prowess skyrocketing in no time!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
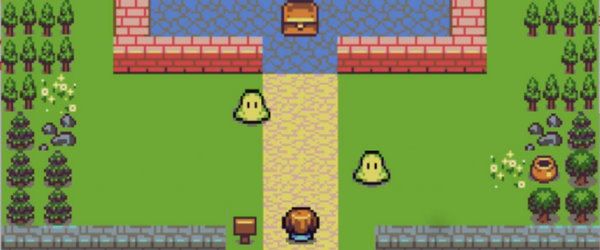
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.