Welcome to your next step in your programming journey! Today, we’ll cover a fascinating and versatile tool: converting curl commands to C#. If you’re someone who works with APIs or web services, chances are you’ve come across curl quite often. But what if you need to perform the same tasks in a C# application? That’s where learning to convert curl to C# becomes incredibly beneficial. It’s an integral skill to expand your programming repertoire and increase your projects’ flexibility.
Table of contents
What is curl?
curl is a command-line interface used for transferring data with URLs. It supports numerous protocols such as HTTP, HTTPS, FTP, and more. It’s a widely used tool for testing APIs, troubleshooting network issues, and downloading files from the internet.
What is C#?
C# (pronounced “C Sharp”) is a modern, object-oriented, and type-safe programming language. As part of the .NET ecosystem, C# is used extensively in enterprise applications, games, web services, and even mobile apps with the help of cross-platform frameworks like Xamarin.
Why learn to convert curl to C#?
Understanding how to convert curl commands to C# gives you a significant advantage in dealing with APIs or web services in a C# environment as it facilitates more streamlined testing and integration. This knowledge allows you to leverage the power of curl, well-known for its ability to interface with a myriad of protocols and services, yet keep these interactions encapsulated within your familiar and secure C# applications.
Is it complicated?
Not at all. While the idea of translating commands from one language to another can seem daunting, the process is very logical and systematic. This tutorial will guide you through the various steps involved in converting curl to C# code. Whether you’re a beginner in the world of programming or a seasoned coder, you’ll find this tutorial well within your reach.
Speaking of journeys, we understand that this is only one stop in your larger programming adventure. At Zenva, we firmly believe that learning is a continuous process, and we’re here to facilitate that with a variety of engaging resources on various technologies and concepts. After you’re clear on curl to C# conversions, consider broadening your horizons with our Unity Mini-Degree for those interested in game development. You’ll find it on our website here.
Let’s now get ready to dive into the incredible world of curl to C# conversion!
Getting Started with cURL
Before we get into converting cURL to C#, let’s dive into some basic cURL commands. Here are a few useful cURL commands that you’ll frequently encounter. Scripts were tested on Ubuntu 18.04, but they should work on any system with a bash shell.
Making a GET request
This is the simplest form of a cURL command to make an HTTP GET request.
curl http://example.com
Downloading a file with cURL
To download a file using cURL, we use the -O option.
curl -O http://example.com/myfile.txt
POST Request with JSON data
Posting JSON data can be done using the -d option, and setting the appropriate header with -H.
curl -d '{"key1":"value1", "key2":"value2"}' -H "Content-Type: application/json" -X POST http://example.com/endpoint
Sending headers with cURL
Headers can be sent with the -H option. This can be used to set the content type, send authentication tokens, and other info.
curl -H "Content-Type: application/json" http://example.com
Please note that while we’re using placeholder URLs (like http://example.com) in our examples for safety reasons, it’s essential that you replace them with the URLs relevant to you when testing these commands.
Converting cURL to C#
Now that we have some grasp on cURL, let’s get into how to perform these tasks with C#. Remember, C# is an object-oriented language, allowing us to use classes and objects to accomplish these tasks.
Making a GET request
With C#, you can make an HTTP GET request using HttpClient.
HttpClient client = new HttpClient(); var result = await client.GetAsync("http://example.com");
Downloading a file
Downloading a file with C# involves making an HTTP GET request and then writing the response content to a file.
HttpClient client = new HttpClient(); var response = await client.GetAsync("http://example.com/myfile.txt"); var content = await response.Content.ReadAsByteArrayAsync(); System.IO.File.WriteAllBytes("myfile.txt", content);
POST Request with JSON data
Sending a POST request with JSON data in C# requires serializing a .NET object to JSON and sending it as StringContent.
HttpClient client = new HttpClient(); var myObject = new { key1 = "value1", key2 = "value2"}; var json = Newtonsoft.Json.JsonConvert.SerializeObject(myObject); var data = new StringContent(json, Encoding.UTF8, "application/json"); var response = await client.PostAsync("http://example.com/endpoint", data);
Sending headers with C#
To send headers with an HttpClient in C#, you can simply add them to the DefaultRequestHeaders property of the HttpClient.
HttpClient client = new HttpClient(); client.DefaultRequestHeaders.Add("Content-Type", "application/json"); var response = await client.GetAsync("http://example.com");
Remember to replace the URLs examples with your actual ones when testing these C# snippets.
Advanced Operations in C#
As you get more accustomed to converting curl to C#, you’ll often find it necessary to undertake more complex tasks. Here are a few advanced operations that C# can handle efficiently:
Authentication with cURL and C#
Authentication is an integral part of interacting with web services. Let’s compare how we handle this with curl and C#:
With curl, we can send an authenticated request like so:
curl -u username:password http://example.com
In C#, we use the HttpClient.DefaultRequestHeaders.Authorization property to add an authentication header:
HttpClient client = new HttpClient(); var byteArray = Encoding.ASCII.GetBytes("username:password"); client.DefaultRequestHeaders.Authorization = new System.Net.Http.Headers.AuthenticationHeaderValue("Basic", Convert.ToBase64String(byteArray)); var response = await client.GetAsync("http://example.com");
Handling HTTP redirects
By default, curl doesn’t follow HTTP redirects. However, we can change this behavior with the -L option:
curl -L http://example.com
In C#, HttpClient automatically follows HTTP redirects by default. If you want to change this behavior, you must modify the HttpClientHandler:
HttpClientHandler httpClientHandler = new HttpClientHandler(); httpClientHandler.AllowAutoRedirect = false; HttpClient client = new HttpClient(httpClientHandler); var response = await client.GetAsync("http://example.com");
File Uploads with Multipart Form Data
Uploading files is a common task when dealing with web services. Here’s how to perform this operation in both curl and C#:
With Curl, we use the -F option to upload a file:
curl -F '[email protected]' http://example.com/upload
And in C#, it can be achieved as follows:
HttpClient client = new HttpClient(); var content = new MultipartFormDataContent(); content.Add(new ByteArrayContent(System.IO.File.ReadAllBytes("myFile.txt")), "file", "myFile.txt"); var response = await client.PostAsync("http://example.com/upload", content);
Conversion of cURL commands to C# requires a basic understanding of HTTP and a solid grasp on C# programming. It allows you to bring the power of cURL into your C# applications, leading to more efficient API interactions and code consistency. The knowledge you’ve gained through this tutorial will be of great benefit when dealing with APIs, making your developer journey a smooth and enjoyable one. We hope we’ve made this topic crystal clear, and remember, we’re here to support you. Keep on learning!
Advanced cURL to C# Conversion Techniques
Let’s explore some more advanced code conversions that can be particularly helpful when you’re working with more complex scenarios in C#.
Using Query Parameters
Query parameters are often used to filter or sort data in API calls. With curl, the query parameters are simply included in the URL:
curl http://example.com/api?param1=value1¶m2=value2
With C#, we can include query parameters using the UriBuilder class combined with the NameValueCollection class to manage multiple parameters efficiently:
var builder = new UriBuilder("http://example.com/api"); var query = HttpUtility.ParseQueryString(builder.Query); query["param1"] = "value1"; query["param2"] = "value2"; builder.Query = query.ToString(); HttpClient client = new HttpClient(); var response = await client.GetAsync(builder.ToString());
Configuring Timeouts
There may be situations where requests take longer than expected. It’s often good practice to set a timeout to prevent an endlessly hanging request.
With curl, we can set a timeout (in seconds) like this:
curl --max-time 10 http://example.com
In C#, we can accomplish this by setting the HttpClient.Timeout property:
HttpClient client = new HttpClient(); client.Timeout = TimeSpan.FromSeconds(10); var response = await client.GetAsync("http://example.com");
Working with Cookies
Many services require the use of cookies for purposes such as managing sessions or storing small amounts of data.
In curl, we can send cookies as follows:
curl -b "cookieName=cookieValue" http://example.com
In C#, it’s a little more involved as we need to use the HttpClientHandler and CookieContainer classes:
HttpClientHandler handler = new HttpClientHandler(); handler.UseCookies = true; handler.CookieContainer = new CookieContainer(); handler.CookieContainer.Add(new Cookie("cookieName", "cookieValue", "/", "example.com")); HttpClient client = new HttpClient(handler); var response = await client.GetAsync("http://example.com");
HTTP Versioning
For advanced control, you might want to specify which HTTP version your client should use when communicating with the server.
In curl, we specify the HTTP version using the –http option:
curl --http2 http://example.com
In C#, we can set the HTTP version using the HttpClient.DefaultRequestVersion property:
HttpClient client = new HttpClient(); client.DefaultRequestVersion = HttpVersion.Version20; var response = await client.GetAsync("http://example.com");
Converting curl commands to C# might appear challenging at first glance but with a little practice, it soon becomes a straightforward process. Remember, the ultimate goal is to enhance the communication between client and server, and streamline your workflow in C#. These examples should arm you with the knowledge and skills needed for any API challenges you might encounter. As always, we’re here to help you navigate your learning journey. Happy learning!
Take the Next Step in Your Learning Journey
By now, you should have a robust understanding of how to convert curl commands to C# and use this knowledge to streamline your web interactions within C# applications. However, remember that every programming journey is ongoing, with the learning never-ending. The great news is that we’re here to support you every step of the way.
You’ve seen firsthand the power C# holds within the realms of web services and APIs, but did you know that it’s also widely used in the exciting world of game development? If you’re intrigued by the idea of creating your own games, then we highly recommend considering our Unity Game Development Mini-Degree offered by Zenva Academy. This comprehensive program covers everything you need to get started with Unity, one of the most popular game engines worldwide. Unity’s potential isn’t limited just to games — it’s also widely used in the film, architecture, and education sectors.
Our highly-rated courses are project-based, accessible 24/7, and designed to be flexible to suit your schedule. You can build a diverse portfolio of Unity games and projects, gain in-demand skills, and potentially unlock fantastic opportunities within the gaming industry. You might also want to explore our broader collection of Unity Courses to further expand your learning.
Remember, with Zenva, you’re never alone on your coding journey. From beginner to professional, we’ve curated over 250 supported courses so that you can keep pushing your boundaries, learning new skills, and excelling in your field. Happy learning!
Conclusion
Congratulations on expanding your C# programming prowess! Converting curl commands to C# opens up a world of new possibilities for your projects, making them more adaptable and efficient, especially when interacting with APIs. Whether you simply want to add to your programming toolset or seek to empower your C# applications with the extensive functionalities of curl, we hope this guide offered valuable insights and guidance.
At Zenva, we’re on a mission to offer you comprehensive, high-quality, and engaging learning pathways. With the knowledge you’ve gained here, you might be eager to explore other areas of software development. Why not check out our Unity Game Development Mini-Degree to delve into game creation using C#? Remember, we’re here to accompany you on every step of your learning journey and help you unlock a world of possibilities.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
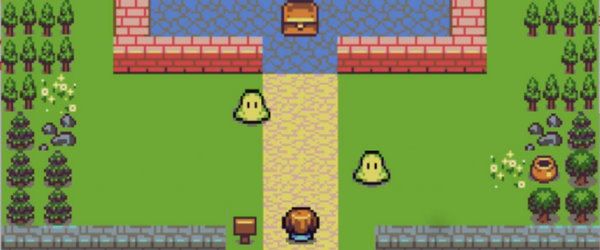
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.