Welcome to our tutorial on Clock Pygame, where we explore the powerful yet easy-to-use tool for creating interactive and dynamic games. We promise this will be an engaging journey where you’ll acquire valuable skills and knowledge.
Table of contents
What Is Clock Pygame?
Understanding Pygame is the precursor to mastering Clock Pygame. Essentially, Pygame is a collection of fun, powerful Python modules that let developers create video games. It’s open-source, meaning wide access and numerous possibilities.
At the heart of Pygame, we have the Clock Pygame. It’s an essential part of Pygame that interprets the time aspect. Without it, all your game elements would fall into chaos.
What Is Clock Pygame For?
Clock Pygame handles essential game elements like rendering, game updates, and character motion. It allows you to cap the frame rate to a specific number, control game speed, and achieve overall game synchronicity.
Without Clock Pygame, your changes from frame to frame would not be precise. In game development, precision is critical. If, for instance, your game updates too fast, it may work well on your computer but fail on slower ones. Luckily, Clock Pygame regulates this for you.
Why Should I Learn Clock Pygame?
Because it unlocks incredible potential for you! Understanding Clock Pygame lets you create engaging, dynamic games. It enables you to make your games universally playable, regardless of the player’s hardware speed.
Furthermore, it not only benefits you creatively but also professionally. The gaming industry is ever-expanding, and game developers are highly sought after. Learn Clock Pygame, and you equip yourself with a valuable asset in this profitable industry.
What’s more, learning Clock Pygame ties into the broader universe of understanding Pygame, making game development a breeze.
Are you ready now? Let’s delve into the engaging world of Clock Pygame!
Creating a Basic Clock
We can launch our tutorial with a basic clock setup. This setup involves initializing Pygame, then creating a clock object.
import pygame pygame.init() clock = pygame.time.Clock()
You’ve now succeeded in initializing Pygame and creating a Clock object!
Setting Frame Rate
Pygame lets you set a maximum frame rate for your game. To do this, we use the tick() function, presented in the coding example below.
<a class="wpil_keyword_link" href="https://gamedevacademy.org/best-fps-tutorials/" target="_blank" rel="noopener" title="FPS" data-wpil-keyword-link="linked">FPS</a> = 60 clock.tick(FPS)
Here, we’ve set the frame rate to 60 frames per second. The tick function helps maintain a relatively constant frame rate across different computers.
Maintaining Game Loop
In every game, there is a game loop that continues running as long as the game is active. Let’s setup a simple one.
running = True while running: clock.tick(FPS)
We’ve created a basic game loop. The run variable checks if the game is running. While the game is running, the frame rate is capped at the FPS we initialized earlier.
Calculating Time
The clock function also lets you calculate time between frames. You can keep track of this time by using the get_time() function.
frame_time = clock.tick(FPS) print("Time passed since last frame: ", frame_time)
In this situation, we print the duration between frames. The function get_time() is our savior here, returning the duration in milliseconds.
This wraps up the building blocks of using Clock Pygame. However, there’s always more to learn. Endless opportunities beckon in the realms of game creation. Next, we step further in-depth, combining these building blocks into full-fledged game development code. Are you geared up to continue this enthralling journey?
Controlling Event Loop
One valuable aspect of Clock Pygame is that it lets you control the event loop. This happens within the game loop we created earlier. You can check for events such as closing the game window.
running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False clock.tick(FPS)
Our game now detects if the game window is closed and ceases to run when it happens.
Creating Animation
Clock Pygame can also play a crucial role in animating game characters by controlling their frame rate. Let’s create a basic square animation.
import pygame pygame.init() WIN_WIDTH = 800 WIN_HEIGHT = 600 win = pygame.display.set_mode((WIN_WIDTH, WIN_HEIGHT)) clock = pygame.time.Clock() FPS = 30 WHITE = (255, 255, 255) BLUE = (0, 0, 255) x = 50 y = 50 width = 64 height = 64 vel = 5 run = True while run: clock.tick(FPS) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: x -= vel if keys[pygame.K_RIGHT]: x += vel if keys[pygame.K_UP]: y -= vel if keys[pygame.K_DOWN]: y += vel win.fill(WHITE) pygame.draw.rect(win, BLUE, (x, y, width, height)) pygame.display.update() pygame.quit()
In the above code, we built on the basics we’ve been learning so far. This code initiates a small square that can be moved around on the screen using the appropriate keys.
We create a window, set properties for our square, and add event listeners for key presses. When you run this code, you have a square that can move up, down, left, or right across the screen, with its frame rate controlled by Clock Pygame.
Moving Forward
Now you know how Clock Pygame paves the way for mastering game development. Take your time digesting these concepts and code snippets. Practice makes perfect, so be sure to write out and experiment with the examples shared here!
Our goal here at Zenva is to provide you with the tools necessary to turn you into a seasoned game developer and beyond. Remember, the journey of a thousand codes starts with a single function. Ready for the next level? It’s game on!
Tracking Time with Clock Pygame
Beyond limiting frame rates and synchronizing game loops, you can use Clock Pygame to keep track of time. For example, giving power-ups a certain duration.
start_ticks=pygame.time.get_ticks() #starter tick while run: #... seconds=(pygame.time.get_ticks()-start_ticks)/1000 #calculate how many seconds if seconds>10: # if more than 10 seconds close the game run = False
This script records the starting time (start_ticks), then calculates the elapsed time since then (seconds). If 10 or more seconds have passed, the game ends.
Adding Multiple Characters
Clock Pygame can do more complex tasks with ease, like controlling multiple characters.
WHITE = (255,255,255) BLUE = (0,0,255) RED = (255,0,0) class Character: size = 50 def __init__(self, x, y, color): self.x = x self.y = y self.color = color def draw(self): pygame.draw.rect(window, self.color, [self.x, self.y, self.size, self.size]) character1 = Character(50, 50, BLUE) character2 = Character(200, 200, RED) while True: clock.tick(FPS) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() window.fill(WHITE) character1.draw() character2.draw() pygame.display.update()
In this script, we defined a class for characters and created two instances. Each character is drawn in each game loop iteration, enabling them to exist simultaneously.
Adding user interactable characters
Allowing user control is another crucial part in game development. Clock Pygame can handle user-controlled characters.
class Player(Character): def move(self): keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: self.x -= 5 if keys[pygame.K_RIGHT]: self.x += 5 if keys[pygame.K_UP]: self.y -= 5 if keys[pygame.K_DOWN]: self.y += 5 character1 = Player(50, 50, BLUE) while True: clock.tick(FPS) for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() window.fill(WHITE) character1.move() character1.draw() pygame.display.update()
In this example, we have created a subclass Player inherited from Character. Players are able to move by pressing appropriate keys.
Please note that Clock Pygame’s functionality extends far beyond these examples. It’s a robust tool, with many intricacies deserving exploration. As we said earlier, the beauty of Clock Pygame lies within the Pygame framework, which is a gateway to more complex, rich game designs. Stay with us at Zenva as we show you the ropes in game development. Up for more fun in the magical world of Pygame?Awesome work getting to grips with Clock Pygame so far! The skills you’ve acquired are essential stepping stones on the path to mastering game development. But what’s the next move? How do you continue expanding your knowledge and skills?
We wholeheartedly recommend checking out our Python Mini-Degree. This detailed collection of Python courses covers everything from programming fundamentals to data structures, algorithms, and more advanced topics like game and app development. The courses are project-based and adaptable to your learning pace, so you can confidently deepen your Python skills and build a portfolio to impress potential employers. The Python Mini-Degree is an opportunity to learn the world’s most widely-used language and acquire real-world skills that are highly transferable.
Want to dive deeper into Python? Browse our catalog of Python Courses. With over 250 supported courses, Zenva offers tailor-made learning paths in programming, game development, and artificial intelligence, allowing you to progress from beginner to professional at your own pace.
Remember, a journey of a thousand lines of code begins with a single function. Keep coding, keep learning, and you’ll undoubtedly reach your goals. Onwards, brave coder!
Conclusion
Congratulations on your introduction to Clock Pygame! You’ve made a vital step in your game development journey with Python and Pygame. By practicing and tinkering with the code examples provided, you can take your understanding to greater heights. Remember, consistency and experimentation are the driving forces in mastering game development.
We encourage you to take your newfound knowledge even further with Zenva’s Python Mini-Degree. This consolidated learning path will guide you through the vast world of Python, cascading from fundamental to advanced concepts, while enabling you to create projects for your portfolio. At Zenva, we are passionate about helping learners like you, bridging the gap from beginner to professional developer. Happy Coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
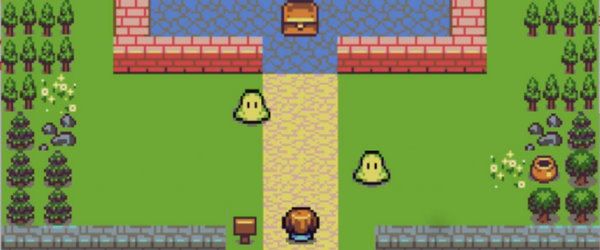
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.