In this article, we’ll be unraveling the mysteries of the “get” keyword in C#. Used widely in setting and retrieving data within objects, this crucial element of C# often baffles beginners. But fear not! We’ve got you covered, as we’ll dissect the concept with clear explanations and engaging examples.
Table of contents
What is the “get” keyword in C#?
The “get” keyword in C#, falls under the umbrella of ‘Properties’. A Property in C#, is somewhat a blend of a variable and a method, offering a more controlled way to set and retrieve an object’s properties.
They include a “get” accessor which provides a way to read the value of a private variable, and sometimes a “set” accessor that offers a way to assign a value. The “get” keyword offers a controlled way to read this data, ensuring that the encapsulation principle of Object-Oriented Programming isn’t violated.
Why Should I Learn About the C# “get” Keyword?
Understanding the “get” keyword in C# is fundamental due to several reasons:
- It fosters the practice of good software design principles, especially encapsulation, a key component of Object-Oriented Programming.
- Through its use in Properties, it eliminates the need for less secure public fields for data access.
- It ensures data integrity by providing controlled access to private variables.
Grasping how it works is thus essential for anyone aiming to be a proficient C# developer, or in game development using Unity engine, which heavily utilizes C#.
Creating a Basic Get Property in C#
To set the stage, let’s start off with a basic example showing how to create a get property in C#:
class Program { private string name; public string Name { get { return name; } } }
Here, we have a private variable ‘name’. We have a property ‘Name’ that, using the ‘get’ keyword, will return the value of ‘name’ when accessed. There is no ‘set’ accessor, so you cannot assign a value to ‘name’ via the ‘Name’ property.
Creating a Read-Write Property
Let’s develop our example to include ‘set’:
class Program { private string name; public string Name { get { return name; } set { name = value; } } }
Now, using the ‘set’ keyword, we can set a value to our ‘name’ variable through the ‘Name’ property. The value assigned to ‘Name’ will be placed in the ‘name’ variable.
Get and Property in Action
How does that apply outside of the class? Have a look:
class Program { private string name; public string Name { get { return name; } set { name = value; } } } Program p = new Program(); p.Name = "Learning C#"; Console.Writeline(p.Name);
In this example, we’re assigning the string “Learning C#” to our ‘Name’ property which sets the value of ‘name’. When we print the value of ‘Name’, it retrieves the value of ‘name’ through the ‘get’ accessor and prints “Learning C#”.
Expression-Bodied ‘get’ Property
Let’s not forget that C# 6.0 introduced the usage of lambda expressions to define ‘get’ and ‘set’ accessors. Here’s how:
class Program { private string name; public string Name => name; }
Just like our first example, this property only has the ‘get’ accessor, and cannot assign values. It still returns the value of ‘name’ when accessed.
Using Validation in Property Setters
Properties can prove particularly useful when needing to impose validations. Consider the following example:
class Program { private int age; public int Age { get { return age; } set { if (value 100) throw new ArgumentException("Invalid age value"); age = value; } } }
Here we’ve ensured that the ‘age’ variable isn’t set to an unacceptable value. An attempt to assign a negative value or a value over 100 throws an ArgumentException.
Working with Auto-Implemented Properties
C# also presents the convenience of auto-implemented properties, where you don’t need to declare a backing variable. Let’s see:
class Program { public string Hobby {get; set;} }
Without the need of an explicit private variable, ‘Hobby’ can still get and set values. The keyword ‘value’ in these cases refers to an implicit backing variable which C# creates automatically.
Read-Only Auto-Implemented Property
An auto-implemented property can also be made read-only:
class Program { public string Hobby {get; init;} }
The ‘init’ keyword introduced in C# 9.0 specifies that the property can only be set during the initialization or in the object initializer.
Let’s look at a try:
class Program { public string Hobby {get; init;} } Program p = new Program() { Hobby = "Coding" }; Console.Writeline(p.Hobby); // Outputs "Coding" p.Hobby = "Music"; // Error: The object is read-only
‘Hobby’ is successfully set to “Coding” during initialization. However, later attempts to change the value result in an error.
Auto-Implemented Property with Private Setters
Private setters give even more controlled access:
class Program { public string Hobby {get; private set;} }
You can only set the ‘Hobby’ property from within the class, protecting it from being arbitrarily changed from outside. This is a great example of encapsulation.
Using Getters with Classes and Structs
The real joy of using ‘get’ comes when we store complex data types such as classes or structs in our properties. Let’s dive in deeper. Imagine we have a simple class that represents a 3D vector:
class Vector3 { public float X, Y, Z; }
And a class ‘Entity’ that has a Vector3 instance ‘Position’ as a property:
class Entity { private Vector3 position; public Vector3 Position { get { return position; } set { position = value; } } }
Through the ‘Position’ property, we can control and retrieve the entirety of an entity’s position data. Ponder on this:
Entity entity = new Entity(); //Setting position entity.Position = new Vector3 { X = 10, Y = 20, Z = 30 }; //Getting the position Vector3 pos = entity.Position; Console.WriteLine($"Position: X:{pos.X}, Y:{pos.Y}, Z:{pos.Z}"); //Outputs: "Position: X:10, Y:20, Z:30"
We’re setting ‘Position’ and getting its individual components effortlessly. An important note here is that ‘get’ returns a copy of the ‘position’, not a reference, since ‘Vector3’ is a struct (value type). This guarantees data integrity.
Properties with Full Getters and Setters
Often, you want to execute some logic when setting a property, or computing a property’s value dynamically when getting it. For this, you can use a full property with both get and set:
class Square { private double sideLength; public double SideLength { get { return sideLength; } set { if (value < 0) sideLength = 0; else sideLength = value; } } public double Area { get { return sideLength * sideLength; } } }
In this example, trying to set ‘SideLength’ to a negative number will default it to zero. The ‘Area’ is computed when it’s accessed, always returning the correct value even as ‘SideLength’ changes.
Let’s have a look:
Square sq = new Square(); sq.SideLength = 5; Console.WriteLine(sq.Area); // Outputs: "25" sq.SideLength = -2; Console.WriteLine(sq.SideLength); // Outputs: "0" Console.WriteLine(sq.Area); // Outputs: "0"
We’ve only started to delve into the practical and vast utility of ‘get’. As you further your C# journey and learn more nuanced concepts, you’d grasp the striking role ‘get’ plays in effective and logical programming.
Where to Go Next
Your understanding of the ‘get’ keyword is a testament to the progress you’ve made in your journey as a budding C# programmer. But remember, it’s one step in a much broader and exciting journey. As your skills grow, so should your ambitions. So, what’s your next move?
We encourage you to keep expanding your programming skills, and an ideal way forward could be venturing into game development using Unity, one of the world’s most popular game engines.
Our Unity Game Development Mini-Degree at Zenva Academy is an extensive set of 20 courses featuring over 36 hours of content that takes you from creating simple 2D games to managing complex 3D environments, integrating audio effects, cinematic cutscenes, and more.
In more than 250 supported courses, Zenva offers both beginners and those who’ve passed the basic stage a chance to develop and consolidate their coding skills. Be it game creation, programming, or AI – we equip you with the knowledge to go from beginner to professional, with the flexibility to learn at your own pace.
Don’t set limits on your potential – check out our broad collection of Unity courses to begin or continue your journey into the fascinating realm of game development. Your passion combined with our knowledge can create extraordinary things!
Conclusion
Grasping the ‘get’ keyword in C# is a significant milestone, and we sincerely hope this guide has brought clarity and insight. Pat yourself on the back! You’ve added a potent weapon to your C# armory! But don’t stop there. Drive that momentum forward to explore the more exciting aspects of C# programming and beyond.
Look forward to stepping into the extraordinary world of game development, enhancing your C# skills, and expanding your understanding? That’s where Zenva steps in. Our comprehensive Unity Game Development Mini-Degree is your gateway to the fascinating universe of game creation. Leverage our resources to create not just games, but also your bright future. What are you waiting for? Your learning journey awaits you!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
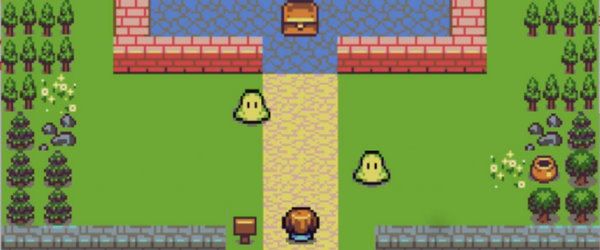
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.