Have you ever gotten lost in your code, searching for a specific element or value amidst a sea of data? Most programmers have, and that’s precisely why learning about C# find methods can be a true game-changer. In this comprehensive guide, we’ll explore this fascinating topic, with short, efficient examples that will help you understand and remember the principles.
Table of contents
What is C# Find?
The C# Find method is a handy tool that allows programmers to locate specific elements within arrays or collections. The function searches for the first element that meets a specific condition. We specify this condition through a function or a lambda expression.
Why Should I Learn It?
Well, mastering the Find method can save you hours of manual searching through data. It is important for all levels of developers, beginners and veterans alike. It helps simplify code, making it more readable and efficient. With numerous applications in game mechanics, data analysis or manipulation, learning to utilize the Find method effectively is certainly a valuable skill in the programming world.
Using the C# Find Method with Arrays
Let’s get started by using the Find method on an array of integers. We will search for the first number greater than 10:
int[] numbers = { 2, 9, 7, 14, 25, 30 }; int foundNumber = Array.Find(numbers, n => n > 10); Console.WriteLine(foundNumber);
This code will print 14 because it’s the first number in the array that’s greater than 10.
Utilising Multiple Conditions with the C# Find Method
The Find method can also handle multiple conditions. Here’s an example of how to find the first number that is both odd and greater than 10:
int[] numbers = { 2, 9, 7, 14, 25, 30 }; int foundNumber = Array.Find(numbers, n => n > 10 && n % 2 != 0); Console.WriteLine(foundNumber);
This code will print 25, the first number that meets both requirements.
Looking for Objects in C#
The Find method has applications beyond simple numeric arrays. We can use it to find specific objects in an array of objects. Consider this array of car objects, each has a make, model and year:
Car[] cars = { new Car { Make = "Toyota", Model = "Camry", Year = 2012 }, new Car { Make = "Honda", Model = "Accord", Year = 2010 }, new Car { Make = "Ford", Model = "Focus", Year = 2013 }, }; Car foundCar = Array.Find(cars, car => car.Model == "Accord"); Console.WriteLine($"Found car is a : {foundCar.Make} {foundCar.Model}");
This code snippet searches for the first car object that has “Accord” as a model. In our example, Honda Accord will be found and printed on the console.
Finding Elements In An Array Using a Separate Method
Sometimes, we want to use more complex logic when trying to find an element. This is where it becomes advantageous to use a separate method:
bool IsOddNumber(int n) { return n % 2 != 0; } int[] numbers = { 2, 9, 7, 14, 25, 30 }; int foundNumber = Array.Find(numbers, IsOddNumber); Console.WriteLine(foundNumber);
The code snippet above creates a function “IsOddNumber” and passes it as the condition into the Array.Find method. The printed output would be 9, which is the first odd number in the given array.
When The Find Method Can’t Find Anything
What happens when the Find method does not find an element that fulfils the given condition? Let’s find out:
int[] numbers = { 2, 4, 6, 8, 10 }; int foundNumber = Array.Find(numbers, n => n > 10); Console.WriteLine(foundNumber);
Since none of the numbers in the array are over 10, the Find method will return 0, the default value of integer in C#.
Using FindAll Method in C#
Sometimes we might want more than just the first result. The Array.FindAll method returns all elements that satisfy the condition. Let’s give it a try:
int[] numbers = { 2, 9, 7, 15, 24, 30 }; int[] oddNumbers = Array.FindAll(numbers, n => n % 2 != 0); foreach (int number in oddNumbers) { Console.WriteLine(number); }
This will output every odd number in the array: 9, 7, 15. The FindAll method is extremely helpful in capturing all relevant data from your array.
Using Last and LastOrDefault Methods
Now, what if we are interested in the last element that meets our condition, not the first? Enter the Last and LastOrDefault methods:
List numbers = new List { 2, 9, 7, 14, 25, 30 }; int lastOddNumber = numbers.Last(n => n % 2 != 0); Console.WriteLine(lastOddNumber);
This example prints the last odd number in the list: 25. It’s worth noting that these methods are for List, not Array.
Default Return Value in Find Method
Lastly, let’s see what the Find method returns when we look for a non-existing item or condition in the list:
List fruits = new List { "apple", "banana", "grape" }; string foundFruit = fruits.Find(fruit => fruit == "pear"); Console.WriteLine(string.IsNullOrEmpty(foundFruit) ? "Fruit not found" : foundFruit);
Since “pear” is not in our list, the Find function will return null.
In summary, the Find methods in C# are extremely versatile and can help programmers navigate their data with ease. We encourage you to practice and explore these methods further to enhance your coding efficiency and readability.
Using FindIndex in C#
Another useful function is the FindIndex method. It returns the index of the first element that satisfies the condition. Let’s check out an example:
List numbers = new List { 2, 9, 7, 14, 25, 30 }; int index = numbers.FindIndex(n => n % 2 != 0); Console.WriteLine(index);
The output here will be 1, the index of the first odd number in the list.
If you want to find the index of the last element that meets a condition, you can use the FindLastIndex method:
List numbers = new List { 2, 9, 7, 14, 24, 30 }; int lastIndex = numbers.FindLastIndex(n => n % 2 == 0); Console.WriteLine(lastIndex);
This time, it prints out 5, which is the index of the last even number in the list.
Performing Complex Search with Find and Predicate
When working with complex types, we can use the Find method with predicate, a function to define the conditions of the search. Here we will find a specific student in a list of students:
List students = new List { new Student { FirstName = "John", LastName = "Doe", Age = 21 }, new Student { FirstName = "Jane", LastName = "Smith", Age = 23 }, new Student { FirstName = "Bob", LastName = "Johnson", Age = 20 }, }; Student foundStudent = students.Find(s => s.FirstName == "Jane" && s.LastName == "Smith"); Console.WriteLine($"Found Student: {foundStudent.FirstName} {foundStudent.LastName}, Age: {foundStudent.Age}");
This should print: “Found Student: Jane Smith, Age: 23”. The Find method coupled with the Predicate function can indeed do wonders!
Using Find to Match Entire Collections in C#
Now, let’s use the Find method to match a whole collection of items:
List numbers = new List { 2, 9, 7, 14, 24, 30 }; List subNumbers = new List { 14, 24, 30 }; bool isSubset = numbers.FindAll(n => subNumbers.Contains(n)).Count == subNumbers.Count; Console.WriteLine("Is subset " + isSubset);
Here, the FindAll method is used to check if all elements in the subNumbers list are in the numbers list. The output “Is subset True” indicates that all elements of subNumbers do indeed exist in numbers.
Eliminating Elements with RemoveAll Method
Finally, the RemoveAll method will eliminate all elements from a collection that meet a specific condition:
List numbers = new List { 2, 9, 7, 14, 25, 30 }; int removed = numbers.RemoveAll(n => n % 2 == 0); Console.WriteLine("Removed " + removed + " items."); Console.WriteLine("Items left: " + numbers.Count);
This code removes all even numbers from our list and outputs “Removed 3 items. Items left: 3.”
There you have it! A thorough exploration of the Find methods in C#. As you can see, these methods provide powerful tools for finding, filtering, and manipulating data in your collections. We invite you to incorporate them into your code to enhance its readability, efficiency, and overall performance.
Where to go next with your learning?
As you continue to explore the exciting world of programming and game development, we invite you to delve even deeper by checking out our incredible Unity Game Development Mini-Degree. This comprehensive collection of courses will guide you through the vast landscape of Unity, one of the most renowned gaming engines globally, utilized in multiple industries beyond gaming, like architecture, film and animation, and healthcare.
Our Mini-Degree features 20 courses with 36 hours of content, giving you the chance to enhance your knowledge and skills and even create your own 2D and 3D games. Its curriculum includes challenging projects that will help you build your portfolio and shine in the industry. We aim to cater to all learning levels, offering content that can benefit the novice programmer and the experienced developer alike.
For a broader collection of courses, check out our Unity category. At Zenva, we provide the stepping stones for your learning journey, arming you with the key skills to go from a beginner to a professional. Join our community of over 1 million learners and start shaping the coding future today!
Conclusion
Understanding and using the Find methods in C# can elevate your programming practice to new heights. The ease of navigating, filtering, and manipulating data they provide is invaluable. As you step further into the fascinating landscape of coding and game development, remember: the key lies in continual learning and practice.
At Zenva, we’re here to support you on this journey. With our rich collection of courses, like our distinguished Unity Game Development Mini-Degree, we provide all the tools you need to succeed. Whatever your ambition, we can help you transform it into reality. So, take the plunge and start coding today!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
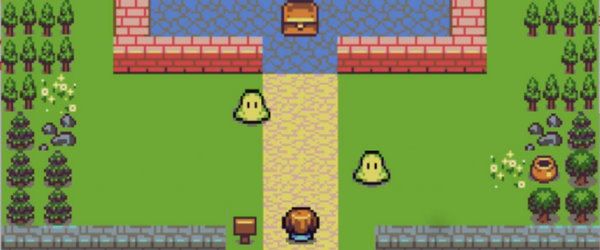
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.