Welcome to the exciting world of C++, a language renowned for its performance and efficiency. Within this comprehensive tutorial, we will explore a facet ripe with potential – the atomic operations in C++. If you are passionate about coding, game creation, or simply intrigued about the nuances behind smooth, concurrent programming, buckle up for a thrilling ride!
Table of contents
What Are Atomic Operations?
Think of atomic operations as the ‘unsung heroes’ behind the fluidity of multi-threaded applications. In C++, atomic operations involve handling operations that are carried out in a single, unbroken step.
Why Should I Learn Atomic Operations?
The beauty of atomic operations lies in their simplicity and power. Learning atomic operations can revolutionize the way you approach concurrent programming, ensuring your applications run smoothly without lags or glitches caused by race conditions.
Mastering this topic will not only refine your programming skills but also enable you to create more streamlined and efficient multi-threaded applications without worry of unexpected behavior. Atomic operations can be a game-changer, whether you are pursuing game creation, dynamic software development, or perhaps compiling an advanced computation program.
Unlocking the Potential of Atomic Operations
To truly leverage the strengths of atomic operations, you need to grasp both the ‘what’ and the ‘how’. This means knowing when to use them and understanding the step-by-step process of their implementation. Consider this the key to unlocking C++’s full potential and taking one step forward in your journey towards coding excellence.
Understanding the Basics: Atomic Variables
Before we dive into the actual atomic operations, let’s understand atomic variables. These are special types of variables in C++ that we can use in multi-threading to avoid race conditions.
Here is an example of how you can declare an atomic variable in C++:
#include <atomic> std::atomic<int> atomicVariable;
This statement declares an atomic integer. You can use it in your program just like a normal integer variable, but it has built-in protections against race conditions.
Atomic Operations: Load and Store
With atomic variables, you can carry out operations such as load and store. The load operation reads the value of the atomic variable, while the store operation updates the value.
Here’s an example of how to use these operations:
#include <atomic> std::atomic<int> atomicVariable(10); int value = atomicVariable.load(); // Loads the value into 'value' atomicVariable.store(20); // Changes the value of the atomicVariable to 20
Fetch Operations
C++ also provides operations that combine the load and store operations. These operations are known as fetch operations.
These operations are valuable because they ensure that the load and store operations happen as a single unbroken sequence. Here’s an example:
std::atomic<int> atomicVariable(10); int fetchedVal = atomicVariable.fetch_add(5); // Adds 5 to the atomic variable and returns the old value
Atomic Flags
Atomic flags are a kind of atomic variable that can only hold boolean values. They are useful for simple checks in your multi-threaded applications.
Here’s an example of how to use an atomic flag:
#include <atomic> std::atomic_flag flag = ATOMIC_FLAG_INIT; flag.clear(); if (!flag.test_and_set()) { std::cout << "Flag was not set before!"; }
This code initializes an atomic flag, clears it, and then tests and sets it. If the flag was not set before the call to test_and_set, it outputs the given message.
More Fetch Operations: Subtract and XOR
Fetch operations are not only limited to addition. C++ extends the convenience of fetch operations to subtraction and XOR operations.
The fetch_sub operation subtracts a given value from the atomic variable and returns the previous value. Let’s see how this works:
std::atomic<int> atomicVariable(10); int prevValue = atomicVariable.fetch_sub(5); // Subtracts 5 from the atomic variable and returns the old value
Similarly, the fetch_xor operation carries out an XOR operation on the atomic variable with a given value and returns the old value. Here’s a quick example:
std::atomic<int> atomicVariable(10); int oldValue = atomicVariable.fetch_xor(5); // Xors 5 with the atomic variable and returns the old value
Exchange Operation
To swap the value of an atomic variable with a new value and get the old value in return, you can use the exchange operation. Here’s how:
std::atomic<int> atomicVariable(10); int oldValue = atomicVariable.exchange(20); // Exchanges the value of the atomic variable with 20 and gets the old value back
Compare Exchange Operations
C++ also provides compare-exchange operations – complex but critical utilities for managing atomic variables. These operations compare the current value of an atomic variable with an expected value, and if they match, the operation replaces the current value with a new value.
Here’s how to do a compare-exchange operation:
std::atomic<int> atomicVariable(10); int expected = 10; bool exchanged = atomicVariable.compare_exchange_strong(expected, 20); // If atomicVariable was 10, it is now 20, and 'exchanged' is true
These are advanced operations that allow for detailed control in concurrent programming, facilitating concurrency control at an even finer granularity.
Wrapping It Up
Atomic operations in C++ offer the delightful fusion of simplicity and power, ensuring that your concurrent programs run smoothly and efficiently. By understanding the above operations, you can control concurrency at a granular level, craft high-performance programs, and elevate your skills to the next level.
At Zenva, we offer comprehensive, high-quality tutorials on a range of coding topics that help you evolve from a beginner to a pro. Stay tuned for more such informative pieces. Happy Coding!
More on Compare-Exchange: the Weak Variant
Beyond the compare_exchange_strong operation, C++ also provides a weak variant – compare_exchange_weak. This operation might fail even when the current value is the same as the expected value. However, this operation provides better performance on some platforms. Here’s an example:
std::atomic<int> atomicVariable(10); int expected = 10; bool exchanged = atomicVariable.compare_exchange_weak(expected, 20);
Memory Order in Atomic Operations
Memory order is an important concept in C++ atomic operations. By default, all atomic operations in C++ use a memory order known as memory_order_seq_cst that ensures a sequential consistency. However, you can specify other memory orders for optimization.
Here’s a pass at what implementing fetch_add with a relaxed memory order looks like:
std::atomic<int> atomicVar(10); int oldVal = atomicVar.fetch_add(5, std::memory_order_relaxed); // Adds 5 to atomicVar and returns old value
Memory Order: Acquire and Release
Besides relaxed memory order, two very useful memory orders are memory_order_acquire and memory_order_release. The acquire memory order ensures that no reads or writes in the current thread can be reordered before this load. Conversely, the release memory order guarantees that no reads or writes in the current thread can be reordered after this store.
Check out these examples:
std::atomic<int> atomicVar1(10), atomicVar2(20); atomicVar1.store(15, std::memory_order_relaxed); // Store with relased memory order int otherVal = atomicVar2.load(std::memory_order_acquire); // Load with acquire memory order
Memory Order: Acq-Rel and Consumed
Building on the acquire and release memory orders, C++ offers the memory_order_acq_rel and memory_order_consume orders. The former is a combination of acquire and release semantics, while the latter guarantees that if a load operation with this memory order returns a value produced by a store operation, any dependent loads will only see values written before that store.
std::atomic<int> atomicVar(10); atomicVar.store(15, std::memory_order_acq_rel); // Store with acq_rel memory order std::atomic<int>& atomicVarRef = atomicVar; int otherVal = atomicVarRef.load(std::memory_order_consume); // Load with consume memory order
There’s a lot more depth to atomic operations in C++. These operations provide a wide spectrum of control over the way your concurrent applications execute, allowing for deep control over multi-threading and concurrency.
At Zenva, we aim to provide engaging tutorials that break down complex concepts into digestible chunks. We hope that this tutorial has been helpful in your journey to mastering atomic operations in C++. When it comes to learning coding, we are your one-stop platform. For more top-notch content, stay tuned to our blog!
Your journey into C++ doesn’t have to end here with atomic operations. The world of C++ is vast with so many more exciting concepts waiting for you to uncover. We’re glad you’ve taken these essential steps, and we’re here to help you continue with a more profound exploration.
If you’re itching to dive even deeper, we encourage you to explore our C++ Programming Academy offered by Zenva Academy. It focuses on teaching the fundamental aspects of C++, from its basic syntax and program flow, through to object-oriented programming, and even popular game mechanics. The Academy offers flexible, beginner-friendly courses to help you grow from a novice to a proficient C++ programmer, all at your own pace. Plus, our courses aren’t just about listening! They include interactive lessons, hands-on coding practice, and rewarding certificates upon completion.
Our C++ courses offer a wide spectrum of content that will surely match your needs, regardless of whether you are just starting out or already comfortable with the basics. So, gear up and and continue learning with Zenva – a pathway to go from beginner to professional!
Conclusion
To conclude, we explored the power and simplicity of atomic operations in C++, which is key to orchestrating smooth, lag-free concurrent programs. But remember, the journey is only starting – the world of C++ has several other fascinating concepts and opportunities awaiting you.
Ready to dive deeper and explore more about C++? Our C++ Programming Academy has got you covered. Join us at Zenva, a trailblazer in coding education, to swim across the ocean of C++, from mastering the basics to unravelling the most advanced features. Take the leap, keep learning, keep growing, and keep coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
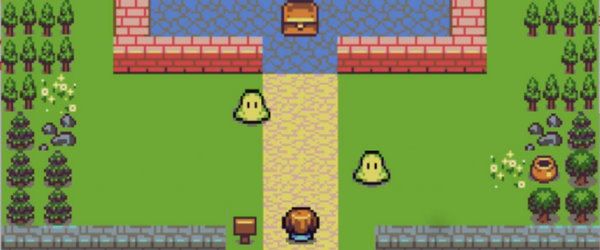
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.