Welcome to our tutorial on pseudocode, a fundamental skill that transcends specific programming languages. Here, we dive into the concept of pseudocode and explore how it can be an invaluable tool for programmers of all skill levels. Whether you’re taking your first steps in programming or have years of experience under your belt, mastering pseudocode will enhance your coding practices and make tackling programming problems a smooth process. So, let’s embark on this journey to demystify pseudocode and discover why it is a staple in a coder’s toolkit.
Table of contents
What is Pseudocode?
Pseudocode is a simplified, half-code, half-natural language that’s used by developers to plan and communicate algorithms before they’re implemented in a specific programming language. It allows you to focus on the core logic of the algorithm without getting bogged down by the syntax and particulars of any coding language. Pseudocode doesn’t run on a machine; instead, it serves as a blueprint for the actual code you’ll write later.
What is Pseudocode Used For?
Imagine you’re crafting the instructions for a new game or building a complex application. Pseudocode is your planning ally, helping you to:
– Sketch out the structure of your program or game logic.
– Communicate your ideas with others, including programmers who might use different languages.
– Identify potential issues or improvements in your logic early on.
– Simplify the process of coding by breaking down problems into smaller, more manageable steps.
Why Should I Learn It?
Understanding and writing pseudocode is an essential skill for any developer. It can vastly improve your problem-solving abilities and is a crucial part of algorithm design. Pseudocode enables you to:
– Clarify your thoughts and design algorithms without the distraction of coding syntax.
– Transition smoothly into coding, as you’ll have a clear outline to guide your work.
– Enhance collaboration with ease, regardless of the programming languages different team members use.
By learning to effectively use pseudocode, you’ll set a strong foundation that supports the rest of your development work, making it an undisputable skill to add to your programming arsenal.
Basic Structure in Pseudocode
Before jumping into actual pseudocode examples, it’s important to grasp that there is no one-size-fits-all format for writing it. Pseudocode is about clarity and simplicity, so as long as your logic is comprehensible and follows a consistent format, you’re on the right track. Let’s start with some building blocks:
Declaring Variables:
In pseudocode, you can declare variables simply by stating their intended purpose.
DECLARE age AS INTEGER DECLARE name AS STRING
Assigning Values:
Once variables are declared, you can assign values to them just as easily.
age = 25 name = "Alex"
Conditional Statements:
If-else statements are used to execute code based on a condition.
IF age > 18 THEN PRINT "You are an adult." ELSE PRINT "You are under age." END IF
Loops:
Loops are used for iterating over a set of instructions multiple times.
FOR EACH student IN classList PRINT student.name END FOR
More About Loops and Conditional Logic
To further understand loops and conditionals, let’s look at a variety of examples:
While Loop:
Sometimes you want to execute code as long as a condition is true. Here’s how you might represent that with pseudocode.
WHILE count < 10 DO PRINT count count = count + 1 END WHILE
Repeating Until a Condition is Met:
A slight variation of the while loop, the repeat-until structure, runs at least once and continues until a condition is met.
REPEAT userGuess = GET User Input IF userGuess != correctNumber THEN PRINT "Try again." END IF UNTIL userGuess = correctNumber
Complex Conditions:
Your logic can often depend on multiple conditions.
IF temperature > 30 THEN PRINT "It's a hot day." ELSE IF temperature > 20 AND temperature <= 30 THEN PRINT "It's a warm day." ELSE PRINT "It's quite cold today." END IF
Nested Loops:
These loops exist within another loop and are essential for handling multi-dimensional data.
FOR i = 1 TO 3 DO FOR j = 1 TO 3 DO PRINT "Position [", i, ",", j, "]" END FOR END FOR
Notice how each code snippet follows a straightforward logic without any specific syntax, which would be present in a programming language. The goal is to map out how the program should flow, creating a blueprint that can later be translated into an actual coded algorithm. Stay tuned for our next section where we’ll delve into functions and user interaction in pseudocode.In the previous section, we covered the basics of loops and conditionals in pseudocode. Now, let’s continue this journey and delve into functions and user interactions, two integral parts of most programming tasks.
Defining a Function:
Functions help organize your code into reusable blocks. Here’s how you might define a simple function in pseudocode.
FUNCTION CalculateSum(a, b) result = a + b RETURN result END FUNCTION
Calling a Function:
Once a function is defined, you can call it and use its return value:
sumResult = CalculateSum(5, 7) PRINT sumResult
User Defined Functions with Conditions:
You can create more complex functions that have conditional logic within them:
FUNCTION CheckAdult(age) IF age >= 18 THEN RETURN "Adult" ELSE RETURN "Minor" END IF END FUNCTION userStatus = CheckAdult(userAge) PRINT userStatus
User Interaction:
Interacting with the user is a common requirement, and here’s how it might look:
PRINT "Enter your name: " userName = GET User Input PRINT "Hello, " + userName + "!"
Error Handling:
It’s essential to plan for error handling in your program. Here’s a simple pseudocode example representing error checking:
FUNCTION DivideNumbers(x, y) IF y = 0 THEN PRINT "Error: Division by zero." RETURN END IF result = x / y RETURN result END FUNCTION
Looping with User Interaction:
Your users might interact with a loop, such as in a menu system:
REPEAT PRINT "1. Play game" PRINT "2. Highscores" PRINT "3. Exit" userChoice = GET User Input IF userChoice = 1 THEN CALL PlayGame() ELSE IF userChoice = 2 THEN CALL ShowHighscores() END IF UNTIL userChoice = 3
Implementing Arrays:
Arrays are used for storing a list of items. In pseudocode, you can manipulate them like this:
DECLARE scores AS ARRAY OF INTEGER SET scores = [75, 88, 92, 57, 83] FOR EACH score IN scores DO IF score > 85 THEN PRINT "High score!" ELSE PRINT "Keep trying!" END IF END FOR
These code examples display how various programming constructs can be expressed in an easy-to-understand pseudocode format. By writing pseudocode, you can work through complex ideas and ensure your logic is sound before getting tangled in the syntax details of actual code. This planning stage is critical in developing successful applications and games, something we at Zenva place a great deal of emphasis on in our tutorials and courses. Keep these examples as a reference as you tackle your next coding project, and remember, planning with pseudocode is a key element in building a solid coding foundation. Happy coding!Now that we have covered functions and simple data structures like arrays, let’s expand our pseudocode examples to include more complex data structures and operations. This will further illustrate the versatility of pseudocode in planning algorithms and understanding problem-solving strategies.
Working with Dictionaries:
Dictionaries or associative arrays are data structures that store data in key-value pairs. Here’s how you can depict a dictionary in pseudocode:
DECLARE userScores AS DICTIONARY SET userScores = {"Alex": 90, "Jordan": 85, "Sam": 78} FOR EACH key, value IN userScores DO PRINT key + " scored " + value END FOR
Handling Multidimensional Arrays:
To work with complex data, you may need a grid or table, which is where multidimensional arrays come in handy.
DECLARE grid AS ARRAY OF ARRAY OF INTEGER SET grid = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] FOR i = 0 TO LENGTH(grid) - 1 DO FOR j = 0 TO LENGTH(grid[i]) - 1 DO PRINT grid[i][j] END FOR END FOR
Linked Lists:
Linked lists are a basic data structure where each element contains a value and a reference to the next element.
DECLARE Node AS STRUCTURE value nextNode END STRUCTURE FUNCTION AddToLinkedList(headNode, newValue) current = headNode WHILE current.nextNode != NULL DO SET current = current.nextNode END WHILE SET current.nextNode = NEW Node current.nextNode.value = newValue current.nextNode.nextNode = NULL END FUNCTION
Stack Operations:
A stack allows you to add or remove items from the top of a pile, often described with ‘push’ and ‘pop’ operations.
DECLARE stack AS ARRAY FUNCTION Push(item) APPEND item TO stack END FUNCTION FUNCTION Pop() IF LENGTH(stack) > 0 THEN RETURN stack[LENGTH(stack) - 1] REMOVE LAST ITEM FROM stack ELSE PRINT "Error: Stack is empty." END IF END FUNCTION
Implementing a Queue:
A queue ensures processing in a first-in, first-out (FIFO) manner, where elements are added to the end and removed from the front.
DECLARE queue AS ARRAY FUNCTION Enqueue(item) APPEND item TO queue END FUNCTION FUNCTION Dequeue() IF LENGTH(queue) > 0 THEN RETURN queue[0] REMOVE FIRST ITEM FROM queue ELSE PRINT "Error: Queue is empty." END IF END FUNCTION
Searching Algorithms:
There are times when you need to search through data. Here’s how a linear search could be depicted:
FUNCTION LinearSearch(list, itemToFind) FOR EACH item IN list DO IF item = itemToFind THEN RETURN "Item found." END IF END FOR RETURN "Item not found." END FUNCTION
Sorting Algorithms:
Sorting is common in many applications. Here’s how a simple bubble sort algorithm might be represented:
FUNCTION BubbleSort(array) FOR i = 0 TO LENGTH(array) - 1 DO FOR j = 0 TO LENGTH(array) - i - 1 DO IF array[j] > array[j + 1] THEN DECLARE temp AS INTEGER temp = array[j] array[j] = array[j + 1] array[j + 1] = temp END IF END FOR END FOR RETURN array END FUNCTION
Remember, the goal of pseudocode isn’t to create runnable code but to lay out the logic that drives it. These examples illustrate the point at which we go from ideas to a structured plan that can be translated into any programming language. It’s a crucial skill that we, at Zenva, advocate for and incorporate into our educational content, guiding you from concept to code. Keep this approach in your toolkit as you continue to develop and refine your programming proficiency.
Where to Go Next with Your Coding Journey
With a solid understanding of pseudocode under your belt, you’re well-equipped to take the next steps in your programming journey. Pseudocode is like the blueprint for your coding projects, and it’s time to start building. We encourage you to explore real-world coding with our Python Mini-Degree, where you can bring your blueprints to life. While this program doesn’t focus exclusively on pseudocode, it’s packed with invaluable knowledge that’s perfect for transforming your conceptual understanding into tangible programming skills.
Python is lauded for its simplicity yet powerful capabilities across various domains, from game development to data science. By delving into our Python courses, you’ll not only learn the syntax and semantics of one of the most in-demand programming languages, but you’ll also engage in hands-on projects that will bolster your experience and enhance your portfolio.
If you’re looking for an even broader array of topics, our assortment of Programming courses is the ideal destination. Here, you’ll find over 250 fully-supported courses that cater to beginners and seasoned programmers alike. At Zenva, you can go from beginner to professional at your own pace, building a robust skill set that’s coveted in today’s job market. Join our community, and continue shaping your future in tech with Zenva.
Conclusion
Mastering pseudocode is akin to sharpening the essential tools in your programming toolkit. It lays the groundwork for articulate, effective coding, and paves the way for seamless transition into any programming language. As you progress from planning to coding with our Python Mini-Degree, you’ll find that the principles of pseudocode are applicable every step of the way, allowing for clearer logic, better problem solving, and smoother collaboration.
At Zenva, our mission is to provide you with the most comprehensive and up-to-date learning experiences, equipping you for the demands of the tech industry. We invite you to continue your coding journey with us, connect with a vibrant community of learners, and transform the theoretical knowledge of pseudocode into real-world applications. Embrace the joy of creation as you turn your ideas into reality, one line of code at a time.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
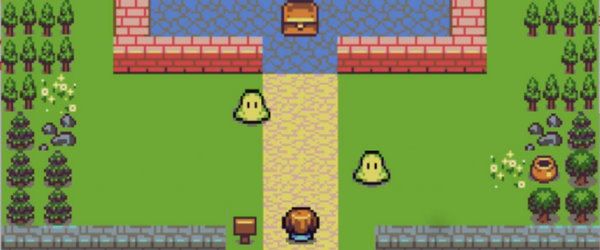
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.