Welcome to the world of debugging, an essential yet sometimes elusive skill set that is crucial for programmers of all levels. Whether you’re just starting out or you’ve coded a few applications, understanding debugging is key to improving your code and becoming a more effective developer. In this tutorial, you’ll learn the core concepts of debugging, the reasons behind its importance, and how mastering these techniques can make you a more competent and confident coder.
Table of contents
What Is Debugging?
At its most basic, debugging is the process of finding and resolving defects or problems within a program. These issues, commonly referred to as bugs, can range from syntax errors and typos to more complex logical or runtime errors. Debugging involves not only identifying these errors but also understanding why they occurred and how to fix them in a way that prevents future issues.
What Is Debugging For?
Think of debugging as the detective work in programming. When a piece of software doesn’t run as expected, debugging is the methodology that helps you find the root cause and devise a solution. Debugging ensures that programs run smoothly, meet their design specifications, and provide a good experience for the user.
Why Should You Learn Debugging?
Effective debugging skills can save hours of frustration and work. Instead of aimlessly tweaking code or making arbitrary changes in hopes of a quick fix, a structured approach to debugging provides a clear path to resolving issues. Learning how to debug efficiently is critical for:
- Enhancing the quality and reliability of your software
- Increasing your productivity as a programmer
- Fostering a deeper understanding of how your code works
- Building confidence in tackling complex coding challenges
Moreover, employers highly value developers with strong debugging skills, as it leads to less downtime and more robust applications. Whether you’re working on personal projects or aiming for a career in programming, becoming proficient in debugging is an invaluable asset.
Identifying Syntax Errors
Syntax errors are the first type of bugs new developers often encounter. They occur when the code doesn’t adhere to the language’s rules or grammar. Luckily, these are usually caught quickly by the language’s compiler or interpreter.
// Example of a syntax error in JavaScript consoel.log('Hello, world!');
In the above example, ‘console’ is misspelled as ‘consoel’. This will lead to an error when you try to run the script. Debugging syntax errors requires careful reading of error messages and a review of your code to correct typos and syntax issues.
Resolving Logical Errors
Logical errors occur when your program runs without crashing, but it produces the wrong result. These errors are more devious because the code is syntactically correct. Common logical errors include incorrect assumptions or mistakes in algorithms used to solve problems.
// Logical error example in Python def calculate_average(nums): sum = 0 for num in nums: sum += num return sum # Should return the sum divided by the length of the list # Corrected version def calculate_average(nums): sum = 0 for num in nums: sum += num return sum / len(nums)
In the example, the first version of the function calculates the sum but forgets to divide by the number of items to get the average. Debugging requires logic and reevaluation of the code to ensure it meets the required specifications.
Finding and Fixing Runtime Errors
Runtime errors are ones that occur while the program is running. These are often harder to debug since they might only occur under certain conditions or with specific inputs.
// Example of a runtime error in JavaScript function divide(a, b) { return a / b; } console.log(divide(10, 0)); // Error: division by zero
In this example, the function divide is designed to work with non-zero denominators, but it does not handle the case when ‘b’ is zero. To fix this error, you need to add a check to ensure ‘b’ is not zero before performing the division.
// Corrected version with error handling function divide(a, b) { if (b === 0) { throw new Error('Cannot divide by zero'); } return a / b; }
The updated function now checks for division by zero and throws an error if the condition is met, preventing a catastrophic runtime error.
Dealing with Compilation Errors
Compilation errors are specific to compiled languages like C++ or Java and occur when the source code cannot be converted into executable code. Understanding and fixing these errors requires knowing the compiler’s feedback.
// Compilation error in C++ int main() { int number = "42"; // Error: assignment of incompatible data types return 0; }
The error above shows an attempt to assign a string literal to an integer variable. The compiler rejects this because it’s an incompatible type assignment.
// Corrected version with proper type assignment int main() { int number = 42; return 0; }
After correcting the data type, the code compiles successfully. Fixing compilation errors often involves understanding type systems and memory management.
With these examples, we have seen common categories of errors and begun to understand how debugging can address these issues. Remember, a good debugging strategy is methodical and systematic, significantly reducing the time and frustration associated with fixing code.
Mastering debugging involves encountering a variety of errors and learning from them. Let’s explore some more code examples that demonstrate common errors you’ll likely need to debug in your coding journey.
Understanding Scope Errors
A scope error happens when a variable is used outside of the context it was defined in. Different programming languages have different scope rules, but it’s generally tied to where variables and functions are declared.
// Scope error example in JavaScript function greet() { let greeting = "Hello, world!"; } console.log(greeting); // Error: greeting is not defined
In this JavaScript example, the variable ‘greeting’ is defined within the scope of the ‘greet’ function. Trying to access it outside of that function results in an error. The fix involves understanding where and how you’re allowed to use your variables.
// Corrected version function greet() { let greeting = "Hello, world!"; console.log(greeting); } greet(); // Correctly logs "Hello, world!"
The corrected version ensures the variable is used within its defined scope.
Addressing Type Errors
Type errors can often occur in languages with dynamic typing, like JavaScript, when operations are performed on incompatible types.
// Type error in JavaScript let num = 5; let result = num.toUpperCase(); // Error: num.toUpperCase is not a function
Here, the toUpperCase() method is called on a number type, which is nonsensical as this method is meant for strings. To avoid such errors, ensure the operations are compatible with the type of data you’re working with.
// Corrected version let num = 5; let result = num.toString().toUpperCase(); // Converts number to string and then to uppercase
The corrected code first converts the number to a string before calling the toUpperCase() method.
Dealing with Concurrency Issues
In multithreaded or asynchronous programming environments, concurrency issues such as race conditions can be particularly tricky to debug because they may only occur intermittently.
// Hypothetical race condition in an asynchronous JavaScript function let count = 0; function increment() { count += 1; } setTimeout(increment, 100); // Intends to increment count after 100 ms setTimeout(increment, 100); // Intends to increment count after another 100 ms // There's a chance both operations will read the original count value before either increments it
To resolve these race conditions, you need to ensure the critical section is accessed in a managed way, either by using locks, semaphores, or other concurrency control methods.
Null Reference Errors
Null reference errors occur when a variable that is expected to refer to an object is actually null or undefined. This often results in the infamous “null reference exception.”
// Null reference example in Java public class TestNull { public static void main(String[] args) { String str = null; System.out.println(str.length()); // Error: NullPointerException } }
This Java code is trying to call a method on a null object, which causes a NullPointerException. To fix this, you must ensure that the variable is properly initialized before you use it.
// Corrected version public class TestNull { public static void main(String[] args) { String str = "Zenva"; System.out.println(str.length()); // Outputs 5 } }
After initializing the string variable ‘str’, the error is resolved and the length of the string is correctly calculated.
Through these examples, we illustrated issues such as scope, type, concurrency, and null reference errors. It is evident that a methodical approach to debugging can help diagnose and fix these problems efficiently. Remember, the more you practice, the better you will become at quickly identifying and resolving bugs in your code. Embrace the challenge—happy debugging!
As we dive further into the abyss of potential errors that can plague our code, let’s venture into some more complex territories that tend to confuse even seasoned coding veterans. We’ll cover a range of issues that include race conditions, memory leaks, and more. Grab your detective hat, it’s time to debug!
Race conditions, often found in concurrent programming, can cause erratic behavior in applications, as operations may not complete in the sequence you expect. Here’s a simplified version of what that might look like:
// Race condition in JavaScript let balance = 100; function withdraw(amount) { if (balance >= amount) { console.log(`Withdrawing ${amount}`); balance -= amount; console.log(`New balance: ${balance}`); } else { console.log('Insufficient funds'); } } withdraw(80); // Expected balance: 20 setTimeout(() => withdraw(30), 0); // Expected balance: -10, but actually, Insufficient funds
In this case, the asynchronous nature of JavaScript’s setTimeout can introduce a race condition where the order of execution isn’t as you might predict, leading to inconsistent behavior.
Memory leaks are another common problem. They occur when allocated memory is not properly cleaned up after use, which can lead to decreased application performance or even crashes:
// Memory leak example in C++ #include int main() { int* ptr = new int(10); // Some complex logic that forgets to delete the allocated memory // Leads to a memory leak as memory is not released back to the system return 0; }
To mitigate such memory leaks, we must ensure that every new allocation has a corresponding delete operation:
// Fixed memory leak example #include int main() { int* ptr = new int(10); // Correctly paired delete operation delete ptr; return 0; }
Always remember to manage dynamic memory properly to prevent these kinds of leakage issues.
Exception handling is another critical area that many developers struggle with. Proper exception handling ensures that your program can gracefully deal with unexpected events. Here is an example without proper exception handling:
// Example without exception handling in Python def divide(x, y): return x / y print(divide(10, 2)) # Works fine print(divide(10, 0)) # Causes ZeroDivisionError
With proper exception handling, we can catch potential errors and react accordingly:
// Example with exception handling in Python def divide(x, y): try: return x / y except ZeroDivisionError: print("You can't divide by zero!") print(divide(10, 2)) print(divide(10, 0))
The key takeaway is to expect the unexpected and plan for ways in which your code could fail.
Handling incorrect data types is another challenge that requires careful debugging:
// Incorrect data type in Python def increment(number): try: return number + 1 except TypeError: print('The provided input is not a number') increment('Zenva') // Outputs: The provided input is not a number
Python’s dynamic typing requires you to be vigilant about the types of data your functions are processing. Using try…except blocks, like above, can save your code from unexpected crashes.
An unhandled edge case occurs when a specific condition is not taken into account when writing a program. These can cause the software to break in surprising ways.
// Example of unhandled edge case in JavaScript function joinNames(names) { return names.join(' & '); } console.log(joinNames(['Anna', 'Bob'])); // Works: "Anna & Bob" console.log(joinNames([])); // Edge case: returns empty string instead of an error or a default message
We could solve this unhandled edge case with a conditional check:
// Fixed the unhandled edge case function joinNames(names) { if(names.length === 0) { return 'No names provided'; } return names.join(' & '); } console.log(joinNames(['Anna', 'Bob'])); // Works: "Anna & Bob" console.log(joinNames([])); // "No names provided"
Thorough testing for all potential inputs and cases can help identify and resolve such edge cases.
As you dissect these examples, it’s clear that debugging is an art that requires meticulous attention to detail and a strong understanding of your programming tools and environment. But fear not, as the more bugs you tackle, the sharper your debugging skills will become. Embrace the practice, continue learning from each mistake, and remember, every bug you squash makes you a stronger developer. Happy coding!
Where to Go Next in Your Coding Journey
Armed with debugging knowledge, you are now better equipped to tackle programming challenges with confidence. But don’t stop here! Continue your coding journey with us at Zenva, where there’s always more to learn and explore. For those passionate about mastering Python, we encourage you to take a look at our Python Mini-Degree. This comprehensive program will deepen your understanding of Python and expand your skill set into realms such as game development, app creation, and even AI.
Flexibility is key in learning, and the Python Mini-Degree fits into any schedule, allowing you to grow at your own pace and build a portfolio of impressive projects. Whether you’re a beginner or looking to hone existing skills, our courses are crafted to transition you from basic know-how to advanced, industry-relevant applications. Moreover, for a broader look at the programming landscape, our array of programming courses cover languages and technologies that are shaping today’s tech world.
Remember, the journey of a thousand lines of code begins with a single debug. Embrace continuous learning with Zenva, and advance your career with the latest in coding, game development, and AI!
Conclusion
In the grand tapestry of programming, debugging is the thread that corrects and perfects, transforming tangled code into a seamless creation. Whether it’s squashing a simple syntax error or unraveling a complex race condition, the skills you develop will echo through every line of code you write. And remember, the journey doesn’t end here; it evolves with each new skill you master and every project you complete.
We at Zenva are committed to supporting you every step of the way. With our Python Mini-Degree, and a plethora of meticulously crafted courses, your path from novice to seasoned developer is clear and attainable. Keep experimenting, keep solving problems, and continue to grow with us. Your potential is limitless, and we’re here to help you unlock it. Embrace the craft, commit to learning, and watch as you transform the world, one debug at a time.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
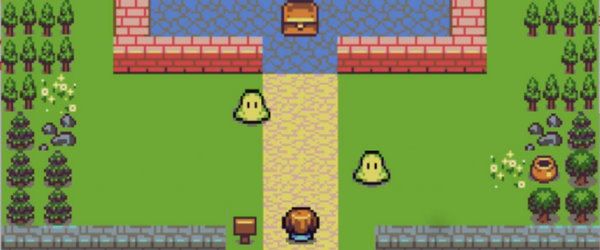
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.