Understanding the pillars of programming is crucial, and classes are one of those foundational structures that we encounter in various programming paradigms. Whether you’re just starting on this exciting coding journey or are looking to solidify your knowledge, grasping the concept of classes is essential. It opens doors to a world of more organized and efficient code management and lays the groundwork for advanced programming techniques.
What Are Classes in Programming?
Table of contents
What is a Class?
A class in programming is a blueprint, a template from which objects are created. It encapsulates data for the object and methods to manipulate that data. Just as architects use blueprints to build a house, programmers use classes to create instances of objects that will behave and interact according to the class’s design.
What Are Classes Used For?
Classes serve as a foundational construct in object-oriented programming (OOP). They help programmers organize and group data and behaviors, promoting code reusability and scalability. With classes, you can create complex data models that reflect real-world entities and their interactions, facilitating a more intuitive development process.
Why Should I Learn About Classes?
Learning about classes is pivotal for anyone interested in software development. Classes allow you to think about the code in terms of real-life objects, thus making the transition from concept to code smoother and more natural. They are used in many high-level programming languages and understanding them can provide a competitive edge in a variety of coding disciplines.
Defining a Class in Python
Let’s begin with defining a simple class in Python. We define a class using the class keyword followed by the class name and a colon. Below is how you can define a basic class named Animal.
class Animal: pass
This class currently doesn’t do much because we’ve just used the pass statement which is a placeholder in Python. Let’s add some properties to our class.
class Animal: def __init__(self, name, species): self.name = name self.species = species
In this example, the __init__ method is what we call a constructor in Python. It’s called automatically when you create a new instance of the class. The self parameter refers to the instance itself.
Creating an Instance of a Class
With our class defined, we can now create an instance of the Animal class. To do this, you simply call the class as if it were a function, passing the necessary arguments defined in the __init__ method.
my_pet = Animal("Fido", "Dog") print(my_pet.name) # Output: Fido print(my_pet.species) # Output: Dog
Here, my_pet is an instance of the Animal class, with name and species properties set to ‘Fido’ and ‘Dog’ respectively.
Adding Methods to a Class
Classes are not just about storing data – they can also contain functions, known as methods, which add behavior to the instances.
class Animal: def __init__(self, name, species): self.name = name self.species = species def make_sound(self, sound): print(f"{self.name} says {sound}") my_pet = Animal("Fido", "Dog") my_pet.make_sound("woof woof") # Output: Fido says woof woof
Here, the make_sound method allows the my_pet object to perform an action that outputs a string representing the sound the animal makes.
Understanding Class and Instance Variables
In Python, there’s a distinction between class variables (shared across all instances) and instance variables (unique to each instance). Let’s see an example to clarify the difference.
class Animal: kingdom = 'Animalia' # This is a class variable def __init__(self, name, species): self.name = name # These are instance variables self.species = species my_pet = Animal("Fido", "Dog") print(my_pet.kingdom) # Output: Animalia print(Animal.kingdom) # Output: Animalia
The kingdom variable is shared across all instances of the class, whereas name and species are specific to each instance.
Private Variables and Methods
In some cases, you may want to hide certain parts of your class from the outside world. In Python, we can’t truly make attributes and methods private, but we can indicate that they are meant to be treated as private by prefixing them with an underscore.
class Animal: def __init__(self, name, species): self.name = name self._secret = "I can talk!" def _private_method(self): print("This is a private method!") def public_method(self): print("This is a public method that can call a private method.") self._private_method() my_pet = Animal("Fido", "Dog") # This will work but it is not recommended to use private attributes or methods. my_pet._private_method() # Output: This is a private method! my_pet.public_method() # Output: This is a public method that can call a private method.
The use of a single underscore before the method _private_method and variable _secret is a convention to indicate that they should not be accessed directly, although Python does not enforce this rule strictly.
Mastering these class basics sets the foundation for diving deeper into object-oriented programming and understanding more complex concepts such as inheritance, polymorphism, and encapsulation. Stick with it, and you’ll find that classes are integral to writing clean, manageable, and scalable code in various programming disciplines.
Continuing with our exploration of classes, we’ll delve into more advanced topics, such as class inheritance, polymorphism through methods, class methods, static methods, and property decorators. These concepts are critical to leveraging the full power of object-oriented programming in Python.
Inheritance in Python
Inheritance is a way to form new classes using classes that have already been defined. The newly formed classes are called derived classes, and the classes that we derive from are called base classes. Derived classes can override or extend the functionalities of base classes.
class Mammal(Animal): def __init__(self, name, species, warm_blooded=True): super().__init__(name, species) self.warm_blooded = warm_blooded my_mammal = Mammal("Whiskers", "Cat") print(my_mammal.warm_blooded) # Output: True
Here, the Mammal class inherits from Animal, and we use the super() function to call the __init__ method of the parent class.
Method Overriding
Derived classes may override methods of their base classes. This is useful for altering or extending the behavior of the base class method.
class Mammal(Animal): def make_sound(self, sound): print(f"The mammal named {self.name} says {sound}") my_mammal = Mammal("Whiskers", "Cat") my_mammal.make_sound("meow") # Output: The mammal named Whiskers says meow
In this example, the make_sound method is overridden in the Mammal class, giving it a slightly different behavior from the one in the Animal class.
Class Methods and Static Methods
Aside from instance methods, which operate on an instance of a class, we also have class methods and static methods.
A class method takes cls as the first parameter and works with the class itself, not instances, while a static method doesn’t need a reference to either class or instance and can be called on the class itself.
class Animal: @classmethod def kingdom_info(cls): return f"All animals belong to kingdom: {cls.kingdom}" @staticmethod def static_method(): print("This is a static method. It doesn't take cls or self as parameters.") print(Animal.kingdom_info()) # Output: All animals belong to kingdom: Animalia Animal.static_method() # Output: This is a static method. It doesn't take cls or self as parameters.
Property Decorators – Getters and Setters
Property decorators let us define methods that can be accessed like attributes. This allows us to add logic to attribute access without changing the class interface.
class Animal: def __init__(self, name, species): self._name = name self._species = species @property def name(self): return self._name.title() @name.setter def name(self, value): if isinstance(value, str): self._name = value else: raise ValueError("Name must be a string") my_animal = Animal("fido", "dog") print(my_animal.name) # Output: Fido my_animal.name = "Spot" print(my_animal.name) # Output: Spot
In the example above, the name method is now a property with a getter that formats the name, and a setter that validates the input.
Understanding these more sophisticated features of classes helps you to structure your Python programs much more effectively, ultimately leading to cleaner, more robust, and maintainable code.
Expanding our discussion of classes in Python, we’ll explore multiple inheritance and delve into the use of abstract base classes. We’ll also see how context managers can be created using classes, among other advanced features.
Multiple inheritance allows a class to inherit features from more than one parent class, offering a high level of flexibility and enabling the creation of complex hierarchies.
class Aquatic: def __init__(self, name): self.name = name def swim(self): return f"{self.name} is swimming." class Terrestrial: def __init__(self, name): self.name = name def walk(self): return f"{self.name} is walking." class Amphibian(Aquatic, Terrestrial): def __init__(self, name): super().__init__(name) frog = Amphibian("Froggy") print(frog.swim()) # Output: Froggy is swimming. print(frog.walk()) # Output: Froggy is walking.
In this example, the Amphibian class inherits from both Aquatic and Terrestrial, allowing the frog instance to use methods from both parent classes.
Abstract Base Classes (ABCs) in Python are used to create a class that cannot be instantiated, but can be subclassed. This is useful for creating a base class that defines a common API for a set of subclasses.
from abc import ABC, abstractmethod class Animal(ABC): @abstractmethod def make_sound(self): pass class Dog(Animal): def make_sound(self): return "Woof!" # Cannot instantiate an abstract class # animal = Animal() # This will raise an error dog = Dog() print(dog.make_sound()) # Output: Woof!
Here, Animal is an abstract base class with an abstract method make_sound. The Dog subclass provides its own implementation of the method.
Classes can also provide a convenient way to create context managers in Python, which is useful for managing resources with the help of the with statement.
class ManagedFile: def __init__(self, name): self.name = name def __enter__(self): self.file = open(self.name, 'w') return self.file def __exit__(self, exc_type, exc_val, exc_tb): if self.file: self.file.close() with ManagedFile('hello.txt') as f: f.write('Hello, world!')
The ManagedFile class implements the context management protocol via the __enter__ and __exit__ methods, ensuring that the file is properly closed after its block is executed.
Python supports operator overloading, which means you can define how operators like +, –, * work with your class instances.
class Vector2D: def __init__(self, x, y): self.x = x self.y = y def __add__(self, other): return Vector2D(self.x + other.x, self.y + other.y) def __str__(self): return f"({self.x}, {self.y})" vec1 = Vector2D(5, 7) vec2 = Vector2D(3, 9) vec3 = vec1 + vec2 print(vec3) # Output: (8, 16)
In this example, the Vector2D class defines the __add__ method, which allows us to add two Vector2D instances with the + operator.
Through the use of these advanced features, classes in Python can be tailored very precisely to your programming needs, providing you with powerful tools to write elegant and efficient object-oriented code.
Continue Your Python Journey with Zenva
You’ve taken some pivotal first steps in understanding classes and object-oriented programming in Python. But the path to mastery is a continuous journey. At Zenva, we know that learning doesn’t stop after your initial steps. That’s why we encourage you to keep building on what you’ve learned with our Python Mini-Degree, a comprehensive collection of courses tailored to elevate your skills from beginner levels all the way to creating your own real-world applications.
Our Python Mini-Degree covers a wide range of topics, equipping you to tap into various industries where Python is a key player. The modular course structure gives you the freedom to learn at your own pace and delve deeper into areas of interest, such as game development, app development, and even cutting-edge fields like data science and machine learning.
If you’re looking to broaden your coding horizons even further, our extensive catalog of Programming courses at Zenva Academy is sure to have something for you. From front-end to back-end, we provide a variety of courses that cater to all levels of experience, empowering you to mold your expertise precisely to your career goals and passions. Join us, and let’s turn your coding aspirations into reality.
Conclusion
As you’ve seen through these insights into Python’s classes and advanced features, the world of programming is vast and full of potential. Mastering Python classes is like unlocking a new level in a game—the challenges grow, but so does your ability to navigate and create within the digital landscape. We at Zenva are committed to supporting you every step of the way as you continue to develop your skills and carve out your niche in the programming world.
Don’t let your journey end here. With every concept mastered, you’re one step closer to being the developer you aspire to be. Explore our Python Mini-Degree and build on your knowledge. The future is coded by people like you—creative, persistent, and ready to make an impact. Together, let’s code that future into existence!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
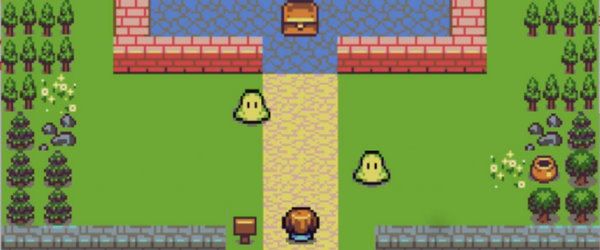
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.