Welcome to our journey into the world of datatypes, the fundamental building blocks of any programming experience! As a coder, regardless of your level of expertise, understanding datatypes is as crucial as knowing the alphabets for reading. It’s the starting point that will lead you to comprehend how data is stored, manipulated, and interacted within your code. Whether you’re just starting out or you’ve been at it for a while, grasping datatypes will sharpen your coding skills and expand what you can achieve with them. So, let’s dive into the fascinating universe of datatypes, where every variable has a story, and every piece of data, a place to belong.
What are Datatypes?
Table of contents
Understanding Datatypes
Datatypes are essentially labels that we give to different kinds of data to inform the computer about how it should be used. Just like you wouldn’t pour orange juice into your car’s gas tank, a computer needs to know whether it’s dealing with numbers, text, or something else entirely.
The Purpose of Datatypes
Datatypes serve several purposes in programming languages. They help in:
- Allocating memory: Different datatypes need different amounts of memory.
- Defining operations: Certain operations are only applicable to certain datatypes.
- Forming the basis of type checking: The programming language can thus prevent errors by ensuring the correct usage of data.
Why Should I Learn About Datatypes?
Knowing your datatypes is akin to having the right tools for a job. It is not just about avoiding errors, but also about:
- Efficiency: Using the right datatype means your program can run faster and more efficiently.
- Clarity: Clear, understandable code is crucial for collaboration and maintenance.
- Capability: The more you know about datatypes, the more complex and interesting problems you can solve with your code.
Embracing the variety of datatypes will unlock new potentials in your programming repertoire, making it an essential subject to master for any enthusiastic developer.
Common Datatypes in Programming
In most programming languages, there are a handful of fundamental datatypes that you’ll encounter regularly. These include integers, floating-point numbers, characters, and strings. Each language might have its own peculiarities, but the core idea remains consistent. Let’s take a closer look with some examples to illustrate these common datatypes.
Integers
An integer is a whole number without any fractional part. They are commonly used for counting, indexing, and operations that require precision without decimals.
int age = 30; int numberOfApples = 5; int distanceInMeters = 800;
Floating-Point Numbers (Floats)
Floating-point numbers, or floats, are numbers with decimal points. These are used when you need to perform calculations that require fractional parts.
float temperature = 36.5; float averageScore = 88.6; float price = 199.99;
Characters
Characters are individual letters, numbers, punctuation, etc. They are usually denoted by single quotes in most programming languages.
char initial = 'J'; char punctuation = '!'; char grade = 'A';
Strings
Strings are sequences of characters representing text. They are typically enclosed in double quotes. Strings are used whenever you need to work with textual data.
String name = "Jane Doe"; String greeting = "Hello, World!"; String favoriteQuote = "To be or not to be.";
Understanding and using these basic datatypes effectively is the first step towards writing robust and efficient code. As you become more comfortable with these, you’ll be able to utilize them in more complex ways to build intricate programs.
Booleans
A Boolean is a datatype that can only take two values: true or false. This is often used in control flow statements and conditions.
boolean isRaining = false; boolean hasPassedTest = true; boolean isLeapYear = false;
When working with Booleans, you’ll often pair them with if-else statements to direct the flow of your program based on certain conditions.
Using Datatypes in Variables
Variables are named storage locations in memory, each of which holds a value of a specific datatype. Here are some examples of how to define variables with different datatypes:
// An integer variable int score = 100; // A float variable float interestRate = 4.5f; // A character variable char grade = 'A'; // A string variable String message = "Hello, Zenva learners!"; // A boolean variable boolean isSubscribed = true;
Remember, the way you define variables might differ slightly based on the programming language you’re using. However, the concept of datatypes remains a constant thread throughout. Master these, and you’ll be able to handle more nuanced scenarios in your coding projects.
In the next part of our tutorial, we will explore more examples and delve into how we can manipulate these datatypes to create functional and interactive programs.
Manipulating Data Types
Now that we’ve discussed what data types are and how to define variables with them, let’s explore how you can manipulate these data types in different scenarios. This will include performing operations, making decisions with them, and combining them to form more complex expressions.
Arithmetic Operations with Numbers
Numeric data types like integers and floats support arithmetic operations that let you perform basic math. These include addition (+), subtraction (-), multiplication (*), and division (/).
// Adding two integers int a = 10; int b = 20; int sum = a + b; // sum is 30 // Subtracting two floats float x = 5.75f; float y = 2.30f; float difference = x - y; // difference is 3.45 // Multiplying an integer and a float int quantity = 10; float pricePerUnit = 3.50f; float totalCost = quantity * pricePerUnit; // totalCost is 35.0
Understanding arithmetic operations is essential for calculations and forming algorithms that solve problems or answer questions with numerical data.
Concatenating Strings
String concatenation is the process of appending one string to the end of another. This is typically achieved using the plus (+) operator.
// Concatenating strings String firstName = "John"; String lastName = "Doe"; String fullName = firstName + " " + lastName; // fullName is "John Doe" String part1 = "Game"; String part2 = "Dev"; String combined = part1 + part2; // combined is "GameDev"
Being able to concatenate strings is fundamental for creating messages, constructing dynamic outputs, and handling textual data in a readable format.
Working with Booleans and Conditions
Booleans are central to decision-making in programming. By combining Booleans with conditional statements, such as ‘if’, ‘else if’, and ‘else’, we can influence the flow of our programs based on certain conditions being true or false.
// Using Boolean values in a conditional boolean isSunny = true; if (isSunny) { System.out.println("Wear sunglasses!"); } else { System.out.println("Bring an umbrella!"); }
Conditionals are the building blocks of logic in programming, critical for creating dynamic, responsive, and intelligent behavior in software.
Type Casting
Sometimes, you might need to convert a value from one data type to another; this is known as type casting. Implicit casting happens automatically when it’s safe to do so, such as converting an int to a float. Explicit casting is required when there’s a risk of losing information, like converting a float to an int.
// Implicit casting from int to float int highScore = 100; float convertedScore = highScore; // No data is lost; casting is implicit // Explicit casting from float to int float gradeAverage = 95.75f; int roundedGrade = (int) gradeAverage; // Decimal is truncated; casting is explicit
Casting is a powerful tool that you’ll use to convert data types in order to perform operations and functions that require specific types of inputs.
As we progress in our learning journey with Zenva, understanding and using datatypes will become second nature. The more you practice, the more intuitive your decisions will become about which datatype to use and when. Combine this knowledge with other programming concepts and you’ll be writing sophisticated and efficient code in no time. Keep honing your skills, and soon enough, datatypes will be a part of your esteemed toolkit as a developer. Happy coding!
With the foundational understanding of datatypes in place, it’s time to dive deeper into practical examples. By exploring additional operations and scenarios, we’ll demonstrate how data types can be manipulated to perform a variety of functions. We’ll see how they interact with each other and the importance of considering data types when writing conditional logic and loops.
Let’s explore some coding examples that highlight the versatility and importance of understanding datatypes:
Complex Operations with Numeric Datatypes
More complex calculations often involve both integers and floats, and you need to be careful about the result’s data type. Here’s an example where you might run into issues:
// Division of integers int totalPixels = 1080; int numberOfLines = 12; int pixelsPerLine = totalPixels / numberOfLines; // Results in 90, not 90.0 // To get a float result, cast at least one operand to float float precisePixelsPerLine = (float)totalPixels / numberOfLines; // Now results in 90.0
Understanding how different numeric data types interact in operations helps prevent unintentional loss of precision.
Character Operations
Characters aren’t just for text; they also have numerical representations. This allows for operations such as determining the character code or performing arithmetic to get related characters:
// Getting the numerical (ASCII) value of a character char character = 'A'; int asciiCode = (int)character; // asciiCode is 65 // Calculating the next character in the alphabet char nextCharacter = (char)(character + 1); // nextCharacter is 'B'
These character operations are fundamental in encryption, data processing, and other fields where the data’s textual representation is significant.
Using Strings in Conditional Logic
Strings are often used in conditional logic to make decisions based on text content. Here’s an example with an ‘equals’ method, which is safer and more accurate than using the ‘==’ operator with strings:
// Correct way to compare strings String correctPassword = "secure123"; String userInput = "secure123"; if (correctPassword.equals(userInput)) { System.out.println("Access granted."); } else { System.out.println("Access denied."); }
Comparing strings correctly is crucial in applications ranging from user authentication systems to data validation processes.
Complex Boolean Conditions
In programming, it’s common to come across complex conditions that involve combining Boolean expressions. The logical operators ‘&&’ (AND), ‘||’ (OR), and ‘!’ (NOT) are used to build these expressions:
// Logical operators with Boolean values boolean hasHighScore = true; boolean hasGoodCredit = false; if (hasHighScore && hasGoodCredit) { System.out.println("Loan approved."); } else { System.out.println("Loan denied."); } // Using NOT to invert a Boolean boolean isRainPredicted = true; if (!isRainPredicted) { System.out.println("No need to carry an umbrella."); } else { System.out.println("Better take your umbrella just in case."); }
Understanding these logical operators is vital for controlling the flow of your programs and creating complex decision-making structures.
Combining DataType Operations
It often happens that different data types are used together within calculations and logic. Here’s how you might combine them:
// Combining an integer and a string in a message int cookiesLeft = 3; String message = "There are " + cookiesLeft + " cookies left."; System.out.println(message); // Using Boolean values inside a String message boolean isPremiumUser = true; String accountType = "User account type: " + (isPremiumUser ? "Premium" : "Standard"); System.out.println(accountType);
These examples exhibit the practical integration and conversion of datatypes, which is instrumental in creating comprehensive and interactive applications.
Grasping the intricacies of datatypes is only the beginning of your journey with us at Zenva. As you practice with real-world coding problems, the importance and power of correctly using datatypes will become increasingly apparent. Always keep in mind that the understanding and proper use of datatypes are foundational to any successful programming endeavor, which is why we emphasize their study. Continue sharpening your datatype skills, and you’ll be well on your way to mastering the art of coding!
Continue Your Learning Journey with Zenva
Embarking on the path of mastering programming can be thrilling and, at times, challenging. However, there’s no better way to cement your knowledge and skillset than to keep learning and applying what you’ve learned. Unraveling the intricacies of datatypes is just the beginning. To dive deeper into the world of coding and to embrace the full breadth of what programming can offer, we invite you to explore our Python Mini-Degree. It’s a substantial leap towards fluency in one of the most versatile programming languages out there.
Python’s ease of use and powerful potential in various fields make it an excellent choice for both novice coders and seasoned developers. Our Python Mini-Degree will guide you through coding fundamentals, all the way to real-world applications, such as game development, AI, and data science. You will build a robust foundation, create projects that excite you, and gain valuable skills that can be the cornerstone of your career.
For those who wish to broaden their expertise even further, browse our extensive catalog of Programming courses. With Zenva, you’re not just learning; you’re preparing to make an impact in the tech world. Begin your next chapter today and transform potential into expertise.
Conclusion
In the landscape of technology, where change is the only constant, the one timeless skill is understanding the basics. Datatypes are the alphabet of the programming language, and mastering them is your first step towards becoming fluent. As we’ve explored, the practical application of datatypes is vast and varied – from creating simple calculators to orchestrating complex systems. Now equipped with this essential knowledge, you are ready to delve into the greater challenges and rewards that coding offers.
At Zenva, our mission is to provide you with the tools, knowledge, and support to navigate the tech realm confidently. Whether it’s through our Python Mini-Degree or any other comprehensive course we offer, your journey from eager learner to savvy developer is just one click away. Take that first step with us, and let’s code not just with proficiency, but with creativity and passion in a world that thrives on innovation.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
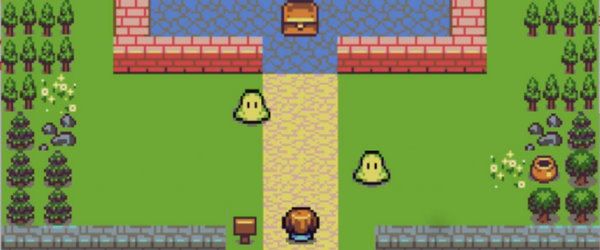
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.