Delving into game development and the exciting realm of animations can be a rewarding journey. This tutorial aims to guide you through Pygame animations, a vital part of creating dynamic and interactive games. Pygame provides a powerful framework to control and manipulate game entities, enabling developers to create more engaging games.
Table of contents
What are Pygame animations?
Pygame animations are part of the Pygame library in Python, specifically designed to facilitate game development. They allow for the smooth movement of game characters and objects, enhancing the overall visual experience of your games.
Why should I learn Pygame animations?
Learning Pygame animations will equip you with the necessary tools to make your games more lively and interactive. Animations are a key aspect of game development, transforming static images into vivid, dynamic scenes. Additionally, they are a great stepping stone for anyone aiming to delve deeper into game programming.
What can I achieve with Pygame animations?
With Pygame animations, the possibilities are endless. You can make game characters move, objects oscillate, and create a myriad of effects to enhance the user experience. By mastering this tool, you will have a significant advantage in Python game development.
Installing Pygame
Before we dive into code examples, let’s make sure Pygame is correctly installed. Installing this Python module can be performed easily via pip, Python’s package installer. Type the following command line instruction in your terminal:
pip install pygame
Creating a Basic Pygame Window
Let’s start with the basic blueprint of every Pygame application: a display window. Pygame includes a module called display, that allows us to create a game window. Here’s a basic script to create an empty window.
import pygame # Initialize all imported pygame modules pygame.init() # Set window dimensions window_size = (800, 600) # Create game window game_window = pygame.display.set_mode(window_size) # Dummy game loop running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # Uninitialize all pygame modules pygame.quit()
Animating A Simple Rectangle
Let’s animate a simple rectangle to get a basic understanding of five important elements: the game loop, the update of the object’s position, the drawing of the object, the filling of the screen, and the flipping of the display.
import pygame pygame.init() # Window window_size = (800,600) game_window = pygame.display.set_mode(window_size) # Rectangle x = 50 y = 50 width = 64 height = 64 vel = 5 # Game Loop run = True while run: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False # Movement keys = pygame.key.get_pressed() if keys[pygame.K_LEFT] or keys[pygame.K_a]: x -= vel if keys[pygame.K_RIGHT] or keys[pygame.K_d]: x += vel if keys[pygame.K_UP] or keys[pygame.K_w]: y -= vel if keys[pygame.K_DOWN] or keys[pygame.K_s]: y += vel # Draw Rectangle and Update Display game_window.fill((0,0,0)) pygame.draw.rect(game_window, (255,0,0), (x, y, width, height)) pygame.display.update() # Quit Pygame pygame.quit()
Animating a Sprite
We have animated a simple object, but games usually contain characters or sprites. Let’s animate a simple sprite image.
import pygame, sys, os pygame.init() # Window window_size = (800,600) game_window = pygame.display.set_mode(window_size) # Sprite sprite = pygame.image.load(os.path.join('Assets', 'mysprite.png')) sprite_rect = sprite.get_rect() # Game Loop run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False # Movement keys = pygame.key.get_pressed() if keys[pygame.K_LEFT] or keys[pygame.K_a]: sprite_rect.x -= 5 if keys[pygame.K_RIGHT] or keys[pygame.K_d]: sprite_rect.x += 5 if keys[pygame.K_UP] or keys[pygame.K_w]: sprite_rect.y -= 5 if keys[pygame.K_DOWN] or keys[pygame.K_s]: sprite_rect.y += 5 # Update Display game_window.fill((0,0,0)) game_window.blit(sprite, sprite_rect) pygame.display.update() # Quit Pygame pygame.quit()
Remember to replace ‘Assets/mysprite.png’ with the path to your sprite file. In this example, our game character can be moved in any direction using the arrow keys or WASD.
Animating Multiple Sprites
Most games involve more than one entity. Multiple sprites can be animated using Pygame’s Sprite and Group objects.
import pygame, sys, os pygame.init() # Window window_size = (800,600) game_window = pygame.display.set_mode(window_size) # Sprite Class class MySprite(pygame.sprite.Sprite): def __init__(self, image_path, x, y): super().__init__() self.image = pygame.image.load(image_path) self.rect = self.image.get_rect() self.rect.x = x self.rect.y = y # Sprites sprite1 = MySprite(os.path.join('Assets', 'mysprite1.png'), 50, 50) sprite2 = MySprite(os.path.join('Assets', 'mysprite2.png'), 200, 200) # Group sprites = pygame.sprite.Group(sprite1, sprite2) # Game Loop run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False # Update Display game_window.fill((0,0,0)) sprites.draw(game_window) pygame.display.update() # Quit Pygame pygame.quit()
Two sprites are created and added to a Group. In each iteration of the game loop, the entire group of sprites is drawn on the game window.
Animating Sprites with Keyboard Interaction
Let’s make our game more interactive by moving one of our sprites using keyboard inputs.
import pygame, sys, os pygame.init() # Window window_size = (800,600) game_window = pygame.display.set_mode(window_size) # Sprite Class class MySprite(pygame.sprite.Sprite): def __init__(self, image_path, x, y): super().__init__() self.image = pygame.image.load(image_path) self.rect = self.image.get_rect() self.rect.x = x self.rect.y = y # Sprites sprite1 = MySprite(os.path.join('Assets', 'mysprite1.png'), 50, 50) sprite2 = MySprite(os.path.join('Assets', 'mysprite2.png'), 200, 200) # Group sprites = pygame.sprite.Group(sprite1, sprite2) # Game Loop run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False # Movement keys = pygame.key.get_pressed() if keys[pygame.K_LEFT] or keys[pygame.K_a]: sprite1.rect.x -= 5 if keys[pygame.K_RIGHT] or keys[pygame.K_d]: sprite1.rect.x += 5 if keys[pygame.K_UP] or keys[pygame.K_w]: sprite1.rect.y -= 5 if keys[pygame.K_DOWN] or keys[pygame.K_s]: sprite1.rect.y += 5 # Update Display game_window.fill((0,0,0)) sprites.draw(game_window) pygame.display.update() # Quit Pygame pygame.quit()
In this example, we have added keyboard events to move the sprite1 object. The movement is handled in the game loop.
Animating Sprites with Mouse Interaction
What about the mouse? Let’s create some mouse interaction by animating a sprite towards the mouse’s current position when clicking the left mouse button.
import pygame, sys, os pygame.init() # Window window_size = (800,600) game_window = pygame.display.set_mode(window_size) # Sprite Class class MySprite(pygame.sprite.Sprite): def __init__(self, image_path, x, y): super().__init__() self.image = pygame.image.load(image_path) self.rect = self.image.get_rect() self.rect.x = x self.rect.y = y # Sprite sprite = MySprite(os.path.join('Assets', 'mysprite.png'), 50, 50) # Game Loop run = True while run: for event in pygame.event.get(): if event.type == pygame.QUIT: run = False elif event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: # Left mouse button. # Get mouse position. pos = pygame.mouse.get_pos() # Update sprite position. sprite.rect.x = pos[0] sprite.rect.y = pos[1] # Update Display game_window.fill((0,0,0)) game_window.blit(sprite.image, sprite.rect) pygame.display.update() # Quit Pygame pygame.quit()
This example shows how we can create interaction with the mouse. The sprite follows the position of the mouse when the left button is clicked.
Adding Frame Rate
In the previous examples, you may have noticed that the sprite animations might not look very smooth. This often indicates the absence of a frame rate control mechanism. Let’s add a clock to fix this and limit the frame rate.
import pygame, sys, os pygame.init() # Window window_size = (800,600) game_window = pygame.display.set_mode(window_size) # Sprite Class class MySprite(pygame.sprite.Sprite): def __init__(self, image_path, x, y): super().__init__() self.image = pygame.image.load(image_path) self.rect = self.image.get_rect() self.rect.x = x self.rect.y = y # Sprite sprite = MySprite(os.path.join('Assets', 'mysprite.png'), 50, 50) # Clock clock = pygame.time.Clock() # Game Loop run = True while run: clock.tick(60) # Limit to 60 frames per second. for event in pygame.event.get(): if event.type == pygame.QUIT: run = False elif event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: # Left mouse button. # Get mouse position. pos = pygame.mouse.get_pos() # Update sprite position. sprite.rect.x = pos[0] sprite.rect.y = pos[1] # Update Display game_window.fill((0,0,0)) game_window.blit(sprite.image, sprite.rect) pygame.display.update() # Quit Pygame pygame.quit()
In this example, we create a Clock object and call tick(60) in the game loop to limit the game to 60 frames per second.
Animating with Smooth Movement
Our sprite’s movement can be made smoother by making it gradually accelerate towards its target, instead of jumping directly to the target position.
import pygame, sys, os pygame.init() # Window window_size = (800,600) game_window = pygame.display.set_mode(window_size) # Sprite Class class MySprite(pygame.sprite.Sprite): def __init__(self, image_path, x, y): super().__init__() self.image = pygame.image.load(image_path) self.rect = self.image.get_rect() self.rect.x = x self.rect.y = y # Sprite sprite = MySprite(os.path.join('Assets', 'mysprite.png'), 50, 50) # Clock clock = pygame.time.Clock() # Target Position target_pos = [sprite.rect.x, sprite.rect.y] # Game Loop run = True speed = 5 while run: clock.tick(60) # Limit to 60 frames per second. for event in pygame.event.get(): if event.type == pygame.QUIT: run = False elif event.type == pygame.MOUSEBUTTONDOWN: if event.button == 1: # Left mouse button. # Get mouse position. target_pos = list(pygame.mouse.get_pos()) # Smooth Movement dx = target_pos[0] - sprite.rect.x dy = target_pos[1] - sprite.rect.y if abs(dx) > 3: sprite.rect.x += speed if dx > 0 else -speed if abs(dy) > 3: sprite.rect.y += speed if dy > 0 else -speed # Update Display game_window.fill((0,0,0)) game_window.blit(sprite.image, sprite.rect) pygame.display.update() # Quit Pygame pygame.quit()
This way, the sprite gradually moves towards the mouse click position, providing a smoother visual effect.
Successfully animating sprites is a significant move in your game development journey, but there’s always more to learn and improve. The use of Pygame is just one tool in your expanding toolbox. Now that you’ve grasped the concepts of Pygame animations, you might be wondering where to go next.
Zenva, providing over 250 professional and beginner courses in programming, game development, and AI, can guide you further. Thus, we highly encourage you to continue your learning journey with our comprehensive Python Mini-Degree. This collection of courses is designed to teach you Python programming’s breadth and depth, from the basic syntax and data structures to advanced topics like building games, developing apps, and delving into data science and machine learning. It equips you with versatile skill sets, valuable in a variety of sectors.
Additionally, you can check out our complete set of Python courses. Moving forward, Zenva is here to support and guide you in your quest to go from novice to professional.
Conclusion
With the knowledge of Pygame animations now in your tool-belt, you are better equipped to make engaging and dynamic games. These elements provide a lush, interactive experience for the users, making your games more enjoyable and lifelike!
At Zenva, we believe in fostering a natural growth curve in your coding journey. Be sure to check out our Python Mini-Degree course for a comprehensive and structured approach to expanding your Python skills. With a multitude of topics covered, you’ll find every resource you need to succeed in your coding journey!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
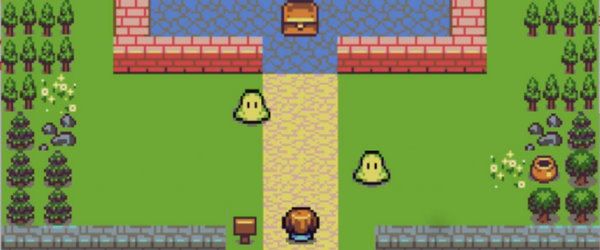
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.