Sure, let’s get started with the introduction to our Python Pygame buttons tutorial.
Welcome to an engaging, informative journey into creating buttons in Pygame. Pygame is a Python library designed for game creation, including UI elements such as buttons. Through this tutorial, we’ll uncover the simplicity and functionality of crafting buttons in Pygame, providing the cornerstones of interactivity for your games.
Table of contents
What are Buttons in Pygame?
Buttons in Pygame are interactive elements commonly used in game design. They are clickable areas on the screen that, when pressed, can trigger certain actions. From starting a game to moving a character, buttons form the core bridge between the user and the game.
What is Their Purpose?
In Pygame, buttons serve to facilitate player interaction, enhance the visual display of games, and effectively manage the game flow. They can lead to different game states, making them integral to game navigation and logic.
Why Should I Learn to Create Buttons in Pygame?
Learning to create buttons in Pygame empowers you to design interactive and dynamic games. It equips you with a critical skill to enhance game feel, improve user experience, and understand design principles more effectively. Plus, it’s an engaging and valuable skill to acquire in your coding journey.
Creating a Basic Button in Pygame
Let’s start with creating a basic button. The first thing you need to do is set up your game environment, initialize Pygame, and create a window.
import pygame pygame.init() window = pygame.display.set_mode((800, 600))
With that done, our next step is to define our button. This is done by creating a rectangular surface, which we’ll do using the pygame’s Rect class. For simlicity, let’s create a red square button.
red_button = pygame.Surface((50, 50)) red_button.fill((255, 0, 0))
Placing and Drawing a Button
You can place this button on the window using the `blit` method. Let’s put it at a random position, say (200, 200), and then update the display.
window.blit(red_button, (200, 200)) pygame.display.update()
The blit function takes two parameters: the source surface and the coordinates for the top-left corner of the source surface. The `pygame.display.update` function is used to update the display window.
Making the Button Interact-able
Now, while we have a visible button, it’s not yet interactable. Let’s handle that by introducing click events into our Pygame event loop.
run = True while run: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False if event.type == pygame.MOUSEBUTTONUP: pos = pygame.mouse.get_pos() if red_button.get_rect().collidepoint(pos): print("Button clicked!")
In Pygame, the MOUSEBUTTONUP event is triggered whenever you click on the window, providing us with the position of the mouse pointer. Our code checks whether the position of the mouse pointer when clicked matches the position of our button – if it does, it prints a message that will appear in our console.
Adding Text to Button
Our button is functional, but let’s improve it by adding a label. Pygame allows text rendering through font objects.
font = pygame.font.Font(None, 35) text = font.render('Click Me!', True, (255, 255, 255))
The `render` function creates a Surface object from the text given. This object can then be drawn onto our button just like before.
red_button.blit(text, (5, 5)) window.blit(red_button, (200, 200)) pygame.display.update()
Changing Button Color On Hover
Next, let’s make our button more dynamic by changing its color when the mouse is hovered over it.
hovered = False run = True while run: pygame.time.delay(100) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False if event.type == pygame.MOUSEMOTION: pos = pygame.mouse.get_pos() if red_button.get_rect().collidepoint(pos): hovered = True else: hovered = False if hovered: red_button.fill((200, 0, 0)) else: red_button.fill((255, 0, 0)) red_button.blit(text, (5, 5)) window.blit(red_button, (200, 200)) pygame.display.update()
We introduced a new flag, `hovered`, that checks if the mouse is currently over the button. If it is, we change the color of the button to a darker red. When the mouse moves away, the button changes back to the original red color.
Multiple Buttons
Finally, let’s dive into creating multiple buttons. We start by defining several buttons and positioning them differently on the screen.
red_button2 = pygame.Surface((50, 50)) red_button2.fill((255, 0, 0)) red_button3 = pygame.Surface((50, 50)) red_button3.fill((255, 0, 0))
Next, space these buttons out on the screen and load them in the window. Distinguish each button by the action they trigger upon being clicked.
window.blit(red_button2, (400, 200)) window.blit(red_button3, (600, 200)) pygame.display.update()
Remember to implement the respective click events for these new buttons. Your game now has distinct buttons that users can interact with!
Enhancing Button Styles
Let’s venture further and enhance our buttons’ visual appeal. One effective approach is employing images for your buttons.
start_img = pygame.image.load('start.png') start_button = pygame.Surface(start_img.get_rect().size) start_button.blit(start_img, (0, 0))
With this, we load an image using the `pygame.image.load` function and create a surface that matches its dimensions. Then we bind the image onto the button. It’s important to ensure that your image file is in the same directory as your Python script.
Button Classes for Efficient Code
At this point, you might have noticed that our code is becoming repetitive, especially when adding more buttons. To make the process more efficient, we can encapsulate button properties and behaviors within a Python class.
class Button(): def __init__(self, color, x,y,width,height, text=''): self.color = color self.x = x self.y = y self.width = width self.height = height self.text = text
We’ve defined properties such as color, coordinates, dimensions, and text. We can also define a method to draw the button.
def draw(self,win): pygame.draw.rect(win, self.color, (self.x,self.y,self.width,self.height),0)
This class encapsulates the concept of a button in our game, reducing redundancy and making our code cleaner and more manageable.
Using The Button Class
With our Button class ready, we can easily create, draw, and manage our buttons. They are now objects that carry their properties and behaviors. Creating a new button has become as simple as declaring a new instance of the Button class.
start_button = Button((255, 0, 0), 200, 200, 50, 50, 'Start') start_button.draw(window)
The button class makes it easy to include any amount of buttons in the game with ease, manage their properties, and handle their behaviors in a way that is true to the principles of object-oriented programming. It’s a big leap forward from where we started, bringing us numerous possibilities and opportunities in game design.
Summary and Next Steps
Learning to create, style, and manage buttons in Pygame is an invaluable skill for any aspiring game developer. It is the foundation of player-game interaction and contributes significantly to game feel and player experience.
We at Zenva encourage you to explore beyond what you’ve learned here. Try creating different buttons styles or building a menu system! Remember, the sky’s the limit when it comes to creativity in your game development journey.
Stay tuned to our blog for more GameDev tutorials.
Further Learning and Next Steps
Keep the momentum of your learning journey going! If you’ve enjoyed learning about buttons in Pygame and are excited about furthering your Python and game development skills, we recommend our comprehensive Python Mini-Degree at Zenva.
Our Python Mini-Degree is an amalgamation of project-based courses which guide you through coding basics, algorithms, object-oriented programming, game development, and even app development. This wide variety means you’ll be building your own games, algorithms, and real-world apps, all in Python!
Python’s simplicity and versatility make it a preferred choice, especially in key industries like data science, machine learning, and space exploration. The skills you learn can open doors to a myriad of job opportunities and boost your career trajectory in the technology industry.
We also offer a wider collection of Python courses to fit your needs. Whether you’re a beginner or an established coder wishing to level up, there’s always something new to learn with us at Zenva.
Conclusion
To wrap up, the art of crafting buttons in Pygame is more than just a technical skill – it’s the key to transforming your static games into interactive experiences. Understanding the anatomy of these fundamental game elements is the first step towards creating engaging, dynamic content for your players.
Your journey doesn’t stop here. Keep refining your game building skills and explore our Python Mini-Degree for an in-depth dive into Python’s vast landscape, from game development to real-world applications. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
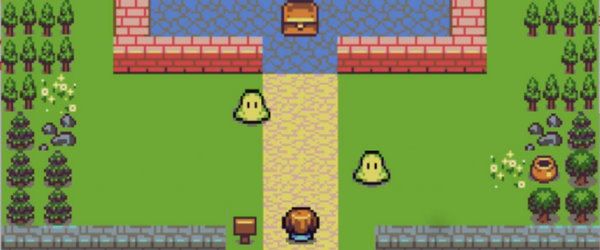
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.