Walk into the vibrant world of game development with us as we take a deep dive into the nut and bolts of Pygame’s color filling techniques. This tutorial guarantees an engrossing learning experience as we uncover the magical wand of color in Pygame, an open-source library designed for making video games in Python.
Table of contents
Pygame and fill color – What is it?
Pygame is a powerful Python module used for creating visually appealing video games. One of its most utilized features is the Fil module. The fill function in Pygame is invaluable as it allows developers to fill an entire surface object, or a specific rectangular area of the surface, with a solid color.
Why learn about Pygame fill color?
Manipulation of color is a pivotal aspect in game development. It significantly impacts the game’s aesthetics and user experience – a dull color palette might translate as a boring game, while vibrant visual features can make the game feel exciting.
The power of fill color in Pygame
Using the fill function can change the entire atmosphere of our game, from the background color to the color of game objects. Expert knowledge in color manipulation can consequently level up our game creation process, making our games look more professional and enticing.
Furthermore, understanding the Pygame’s fill functionality offers a steep learning curve in game programming basics. This is why we believe in delivering a comprehensive guide on this topic. So, let’s dive in!
Understanding Pygame’s fill Method
Pygame represents colors in RGB format – Red, Green, Blue. Each color varies from 0 to 255, where 0 represents the color’s weakest intensity and 255 its strongest intensity. In this context, the color Black (0, 0, 0) signifies a lack of colors whereas White (255, 255, 255) represents the superposition of all colors at their maximum intensity.
Let’s code a simple Pygame window and fill it with a color. In this case, we’ll color it white.
Here’s the necessary procedure to create a white surface:
import pygame pygame.init() # Set the dimensions of the window gameDisplay = pygame.display.set_mode((800,600)) # Fill the surface object white gameDisplay.fill((255, 255, 255)) pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
In the above example the `fill` function fills the entire window with white color. The function receives as argument a tuple representing the RGB value of the color.
Filling a Specific Rectangle – A Basic Example
How about not filling our entire game screen, but only specific rectangular areas of it? Let’s see with Pygame how this is done. For this code snippet, we will create a green rectangle.
# Set the dimensions of the window gameDisplay = pygame.display.set_mode((800,600)) # Fill the entire surface object white gameDisplay.fill((255, 255, 255)) # Fill a specific rectangular area with green color pygame.draw.rect(gameDisplay, (0, 255, 0), (150, 100, 200, 100)) pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
In this example, along with the color we needed to specify the rectangle’s position and dimensions. The tuple `(150, 100, 200, 100)` specifies the rectangle’s top-left corner position (150, 100), its width (200), and its height (100).
This was just to whet your appetite. In the next step, we’ll see more advanced examples of color filling.
Color Filling Different Shapes
Who said that we can only fill rectangles? With Pygame, we can also fill different shapes like circles and polygons. Let’s explore how to accomplish this.
Filling a Circle
For this, we will use the `pygame.draw.circle()` function. Here’s a code snippet to demonstrate filling a circle:
gameDisplay = pygame.display.set_mode((800,600)) gameDisplay.fill((255, 255, 255)) # Fill the surface object white # Fill a circle with red color pygame.draw.circle(gameDisplay, (255, 0, 0), (400, 300), 50) pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
In the above code, in addition to the color we provided the circle’s center (`(400, 300)`) and radius (`50`).
Filling a Polygon
Filling a polygon is a little more complicated than filling a rectangle or circle, as we need to provide the coordinates of each of the polygon’s vertices.
gameDisplay = pygame.display.set_mode((800,600)) gameDisplay.fill((255, 255, 255)) # Fill the surface object white # Fill a polygon with blue color pygame.draw.polygon(gameDisplay, (0, 0, 255), [(400,300), (500,320), (450,250), (350,250)]) pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
Here, we’ve specified the vertices of the polygon with the use of tuples.
Filling Areas with Gradients
When the `fill` function isn’t enough, we can create gradients to fill our games with color. While Pygame doesn’t support gradients natively, we can create our own.
gameDisplay = pygame.display.set_mode((800,600)) # Gradient from green to white for i in range(300): pygame.draw.line(gameDisplay, (0, i, 0), (0, i), (800, i)) pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
In this one, we’ve used the `pygame.draw.line()` function to draw many horizontal lines with varying color intensities, simulating a vertical gradient effect.
Through a deeper understanding and clever usage of Pygame’s color filling techniques, developers can bring their video games to life with vibrant backgrounds, animated characters and dynamic visual elements – a key factor in generating engaging player experiences.
More Ways to Play with Colors
As we move forward, we’ll learn how to fill surfaces with different alpha values, create color overlays, and fill in text! Let’s march on.
Filling a Surface with Alpha Value
We can fill a surface with an alpha value to create transparent colors. For this, we’ll first need to create a surface, set its transparency value with `set_alpha()` method and then fill it.
gameDisplay = pygame.display.set_mode((800,600)) gameDisplay.fill((255, 255, 255)) # Fill the surface object white surface = pygame.Surface((100, 100)) surface.set_alpha(128) # Set alpha value surface.fill((255, 0, 0)) # Fill the surface object with red color gameDisplay.blit(surface, (250, 250)) # Apply the surface pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
In the above snippet, we are creating a semi-transparent red square. Using alpha value can render the illusion of depth, supporting us to produce shadow and lighting effects.
Creating Color Overlays
Using Pygame, we can also create color overlays. Overlays can enhance user experience by highlighting specific objects or guiding the player through a difficult level.
gameDisplay = pygame.display.set_mode((800,600)) gameDisplay.fill((255, 255, 255)) # Fill the surface object white overlay = pygame.Surface((800, 600)) overlay.set_alpha(128) # Semi-transparent overlay overlay.fill((0, 0, 255)) # Create blue colored overlay gameDisplay.blit(overlay, (0, 0)) # Draw the overlay from top-left corner pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
Here, we are drawing a semi-transparent blue overlay across our entire game screen.
Filling-In Text
Lastly, let’s get creative with colors by applying it on text. Here’s a simple demonstration:
gameDisplay = pygame.display.set_mode((800,600)) gameDisplay.fill((255, 255, 255)) # Fill the surface object white font = pygame.font.Font(None, 36) # Choose the font for the text text = font.render("Hello, Zenva!", 1, (0, 0, 255)) # Generate the text gameDisplay.blit(text, (250, 250)) # Draw the text on the screen pygame.display.update() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() quit()
We first choose our desired font, then create the text using the `pygame.font.Font.render()` method, where we specify the string, anti-aliasing parameter and the color.
Conclusively, color filling in Pygame infuses life into our games, ensuring they are visually delightful, engaging and enjoyable. Through the right use of Pygames’s color-related functions, we at Zenva continue to educate and encourage budding coders and game developers to bring their ideas to life!
Keep Growing with Zenva
If this exciting journey through color filling in Pygame has sparked your interest, we highly encourage you to keep going! Learning never stops, and there are so many exciting facets of Python and Pygame yet to explore.
As your trusted guide, we invite you to check out our comprehensive Python Mini-Degree program. Covering a diverse range of topics from the basics of coding to advanced game and app development, the mini-degree program empowers you to create dynamic games, real-world apps and solve complex algorithms. As Python powers data science, machine learning and computer vision industries, this could be your step towards high-demand job opportunities in various fields.
Additionally, you can browse through our vast collection of Python courses that cater to learners at all levels. The best part of learning with us at Zenva is flexibility! You can have access to all our courses at any time, on any device. Our curriculum is designed to be at your own pace, with unique features like live coding lessons, quizzes, and completion certificates. It’s time to turn on your game development mode and start your learning adventure with Zenva. We look forward to being a part of your success story!
Conclusion
You’ve taken a vibrant journey exploring Pygame’s color filling techniques with us, unlocking the mesmerizing world of colors and enhancing the aesthetics of your game development process. Remember, the art of color filling is not just for your game’s backgrounds, but to capture players’ attention, create moods, and add depth to your game!
Embrace the color palette of Pygame and light-up your world of gaming. With Zenva, continue turning your imagination into compelling game narratives. Check out our rich resource of courses in Python Mini-Degree program and add vibrant colors of knowledge to your programming journey. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
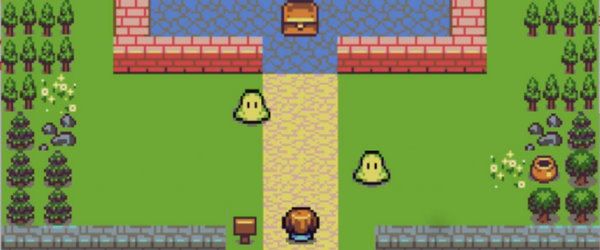
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.