Today’s tutorial delves into the wonderful world of Pygame, placing a specific focus on scaling images. Pygame is a powerful set of Python modules designed for writing video games, and learning how to scale images within this platform plays an integral part in game development.
Table of contents
What is Image Scaling?
Image scaling is the process of sizing a graphics file up or down. This is a particularly useful skill for developers who frequently work with 2D visuals. Whether you’re working on a retro-style platformer or an elaborate RPG, having the ability to manipulate the dimensions of your images is essential for good gameplay and an engaging user interface.
Why Learn Image Scaling?
In game development, image scaling is a fundamental skill. With it, you can create animations, dynamic textures or adjust the proportions of sprite images for different resolutions. Not only does this skill improve the visual aesthetics of your game, it opens up a whole host of creative possibilities for your game mechanics. In sum, mastering image scaling is a pivotal step towards becoming a confident and versatile game developer.
Without further ado, let us delve into the exciting coding journey ahead and explore how to scale images in Pygame!
Scaling Images with Pygame
To kick off our coding tutorial, let’s look at a basic example of how to load and scale an image using Pygame.
# import pygame module import pygame # initialize Pygame pygame.init() # load image image = pygame.image.load('example.jpg') # scale image scaled_image = pygame.transform.scale(image, (200, 200))
In this example, an image named ‘example.jpg’ is loaded and then resized using the pygame.transform.scale() function.
Exploring the Pygame Transform Module
The Pygame transform module is a powerful tool in image manipulation. It contains other functions you can also use to rotate, flip or even smooth scale your images. Here’s another example that demonstrates how to use the smooth scaling method:
# import pygame module import pygame # initialize Pygame pygame.init() # load image image = pygame.image.load('example.jpg') # smooth scale image smooth_scaled_image = pygame.transform.smoothscale(image, (200, 200))
In this code snippet, the pygame.transform.smoothscale() function smooth scales the image to the desired size.
Continuous Learning with Zenva
If you found this tutorial insightful and you’re eager to learn more, we highly recommend our Python Mini-Degree. It’s a comprehensive course that delves into Python programming, including modules like Pygame.
At Zenva, we believe in providing high-quality education that caters to all levels of learners. Whether this is your first dive into programming, or you’re an experienced developer looking to expand your toolkit, our Python Mini-Degree is designed to advance your learning at your own pace.
Conclusion
Image scaling is an invaluable skill in crafting engaging, dynamic games. We have explored the basics of how to manipulate image sizes using Pygame, from loading images to adjusting their dimensions. We also gave you a sneak peek into the functionalities of the Pygame transform module.
Embrace the next steps in your coding journey and delve deeper into Python programming with our Python Mini-Degree. Keep striving, continue learning and before long, you’ll have a well-rounded skill set that can propel you in your game creation or any programming venture you wish to undertake. Happy coding!
Remember, at Zenva, we are dedicated to fostering a learning environment that suits you, and the journey doesn’t stop here. After all, there is no end to education. It’s not that you read a book, pass an examination, and finish with learning. The whole of life, from the moment you are born to the moment you die, is a process of learning. So, go forth and keep exploring the friendly horizons of programming!
Loading and Displaying Scaled Images
Once you’ve scaled your images, you’ll want to display them in your Pygame window. First, let’s discuss how to load and display an image using Pygame, and then we’ll add in our scaling function.
# import pygame module import pygame # initialize Pygame pygame.init() # Create game window win = pygame.display.set_mode((500, 500)) # Load image image = pygame.image.load('example.jpg') # display image in game window win.blit(image, (0, 0)) # Update display pygame.display.update()
Now, let’s include our scaling function:
# import pygame module import pygame # initialize Pygame pygame.init() # Create game window win = pygame.display.set_mode((500, 500)) # Load and scale image image = pygame.image.load('example.jpg') image = pygame.transform.scale(image, (200, 200)) # display scaled image in game window win.blit(image, (0, 0)) # Update display pygame.display.update()
In this example, we first create a Pygame window of 500×500 pixels. Then we load and scale our image, and use the blit() method to draw it onto our game window.
Manipulating Scaled Images
Now let’s dive deeper into manipulating our scaled image, and explore the rotation function:
# import pygame module import pygame # initialize Pygame pygame.init() # Create game window win = pygame.display.set_mode((500, 500)) # Load and scale image image = pygame.image.load('example.jpg') image = pygame.transform.scale(image, (200, 200)) # Rotate scaled image rotated_image = pygame.transform.rotate(image, 90) # Display rotated image in game window win.blit(rotated_image, (0, 0)) # Update display pygame.display.update()
In this code, we use pygame.transform.rotate() to rotate our scaled image by 90 degrees. Next, let’s see how we can flip the image:
# import pygame module import pygame # initialize Pygame pygame.init() # Create game window win = pygame.display.set_mode((500, 500)) # Load and scale image image = pygame.image.load('example.jpg') image = pygame.transform.scale(image, (200, 200)) # Flip scaled image flipped_image = pygame.transform.flip(image, True, False) # Display flipped image in game window win.blit(flipped_image, (0, 0)) # Update display pygame.display.update()
In this example, the function pygame.transform.flip() is used. The first parameter after the referenced image is whether to flip the image horizontally, and the second parameter indicates whether to flip it vertically.
Please note that you need to put these coding examples in a Pygame loop. However, for the purpose of easy understanding, we have kept the codes inside the shortest possible format.
Remember, understanding these image transformations can give you greater flexibility in game design, and allow you to take your creativity to new heights!
Dynamically Scaling Images
Instead of just statically scaling an image to a fixed size, let’s see how we might dynamically adjust image dimensions in response to an event.
Here’s a basic example of dynamically increasing the size of an image when the space bar is pressed:
# import pygame module import pygame # initialize Pygame pygame.init() # Create game window win = pygame.display.set_mode((500, 500)) # Load image image = pygame.image.load('example.jpg') image_size = 200 running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False # detect space bar press if event.type == pygame.KEYDOWN: if event.key == pygame.K_SPACE: image_size += 10 # Scale and display image image = pygame.transform.scale(image, (image_size, image_size)) win.blit(image, (0, 0)) # Update display pygame.display.update() pygame.quit()
In this example, we’ve added a Pygame event loop to our game window. We start with an initial image size of 200 pixels, and every time the space bar is pressed, we increase the size of the image by 10 pixels.
Scaling Images to Window Size
Another common scenario in game development is scaling an image to fit the game window, no matter its size. Here’s how you might do it:
# import pygame module import pygame # initialize Pygame pygame.init() # Set window size win_width = 800 win_height = 600 # Create game window win = pygame.display.set_mode((win_width, win_height)) # Load and scale image image = pygame.image.load('example.jpg') image = pygame.transform.scale(image, (win_width, win_height)) # Display scaled image in game window win.blit(image, (0, 0)) # Update display pygame.display.update()
In this example, we first set our window size, then we load and scale our image to match the window dimensions.
Scaling Images relative to their Original Size
Sometimes, you may want to scale an image relative to its original size. Let’s look at an example of making an image half of its original size:
# import pygame module import pygame # initialize Pygame pygame.init() # Create game window win = pygame.display.set_mode((500, 500)) # Load image image = pygame.image.load('example.jpg') # Get original size of the image orig_width, orig_height = image.get_size() # Scale image scaled_image = pygame.transform.scale(image, (orig_width//2, orig_height//2)) # Display scaled image in game window win.blit(scaled_image, (0, 0)) # Update display pygame.display.update()
In this example, the get_size() function is used to get the original size of the image, which in turn is used to calculate the new dimensions.
These examples should give you a good understanding of how to work with image scaling in Pygame. Learning how to control your game’s images can allow you to create visually engaging and unique gaming experiences that cater to various display sizes and resolutions. Keep practicing and experiment with different scenarios to become a more versatile game developer! Remember, in the world of game creation, your creativity is your only limit!
Scaling Spritesheets
Another common usage of scaling in pygame is resizing spritesheets. Once a spritesheet is loaded, individual images (sprites) can be extracted and scaled to a uniform size, making them easier to manage and work with. Let’s see how to do that.
# import pygame module import pygame # initialize pygame pygame.init() # Load a spritesheet spritesheet = pygame.image.load('spritesheet.png') # Define the size of each sprite sprite_width = 32 sprite_height = 32 sprite_scale_factor = 2 # The size multiplier # Define the size of the scaled sprite scaled_sprite_width = sprite_width * sprite_scale_factor scaled_sprite_height = sprite_height * sprite_scale_factor # Create an empty list to store the scaled sprites scaled_sprites = [] # Iterate over the sprites in the spritesheet for y in range(spritesheet.get_height() // sprite_height): for x in range(spritesheet.get_width() // sprite_width): # Extract individual sprite sprite = pygame.Surface((sprite_width, sprite_height)) sprite.blit(spritesheet, (0, 0), (x * sprite_width, y * sprite_height, sprite_width, sprite_height)) # Scale the sprite scaled_sprite = pygame.transform.scale(sprite, (scaled_sprite_width, scaled_sprite_height)) # Add the scaled sprite to the list scaled_sprites.append(scaled_sprite)
This code example shows how to load a spritesheet, extract individual sprites, scale them, and store them in a list.
Resizing Images by Percentage
If you want to resize an image by a certain percentage, rather than a fixed pixel size, you can do so by:
# import pygame module import pygame # initialize pygame pygame.init() # Load image image = pygame.image.load('example.jpg') # Set scale factor scale_factor = 0.5 # Reduce the size by 50% # Get the original size of the image orig_width, orig_height = image.get_size() # Calculate new size new_size = (int(orig_width * scale_factor), int(orig_height * scale_factor)) # Scale image scaled_image = pygame.transform.scale(image, new_size)
In this example, we resize the image by a certain percentage using a scale factor.
Aspect Ratio and Scaling
When scaling an image, maintaining the aspect ratio (the ratio between the width and height) is often important. Let’s look at an example where we will keep the aspect ratio intact:
# import pygame module import pygame # initialize pygame pygame.init() # Load image image = pygame.image.load('example.jpg') # Set desired width desired_width = 400 # Get the original size of the image orig_width, orig_height = image.get_size() # Calculate new height maintaining the aspect ratio new_height = int(desired_width * orig_height / orig_width) # Scale image scaled_image = pygame.transform.scale(image, (desired_width, new_height))
In this example, we set a desired width, then calculate the corresponding height by keeping the same aspect ratio of the original image.
By practicing these techniques, and combining them in different ways, you can manipulate your Pygame images as required to create polished, engaging games. All these functions, methods, and properties will continue to reinforce and broaden your Pygame skill set. Happy coding!
Your Next Steps in Python and Pygame
You’ve made significant strides today by delving deep into image scaling in Pygame, a fundamental skill for any aspiring game developer. We hope that you’ve enjoyed this tutorial and feel more equipped to manipulate graphical content in interesting and engaging ways.
Want to delve deeper into Python programming and game development? Our Python Mini-Degree is exactly what you need. This comprehensive collection of courses covers everything from coding basics to complex algorithms, object-oriented programming, game development, and beyond. Suitable for beginners and experienced programmers alike, you can access these step-by-step courses anytime and anywhere. The content is updated regularly to align with industry developments.
Prefer a broader array of topics? Check out our catalog of Python courses to pick exactly what suits your learning goals.
At Zenva, we’re committed to fostering a learning environment that adapts to your needs and helps you on your journey from beginner to professional. We count it a privilege to join you on this journey and help bring your programming ideas to life. Your next big step awaits – strengthen your Python and game development skills with Zenva today!
Conclusion
And there you have it – a comprehensive guide on image scaling in Pygame! We’ve covered everything from basic image resizing to dynamic scaling and advanced manipulations like rotating and flipping images, scaling spritesheets, preserving aspect ratios, and more. These skills will prove to be invaluable as you venture deep into the world of game development and coding.
The journey of learning never really ends; it’s an ongoing process best enjoyed with a drive for discovery and improvement. At Zenva, we encourage you to keep up the momentum and continue growing as a developer. Check out our Python Mini-Degree and take full control of mastering Python programming. It’s a course designed for all skill levels, whether you’re just starting out, looking to refine your skills, or looking to pivot into new skills altogether. Remember, the best way to predict the future is to create it. So, grab your coding future with both hands, and let’s make something amazing!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
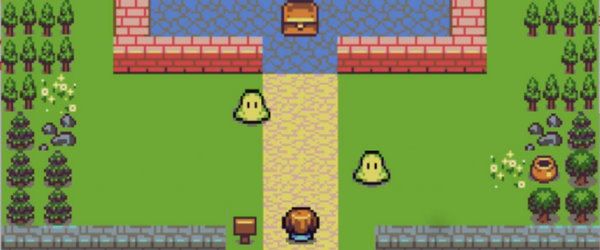
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.