In this dive into the world of Pygame, a very popular and intuitive open-source library designed for Python game development, we will explore one very useful graphic method: rotating images. A key to generating dynamic and interactive game environments, this feature allows game characters and objects to rotate, creating a more fluid and realistic gaming experience.
Table of contents
What is Pygame Image Rotation?
Pygame image rotation refers to the ability to change the orientation of an image in a Pygame environment. This is done using the pygame.transform.rotate() function which takes as parameters the original image and the angle by which it ought to be rotated.
Why Learn Pygame Image Rotation?
Being proficient in controlling image rotation in Pygame is a crucial tool in developing engaging games. Not only can it be used to control character and object movements, but it can also be utilized for creating unique game effects, such as spinning collectibles or changing viewing angles.
Whether developing a racing game with cars that need to turn, a platformer with rotating obstacles, or simply adding spinning animations, mastering Pygame image rotation can elevate a game to new dimensions of interactivity. Therefore, learning how to use this tool is an essential skill for anyone interested in game development. This knowledge can make your game more appealing and captivate your audience in a unique way. It’s all about adding layers to your game and Pygame’s image rotation is one of these layers.
Now that we’ve understood the ‘what’ and ‘why’, let’s dive into the ‘how’. So, roll up your sleeves, fire up your code editor, and let’s get coding!
Getting Started with Pygame Image Rotation
Before we can work with image rotation, it’s necessary to understand how to setup a basic Pygame window. Here’s a simple code snippet to create a 800×600 window using Pygame:
import pygame pygame.init() display_width, display_height = 800, 600 game_display = pygame.display.set_mode((display_width, display_height)) pygame.display.set_caption('Zenva Pygame Tutorial') pygame.quit()
In this simple script, we first initialized Pygame and then set some display parameters.
Working with Images in Pygame
Now let’s add an image to our Pygame window. For this example, let’s imagine we have an image file named ‘player.png’ located in the same directory as our script.
import pygame pygame.init() display_width, display_height = 800, 600 game_display = pygame.display.set_mode((display_width, display_height)) player_image = pygame.image.load('player.png') game_display.blit(player_image, (0, 0)) pygame.display.update() pygame.quit()
This script is similar to the first one, except this time around we’re loading an image into the script with pygame.image.load() and drawing this image onto our Pygame window at position (0,0) using game_display.blit().
Rotating Images in Pygame
Now that we have an image appearing in our game window, let’s make it rotate! Here’s how you can rotate an image in Pygame:
import pygame pygame.init() display_width, display_height = 800, 600 game_display = pygame.display.set_mode((display_width, display_height)) player_image = pygame.image.load('player.png') rota_image = pygame.transform.rotate(player_image, 180) game_display.blit(rota_image, (0, 0)) pygame.display.update() pygame.quit()
The new line of code, pygame.transform.rotate(), takes two parameters: the image we want to rotate and the degree by which we want to rotate it. In this case, we are rotating our image by 180 degrees.
Continuously Rotating an Image in Pygame
For our final example, let’s continuously rotate our image. To achieve this, we’ll make use of Python’s while loop.
import pygame pygame.init() display_width, display_height = 800, 600 game_display = pygame.display.set_mode((display_width, display_height)) player_image = pygame.image.load('player.png') angle = 0 running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False rota_image = pygame.transform.rotate(player_image, angle) game_display.blit(rota_image, (0, 0)) pygame.display.update() angle += 1 pygame.quit()
In this script, we continuously rotate our image by a single degree in a clockwise direction until the Pygame window is closed. The while loop helps create this continuous rotation effect, and the angle variable is used to determine the rotation of the image.
Dealing with Rotation and Image Quality
As you create more complex games, you’ll need to deal with more specific challenges related to image rotation. One issue you might experience is the loss of image quality due to continuous rotation.
Whenever a bitmap image is rotated, the pixels are re-mapped into the new orientation. This can lead to repetition of the process, causing distortion of the image. Let’s visualize this issue with example:
import pygame pygame.init() display_width, display_height = 800, 600 game_display = pygame.display.set_mode((display_width, display_height)) player_image = pygame.image.load('player.png') angle = 0 running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if angle > 360: angle = 0 else: angle += 1 rota_image = pygame.transform.rotate(player_image, angle) game_display.blit(rota_image, (0, 0)) pygame.display.update() pygame.quit()
After running the above script, you will notice the image getting blurrier with each rotation. In order to combat this, we can make sure we always rotate from the original image like so:
# ... (previous code) while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if angle > 360: angle = 0 else: angle += 1 rota_image = pygame.transform.rotate(player_image, angle) game_display.fill((0,0,0)) game_display.blit(rota_image, (0, 0)) pygame.display.update() pygame.quit()
The addition of ‘game_display.fill((0,0,0))’ will make sure the previous frame is cleared before drawing the new image.
Centering the Rotating Image
Rotating the image from its origin (top left corner by default) might not always present the best visual effect. What you might want to achieve is rotating the image around its center.
# ... (previous code) while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if angle > 360: angle = 0 else: angle += 1 rota_image = pygame.transform.rotate(player_image, angle) rect = rota_image.get_rect(center=(400,300)) game_display.fill((0,0,0)) game_display.blit(rota_image, rect.topleft) pygame.display.update() pygame.quit()
Here, we’ve used get_rect() to get the dimensions of the new rotated image. We then set the center of this image using the center parameter.
Rounding Up
As you’ve seen, image rotation in Pygame is both a necessary and powerful tool in developing engaging Python games. By allowing you to change the visual orientation of characters, objects, and entire scenes, your gaming world becomes far richer and more interactive.
Image rotation in Pygame, just like many other game development techniques, takes time to master. But with good understanding of the Pygame image rotation functionality, patience, and a bit of creativity, your gaming worlds have no bounds. Keep creating, keep learning, and most importantly, keep having fun. Happy coding!
Rotating an Image to Face the Mouse Cursor
A common application of Pygame image rotation stems from the need to orient a character or object towards a certain point, for example, the mouse cursor. Let’s go through an example where we orient our image to follow the mouse cursor.
import pygame import math pygame.init() display_width, display_height = 800, 600 game_display = pygame.display.set_mode((display_width, display_height)) player_image = pygame.image.load('player.png') running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False mouse_x, mouse_y = pygame.mouse.get_pos() rel_x, rel_y = mouse_x -300, mouse_y -300 angle = (180 / math.pi) * -math.atan2(rel_y, rel_x) rotated_image = pygame.transform.rotate(player_image, angle) rect = rotated_image.get_rect(center=(300, 300)) game_display.fill((0,0,0)) game_display.blit(rotated_image, rect.topleft) pygame.display.flip() pygame.quit()
In this script, pygame’s .mouse.get_pos() function returns the position of our mouse and using this position, we can calculate the angle between the image and the mouse pointer. The “atan2” is a math function to calculate the angle in radians, so we convert it to degrees.
Scaling the Rotating Image
As our ‘player.png’ spins, it might not be of desirable size — it could be too big or too small to be practical within our game. Enter image scaling, which in Pygame can be simply accomplished using the pygame.transform.scale() function:
# ... (previous code) player_image = pygame.image.load('player.png') player_image = pygame.transform.scale(player_image, (60, 60)) # ... (code continues)
Here we’ve scaled the ‘player.png’ image to a width and a height of 60 pixels.
Smooth Scaling
For smooth scaling, Pygame provides another function pygame.transform.smoothscale(), which gives a smoother result but it’s slower compared to pygame.transform.scale().
# ... (previous code) player_image = pygame.image.load('player.png') player_image = pygame.transform.smoothscale(player_image, (60, 60)) # ... (code continues)
With pygame.transform.smoothscale() now every time the image rotates, we see smoother image rendering.
Flipping the Image
Another powerful function provided by Pygame is flipping the image, either horizontally, vertically, or both, which could be used in many scenarios for achieving various game effects.
# ... (previous code) flipped_image = pygame.transform.flip(player_image, True, False) game_display.blit(flipped_image, rect.topleft) # ... (code continues)
Here’s you can see the flipping in action. We used pygame.transform.flip(), which takes three parameters – the image to be flipped, and two boolean values specifying whether we want to flip the image horizontally and vertically.
In the example above, we are flipping the image horizontally.
As we see, the deeper we dive into Pygame, the more we uncover its powerful functions designed to empower game developers. Whether it’s rotating, scaling, or flipping images, Pygame gives us a variety of tools to enliven our game visuals. At Zenva, we believe in making game development accessible and enjoyable for all, so keep experimenting and keep coding!
Your Next Steps in Mastering Game Development
Wow, you’ve come so far in understanding the power and potential of Pygame’s image manipulation capabilities! Now that you’ve absorbed the basics and even dove into some complex manipulations, you might be wondering, “What next?”
At Zenva, we offer an exhaustive range of programming and game development courses that cater to all levels—from complete beginner to professional. We encourage you to check out our Python Mini-Degree, a comprehensive collection of courses centered around Python programming. From diving into coding basics, algorithms, object-oriented programming, to exploring game development and app creation, these courses offer a complete Python learning experience. The Python Mini-Degree teaches popular Python libraries and frameworks such as Kivy, Tkinter, PyGame, and PySimpleGUI through comprehensive, step-by-step projects.
Additionally, we also offer a broad and diverse selection of Python courses for variety in your learning journey. Feel free to explore our Python courses that are designed to suit a range of interests and skill levels. These courses focus on different aspects of Python programming and can help turn your game development dreams into reality.
So, keep up your momentum! The wonderful world of game development awaits you, and it’s brimming with opportunities to create, learn, and grow. From us at Zenva, we wish you the best on your onward journey, and we’re excited to see what you’ll create next. Happy coding!
Conclusion
Coding games and controlling their graphics offers a thrilling ride full of constant learning and growth. Mastering image manipulation techniques, in particular, is certainly an exciting milestone in your game development journey that brings your gaming world to life. The power to rotate, flip, and scale images with Pygame’s robust functions takes you one step closer to creating games that captivate and mesmerize your audience.
At Zenva, we believe in making this journey engaging and intuitive for all. We invite you to explore our comprehensive and interactive Python Mini-Degree. With its focus on real-world applications, it is specifically designed to equip you with a holistic understanding of Python programming along with a wealth of practical knowledge to embark upon exciting game development projects. And remember, as with any coding skill, the secret to successful learning is hands-on experience and perseverance. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
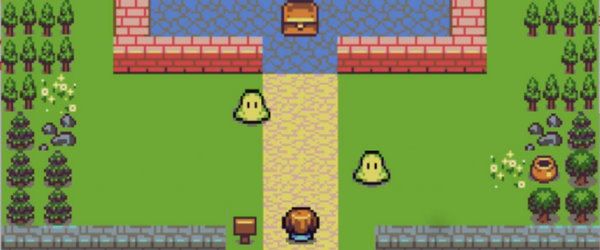
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.