Welcome, future coders and established game developers alike! Today, we’re delving into the exciting world of C# ‘n’, a version of the popular programming language C# that’s embraced by many for its simplicity and versatility.
Table of contents
What is C# ‘n’
C# ‘n’ is a powerful and user-friendly programming language widely adopted for game development, especially in engines like Unity. It’s an extension of C#, incorporating syntactic sugar that helps create efficient and easily readable code.
What is It Used For?
C# ‘n’ is predominantly utilized in game development thanks to its compatibility with popular game engines. This language is prized for its useful features including automatic memory management and robust base class libraries, crucial for creating rich, dynamic game mechanics.
Why Should I Learn C# ‘n’?
By mastering C# ‘n’, you add a powerful tool to your development toolkit. This knowledge is integral to creating compelling and intricate games, and is appealing to potential employers in the gaming industry. Besides, learning C# ‘n’ serves as a stepping stone into more advanced programming languages.
C# ‘n’ Basics
Before we delve into coding in C# ‘n’, let’s understand the basics of the language. These include variables, data-types, functions, and control flow statements.
Declaring Variables
In C# ‘n’, declaring variables is straightforward. You’ll need to first specify the data type, then the variable name, and finally you can assign a value to it.
//Declaring an integer variable int health = 100; //Declaring a String variable string playerName = "GameDev";
Data Types
The fundamental data types in C# ‘n’ that are essential for coding games include int, float, bool, and string.
//Integer type variable int score = 0; //Float type variable float speed = 5.5f; //Boolean type variable bool isAlive = true; //String type variable string characterName = "Zenva";
Functions
Functions, also called methods, are used to perform specific tasks. They can accept parameters and return a value.
//A function to calculate player's health int CalculateHealth(int currentHealth, int damage){ return currentHealth - damage; }
Control Flow Statements
Control flow statements in C# ‘n’, like in any other language, are used to control the flow of execution of the program. They include ‘if’ statement, ‘for’ loop, and ‘while’ loop.
//If statement if(score > 100) { Console.WriteLine("You won!"); } //For loop for(int i=0; i<10; i++) { Console.WriteLine(i); } //While loop while(isAlive){ //Game loop logic here }
Working with Classes and Objects
C# ‘n’ is an object-oriented programming language. As such, it uses the idea of “objects” and “classes”. Classes are blueprints or templates from which objects are created.
//Declaring a class public class Player { //Properties public int health; public string name; //Method public void TakeDamage(int damage) { health -= damage; } } //Creating an object Player player1 = new Player(); player1.health = 100; player1.name = "Zenva";
Arrays and Lists
C# ‘n’ also includes support for arrays and lists. Arrays are fixed-size collections of elements of the same data-type, while lists are dynamic-size collections.
//Array declaration int[] scores = new int[10]; scores[0] = 100; //List declaration List<string> playerNames = new List<string>(); playerNames.Add("Zenva");
Event Handling
Understanding events and how to handle them is crucial to game creation. An “event” refers to an action or occurrence detected within a program.
//Creating an event public delegate void OnPlayerDeath(); public static event OnPlayerDeath onPlayerDeath; //Triggering the event if(player1.health <= 0){ onPlayerDeath?.Invoke(); }
In this case, when our player’s health hits 0 or less, the ‘onPlayerDeath’ event is invoked, triggering any methods set to execute when this event occurs.
Access Modifiers
Last but not least, access modifiers in C# ‘n’ control the accessibility of the class, member variables, and methods. The four major types of access modifiers are Public, Private, Protected, and Internal.
public class Player { //Public variable public int health; //Private variable private string name; //Protected variable protected bool isAlive; //Internal variable internal int score; }
There you have it, a fundamental understanding of C# ‘n’ with practical coding examples. Learning this powerful language can open up a multitude of opportunities, from creating compelling games to landing a role in the thriving gaming industry. Remember, like any language, practice makes perfect, so keep coding and creating in C# ‘n’!
Operator Overloading
In C# ‘n’, we can overload operators to perform different tasks. This adds readability to the code and can make complex calculations simple. For example, to add two vectors, we can overload the ‘+’ operator.
public class Vector { public int X; public int Y; public static Vector operator +(Vector a, Vector b) { return new Vector { X = a.X + b.X, Y = a.Y + b.Y }; } } Vector vec1 = new Vector { X = 1, Y = 1 }; Vector vec2 = new Vector { X = 2, Y = 2 }; Vector vecSum = vec1 + vec2; //vecSum's X = 3, Y = 3
Generics
C# ‘n’ supports Generics, which allow you to design classes and methods that defer the specification of one or more types until the class or method is declared and instantiated by client code.
public class MyClass<T> { private T value; public void SetValue(T val) { this.value = val; } public T GetValue() { return this.value; } } MyClass<int> intObject = new MyClass<int>(); intObject.SetValue(10); intObject.GetValue(); //returns 10
Error Handling
Error handling in C# ‘n’ is done using try, catch, and finally blocks. This assists us in handling runtime errors, improving the stability of the game.
try { int x = 10; int y = 0; int result = x / y; //Throws DivideByZeroException } catch(DivideByZeroException e) { Console.WriteLine(e.Message); } finally { Console.WriteLine("This block executes irrespective of an exception"); }
Delegates and Lambda Expressions
Delegates and Lambda expressions provide a flexible mechanism for encapsulating a method into a delegate object and invoking it. Lambda expressions can help make code more readable and concise.
// Delegate declaration public delegate void MyDelegate(int a, int b); // Method that matches delegate signature public void Add(int a, int b){ Console.WriteLine(a+b); } // Delegate instantiation MyDelegate del = new MyDelegate(Add); // Delegate invocation del(5, 7); // Prints 12 // Lambda Expression Func<int, int, int> add = (a, b) => a + b; Console.WriteLine(add(5, 7)); // Prints 12
By honing your skills in C# ‘n’, you’re equipping yourself with a powerful toolkit for game development. The combination of theory and practical application is the key to mastering this language – so keep practicing!
Where To Go Next?
With the fundamentals of C# ‘n’ under your belt, the road is wide open for you to continue your game development journey. Whether you are just starting or you are an experienced developer looking to expand your skillset, you are on the right track.
We encourage you to broaden your scope with Unity, an extremely popular game engine that adopts C# as its primary scripting language. A fantastic starting point is our comprehensive Unity Game Development Mini-Degree, which covers game mechanics, animation, audio effects, and much more. These are project-based courses that will help you build a professional portfolio. This Mini-Degree is flexible and frequently updated to keep up with industry trends.
In addition, for a broader collection of courses, do check out our Unity Course Collection. With over 250 supported courses from beginner to professional, covering extensive technology areas such as programming, AI, and game development, you can keep growing with us at Zenva. Remember, the journey of game development is a marathon, not a sprint – continue learning, keep coding, and never stop having fun!
Conclusion
Mastering C# ‘n’ and becoming a game developer is a journey that takes passion and dedication. At Zenva, we are committed to providing you with the tools, resources, and knowledge to fulfill your dreams and create incredible games. Dive right into game development and make your mark on the gaming world with C# ‘n’.
Equip yourself with the confidence and knowledge you need with our Unity Game Development Mini-Degree. Turn your dreams into reality and step into your future with Zenva today. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
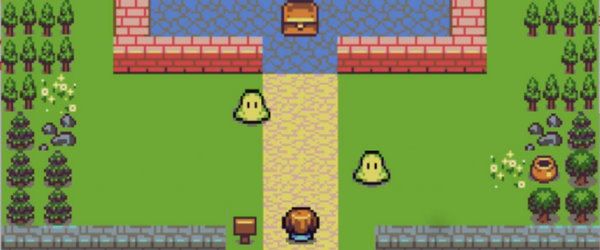
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.