Understanding software maintenance is crucial for any developer, beginner or seasoned. In today’s dynamic world, where technology advances at lightning speed, the ability to maintain and improve existing code is as important as the capacity to create new applications from scratch. This tutorial will guide you through the essentials of software maintenance. While it may not be as glamorous as building something new, maintenance is what keeps software reliable, secure, and efficient over time. By mastering this discipline, you’re equipping yourself with the skills necessary to ensure software longevity and user satisfaction.
Table of contents
What is Software Maintenance?
Software maintenance refers to the process of modifying and updating software after its initial release. It’s about more than just fixing bugs—it encompasses any activity that improves the software’s performance, adaptability, and other attributes without adding entirely new features.
What is it For?
The purpose of software maintenance is to correct any faults, improve the software’s performance or other attributes and adapt the product to a modified environment. This could include addressing new operating systems, hardware changes, or interaction with new software and technologies.
Why Should I Learn It?
Learning software maintenance is essential because it extends the life of the software and makes it more pleasant to use. For businesses, well-maintained software means lower costs in the long run, as it helps to prevent the expensive process of software redevelopment. For developers, this skill can lead to better job opportunities and a deeper understanding of how software evolves over time.
Types of Software Maintenance
Before we dive into code examples, it’s important to understand the four types of software maintenance. Each type serves a different purpose and will require different approaches and code modifications.
- Corrective Maintenance: This type involves fixing bugs and errors found in the software after its deployment.
- Adaptive Maintenance: This refers to updates made to keep the software usable in a changing environment, such as upgrading components to be compatible with new operating systems.
- Perfective Maintenance: This includes enhancing or improving features and performance of the software for a better user experience.
- Preventive Maintenance: Also known as code refactoring, this is the improvement of internal structure without changing external behavior to prevent future problems.
Corrective Maintenance
In corrective maintenance, we identify and correct errors, often after users report them. The process goes from reproducing the bug, identifying its cause, and applying a fix. Below is a typical workflow for correcting a simple logic error in a piece of software.
// Initial Code with a logical error function calculateDiscount(price, discount) { return price * discount; } // Corrected Code function calculateDiscount(price, discount) { return price - (price * discount); }
Here we fixed a logical error where the original function incorrectly calculated the discount by multiplying the price by the discount rate instead of subtracting the discount value from the price.
Adaptive Maintenance
Adaptive maintenance focuses on ensuring that the software continues to function in a new or changing environment. This often involves compatibility issues such as updating your software to work with a new database system:
// Old database connection var db = require('old-database-lib'); // Updated database connection var db = require('new-database-lib'); db.connect({ host: 'example.com', username: 'user', password: 'password' });
Here, the code was updated to require a new database library and use its connection method, reflecting a change in the database system used.
Perfective Maintenance
When we implement perfective maintenance, it’s often to enhance the user experience or functionality. An example could be adding a new feature to an existing application:
// Adding a new feature to an existing app function addTask(task) { tasks.push(task); } // New feature to remove a task function removeTask(index) { tasks.splice(index, 1); }
The original application only had the ability to add tasks. Now we’ve included a new function to remove a task, enhancing the software without altering its primary purpose.
Preventive Maintenance
Refactoring code is a key part of preventive maintenance. Here’s an example where we’re improving code readability and performance without changing what the code does:
// Before refactoring: code is hard to read and inefficient function processData(data) { var result = []; for (var i = 0; i 10) { result.push(data[i]); } } return result; } // After refactoring: more readable and efficient function processData(data) { return data.filter(item => item > 10); }
By using the array’s filter method, we’ve made the function shorter, cleaner, and more expressive while maintaining its original functionality.
We’ve covered a single example for each type of software maintenance, illustrating how different problems and changes necessitate different solutions. As you encounter various maintenance tasks, you’ll develop an intuition for how to approach each one systematically. Remember, maintenance work is about upholding the quality and resilience of software over time, and these examples are just a starting point to help you build that foundational understanding.
Moving forward with additional code examples will allow us to explore more nuanced scenarios typical of software maintenance and how they’re handled. The goal is to provide you with a comprehensive toolkit of strategies and solutions for maintaining robust software.
Let’s delve into more intricate maintenance tasks, such as optimizing code for performance, accommodating new user requirements, or updating legacy systems to modern standards.
Performance Optimization Example:
// Before Optimization: A non-optimized loop for data processing function processLargeDataSet(dataSet) { for (let i = 0; i < dataSet.length; i++) { // time-consuming processing on data } } // After Optimization: Implementing a web worker to offload processing function processLargeDataSet(dataSet) { const worker = new Worker('data-processor.js'); worker.postMessage(dataSet); worker.onmessage = function (e) { console.log('Processing complete', e.data); } }
In this case, we’ve moved the data processing to a web worker, which prevents blocking the main thread and improves the performance of the application, particularly on large data sets.
User Requirement Change Example:
// Before: A simple, unordered list of users function displayUsers(users) { users.forEach(user => { console.log(user.name); }); } // After: User requirement to display users alphabetically function displayUsers(users) { users.sort((a, b) => a.name.localeCompare(b.name)).forEach(user => { console.log(user.name); }); }
This code snippet updates an existing feature to meet new user requirements, in this case, sorting the users alphabetically before displaying them.
Legacy System Update Example:
// Before: Legacy system using an outdated library var http = require('http'); http.createServer(function (request, response) { response.writeHead(200, {'Content-Type': 'text/plain'}); response.end('Hello World\n'); }).listen(8080); // After: Updating to modern library (e.g., Express.js) const express = require('express'); const app = express(); app.get('/', (req, res) => res.send('Hello World')); app.listen(8080, () => console.log(`App listening on port 8080!`));
Here, we have transitioned from using Node’s basic HTTP module to the more contemporary and feature-rich Express.js framework, which simplifies the creation of web servers and is better supported.
Deprecation Handling Example:
// Before: Using a deprecated method of string concatenation var greeting = "Hello, "; var user = "Jane"; console.log(greeting + user); // After: Utilizing template strings for string concatenation let greeting = "Hello, "; let user = "Jane"; console.log(`${greeting}${user}`);
In this example, we’ve refactored the code to replace the outdated string concatenation with the recommended ES6 template strings.
Security Patch Example:
// Before: Code vulnerable to script injection function setUserName(name) { document.getElementById('user-name').innerHTML = name; } // After: Patching security by safely setting text content function setUserName(name) { document.getElementById('user-name').textContent = name; }
This simple change protects against script injection attacks by properly escaping any HTML that might be inadvertently introduced into the user’s input.
Accessibility Improvement Example:
// Before: A button element without proper accessibility <button onclick="submitForm()">Submit</button> // After: Improved accessibility with ARIA attributes <button onclick="submitForm()" aria-label="Submit form">Submit</button>
By adding an ARIA (Accessible Rich Internet Applications) attribute, the button’s purpose becomes clear to screen readers, thus improving the accessibility of the page.
Software maintenance work is a continual balancing act—keeping the application reliable and up-to-date while simultaneously catering to users’ demands and technological advancements. These additional code examples should give you a taste of the diversity of tasks involved, all of which are crucial in ensuring that a piece of software remains functional, secure, and efficient. Through these illustrations, we hope to foster an appreciation for the meticulous and rewarding nature of maintenance in the software development lifecycle.
Maintaining software is like tuning a complex instrument; each tweak can make significant improvements. Let’s enhance our repertoire with more real-world code examples that address common maintenance scenarios.
Database Schema Migration Example:
// Before: A database column named 'fullname' ALTER TABLE users DROP COLUMN fullname; // After: Splitting 'fullname' into 'firstName' and 'lastName' ALTER TABLE users ADD COLUMN firstName VARCHAR(255); ALTER TABLE users ADD COLUMN lastName VARCHAR(255);
This database schema change accommodates a more structured storage of user names, which can be helpful for sorting, searching, and personalization features.
Interface Abstraction Example:
// Before: Directly using a third-party service function sendEmail(recipient, content) { thirdPartyEmailService.send(recipient, content); } // After: Abstracting the email service behind an interface function sendEmail(recipient, content) { emailService.send(recipient, content); } const emailService = { send: function(recipient, content) { // Implementation can easily be changed without affecting 'sendEmail' thirdPartyEmailService.send(recipient, content); } }
This change insulates the main code from dependencies on a specific third-party service, making it easier to replace or upgrade the email service in the future.
Dependency Update Example:
// Before: Using an old version of a library with known vulnerabilities const library = require('[email protected]'); // After: Updating to a newer, secure version of the library const library = require('[email protected]');
Keeping dependencies up-to-date ensures that your software benefits from the latest security patches and feature improvements.
Internationalization Example:
// Before: Hardcoded English texts function displayGreeting() { console.log('Hello, user!'); } // After: Adding support for internationalization function displayGreeting(language) { const greetings = { en: 'Hello, user!', es: '¡Hola, usuario!', fr: 'Bonjour, utilisateur!' }; console.log(greetings[language] || greetings.en); } // Now, calling displayGreeting('es') outputs: "¡Hola, usuario!"
Adapting software to support multiple languages can significantly widen its user base and make it more accessible.
With these examples, we begin to see the variety and details of continuous software care, how each concern—be it security, performance, or user experience—contributes to a well-rounded and robust application.
These maintenance tasks also highlight a crucial aspect of software engineering—the anticipation of change. Good maintainability is not just about making code easy to understand and modify but also designing software systems that can evolve gracefully as requirements and environments shift.
Whether it’s readying the code for future updates, keeping up with the rapid pace of technology, or enhancing user engagement, every change is an investment in the software’s lifecycle. And remember, a robust suite of automated tests can be developed and maintained alongside your code, helping ensure that changes don’t introduce new errors.
Here’s a glimpse into how test-driven development might influence maintenance:
// Before: A function without tests function add(a, b) { return a + b; } // After: Including unit tests that verify the function's behavior function add(a, b) { return a + b; } // Unit tests using a test framework like Jest test('adds 1 + 2 to equal 3', () => { expect(add(1, 2)).toBe(3); });
When you write or modify code, having a robust set of tests to rely on can significantly increase productivity and reduce the risk of introducing bugs.
Mastery of software maintenance ensures that the applications we build and nurture continue to operate effectively, providing value long after their first release. From the fundamentals to the nuanced examples we’ve shared, maintenance remains an essential skill for any developer aiming to create sustainable, adaptable, and quality software.
Where to Go Next
Your journey in the world of software doesn’t end here. Now that you’ve grasped the fundamentals of software maintenance, it’s an ideal time to expand your skill set and delve deeper into the intricacies of programming. We encourage you to continue exploring and mastering new areas within this vast domain.
If you’re keen on broadening your programming knowledge, our Python Mini-Degree is an excellent next step. Python is a versatile language that opens doors to multiple paths, whether you’re interested in web development, data science, or creating your own games. Our courses are hands-on, allowing you to learn by doing, and you’ll build a portfolio of projects to showcase your skills.
For those looking to explore even more programming opportunities, check out our programming courses. With over 250 courses, from beginner to advanced levels, you’re bound to find the right match for your career goals. Learn at your own pace with quality content designed to turn beginners into professionals. Join Zenva Academy’s community of learners and start transforming your passion into tangible, marketable skills.
Conclusion
Software maintenance is an often underappreciated yet vital facet of the development process. It breathes longevity into applications and ensures they adapt and grow in alignment with user needs and technological advancements. As a developer, enhancing your maintenance skills means striving for excellence in your craft and contributing to the technological world with sustainable and efficient software. But don’t stop here—continue to build, learn, and refine.
Join us at Zenva Academy, where we foster the continuous growth of developers like you. With our comprehensive, project-based Python Mini-Degree, we’ll help you take the next big step in your career. Whether you’re beginning your journey or scaling new heights, we’re excited to be part of your learning adventure. Let’s write the future of code together, one line at a time.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
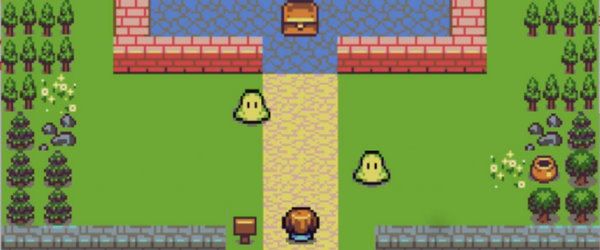
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.