Welcome to the fascinating world of object serialization, where data transforms into a format that can be stored or transmitted and then resurrected back to its original state. This concept is a cornerstone in the development of applications that rely on data persistence, communication, or simply the process of turning complex data structures into a portable and manageable format. As you read through this tutorial, you’ll discover the magic behind this process and how it can serve as a powerful tool in your programming arsenal.
Table of contents
What is Object Serialization?
At its core, object serialization is the process of converting an object’s state into a format that can be stored or transferred. This conversion allows complex data structures to be saved to files, sent over a network, or stored in a database, ensuring that the intricacies of the data are preserved and can be recreated later.
What is it Used For?
Serialization finds its utility in various domains such as saving game states, caching, session storage, and communication between different processes or over the network. Anytime you need to persist the state of your objects beyond the lifetime of the application’s process, serialization comes into play.
Why Should I Learn It?
Understanding object serialization is vital for several reasons:
- It allows for easy file I/O operations, providing a seamless way to save and restore application states.
- It facilitates data exchange, particularly in web services and APIs where data often travels in serialized formats like JSON or XML.
- It’s a crucial step in developing distributed applications where objects need to be transmitted between different parts of the system.
By mastering serialization, you’ll be able to build more robust, flexible and scalable applications that can handle data efficiently, making you a more competent and versatile programmer in the process.
Basic Serialization in Python
Python offers built-in support for serialization through the pickle module. Let’s begin by serializing a simple dictionary object.
import pickle # Here is our dictionary object game_data = { 'player_position': '00,04', 'items_collected': ['sword', 'potion'], 'level': 'forest' } # Now, let's serialize this dictionary into a string using pickle.dumps serialized_data = pickle.dumps(game_data) print(serialized_data) # We can also write the serialized data to a file with open('game_data.pkl', 'wb') as file: file.write(serialized_data)
Once we have serialized our object, the next step is to deserialize it, meaning to recreate the original object from the serialized data.
# Reading the serialized data from the file with open('game_data.pkl', 'rb') as file: loaded_data = file.read() # Deserializing the data to its original form deserialized_data = pickle.loads(loaded_data) print(deserialized_data)
Serialization with JSON
JSON (JavaScript Object Notation) is a common format for serializing and transmitting structured data. Python provides the json module for this purpose.
import json # The original dictionary player_stats = {'name': 'Athena', 'strength': 75, 'health': 100, 'inventory': ['sword', 'shield']} # Serializing to JSON using json.dumps json_data = json.dumps(player_stats) print(json_data) # Saving the JSON data to a file with open('player_stats.json', 'w') as file: json.dump(player_stats, file)
Just as with pickle, we can easily turn the JSON string back into a Python object using the json.load() and json.loads() functions.
# Loading JSON data from a file with open('player_stats.json', 'r') as file: loaded_player_stats = json.load(file) print(loaded_player_stats) # Alternatively, turning a JSON string back into a dictionary json_str = '{"name": "Athena", "strength": 75, "health": 100, "inventory": ["sword", "shield"]}' stats_from_str = json.loads(json_str) print(stats_from_str)
Serialization in JavaScript
Moving onto JavaScript, serialization is typically done directly into JSON using the JSON.stringify and JSON.parse functions. Here’s a basic example:
const player = { name: 'Luna', level: 30, equipment: ['bow', 'quiver', 'boots'] }; // Serializing the object const serializedPlayer = JSON.stringify(player); console.log(serializedPlayer); // Deserializing the JSON string const deserializedPlayer = JSON.parse(serializedPlayer); console.log(deserializedPlayer);
As you can see, in JavaScript, the built-in JSON object makes serialization and deserialization a straightforward task.
Custom Object Serialization
Occasionally, you may encounter objects that don’t serialize smoothly, such as a custom class instance in Python. In such cases, you need to define a method for turning the object into a serializable format.
import pickle class Player: def __init__(self, name, level): self.name = name self.level = level def __str__(self): return f'Player {self.name}, Level {self.level}' # Serialization function def serialize_player(obj): if isinstance(obj, Player): return {'name': obj.name, 'level': obj.level} raise TypeError('Type not serializable') player_instance = Player('Artemis', 42) # Serialize custom object serialized_player = pickle.dumps(player_instance, default=serialize_player) print(serialized_player) # Save to file with open('player_instance.pkl', 'wb') as file: file.write(serialized_player)
Deserialization of custom objects would similarly require a function that knows how to handle the data and recreate the original object. This function would be passed as a parameter to the loading function.
Summary
In this part, we covered the basics of serialization using different programming languages and techniques. These included Python’s pickle and json modules, JavaScript’s JSON object, and how to tackle serialization of custom objects. With these examples, you now have the foundational knowledge to start integrating serialization into your own applications.
Stay tuned for the next part where we will delve more deeply into advanced serialization techniques and look at some real-world use cases. Happy coding!
Serialization is not limited to simple data structures. When working with more complex scenarios and custom object hierarchies, handling serialization effectively becomes even more crucial. Let’s dive into some examples that can broaden your understanding and equip you for these situations.
Advanced Serialization Techniques
Custom serialization can often require special handling. Imagine you have a Python class with attributes that aren’t directly serializable, like a file handle or a connection. You’ll need to define custom __getstate__ and __setstate__ methods to manage these.
import pickle class GameConnection: def __init__(self, address): self.connection = self._create_connection(address) def _create_connection(self, address): print(f"Simulating opening connection to {address}") return "connection-object" def __getstate__(self): # Return the state to be pickled, omitting the non-serializable connection attribute state = self.__dict__.copy() del state['connection'] return state def __setstate__(self, state): # Restore instance attributes self.__dict__.update(state) self.connection = self._create_connection("restored-address") # Instantiate and serialize the GameConnection object game_conn = GameConnection('127.0.0.1') serialized_conn = pickle.dumps(game_conn) # Deserializing restores the object, reinitializing the connection restored_game_conn = pickle.loads(serialized_conn) print(restored_game_conn)
When dealing with JSON serialization, you may need to serialize complex objects that JSON does not inherently know how to handle. We often use the default parameter in the json.dump or json.dumps function to specify a method that will return an appropriate representation for complex types.
import json class Player: def __init__(self, id, name, items=None): self.id = id self.name = name self.items = items if items is not None else [] def to_json(self): # Custom method to convert to JSON-serializable type return {'id': self.id, 'name': self.name, 'items': self.items} player = Player(1, 'Cerberus', ['Sword', 'Shield']) # Serialization using a custom method player_json = json.dumps(player, default=lambda o: o.to_json()) print(player_json)
Let’s also consider the problem in JavaScript, where we might want to deal with classes that have methods. When serializing such objects, we’d typically want to preserve only the data attributes.
class Player { constructor(id, name, items) { this.id = id; this.name = name; this.items = items || []; } addItem(item) { this.items.push(item); } toJSON() { return { id: this.id, name: this.name, items: this.items }; } } const player = new Player(2, 'Echidna', ['Bow', 'Arrow']); const serializedPlayer = JSON.stringify(player); console.log(serializedPlayer); const deserializedPlayer = JSON.parse(serializedPlayer); console.log(deserializedPlayer);
In some cases, you might even need to serialize data that includes circular references. Most serialization tools will fail or throw errors in these situations. However, certain libraries handle these gracefully. For example, JSON.stringify in JavaScript can accept a replacer function that helps manage circular references.
const person = { name: "Gaea" }; // Adding a circular reference person.self = person; const safeSerialize = (obj) => { const seen = new WeakSet(); return JSON.stringify(obj, (key, value) => { if (typeof value === "object" && value !== null) { if (seen.has(value)) { return; // Duplicate reference found, discard key } seen.add(value); } return value; }); }; console.log(safeSerialize(person));
Dealing with Binary Formats
When performance is key, especially in games or high-speed network communications, you might opt for binary serialization formats. Binary formats often result in smaller files and faster processing but lose the readability of textual formats like JSON or XML. Python’s pickle module, for instance, can be used for binary serialization as we’ve seen earlier.
# Python's module 'struct' can also handle binary data import struct # Packing data as binary binary_data = struct.pack('i6sf', 23, b'Echidna', 3.14) print(binary_data) # Unpacking the binary data unpacked_data = struct.unpack('i6sf', binary_data) print(unpacked_data)
Serialization is a fundamental concept in modern programming, bridging the gap between volatile memory states and persistent storage, whether that’s a file, a database, or across a network connection. By incorporating the patterns and techniques showcased in these examples, you’re well-equipped to tackle a breadth of serialization challenges in your future projects.
Always remember: the more you practice and play with serialization, the more intuitive it will become. So, go ahead and serialize the world, or at least, all the objects in your digital one. Happy coding!
Delving deeper into serialization, let’s explore how custom serialization can be essential for preserving the integrity and functionality of complex data structures. We’ll examine various scenarios where serialization goes beyond the basics, including custom class hierarchies, serialization of objects containing other objects, and handling different versions of objects.
In Python, serialization of nested objects is common. For instance, if you have a class that contains instances of other classes, Python’s pickle can handle this smoothly because it serializes the entire object graph. Here’s an example:
import pickle class Item: def __init__(self, name): self.name = name class Player: def __init__(self, name, items): self.name = name self.inventory = items # Nested objects sword = Item('Excalibur') shield = Item('Aegis') player = Player('Athena', [sword, shield]) # Serialize the Player object, which includes Item objects with open('player.pkl', 'wb') as file: pickle.dump(player, file) # Deserialize the Player object with open('player.pkl', 'rb') as file: loaded_player = pickle.load(file) print(loaded_player) print(loaded_player.inventory[0].name) # Outputs: Excalibur
Consider versioning in serialization. If your object’s structure changes over time (like during software updates), you’ll need a way to handle older serialized objects.
class PlayerV2: def __init__(self, name, items, level): self.name = name self.inventory = items self.level = level def __setstate__(self, state): self.__dict__ = state # Provide a default for "level" if it's not in the serialized data (backward compatibility) self.__dict__.setdefault('level', 1) # Simulate loading an old object without the 'level' attribute serialized_old_player = pickle.dumps(Player('Odysseus', [Item('Olive Branch')])) new_player = pickle.loads(serialized_old_player, fix_imports=True) print(new_player.level) # Outputs: 1 (default level for old object)
When you are dealing with custom serialization in JSON, you might need to serialize a class hierarchy where subclasses extend a base class.
import json class BaseEnemy: def to_json(self): return json.dumps({'type': self.__class__.__name__}) class Orc(BaseEnemy): def __init__(self, strength): self.strength = strength def to_json(self): base_json = super().to_json() orc_dict = json.loads(base_json) orc_dict.update({'strength': self.strength}) return json.dumps(orc_dict) orc = Orc(10) print(orc.to_json())
In environments like .NET or Java, which are strongly-typed, deserializing into the correct type is vital. These languages have built-in mechanisms to preserve type information. For example, in C#, you work with BinaryFormatter, DataContractSerializer, or other serialization frameworks which handle complex scenarios.
Here’s an example using the Python module jsonpickle to handle more complex JSON serialization that preserves class type information:
import jsonpickle class Wizard: def __init__(self, name, spells): self.name = name self.spells = spells wizard = Wizard('Merlin', ['Invisibility', 'Teleport']) # Serialize using jsonpickle, which preserves the object's class information serialized_wizard = jsonpickle.encode(wizard) print(serialized_wizard) # Deserialize and maintain the object’s type deserialized_wizard = jsonpickle.decode(serialized_wizard) print(deserialized_wizard) print(type(deserialized_wizard)) # Outputs:
Binary serialization often requires dealing directly with byte arrays. For high-performance and space-efficient serialization in Python, we might use the array module alongside pickle.
import pickle import array # Using the 'array' module for efficient numeric storage int_array = array.array('i', [1, 2, 3, 4, 5]) # Serialize the array to a byte stream serialized_array = pickle.dumps(int_array) print(serialized_array) # Deserialize back into an 'array' object deserialized_array = pickle.loads(serialized_array) print(deserialized_array.tolist()) # Outputs: [1, 2, 3, 4, 5]
In conclusion, serialization can be complex, but it remains a powerful feature that can be tailored to fit your data persistence and transfer needs. By understanding and leveraging these concepts, you can serialize almost any type of object, regardless of complexity, ensuring the ability to maintain state across sessions and platforms.
Remember, practicing with real-world examples like these can solidify your understanding of serialization, and help you create robust, efficient, and maintainable code. Get creative with serialization in your projects, and happy coding!
Furthering Your Coding Journey
Embarking on the journey of programming is exciting, and there’s always so much more to learn and explore. If you’ve enjoyed delving into the world of object serialization and want to broaden your horizons in the versatile and powerful Python language, our Python Mini-Degree is the perfect next step.
Designed to take you from beginner to professional, our curriculum covers a range of topics from the basics to more complex applications such as game development, app creation, and much more. It’s a treasure trove of knowledge for curious minds who aim to create real-world applications and games. You can work at your own pace with our flexible learning schedule and materials, making it ideal for busy lives and varying commitments.
In addition to the Python Mini-Degree, our comprehensive Programming courses offer an even broader collection to satisfy your learning needs. With over 250 courses available, Zenva equips you to tackle new challenges head-on, create innovative projects, and continually grow as a developer. Join our community of over a million learners and developers today and take the next big leap in your coding career.
Conclusion
As we’ve ventured through the intricacies of object serialization, it’s clear that this knowledge is indispensable for developers looking to preserve the state of their applications across sessions, or exchange data between different components and services. Whether you’re saving game states, implementing session storage, or developing complex, distributed systems, serialization is a tool that will serve you time and again. Remember, mastering the art of serialization with Zenva’s Python Mini-Degree is just the beginning of your potential as a coder. The avenues to apply these skills are limitless, and each line of code you write is another step towards realizing your vision.
We at Zenva are committed to providing you with the best learning experience to help you achieve your dreams in technology. Continue to challenge yourself, expand your knowledge, and create with confidence by engaging with our wide range of courses. Your journey is unique, and we look forward to being a part of it every step of the way—happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
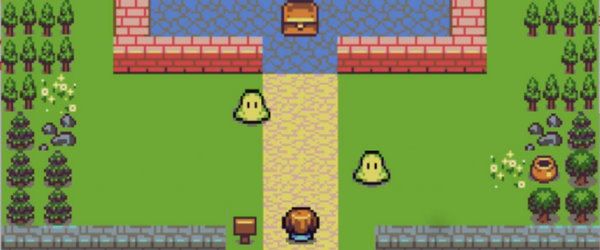
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.