Welcome to the fascinating world of programming constants! As you embark on this coding adventure, you’ll uncover the secrets that help transform scattered code into structured and robust programs. Constant values are one of the cornerstones in the architecture of programming. Understanding them not only deepens your knowledge of coding fundamentals but also equips you with the tools to write more reliable and maintainable software.
Table of contents
What Are Programming Constants?
Constants, as the name suggests, are values in your code that do not change throughout the execution of a program. They are the steadfast rules in the dynamic realm of programming, providing a reliable point of reference amidst the fluid state of variables and data manipulation. In real-world terms, think of a constant as the unchanging rules of a board game or the fixed dimensions of a soccer field.
What Are Constants Used For?
Constants serve a range of purposes, from enhancing the readability of your code to preventing inadvertent changes to critical values. By using constants, programmers can create a clearer and more meaningful context for those ‘magic numbers’ and ‘hardcoded strings’ that often litter a codebase.
Why Should I Learn About Constants?
Embracing constants is a step towards becoming a more disciplined and skilled programmer. They help you to:
– Avoid mistakes caused by the accidental modification of values.
– Make your code more understandable for others (and your future self).
– Facilitate changes and maintenance, as you need to update a constant only in one location rather than hunting down multiple instances throughout your code.
So, with the basics laid out, let’s dive in and explore the immutable world of programming constants through practical examples and everyday analogies. Whether you’re a beginner taking your first steps or an experienced coder looking to brush up on best practices, there’s something here for everyone.
Declaring Constants in Different Programming Languages
In this section, we’ll explore how constants are declared in various popular programming languages. This will give you a practical and applicable understanding of constants across the programming spectrum.
Declaring Constants in JavaScript
In JavaScript, constants are declared using the const keyword. Once set, their value cannot be reassigned.
const PI = 3.14159; console.log(PI); // Outputs: 3.14159 // Attempting to reassign the value of PI will result in an error. // PI = 3.15; // Uncaught TypeError: Assignment to constant variable.
JavaScript enforces the immutability of the constant on a reference level, meaning if the constant is an object or array, the object’s or array’s contents can still be modified.
const COLORS = ['red', 'green', 'blue']; COLORS.push('yellow'); console.log(COLORS); // Outputs: ['red', 'green', 'blue', 'yellow'] // The array itself can't be reassigned though. // COLORS = ['orange', 'pink']; // Uncaught TypeError: Assignment to constant variable.
Declaring Constants in Python
Python does not have a built-in constant type, but it’s a common convention to use all uppercase letters to indicate that a variable should be treated as a constant.
PI = 3.14159 print(PI) # Outputs: 3.14159 # Technically, PI can still be reassigned, but by convention it should not be changed.
Other tools and modules, like enum, can be used to create constants in Python.
from enum import Enum class Colors(Enum): RED = 1 GREEN = 2 BLUE = 3 print(Colors.RED) # Outputs: Colors.RED
Declaring Constants in C++
In C++, the const keyword declares constants. C++ constants must be initialized at the time of their creation.
const double Pi = 3.14159; std::cout << Pi; // Outputs: 3.14159 // Pi = 3.15; // Error: assignment of read-only variable ‘Pi’
For larger projects, C++ also offers ways to define constants in header files for shared usage across multiple files.
// constants.h #ifndef CONSTANTS_H #define CONSTANTS_H namespace Constants { const double Pi = 3.14159; } #endif // main.cpp #include "constants.h" #include <iostream> int main() { std::cout << Constants::Pi; // Outputs: 3.14159 return 0; }
Declaring Constants in Java
Java uses the final keyword to create constants, often combining it with static to create class-level constants.
public class Circle { public static final double PI = 3.14159; public static void main(String[] args) { System.out.println(Circle.PI); // Outputs: 3.14159 } }
Java constants are widely used for defining configuration values, error codes, or fixed application-level data.
public class HttpStatusCodes { public static final int OK = 200; public static final int NOT_FOUND = 404; // ... other status codes }
By now, you should have a solid understanding of constants across different programming languages. These examples are just the beginning, entrusting you with the knowledge of immutable values in code. Our next installment will continue to examine constants with even more coding scenarios, including their application in conditional statements, functions, and complex data structures.
Constants are not just used for primitive data types like integers and strings, but they also play a crucial role in managing application states, configurations, and even complex data structures. Let’s continue our journey with more code examples that showcase the various applications of constants in programming.
Starting with C, you might use macro definitions to create constants with the #define preprocessor directive:
#include <stdio.h> #define MAX_USERS 100 int main() { printf("The maximum number of users is %d.\n", MAX_USERS); return 0; }
In the example above, MAX_USERS acts as a constant representing the maximum number of users allowed. This type of constant is useful for defining limits or sizes that are used across the program.
Moving onto Ruby, constants are declared with an uppercase first letter, and by convention, they should be fully uppercase for full constants:
PI = 3.14159 puts PI # Outputs: 3.14159 class Circle RADIUS = 5 def self.area PI * RADIUS**2 end end puts Circle.area # Outputs: 78.53975
The value of RADIUS inside the Circle class is set as a constant because the radius of a particular circle is not expected to change.
In Swift, you use the let keyword to declare constants, which plays a critical role in managing state in iOS applications:
let maxLoginAttempts = 3 // Attempting to reassign maxLoginAttempts will result in a compiler error. // maxLoginAttempts = 5 // Error: Cannot assign to value: 'maxLoginAttempts' is a 'let' constant
This pattern is prevalent in the design of apps, where a particular value must remain consistent throughout the lifetime of the application.
Here is how constants can be useful in a SQL database procedure or function within flavors like PL/SQL:
CREATE OR REPLACE FUNCTION calculate_area(radius CONSTANT REAL) RETURNS REAL AS $$ DECLARE pi CONSTANT REAL := 3.14159; BEGIN RETURN pi * radius^2; END; $$ LANGUAGE plpgsql;
In this PL/SQL function, pi is defined as a constant to ensure that the calculation uses the same value for pi throughout the function.
Furthermore, constants can also be used in the context of conditional statements and loops. Consider the use of constants in a loop written in Go:
package main import "fmt" const NumIterations = 5 func main() { for i := 0; i < NumIterations; i++ { fmt.Printf("Iteration %d\n", i+1) } }
The constant NumIterations is used to control the loop iterations. This is helpful if the number of iterations is referenced in multiple places or may change in the future.
Lastly, in environments such as .NET, static read-only fields can be used when the constant value may not be known at compile time:
public class Config { public static readonly string ApiEndpoint; static Config() { ApiEndpoint = // Value potentially loaded from a configuration file or environment variable } } // Usage: Console.WriteLine(Config.ApiEndpoint);
Here, ApiEndpoint is set once at runtime, and then it behaves as a constant during the lifecycle of the application. Utilizing static read-only fields in this way is a good practice when dealing with configuration data that shouldn’t change after it’s initially loaded.
As you can see, constants are a versatile tool in a programmer’s toolbox, with applications ranging from simple data type storage to managing configuration and state in complex applications. By mastering the use of constants, you take another step towards writing clean, maintainable, and error-resistant code. Stay tuned as we delve even deeper into more advanced programming concepts that will further hone your development skills!
Building upon our exploration of constants, let’s delve into more sophisticated examples. These cases highlight the roles constants can take on within larger systems and frameworks, showcasing how versatile and indeed indispensable they are in software development.
In Node.js, you might require a set of configuration constants that are exported from a module, forming the backbone of your application’s settings:
// config.js module.exports = Object.freeze({ PORT: 3000, DB_URI: 'mongodb://localhost:27017/myapp', SECRET_KEY: 'your-secret-key' }); // app.js const config = require('./config'); console.log(`Server will run on port: ${config.PORT}`);
The use of Object.freeze ensures that the configuration object cannot be altered, effectively making each property a constant.
Within the Android development environment, constants can define Intent request codes that are used across different components of the app:
public class MainActivity extends AppCompatActivity { private static final int PICK_CONTACT_REQUEST = 1; public void pickContact() { Intent pickContactIntent = new Intent(Intent.ACTION_PICK, Uri.parse("content://contacts")); startActivityForResult(pickContactIntent, PICK_CONTACT_REQUEST); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { if (requestCode == PICK_CONTACT_REQUEST) { // Handle contact pick result } } }
The constant PICK_CONTACT_REQUEST is a unique identifier for the intent used to select a contact, ensuring that the correct result is processed in the onActivityResult method.
When dealing with animation or game development, constants can regulate frame rates or define game physics. Below is an example in Unity using C#:
using UnityEngine; public class GameConstants : MonoBehaviour { public const float PlayerSpeed = 5.0f; public const int MaxLives = 3; void Update() { // Using the constant PlayerSpeed in a calculation for movement float moveDistance = PlayerSpeed * Time.deltaTime; transform.Translate(0, 0, moveDistance); } }
The PlayerSpeed constant is used within the Update method to calculate and apply player movement consistently every frame.
Within web development, constants may also define HTML elements or CSS selectors that JavaScript interacts with. Here’s an example using jQuery:
// Defining a constant for a frequently accessed DOM element const $loginForm = $('#loginForm'); $loginForm.on('submit', function(event) { event.preventDefault(); // Process form submission });
The constant $loginForm prevents repeated querying of the DOM for the login form element, optimizing performance.
In server-side scripting with PHP, constants often configure database connections or store application paths:
// db_config.php define('DB_HOST', 'localhost'); define('DB_USER', 'root'); define('DB_PASS', 'password'); define('DB_NAME', 'my_database'); // Using the constants to create a database connection in another file $db = new mysqli(DB_HOST, DB_USER, DB_PASS, DB_NAME);
The database configuration constants DB_HOST, DB_USER, DB_PASS, and DB_NAME are defined once and used wherever a database connection is required.
Finally, constants can dictate environment states or modes, influencing how an application behaves at runtime. An example in a Flask Python web application might look like this:
# app.py from flask import Flask import os app = Flask(__name__) # Setting a constant for the environment mode ENV_MODE = os.getenv('FLASK_ENV', 'development') if ENV_MODE == 'development': app.debug = True app.config['TESTING'] = True @app.route('/') def index(): return "Hello, World!" if __name__ == '__main__': app.run()
The ENV_MODE constant determines whether the Flask application is running in development mode, potentially setting various flags to adjust its behavior for debugging and testing.
Through these varied examples across different languages and frameworks, you’ve seen how constants fortify code by providing a secure and unchanging reference point. They encapsulate values which are integral to the operation and configuration of programs, underscoring their value to programmers and the importance of understanding their correct implementation. With this knowledge, you are better prepared to create software that is robust, reliable, and easier to maintain.
Continuing Your Programming Journey
Embarking on the path to becoming a seasoned programmer means continuously learning and refining your skills. If you’ve enjoyed this exploration of programming concepts, such as constants, and are eager to deepen your knowledge, we invite you to take the next step with our Python Mini-Degree. This program offers an all-encompassing learning experience that covers the essentials of Python, one of the world’s most popular and versatile programming languages.
Whether you’re a total beginner or looking to build upon existing skills, our Python Mini-Degree is a powerful way to expand your expertise. The curriculum is project-based, enabling you to apply what you’ve learned immediately and create tangible outcomes such as games and applications. Moreover, you’ll join a community of learners and have access to a wealth of resources to support your growth.
For those who wish to explore a broader horizon, our collection of Programming Courses offers valuable insights into various programming languages and technologies. With Zenva, you can confidently build a strong foundation and climb the ladder of programming proficiency, ultimately opening doors to new career opportunities and expanding your creative horizon.
Conclusion
Understanding and utilizing constants is just the beginning of your programming adventure. By mastering such fundamental concepts, you pave the way toward creating robust, efficient, and maintainable code across any project. With Zenva’s Python Mini-Degree, this journey continues, unlocking the full potential of your programming prowess and preparing you to tackle real-world challenges.
Dive into Zenva’s comprehensive courses, where each lesson brings you one step closer to becoming the developer you aspire to be. Build a diverse portfolio, strengthen your skill set, and let learning with us be the constant in your ever-evolving story of programming success.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
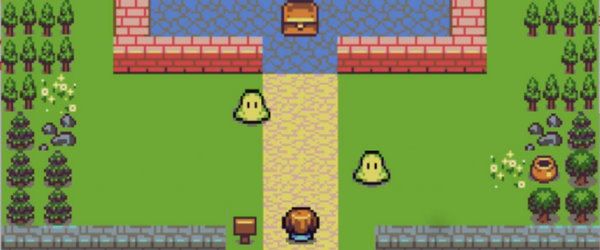
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.