Delving into the realm of programming, a fundamental concept that enables the creation of interactive and dynamic software is “variables.” These are the building blocks that store information; think of them as containers in which we can place data that we wish to keep track of, such as a player’s score in a game or the number of lives left. Equipped with a clear understanding of variables, you can step into a world where your programs respond to user inputs, evolve, and ultimately come to life.
What truly gives variables their power is their versatility and ubiquity in programming. No matter where your coding adventures may lead you, from the simplest scripts to the most complex systems, the knowledge of variables will be a cornerstone of your journey. So, buckle up as we explore the exciting uses and the vital importance of variables in the craft of programming.
Table of contents
What is a Variable?
In simplest terms, a variable is a name given to a storage location in the computer’s memory. Like a labeled box where you can stash an item and retrieve it later, a variable holds our data until we need it.
– They can contain different types of data, such as numbers, text, or even lists.
– Variables can be changed and manipulated through our code, hence the name ‘variable’ – the data they store can vary.
What are Variables Used For?
Variables serve a plethora of functions within programming:
– They act as placeholders for values that can change as our programs run.
– They help us keep track of information and manipulate it to perform operations, make decisions, and control the flow of the program.
Consider a role-playing game where a hero collects coins. We’d use a variable to keep tally of that coin count, which alters as the hero journeys through the game.
Why Should I Learn About Variables?
Learning about variables is paramount:
– As the foundation of programming concepts, understanding variables is crucial for becoming fluent in any programming language.
– They allow for the creation of adaptable code that can handle a variety of inputs and scenarios.
With variables, your code isn’t just a static set of instructions; instead, it becomes a dynamic and interactive experience. Let’s dive into coding with variables to see just how they empower your programs to interact with real-world data.
Creating and Declaring Variables
Let’s start by learning how to create a variable. In programming, we often refer to this process as ‘declaring’ a variable. In many languages, this is done by specifying a type for your variable, which tells the compiler or interpreter what kind of data it can hold.
// In JavaScript, declaring a variable: var score; // In Python, declaring a variable: score = 0
Notice that in JavaScript, we use the keyword `var` to declare a variable, whereas in Python, we simply assign a value to create a variable, as Python is a dynamically typed language.
Now, let’s assign some values to our variables.
// Assigning a value to the previously declared variable in JavaScript: score = 10; // In Python, we declare and assign in the same line: score = 10
Variables can also be declared and assigned a value in one line, which is a common practice.
// Declaring and assigning a variable in one line in JavaScript: var lives = 3; // The same process in Python: lives = 3
Using Variables in Calculations
Variables can be used to perform calculations. This is incredibly useful for anything from simple arithmetic to complex computational algorithms.
Let’s see some basic examples involving arithmetic operations.
// Adding to a variable in JavaScript: var score = 10; score = score + 5; // score is now 15 // In Python, the same operation would look like this: score = 10 score += 5 # score is now 15
Subtraction works in a similar way:
// Subtracting from a variable in JavaScript: var lives = 3; lives = lives - 1; // lives is now 2 // In Python: lives = 3 lives -= 1 # lives is now 2
And we can use variables to hold results of calculations:
// Calculating and storing the result in JavaScript: var totalScore = score + lives * 10; // Python: totalScore = score + lives * 10
The order of operations, as one would expect, applies here too — multiplication is performed before addition.
Updating Variables
Variables are not just placeholders for static information; they can be updated and modified as needed. This allows us to create more dynamic and interactive programs.
Here’s an example of incrementing a score for each task completed in a game.
// Incrementing a value in JavaScript: var tasksCompleted = 0; tasksCompleted++; // tasksCompleted is now 1 // Python has a similar syntax: tasksCompleted = 0 tasksCompleted += 1 # tasksCompleted is now 1
Sometimes we need to concatenate strings or combine a string with variables. This is how we can create a dynamic message:
// Concatenating strings with variables in JavaScript: var playerName = 'Arya'; var greeting = 'Hello, ' + playerName + '! Welcome back to the game.'; // In Python, using f-strings for formatting: playerName = 'Arya' greeting = f'Hello, {playerName}! Welcome back to the game.'
Variable Naming Conventions
Lastly, it’s important to mention that we should follow certain conventions when naming our variables. This helps to ensure that our code is readable and understandable not just to us but to anyone else who might read it.
– Use descriptive names: `playerScore` is better than just `p`.
– Start with a letter, not a number, and don’t use spaces.
– Follow the specific guidelines for the language, like camelCase in JavaScript or snake_case in Python.
var playerScore = 0; // Descriptive and in camelCase for JavaScript player_score = 0 // Descriptive and in snake_case for Python
In the next part of our tutorial, we’ll take a deeper look into different data types that variables can hold and why choosing the correct type matters for our programs.Variables aren’t just restricted to holding singular values like numbers or strings. They can also reference more complex structures, such as Arrays or Objects in JavaScript, and Lists or Dictionaries in Python. These structures allow for the storage and manipulation of collections of data.
Let’s look at how we can utilize variables with these more complex data types.
Working with Arrays and Lists
Arrays in JavaScript and lists in Python are used to store ordered collections of items, which could be numbers, strings, or even other arrays/lists.
// Creating an array in JavaScript: var playerScores = [500, 600, 700]; // Accessing an element from the array: var highScore = playerScores[0]; // highScore is now 500 // Adding an element to the end of the array: playerScores.push(800);
In Python, we use lists, which work in a very similar way:
# Creating a list in Python: player_scores = [500, 600, 700] # Accessing an element from the list: high_score = player_scores[0] # high_score is now 500 # Adding an element to the end of the list: player_scores.append(800)
Both structures can also be modified at specific indexes:
// Updating an element in an array in JavaScript: playerScores[0] = 750; // Python: player_scores[0] = 750
Understanding Objects and Dictionaries
Objects in JavaScript and dictionaries in Python can store data in key-value pairs, providing a way to group related data together.
Here’s an example in JavaScript:
// Creating an object in JavaScript: var player = { name: "Arya", score: 1000, alive: true }; // Accessing a property from the object: var playerName = player.name; // playerName is now "Arya" // Modifying a property: player.score = 1200;
And in Python, we have dictionaries:
# Creating a dictionary in Python: player = { 'name': 'Arya', 'score': 1000, 'alive': True } # Accessing a value using a key: player_name = player['name'] # player_name is now 'Arya' # Modifying a key's value: player['score'] = 1200
These structures are immensely powerful for organizing and storing complex data.
Dynamic Typing and Type Casting
JavaScript and Python are dynamically typed languages, which means a variable can be reassigned to a different type.
// JavaScript: var item = "sword"; item = 500; // No error, item is now a number // Python: item = "shield" item = 250 # No error, item is now an integer
Sometimes, you need to convert from one type to another—this is called type casting.
// Converting a number to a string in JavaScript: var score = 500; var scoreString = String(score); // Python: score = 600 score_string = str(score)
On other occasions, you might need to convert a string to a number.
// JavaScript: var livesString = "3"; var lives = Number(livesString); // Python: lives_string = '3' lives = int(lives_string)
Constants and Immutable Variables
Both JavaScript and Python also allow for the declaration of constants or immutable variables. These are variables that, once set, cannot be reassigned.
// Declaring a constant in JavaScript: const MAX_PLAYERS = 4; // Python (using a naming convention as Python doesn't have 'const'): MAX_PLAYERS = 4
Attempting to reassign a `const` in JavaScript will result in an error, encouraging more predictable code.
Understanding the differences between regular variables and constants is crucial in a coding environment where certain values must remain unchanged throughout the life of a program.
With these examples, we can see that by mastering variables, arrays/lists, objects/dictionaries, and the principles of dynamic typing and constants, we equip ourselves to tackle a wide array of programming challenges. These fundamentals form the backbone of the innovative applications and exciting games we encounter in the digital world.Understanding variable scope is crucial when it comes to programming. Scope defines where variables can be accessed or referenced within our code. Having good command over variable scope helps prevent errors and bugs related to variable access.
Let’s elaborate on the concept with examples.
Global vs. Local Scope
Variables declared outside any function or block are known as global variables and can be accessed from anywhere in the code. Conversely, variables declared within a function are local and can only be accessed within that function.
Here’s a JavaScript example demonstrating global scope:
// A global variable in JavaScript var playerHealth = 100; function takeDamage(damage) { playerHealth -= damage; } takeDamage(10); console.log(playerHealth); // Outputs: 90
Now, a Python example showing local scope:
# A function with a local variable in Python def setHighScore(score): highScore = score # local variable setHighScore(5000) # print(highScore) would raise an error as highScore is not accessible here
Understanding Block Scope
JavaScript introduced `let` and `const` to handle block scoping within `{}` brackets, such as those in conditionals and loops.
// Block scoping with let in JavaScript if (true) { let secret = "I'm block scoped!"; } // console.log(secret); // This will raise an error
Python has no block scope, and variables inside loops or if statements are accessible outside of the block, bearing solely function or global scope.
# Python lacks block scope if True: not_a_secret = "I'm not block scoped!" print(not_a_secret) # No error, would output the string
Hoisting in JavaScript
JavaScript hoists variable declarations to the top of the function or the global context. This means you can refer to a variable before it’s been declared. However, this is not recommended as it can lead to confusion.
// Example of hoisting in JavaScript console.log(toBeHoisted); // Outputs: undefined var toBeHoisted = "I'm hoisted!";
Variables declared with `let` and `const` are also hoisted, but you cannot access them before the actual declaration line (they are in a “temporal dead zone”).
// This will result in a ReferenceError console.log(toBeHoistedLet); // ReferenceError: Cannot access 'toBeHoistedLet' before initialization let toBeHoistedLet = "I'm hoisted differently!";
In Python, there is no concept of hoisting, and attempting to access a variable before declaration will result in a `NameError`.
# Trying to access a variable before declaration in Python will raise an error print(not_declared_yet) # NameError not_declared_yet = "Now I'm declared"
Closures and Encapsulating Variables
A closure occurs when a function remembers and continues to access variables from the scope in which it was declared, even after that scope has finished executing.
JavaScript closures are a powerful feature:
// A closure in JavaScript function createGreet(greeting) { return function(name) { console.log(greeting + ', ' + name + '!'); }; } var greetHello = createGreet('Hello'); greetHello('Arya'); // Outputs: Hello, Arya!
Python functions can also create closures, capturing variables from their outer enclosing scopes.
# A closure in Python def create_greet(greeting): def greeter(name): print(f"{greeting}, {name}!") return greeter greet_hello = create_greet("Hello") greet_hello("Arya") # Outputs: Hello, Arya!
By employing what we’ve covered so far, we harness the full power of variables and the flexible ways we can store and access data across different parts of our code. This not only aids in creating robust logic but also enhances code maintainability and readability, leading to better programming practices overall.
Continue Your Programming Journey with Zenva
Embarking on your coding journey opens a world of possibilities, and mastering the essentials, like variables, sets you on the path to success. But why stop there? With programming, there’s always more to discover, more to create, and more ways to grow your skills. It’s crucial to continue learning and practicing, and at Zenva, we have the perfect way to help you do just that.
Our comprehensive Python Mini-Degree is an ideal next step for those eager to dive deeper into programming. This curriculum provides an extensive range of courses that cover a broad spectrum of Python applications, including game and app development. Python is not only intuitive and beginner-friendly, but also a powerful tool used across many cutting-edge fields.
Furthermore, for those looking to broaden their programming horizons even more, we offer a diverse selection of Programming Courses, suitable for all skill levels. Whether you’ve just started learning the basics or you’re ready to tackle more advanced concepts, Zenva’s courses are designed to elevate your competency and confidence in coding, all while building a portfolio that showcases your achievements.
So, keep up the momentum, and join us at Zenva to turn your programming aspirations into reality. With our courses, you can continue to learn at your own pace and craft your future in the exciting world of technology.
Conclusion
As you continue to explore the vast universe of programming, remember that your journey is much like an exciting game—each new skill is a level up, paving the way to new challenges and achievements. Variables are your allies in this adventure, fundamental tools that allow your code to interact with the world. And as you’ve begun this expedition with variables, there’s an entire expanse of knowledge waiting for you to discover it.
We at Zenva are committed to being your guides through this digital landscape. Our Python Mini-Degree is more than just a set of courses; it’s a gateway to a future in technology that’s brimming with potential. Why not take your next step with us today? Together, we can unlock your full programming potential and turn your passion into a portfolio of impressive, real-world projects. Let the adventure continue!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
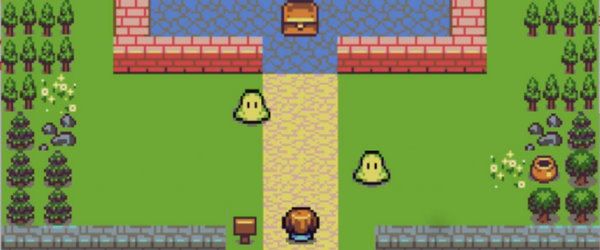
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.