Regular expressions, or regex for short, are incredibly powerful tools for any programmer or developer. They allow you to search, manipulate, and manage text in a way that’s both efficient and effective. Regex can help you solve a vast array of problems – from validating user input to parsing log files. And the beauty of regular expressions is that they are widely supported across different programming languages, including Python, which we’ll be using for our examples. Even if you’re just starting your coding journey, mastering regex will open up a new world of possibilities and efficiency in handling text data. So let’s dive into the world of pattern matching magic!
Table of contents
What Are Regular Expressions?
Regular expressions are sequences of characters that form a search pattern. These patterns can be used for text search and text replace operations in strings. Imagine regular expressions as a secret language that gives you the superpower to tell your computer exactly how to sift through text and find only what you’re looking for.
What Are They Used For?
Think of any situation where text is involved – that’s where regex shines. Whether you’re extracting email addresses from a document, ensuring a password meets complexity requirements, or finding specific game commands in a log file, regex is your go-to tool.
Why Should I Learn Regular Expressions?
Regular expressions are a fundamental skill that will serve you well, no matter your level of expertise. Here are some compelling reasons to learn them:
– **Efficiency:** Regex can reduce complex and lengthy code into a single line of pattern matching genius.
– **Versatility:** Once you master regex, you can use them in many programming languages, not just Python.
– **Advanced Text Processing:** Regex provides you with a way to perform sophisticated text processing that would otherwise be very complicated to implement.
Basic Patterns and Metacharacters
Before we dive into complex patterns, it’s essential to grasp the basics of regex. Here we’ll start with some simple examples that will help you understand how to construct and use regex patterns in Python.
– **Literal Characters**: The most basic pattern consists of literal characters, which match the characters themselves.
import re pattern = r"game" text = "I love playing video games!" match = re.search(pattern, text) if match: print("Match found:", match.group())
This pattern looks for the literal string “game” in the text and, in this case, matches “games”.
– **Dot Metacharacter**: A dot (.) in a regex stands for any character except a newline, making it one of the most versatile metacharacters.
pattern = r"g.me" text = "Do you like to play a game?" match = re.search(pattern, text) if match: print("Match found:", match.group())
This will match “game” in the text, as the dot can represent the ‘a’.
– **Character Classes**: When you need to match any one of several characters, use a character class. This is represented by square brackets [ ].
pattern = r"g[aeiou]me" text = "Let's start the game or maybe a gime?" matches = re.findall(pattern, text) print("Matches found:", matches)
This pattern will match “game” and “gime”, as the pattern specifies a vowel can occur in the second position.
– **The Caret and the Dollar Sign**: Caret (^) is used to match the start of a string, and the dollar sign ($) is used to match the end.
pattern = r"^game" text1 = "game over!" text2 = "start the game!" match1 = re.search(pattern, text1) match2 = re.search(pattern, text2) print("Match at the start of text1:", bool(match1)) print("Match at the start of text2:", bool(match2))
This will match “game” only in `text1` because it starts with “game”, but not in `text2`.
Quantifiers and Grouping
Quantifiers and grouping allow us to match multiple instances of a pattern and to match specific groups of characters.
– **Asterisk Quantifier**: An asterisk (*) means “zero or more” of the preceding element.
pattern = r"ga*me" text = "Let's play a gaaame!" match = re.search(pattern, text) if match: print("Match found:", match.group())
This pattern will match “game”, “gaaame”, or even “gme” because the ‘a’ can appear zero or more times.
– **Plus Quantifier**: A plus (+) is similar to the asterisk, but it means “one or more” of the preceding element.
pattern = r"ga+me" text = "Do you want to play a gaame?" match = re.search(pattern, text) if match: print("Match found:", match.group())
This pattern will match “gaame” but would not match “gme”, because at least one ‘a’ is required.
– **Question Mark Quantifier**: A question mark (?) means “zero or one” of the preceding element.
pattern = r"ga?me" text = "I lost the gme. Let's play another game." matches = re.findall(pattern, text) print("Matches found:", matches)
This pattern will find both “gme” and “game”, because the ‘a’ is optional.
– **Grouping**: Parentheses are used to group together a part of a regex pattern. This enables you to apply quantifiers to entire groups.
pattern = r"(game)+" text = "Do you enjoy a game, game, game?" matches = re.findall(pattern, text) print("Matches found:", matches)
This pattern will match one or more occurrences of the word “game”.
Regex is a subject that grows richer the more you delve into it. By understanding these foundational building blocks, we’re laying the groundwork for tackling even the most challenging text processing tasks with ease. In the next part of our tutorial, we’ll look into more advanced regex features and practical examples that can be put to immediate use. Stay tuned to elevate your coding skills with us!In the previous sections, we’ve covered the basics of regular expressions in Python. Now, let’s elevate our regex knowledge with some more advanced concepts and code examples to help you understand and utilize them effectively.
Advanced Regular Expression Features
Here we’ll delve into non-greedy quantifiers, lookaheads, lookbehinds, and the powerful substitution capabilities of regex.
– **Non-Greedy Quantifiers**: By default, quantifiers like * and + are greedy – they match as much as possible. You can make them non-greedy (lazy) by following them with a question mark.
pattern = r"<.+?>" text = "<div>Hello</div><p>World!</p>" matches = re.findall(pattern, text) print("Non-greedy matches found:", matches)
This will match “<div>” and “</p>” separately rather than the entire string.
– **Lookahead and Lookbehind Assertions**: Lookaheads and lookbehinds allow you to match a pattern that is preceded or followed by another pattern.
– Positive Lookahead: Matches a group before a specific pattern without including it in the result.
pattern = r"game(?= over)" text = "Is the game over? Let's play another game!" matches = re.findall(pattern, text) print("Positive lookahead matches found:", matches)
This will match “game” only when it is followed by ” over”.
– Negative Lookahead: Matches a group that is not followed by a specific pattern.
pattern = r"game(?! over)" text = "Let's start the game! Is the game over?" matches = re.findall(pattern, text) print("Negative lookahead matches found:", matches)
This will match “game” only when it is not followed by ” over”.
– Positive Lookbehind: Matches a group that is after a specific pattern.
pattern = r"(?<=<title>)game" text = "<title>Best game moments</title>" match = re.search(pattern, text) if match: print("Positive lookbehind match found:", match.group())
This will match “game” only when it is preceded by “<title>”.
– Negative Lookbehind: Matches a group that is not after a specific pattern.
pattern = r"(?<!<title>)game" text = "Check out this game, not the <title>game</title>!" matches = re.findall(pattern, text) print("Negative lookbehind matches found:", matches)
This will match “game” only when it is not preceded by “<title>”.
– **Regex Substitution**: One of the most powerful uses of regex is string substitution. Using `re.sub()`, you can find patterns and replace them with new text.
pattern = r"game" replacement = "match" text = "The final game was intense." new_text = re.sub(pattern, replacement, text) print("Substituted text:", new_text)
This will replace the word “game” with “match” in the text.
pattern = r"(\d{2})/(\d{2})/(\d{4})" replacement = r"\2-\1-\3" date = "Today's date is 12/08/2023." new_date = re.sub(pattern, replacement, date) print("Substituted date format:", new_date)
This example shows how you can reformat a date from MM/DD/YYYY to DD-MM-YYYY.
Understanding and using these advanced regex features enhances your capabilities for sophisticated text analysis and manipulation. With practice, you’ll find regex not just a utility but a necessity in many programming scenarios. Keep experimenting with different patterns, and you’ll discover an undeniable efficiency in solving text-related challenges. Stay tuned for even more insightful tutorials, and happy coding!Continuing our exploration of regex, let’s dive into some more practical examples that demonstrate the power of regular expressions in everyday coding situations. These will help solidify your understanding of regex and show you how they can be applied to various problems.
When you deal with data extraction, a common task involves pulling out specific numbers like prices or IDs from a string. Let’s say you have a list of items with their prices and you want to extract just the prices.
pattern = r"\$\d+\.\d{2}" text = "Item1: $15.99, Item2: $23.50, Item3: $11.49" prices = re.findall(pattern, text) print("Prices found:", prices)
This regex pattern matches a dollar sign, followed by one or more digits, a period, and exactly two digits, effectively extracting the price of each item.
Another common operation is validating input formats. For example, you may want to ensure a user has entered a valid email address.
pattern = r"\b[\w.%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b" email = "[email protected]" is_valid = re.fullmatch(pattern, email) print("Is the email valid?", bool(is_valid))
This pattern matches what is typically considered a valid email format, including characters that emails commonly contain such as dots, percents, plus and minus signs, and the @ symbol.
Sometimes, you need to extract multiple pieces of information from a text. For instance, extracting both the domain and the username from an email address can be done using groups in regex.
pattern = r"([\w.%+-]+)@([A-Za-z0-9.-]+\.[A-Z|a-z]{2,})" email = "[email protected]" match = re.search(pattern, email) if match: print("Username:", match.group(1)) print("Domain:", match.group(2))
By using parentheses to create capture groups, you can extract the username and domain separately from the email address input.
Regular expressions can also simplify the process of formatting text. Imagine you have a log file with dates in different formats, and you want to standardize them to a ‘YYYY-MM-DD’ format.
log = """ [12/01/2023 09:11:02] INFO - System started. [01.13.2023 10:16:15] WARNING - Unusual activity detected. [2023-01-14 11:21:42] DEBUG - Performing self-check. """ pattern = r"\[(\d{2})[/-](\d{2})[/.-](\d{4})" replacement = r"[\3-\1-\2]" standard_log = re.sub(pattern, replacement, log) print("Standardized Log:\n", standard_log)
In this example, we are using capturing groups and backreferences in our replacement string to reorder the date components.
Finally, regular expressions can assist in text sanitization tasks. For example, you may want to remove all HTML tags from a string to extract just the textual content.
html_string = "<p>This is a <strong>bold</strong> paragraph.</p>" clean_text = re.sub(r"</?.+?>", "", html_string) print("Sanitized text:", clean_text)
This snippet removes anything that looks like an HTML tag, making sure the text content is clean and ready for further processing or display.
As you’ve seen in these examples, regular expressions are an indispensable tool for a wide range of coding tasks related to text searching, validation, extraction, formatting, and sanitization. The versatility and efficiency of regex make it a valuable skill to enhance your coding toolkit. Keep practicing and refining your regex skills; soon enough, you’ll be adept at employing these patterns to handle all sorts of text-based challenges with confidence and ease.
Continue Your Learning Journey
Now that you’ve stepped into the powerful world of regular expressions, you might be wondering, “Where do I go from here?” As you’ve seen, regex is just one aspect of programming, and there’s a whole universe of coding knowledge waiting for you. We encourage you to keep that momentum going and further your programming prowess.
If you’re looking to develop a robust set of skills in Python, our Python Mini-Degree is the perfect next step. This comprehensive collection of courses will take you from the very basics to more advanced topics, ensuring you build a solid foundation in Python programming. You’ll not only get to grips with coding fundamentals but also explore various applications of Python such as game and app development. Best of all, it’s designed to be flexible, fitting into your schedule while providing a wealth of knowledge to help you grow.
For those of you looking to broaden your programming skills even further, check out our wide range of Programming Courses. Whether you’re considering a career change or seeking to advance in your current field, we have courses that cater to all levels of experience. Join our community of over 1 million learners and developers at Zenva, and let us help you take your coding journey to new heights. Keep learning, keep building, and you’re sure to make great strides in your career and personal projects.
Conclusion
In the end, understanding and applying regular expressions is akin to unlocking a superpower in your text processing abilities. As you move forward, you’ll find these patterns to be invaluable tools in your development toolkit. Remember, regex is just the beginning; programming is a vast and rewarding field, and there’s always something new to learn and master. We’re excited to see where your newfound knowledge will take you and how it will shape your projects and professional endeavors.
We stand with you on this learning journey, eager to offer guidance and support through our comprehensive Python Mini-Degree and other programming courses. At Zenva, our goal is to equip you with practical skills that can make an immediate impact on your career or personal passion projects. So take the leap, continue exploring, and let’s code the future together, one line at a time.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
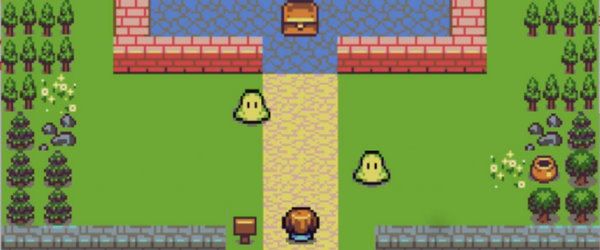
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.