Sorting through a jumble of items, be it the chaotic array of books on a shelf or the jumbled collection of data in a computer, shares a common goal: to impose order, to simplify access, and to streamline processes. When it comes to programming, the act of sorting is just as essential, laying the groundwork for efficient data management and retrieval. In this comprehensive tutorial, we’ll delve into the fascinating world of sorting algorithms – the unsung heroes that make sense of data chaos. Whether you’re a beginner eager to understand the basics or a seasoned developer refining your knowledge, this exploration will enhance your coding arsenal, making you a more adept and efficient programmer.
Table of contents
What is a Sorting Algorithm?
Sorting algorithms are fundamental procedures in computer science, designed to rearrange a sequence of elements into a particular order. This order is typically numerical or lexicographical, drawing parallels to alphabetizing books or arranging playlists by song length.
What is it for?
The utility of sorting stretches across numerous applications:
- Data analysis becomes more feasible when information is sorted.
- Database queries are faster with sorted data.
- Sorting aids in tasks like searching, which heavily rely on ordered data.
Why Should I Learn it?
Embarking on the journey to understanding sorting algorithms opens doors to crucial skills in problem-solving and algorithmic thinking. Here’s why sorting deserves your attention:
- It’s a stepping stone to mastering more complex algorithms and structures.
- Improved efficiency of your code can make or break a project.
- An understanding of sorting algorithms showcases a solid foundational knowledge that potential employers look for.
Keep reading to discover the ins and outs of sorting algorithms through engaging examples and clear explanations that make this technical topic accessible and intriguing. Get ready to sort out the confusion and streamline your coding expertise!
Understanding Bubble Sort
Bubble Sort is one of the simplest sorting algorithms that works by repeatedly stepping through the list, comparing each pair of adjacent items and swapping them if they are in the wrong order. The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted.
The following code is a basic example of Bubble Sort in action:
def bubbleSort(arr): n = len(arr) # Traverse through all elements in the array for i in range(n): # Last elements are already in place for j in range(0, n-i-1): # Swap if the element found is greater than the next element if arr[j] > arr[j+1]: arr[j], arr[j+1] = arr[j+1], arr[j] sampleArray = [64, 34, 25, 12, 22, 11, 90] bubbleSort(sampleArray) print("Sorted array is:", sampleArray)
This Python function demonstrates how to sort an array using Bubble Sort. Notice how we iterate through the list multiple times, swapping elements to ensure the largest number ‘bubbles up’ to the correct position.
Diving into Insertion Sort
Insertion Sort is another elementary algorithm that builds the final sorted array one item at a time. It is efficient for small data sets, and its concept is similar to the way you might arrange playing cards in your hands.
Here’s how you can implement Insertion Sort:
def insertionSort(arr): # Traverse through 1 to length of array for i in range(1, len(arr)): key = arr[i] # Move elements of arr[0..i-1] that are greater than key # to one position ahead of their current position j = i-1 while j >=0 and key < arr[j]: arr[j+1] = arr[j] j -= 1 arr[j+1] = key sampleArray = [12, 11, 13, 5, 6] insertionSort(sampleArray) print("Sorted array is:", sampleArray)
Insertion Sort runs by picking one element (the ‘key’) from the array and comparing it with elements before it, inserting it into its correct position. The algorithm essentially builds a sorted sublist at the beginning of the array, placing each new element where it belongs in this sorted part.
Exploring Selection Sort
Selection Sort is a bit different; this algorithm segments the list into two parts: sorted and unsorted. It repeatedly selects the smallest (or largest, depending on sorting order) element from the unsorted segment and moves it to the end of the sorted segment.
Here is what Selection Sort looks like in a Python function:
def selectionSort(arr): for i in range(len(arr)): # Find the minimum element in the remaining unsorted array min_idx = i for j in range(i+1, len(arr)): if arr[min_idx] > arr[j]: min_idx = j # Swap the found minimum element with the first element of the unsorted segment arr[i], arr[min_idx] = arr[min_idx], arr[i] sampleArray = [64, 25, 12, 22, 11] selectionSort(sampleArray) print("Sorted array is:", sampleArray)
In the above code, we iterate over the array and with each pass, select the smallest unsorted element and swap it with the element at the beginning of the unsorted section, effectively growing the sorted section of the array.
Stay tuned for our next section where we will delve into more complex sorting algorithms, offering a peek into how sorting can be taken to the next level for better efficiency and speed.Great! Let’s press on and explore more intricate sorting algorithms that can handle larger datasets with greater agility. Here, we’ll look at some advanced concepts such as Merge Sort, Quick Sort, Heap Sort, and Radix Sort.
Conquering Merge Sort
Merge Sort is a classic example of a divide-and-conquer algorithm. It works by recursively dividing the array into halves until each half is manageable (typically when it has only one element), and then merging those sorted halves back together.
Let’s examine a Merge Sort implementation in Python:
def mergeSort(arr): if len(arr) > 1: # Finding the mid of the array mid = len(arr) // 2 L = arr[:mid] # Left half R = arr[mid:] # Right half # Recursive call on each half mergeSort(L) mergeSort(R) # Merge the sorted halves i = j = k = 0 # Copy data to temp arrays L[] and R[] while i < len(L) and j < len(R): if L[i] < R[j]: arr[k] = L[i] i += 1 else: arr[k] = R[j] j += 1 k += 1 # Checking if any element was left while i < len(L): arr[k] = L[i] i += 1 k += 1 while j < len(R): arr[k] = R[j] j += 1 k += 1 sampleArray = [38, 27, 43, 3, 9, 82, 10] mergeSort(sampleArray) print("Sorted array is:", sampleArray)
As seen above, the Merge Sort algorithm carefully combines smaller sorted lists into larger sorted ones until the whole list is sorted.
Quick Sort Unveiled
Another divide-and-conquer hero in the sorting algorithm repertoire is Quick Sort. Quick Sort partitions an array into two halves around a pivot element, and then recursively sorts the sub-arrays.
Below is a Quick Sort Python function:
def quickSort(arr, low, high): if low < high: # pi is partitioning index, arr[pi] is now at right place pi = partition(arr, low, high) # Recursively sort elements before and after partition quickSort(arr, low, pi-1) quickSort(arr, pi+1, high) def partition(arr, low, high): # Choose the rightmost element as pivot pivot = arr[high] i = low - 1 for j in range(low, high): # If current element is smaller than or equal to pivot if arr[j] <= pivot: i = i + 1 arr[i], arr[j] = arr[j], arr[i] # Swap the pivot element with the element at i+1 arr[i + 1], arr[high] = arr[high], arr[i + 1] return i + 1 sampleArray = [10, 7, 8, 9, 1, 5] quickSort(sampleArray, 0, len(sampleArray) - 1) print("Sorted array is:", sampleArray)
Quick Sort shines in its average-case performance and is often faster in practice than other O(n log n) algorithms like Merge Sort or Heap Sort.
Delving into Heap Sort
Heap Sort is a comparison-based sorting technique based on a Binary Heap data structure. It’s an in-place algorithm, meaning it doesn’t require extra space for data storage. Here the idea is to first build a max heap from the input data, and then, by repeatedly extracting the maximum element from the heap and replace it with the last item of the heap followed by reducing the size of heap by one.
Below is the Python code snippet for Heap Sort:
def heapify(arr, n, i): largest = i # Initialize largest as root l = 2 * i + 1 # left = 2*i + 1 r = 2 * i + 2 # right = 2*i + 2 # See if left child of root exists and is greater than root if l arr[largest]: largest = l # See if right child of root exists and is greater than root if r arr[largest]: largest = r # Change root, if needed if largest != i: arr[i], arr[largest] = arr[largest], arr[i] # swap # Heapify the root. heapify(arr, n, largest) def heapSort(arr): n = len(arr) # Build a maxheap. for i in range(n // 2 - 1, -1, -1): heapify(arr, n, i) # One by one extract elements for i in range(n - 1, 0, -1): arr[i], arr[0] = arr[0], arr[i] # swap heapify(arr, i, 0) sampleArray = [12, 11, 13, 5, 6, 7] heapSort(sampleArray) n = len(sampleArray) print("Sorted array is:", sampleArray)
Heap Sort uses the properties of a heap to guarantee that the largest element is moved to the end of the sorted section of the array.
Navigating through Radix Sort
Radix Sort is a non-comparative integer sorting algorithm that sorts data with integer keys by grouping keys by individual digits which share the same significant position and value (a kind of bucket sort according to significant digits).
Check out the example for Radix Sort:
def countingSort(arr, exp1): n = len(arr) # The output array elements that will have sorted arr output = [0] * n # Initialize count array as 0 count = [0] * 10 # Store count of occurrences in count[] for i in range(n): index = arr[i] // exp1 count[index % 10] += 1 # Change count[i] so that count[i] now contains actual # position of this digit in output array for i in range(1, 10): count[i] += count[i-1] # Build the output array i = n - 1 while i >= 0: index = arr[i] // exp1 output[count[index % 10] - 1] = arr[i] count[index % 10] -= 1 i -= 1 # Copying the output array to arr[] for i in range(len(arr)): arr[i] = output[i] def radixSort(arr): # Find the maximum number to know the number of digits max1 = max(arr) # Do counting sort for every digit. Note that instead of passing digit number, # exp is passed. exp is 10^i where i is current digit number exp = 1 while max1 // exp > 0: countingSort(arr, exp) exp *= 10 sampleArray = [170, 45, 75, 90, 802, 24, 2, 66] radixSort(sampleArray) print("Sorted array is:", sampleArray)
Radix Sort is efficient for sorting large sets of data because it minimizes the number of comparisons and operates on individual digits.
Now you’ve glimpsed the power and intricacies of various sorting algorithms. From the fundamental bubble and insertion sorts to the speed of quick and merge sorts, each algorithm presents unique advantages for different situations. Our exploration has equipped you with valuable insights into this essential component of programming, ensuring you wield the necessary knowledge to tackle any messy data set with confidence!
Stay tuned for our next tutorials, where we dive even deeper into computer science’s exciting world, always focusing on practical, bite-sized learning to propel you forward in your coding journey. Happy sorting!As we venture further into the realm of sorting algorithms, we’ll turn our attention to some variations and optimizations that enhance the basic algorithms we’ve discussed, and tackle specific sorting challenges with examples and explanations.
Let’s first enhance the Merge Sort by adding an optimized approach that minimizes the space complexity by eliminating the need to create additional arrays for merging the two halves.
def merge(arr, l, m, r): n1 = m - l + 1 n2 = r - m # create temp arrays L = [0] * n1 R = [0] * n2 # Copy data to temp arrays L[] and R[] for i in range(0, n1): L[i] = arr[l + i] for j in range(0, n2): R[j] = arr[m + 1 + j] # Merge the temp arrays back into arr[l..r] i = 0 # Initial index of first subarray j = 0 # Initial index of second subarray k = l # Initial index of merged subarray while i < n1 and j < n2: if L[i] <= R[j]: arr[k] = L[i] i += 1 else: arr[k] = R[j] j += 1 k += 1 # Copy the remaining elements of L[], if there are any while i < n1: arr[k] = L[i] i += 1 k += 1 # Copy the remaining elements of R[], if there are any while j < n2: arr[k] = R[j] j += 1 k += 1 def mergeSortInPlace(arr, l, r): if l < r: # Same as (l+r)//2, but avoids overflow for large l and h m = l + (r - l) // 2 # Sort first and second halves mergeSortInPlace(arr, l, m) mergeSortInPlace(arr, m+1, r) merge(arr, l, m, r) sampleArray = [12, 11, 13, 5, 6, 7] mergeSortInPlace(sampleArray, 0, len(sampleArray) - 1) print("Sorted array is:", sampleArray)
The above implementation of Merge Sort is more space efficient as it uses existing space for the merging process, rather than creating new arrays each time we merge two halves.
Next, we can optimize the Quick Sort by implementing a 3-way partitioning method. This method is particularly useful when the array contains many duplicate elements. The code example below shows a 3-way Quick Sort:
def partition3Way(arr, low, high): if high <= low: return # lt and gt are the pointers that are used to move elements lt = low gt = high v = arr[low] i = low while i <= gt: if arr[i] v: arr[gt], arr[i] = arr[i], arr[gt] gt -= 1 else: i += 1 return lt, gt def quickSort3Way(arr, low, high): if high <= low: return lt, gt = partition3Way(arr, low, high) quickSort3Way(arr, low, lt-1) quickSort3Way(arr, gt+1, high) sampleArray = [12, 11, 13, 5, 6, 7, 5, 6, 12, 11] quickSort3Way(sampleArray, 0, len(sampleArray) - 1) print("Sorted array with duplicates is:", sampleArray)
The 3-way partition Quick Sort handles duplicates by dividing the array into three parts: one part less than the pivot, one part equal to the pivot, and one part greater than the pivot. This reduces the number of swaps and recursive calls when there are many duplicates.
Continuing on, we can examine an efficient method for sorting arrays that exhibit certain patterns, such as nearly sorted data. In such cases, Insertion Sort can prove to be more efficient than even the more sophisticated algorithms due to its adaptive nature. Here’s an example where we use Insertion Sort to optimize for nearly sorted arrays:
def insertionSortAdaptive(arr): for i in range(1, len(arr)): key = arr[i] j = i-1 while j >= 0 and key < arr[j]: arr[j + 1] = arr[j] j -= 1 arr[j + 1] = key sampleArray = [2, 3, 5, 6, 4, 1] insertionSortAdaptive(sampleArray) print("Sorted array is:", sampleArray)
In adaptive Insertion Sort, elements are moved only as much as necessary to insert the element into the correct place. If the array is nearly sorted to begin with, this means very few moves will be made, resulting in an efficient sorting process.
Lastly, let’s touch upon a non-traditional approach known as Counting Sort. This algorithm is not comparison based and instead counts occurrences of elements within a range. However, it is only effective when the range of potential items in the array is not significantly greater than the number of items to be sorted. Here’s a basic example:
def countingSort(arr): output = [0 for i in range(len(arr))] count = [0 for i in range(256)] ans = ["" for _ in arr] # Store count of each character for i in arr: count[i] += 1 # Change count[i] so that count[i] contains the actual # position of this character in the output array for i in range(256): count[i] += count[i-1] # Build the output character array for i in range(len(arr)): output[count[arr[i]]-1] = arr[i] count[arr[i]] -= 1 # Copy the output array to arr, so that arr now # contains sorted characters for i in range(len(arr)): ans[i] = output[i] return ans sampleArray = [1, 4, 1, 2, 7, 5, 2] print("Sorted array is:", countingSort(sampleArray))
Counting Sort creates an auxiliary array to count the occurrence of each value, and then calculates the position of each element in the sorted array. It is particularly efficient when dealing with restricted range integers and can achieve linear time complexity, as opposed to the common O(n log n) time complexity for many other sorting algorithms.
These additional snippets complement our understanding of sorting algorithms, allowing us to choose the most appropriate method depending on the data’s characteristics and requirements of the application. Each of these algorithms has its own strengths, and knowing which one to choose can greatly enhance the performance of your program.
Through these code examples and optimization techniques, we’re adding valuable tools to your repertoire, enabling you to navigate through the challenges of sorting with confidence and expertise. Keep experimenting with these algorithms, and you’ll find your coding skills growing stronger with each sorted array!
Continue Your Learning Journey with Zenva
Embarking on a journey through the world of coding and sorting algorithms is just the beginning. To keep growing your skills and expanding your knowledge, we at Zenva invite you to delve deeper into the diverse and dynamic realm of Python programming with our Python Mini-Degree. Our courses are meticulously crafted to cater to learners at various stages of their education, from beginners to those looking to polish their skills further.
Our Python Mini-Degree offers a thorough exploration of not only basic programming principles but also dives into more advanced topics like algorithms, object-oriented programming, and application development. Through hands-on projects, you’ll gain practical experience that builds a solid foundation and enriches your professional portfolio. Python’s versatility and demand in industries, especially data science, make the skills you’ll acquire invaluable.
For those with a wider interest in programming, our extensive collection of courses covers an array of subjects in the tech landscape. Check out all of our Programming courses to find content that aligns with your aspirations and career goals. At Zenva, we’re committed to providing learners with quality education that’s accessible and can fit into any schedule. Continue your learning journey with us, and take the leap from beginner to professional. Your future in the tech world awaits!
Conclusion
As we bring our exploration of sorting algorithms to a close, we hope you feel empowered to tackle any dataset, no matter how daunting it may seem. With the tools, examples, and optimizations we’ve shared, you’re well on your way to becoming a more efficient and thoughtful programmer. Remember, understanding the underlying principles of these algorithms is more than an academic exercise—it’s a practical skill that will serve you well in numerous coding projects and real-world applications.
We encourage you to keep practicing, keep learning, and never stop refining your craft. For those eager to continue their journey and delve deeper into the richness of Python and other programming languages, our Python Mini-Degree awaits. It’s the perfect next step to enhance your skills and unlock new possibilities in the ever-evolving tech landscape. Join us at Zenva, where learning never stops, and every challenge is another opportunity for growth. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
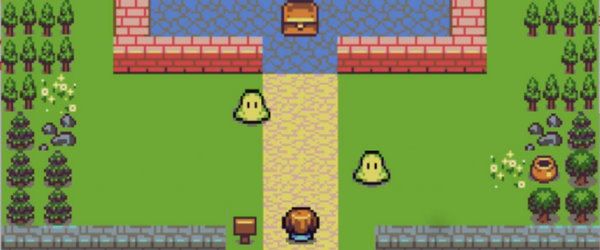
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.