Arrays are a fundamental concept in programming that power countless applications, from basic to-dos to complex game mechanics and sophisticated algorithms. Why should anyone learn about arrays, you might wonder? Simply put, mastering arrays is like sharpening your sword before a battle – it equips you to slice through data-related challenges with ease. Whether you are just starting out or looking to refresh your knowledge, arrays are indispensable tools in your coding arsenal. So, let’s embark on this journey together and untangle the mysteries of arrays in an engaging and accessible way.
Table of contents
What is an Array?
An array is a collection of elements, all of the same type, stored in contiguous memory locations. Think of it as a row of mailboxes, each with a distinctive address or index, and each mailbox can hold a piece of mail or a datum.
What Are Arrays Used For?
Arrays are used to organize data so that a related set of values can be easily sorted, searched, or manipulated. They are ideal for storing data that is inherently sequential in nature, such as:
- High scores in a game
- A list of inventory items
- Days in a month
Why Should I Learn About Arrays?
Understanding arrays is vital because they:
– Serve as the building blocks for more complex data structures.
– Optimize the management and performance of large datasets.
– Are widely used in algorithm development.
– Help in understanding loop control structures through array iteration.
In essence, arrays are a gateway to writing efficient, organized, and scalable code. Let’s dive into coding examples to see arrays in action and unravel their potential!
Creating and Accessing Arrays
Before we manipulate data within arrays, we need to know how to create and access them. Here’s how we declare an array in JavaScript:
let fruits = ['Apple', 'Banana', 'Cherry'];
We’ve just created an array named `fruits` containing three elements. To access an element, we use its index (position in the array), starting at 0:
console.log(fruits[0]); // Outputs: Apple console.log(fruits[1]); // Outputs: Banana
Remember, the first element is at index 0, not 1.
Modifying Array Elements
Arrays are flexible — we can easily change their contents. To modify an element, assign a new value to its index:
fruits[2] = 'Dragonfruit'; console.log(fruits); // Outputs: ['Apple', 'Banana', 'Dragonfruit']
Now, ‘Cherry’ has been replaced with ‘Dragonfruit’.
Looping Through Arrays
To perform operations on each element, we can loop through the array. A common approach is to use a `for` loop:
for (let i = 0; i < fruits.length; i++) { console.log(fruits[i]); }
This loop will print every element in the `fruits` array to the console.
Adding and Removing Elements
JavaScript arrays offer methods to add or remove elements. Let’s add an element to the end of our `fruits` array:
fruits.push('Mango'); console.log(fruits); // Outputs: ['Apple', 'Banana', 'Dragonfruit', 'Mango']
To remove the last element, we use the `pop` method:
let lastFruit = fruits.pop(); console.log(fruits); // Outputs: ['Apple', 'Banana', 'Dragonfruit'] console.log(lastFruit); // Outputs: Mango
These examples scratch the surface of how to work with arrays — there are other methods and techniques which we will explore in the next section of our tutorial. Stay tuned to level up your array-handling skills!
Advanced Array Operations
As we dive deeper into arrays, we encounter advanced operations that enhance our data manipulation capabilities. Let’s explore some of these.
Using `forEach` for Iteration
While a `for` loop is effective, JavaScript’s `forEach` method provides a clearer syntax for iterating over an array:
fruits.forEach(function(fruit) { console.log(fruit); });
This will output each fruit in the `fruits` array, similar to our `for` loop earlier, but with a more functional approach.
Finding Elements in an Array
To check whether an array contains a specific element, we can use the `includes` method:
let hasApple = fruits.includes('Apple'); console.log(hasApple); // Outputs: true
To find the index of a particular element, `indexOf` comes in handy:
let bananaIndex = fruits.indexOf('Banana'); console.log(bananaIndex); // Outputs: 1
And if we want to find an item based on a test function, `find` is our friend:
let longNameFruit = fruits.find(fruit => fruit.length > 6); console.log(longNameFruit); // Outputs: 'Dragonfruit'
Transforming Arrays with `map`
The `map` function creates a new array by transforming every element in the original array:
let uppercaseFruits = fruits.map(fruit => fruit.toUpperCase()); console.log(uppercaseFruits); // Outputs: ['APPLE', 'BANANA', 'DRAGONFRUIT']
Filtering Arrays with `filter`
If we need to create a new array with elements that pass a certain condition, we use `filter`:
let longFruits = fruits.filter(fruit => fruit.length > 6); console.log(longFruits); // Outputs: ['Dragonfruit']
Reducing an Array with `reduce`
The `reduce` method allows us to derive a single value from an array, such as summing numbers or concatenating strings:
let numbers = [1, 2, 3, 4, 5]; let sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue); console.log(sum); // Outputs: 15
Combining Arrays with `concat`
We can combine two or more arrays using the `concat` method:
let vegetables = ['Carrot', 'Potato', 'Cucumber']; let produce = fruits.concat(vegetables); console.log(produce); // Outputs: ['Apple', 'Banana', 'Dragonfruit', 'Carrot', 'Potato', 'Cucumber']
These examples highlight the power and flexibility of arrays in JavaScript. Whether you are adding, removing, transforming, or combining data, arrays provide a set of tools tailored for these tasks. Armed with these skills, you’ll be able to handle a wide range of programming challenges with ease, making arrays a core part of your development expertise.
Remember, these are just a few examples of what can be accomplished with arrays. There’s a vast ocean of possibilities once you start to mix and match these methods in your code, and we at Zenva are thrilled to help you navigate this journey. Keep practicing, keep coding, and soon you’ll wield the power of arrays like a pro!Let’s continue exploring the powerful operations you can perform with arrays by looking at some additional coding examples:
Sorting Arrays
Sorting is a common task when dealing with arrays of data. The `sort` method allows you to order the elements in an array:
let scores = [10, 50, 20, 5, 35]; scores.sort((a, b) => a - b); console.log(scores); // Outputs: [5, 10, 20, 35, 50]
By default, `sort` orders elements as strings, but providing a compare function allows for numerical sorting.
Reversing Arrays
If you need to reverse the order of elements in an array, the `reverse` method makes it a breeze:
let reversedFruits = fruits.slice().reverse(); // Create a copy and reverse it console.log(reversedFruits); // Outputs: ['Dragonfruit', 'Banana', 'Apple']
Notice we used `slice` to avoid mutating the original `fruits` array.
Joining Array Elements
Combining array elements into a single string is often required, and the `join` method is perfect for this operation:
let fruitString = fruits.join(', '); console.log(fruitString); // Outputs: 'Apple, Banana, Dragonfruit'
By passing a string to `join`, you can specify the separator between elements.
Finding the Length of an Array
Determining the number of elements in an array is essential in many contexts. Use the `length` property to get this information:
console.log(fruits.length); // Outputs: 3
Knowing the size of your array can help manage loops and conditionals based on the number of elements.
Checking If An Object Is An Array
Sometimes it’s necessary to confirm if a variable is an array, particularly when the data type is uncertain. The `Array.isArray` method is the best way to do this:
console.log(Array.isArray(fruits)); // Outputs: true console.log(Array.isArray('Hello, world!')); // Outputs: false
Using this method ensures that you’re working with an array and prevents potential errors in your code.
Combining Multiple Array Methods
The real fun begins when you start chaining array methods to perform complex operations. Here’s a combo: filtering out small numbers, sorting the remaining ones, and then mapping them to their square roots:
let numbers = [36, 1, 7, 49, 64]; let transformedNumbers = numbers .filter(number => number > 10) .sort((a, b) => a - b) .map(number => Math.sqrt(number)); console.log(transformedNumbers); // Outputs: [6, 7, 8]
Creating Multidimensional Arrays
Lastly, arrays can be nested to form multidimensional arrays, which are akin to matrices or tables:
let matrix = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ]; // Accessing the element in the second row, third column console.log(matrix[1][2]); // Outputs: 6
These examples give you a taste of the depth that arrays offer. By mastering arrays and their methods, you can solve a variety of complex programming exercises and real-world problems, making them an invaluable part of your developer toolkit.
At Zenva, we believe that the best way to learn is by doing, and we encourage you to take these examples and expand upon them, experimenting with the vast array operations JavaScript offers. Practice making your own combinations and see what creative solutions you can come up with!
Where to Go Next in Your Coding Journey
If you’ve enjoyed unraveling the mysteries of arrays and are eager to advance your programming skills even further, we have the perfect next steps for you. Our Python Mini-Degree is a deep dive into one of the most versatile and beginner-friendly programming languages available today. Through this collection of courses, you’ll gain a thorough understanding of Python, tackling everything from coding fundamentals to game and app development. It’s a fantastic opportunity to learn by building tangible projects that can spruce up your portfolio.
Looking to widen your skill set across multiple programming languages and domains? Our extensive catalogue of Programming courses is tailored to guide you from the basics to the professional level in a flexible and practical manner. Whether you aim to code sophisticated games, applications, or dive into the exciting world of AI, Zenva Academy offers over 250 supported courses to help you achieve your career goals and earn certificates to showcase your accomplishments.
While our courses at Zenva Academy are designed to be comprehensive, they aim to make learning to code as approachable and engaging as possible. The transformation from beginner to an adept programmer is but a few clicks away. Take the next step. Keep learning, keep coding, and let’s build the future one line of code at a time!
Conclusion
Embarking on a journey through the landscape of programming can be as thrilling as it is rewarding. Arrays are just one of the many tools you’ll come to master, each like a thread in the vast tapestry of code that powers our digital world. By continuing to build on your foundational knowledge with hands-on experience through our comprehensive courses, you’re on your way to becoming not just a competent coder, but a true artisan of the craft.
When you’re ready to take the next leap forward, our Python Mini-Degree awaits to unlock new horizons in your coding adventure. Whether your aim is to create groundbreaking software, weave intricate game mechanics, or analyze complex datasets, we at Zenva are here to guide you every step of the way. Dive deep, code passionately, and let’s shape the future. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
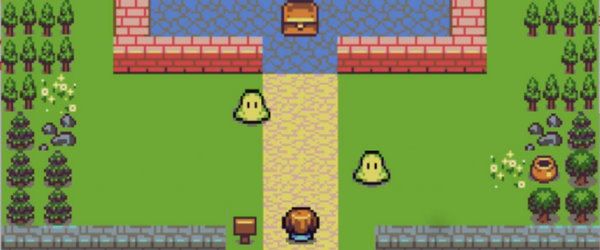
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.