Have you ever wondered how a game character knows when to jump, or how your calculator app correctly adds up your grocery bills? The secret behind these actions often lies in the concept of function parameters—a fundamental cornerstone of programming that enables you to create dynamic and interactive programs. Whether you’re just starting your coding adventure or polishing your skills, understanding function parameters will enhance your ability to craft efficient and powerful software.
What Are Function Parameters?
Table of contents
Function Parameters: The Basics
In programming, functions are like individual tasks or instructions that a program can execute, and parameters are the finer details these tasks need to get carried out effectively. Think of function parameters as the ‘settings’ you can tweak to customize a function’s behavior each time you call it. For instance, when playing a game, if you want a character to move, you might have a function for movement, and parameters could specify the direction and distance.
The Purpose of Function Parameters
Function parameters are designed to make your code more versatile and reusable. Without parameters, you’d have to write a new function every time you wanted to perform a similar action with different specifics. To stick with the gaming analogy, that would be like creating a new jump function for every type of jump in a game, rather than having one jump function that you can customize using parameters.
Why Should I Learn About Function Parameters?
Understanding function parameters is crucial for several reasons:
– They help you write clean and maintainable code by reducing repetition.
– Parameters allow for greater code flexibility and functionality.
– They are foundational in the creation of interactive applications.
By mastering function parameters, you’re not just learning a specific tool; you’re unlocking the capability to create highly customizable and complex systems, which can be an immensely satisfying part of programming. Plus, the concept of function parameters transcends virtually all programming languages, making it a universally valuable skill set.
Defining Functions with Parameters
When defining a function, parameters are placed within the parentheses following the function name. These act as placeholders for the values that will be passed to the function when it is called. Let’s explore this with some examples.
// A simple greeting function with one parameter function greet(name) { console.log("Hello, " + name + "!"); } // Calling the function with the parameter greet("Alice"); // Output: Hello, Alice!
Parameters allow the same function to be used with different inputs, making your code more flexible.
// A function to add two numbers function add(num1, num2) { return num1 + num2; } // Calling the function with different sets of parameters console.log(add(5, 10)); // Output: 15 console.log(add(20, 30)); // Output: 50
By defining parameters, you set the stage for the function to handle various input values, enabling dynamic operations with a single function definition.
Multiple Parameters and Argument Passing
Functions can have multiple parameters separated by commas, allowing for more complex operations.
// A function to calculate the area of a rectangle function calculateArea(width, height) { return width * height; } // Using the function to calculate different areas console.log(calculateArea(5, 10)); // Output: 50 console.log(calculateArea(7, 14)); // Output: 98
Remember, the order in which you pass the arguments matters, as they correspond to the parameters in the order they are listed in the function definition.
// A game character move function function moveCharacter(direction, distance) { console.log("Moving " + direction + " by " + distance + " units."); } // Controlling character movement moveCharacter("forward", 5); // Output: Moving forward by 5 units. moveCharacter("backward", 10); // Output: Moving backward by 10 units.
Each time `moveCharacter` is called, it utilizes the given direction and distance to perform the action, showcasing the value of parameters in controlling the function’s outcome.
Default Parameter Values
JavaScript ES6 introduced default parameters, which allow parameters to have a preset value in case no argument is passed.
// Setting a default value for the name parameter function greet(name = "adventurer") { console.log("Greetings, " + name + "!"); } // Calling the function without specifying the name greet(); // Output: Greetings, adventurer! // Calling the function with a name argument greet("Luna"); // Output: Greetings, Luna!
Default parameters ensure that your function behaves predictably even when some information is not provided.
// A coffee order function with a default type function orderCoffee(type = "black coffee", sugar = 0) { console.log("Order placed: " + type + " with " + sugar + " sugar(s)."); } // Ordering different types of coffee orderCoffee(); // Output: Order placed: black coffee with 0 sugar(s). orderCoffee("cappuccino", 2); // Output: Order placed: cappuccino with 2 sugar(s).
Default values are particularly useful for functions that have optional parameters or when you want to establish common default behavior.
Understanding Scope of Function Parameters
Parameters have local scope within the function, meaning they can only be accessed within the function body. This isolation ensures that external variables with the same name do not interfere with the parameters.
// Parameter 'x' is local to the function function double(x) { return x * 2; } // The variable 'x' outside the function is unaffected let x = 10; console.log(double(x)); // Output: 20 console.log(x); // Output: 10
The same name can be used for a parameter and an external variable without conflict, demonstrating the encapsulated nature of function parameters.
// Function parameters 'a' and 'b' are separate from external variables let a = 5; let b = 10; function subtract(a, b) { return a - b; } console.log(subtract(20, 5)); // Output: 15 console.log(a); // Output: 5 console.log(b); // Output: 10
Even though the parameters `a` and `b` have the same name as external variables, they remain distinct within the function context.
Functions with Variable Number of Arguments
JavaScript supports functions that can handle an unspecified number of arguments using the `arguments` object or the rest parameter syntax `…`.
// Using the 'arguments' object function sumAll() { let total = 0; for(let i = 0; i < arguments.length; i++) { total += arguments[i]; } return total; } console.log(sumAll(1, 2, 3, 4)); // Output: 10
The `arguments` object is an array-like object available within functions that allows iteration over all arguments passed.
// Using the rest parameter syntax function multiplyAll(...nums) { return nums.reduce((product, num) => product * num, 1); } console.log(multiplyAll(1, 2, 3, 4)); // Output: 24
The rest parameter syntax gathers all remaining arguments into an array, making it easier to handle variable numbers of arguments with array methods.
Passing Functions as Arguments
In JavaScript, functions are first-class citizens, meaning you can pass a function as an argument to another function.
// Passing a function as an argument function performOperation(x, y, operation) { return operation(x, y); } function add(a, b) { return a + b; } function multiply(a, b) { return a * b; } console.log(performOperation(10, 5, add)); // Output: 15 console.log(performOperation(10, 5, multiply)); // Output: 50
By passing `add` and `multiply` as arguments to `performOperation`, we dynamically determine which operation to perform.
// An Array method that uses a function as a parameter let numbers = [1, 2, 3, 4, 5]; let squaredNumbers = numbers.map(function(number) { return number * number; }); console.log(squaredNumbers); // Output: [1, 4, 9, 16, 25]
Here, the `map` method accepts a function as a parameter to perform an operation on every element of the array.
By now, you should have a firm grasp of the versatility and power of function parameters. These examples demonstrate just a fraction of the myriad ways parameters can be leveraged to create flexible, sophisticated, and neatly-organized code. Harnessing the full potential of function parameters will undoubtedly enhance your development capabilities, paving the way for more advanced programming techniques and solidifying your understanding of key programming concepts. Keep experimenting with different parameter patterns and their implementations to truly master your craft.Now, let’s dive deeper into function parameters by working with advanced patterns and exploring how we can leverage parameters to achieve greater functionality within our code. Throughout this section, I’ll guide you through several code examples to illustrate these concepts in action.
Let’s start with parameter destructuring, a feature in ES6 that allows us to pass an object to a function and destructure its properties within the function parameters:
// Function with destructured object parameters function createBlogPost({ title, content, author }) { console.log(`Title: ${title}`); console.log(`Content: ${content}`); console.log(`Author: ${author}`); } // Passing an object when calling the function createBlogPost({ title: "Function Parameters in Depth", content: "Understanding function parameters is crucial...", author: "Jane Doe" });
This pattern is especially useful when dealing with configuration objects, allowing easy extraction of specific properties without having to access the object properties one by one.
Next, let’s explore the use of callback functions as parameters, which is a common pattern in asynchronous operations such as event handling or fetching data:
// A sample asynchronous function with a callback function fetchData(url, callback) { // Simulating data fetching with setTimeout setTimeout(() => { let data = "Fetched Data"; callback(data); }, 1000); } // Using the function with a callback fetchData("http://api.example.com/data", function(result) { console.log(result); // Output: Fetched Data });
Callbacks enable you to execute code after an asynchronous operation completes, giving you control over the flow of your program.
Now let’s consider how you can use higher-order functions to configure behavior. Higher-order functions are functions that either take other functions as parameters or return a function:
// Function returning another function function multiplier(factor) { return function(number) { return number * factor; }; } // A multiplier for 2 let doubler = multiplier(2); console.log(doubler(5)); // Output: 10 // A multiplier for 10 let tenfold = multiplier(10); console.log(tenfold(5)); // Output: 50
The `multiplier` function allows you to create customizable multiplier functions, illustrating how returning functions can encapsulate specific behavior.
Let’s also talk about parameter spreading. This is another ES6 feature where you can spread an array to pass its elements as individual arguments to a function:
// A function that requires three arguments function setRGB(red, green, blue) { console.log(`RGB color: (${red}, ${green}, ${blue})`); } // Using spread syntax to pass array elements as arguments let color = [255, 200, 0]; setRGB(...color);
Spreading can be used similarly to pass multiple arguments stored in an array, which is very handy for mathematical operations and array manipulations.
Lastly, consider how you can use parameter values to dynamically set object properties:
// Function using parameter values for object properties function createPerson(name, age, job) { return { name, age, job }; } // Creating a new person object let person = createPerson("Alice", 30, "Engineer"); console.log(person); // Output: // { // name: "Alice", // age: 30, // job: "Engineer" // }
The shorthand property names feature from ES6 allows you to quickly generate objects using parameter values, reducing the verbosity of your code.
Each of these examples unveils another layer of the power and sophistication you can achieve with function parameters in JavaScript. As you continue to apply these patterns into your own projects, you’ll discover not just their utility but also how they can lead to clearer and more expressive programming practices. Keep experimenting, keep iterating, and most importantly, keep enjoying the process of becoming an even more proficient coder.
Continue Your Coding Journey
Your exploration into the world of function parameters is just one step in the vast journey of programming. Taking what you’ve learned about parameters and applying it to broader coding contexts can open up endless possibilities for development and personal growth.
We at Zenva encourage you to keep the momentum going by delving into our Python Mini-Degree, where you can build upon your existing knowledge and tackle new challenges. Python, with its clear syntax and powerful libraries, is an excellent language to solidify your programming foundations while also enabling you to venture into fields such as game development and data science.
If you’re looking to expand your expertise even further, explore our comprehensive collection of Programming courses. Here, we cater to a range of topics and skill levels, from the basics to more advanced concepts. With each course, you can learn at your own pace, create impressive portfolio projects, and earn certificates that showcase your skills to potential employers or clients.
No matter where you are in your coding journey, Zenva is here to support and guide you every step of the way. Let’s continue to grow, learn, and create together!
Conclusion
In mastering function parameters, you have armed yourself with a tool that brings precision and creativity to coding. Every parameter you define or function you call is a chance to refine your craft, create more intricate programs, and streamline your code into a work of digital artistry.
As we conclude this exploration, we invite you to continue honing your skills with Zenva. Check out our Python Mini-Degree and unlock new realms of possibilities in programming. Remember, each line of code you write is a brushstroke in your ever-growing canvas of technological achievements. Keep coding, keep creating, and we at Zenva can’t wait to see what you’ll build next.
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
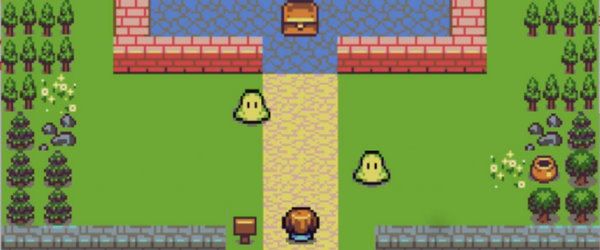
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.