As we dive into the world of game development with the popular platform Roblox, understanding its services becomes crucially important. Tween Service is one such feature available in Roblox Lua that helps to create smooth transitions and movements for various game elements.
Table of contents
What is Roblox Tween Service?
Roblox Tween Service is a powerful tool that allows developers to animate parts, GUI objects, and various other elements in their game. The term ‘Tween’ is short for ‘in-betweening’, which in the context of Roblox, signifies the transition from one property value to another over a specified period.
What is it used for?
We use Tween Service for a variety of functions in game development. Some common uses include:
- Moving game objects from one position to another.
- Transitioning GUI elements for enhanced player experience.
- Animating sequences within the game.
Why should you learn it?
Learning how to use the Tween Service can significantly boost the quality of your Roblox games. It enables:
- Smooth and high-quality animations.
- Improved player interactions within the game.
- Professional-looking transitions and movements.
Also, being adept at using Tween Service in Roblox broadens your development skill set, making you better equipped to create unique and immersive game experiences.
Creating a Basic Tween
To create your first Tween in Roblox, you need a target object and defined properties. The following script could be used to move a Part object from its current position to a new position:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(5) local properties = {Position = Vector3.new(10, 10, 10)} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play()
In this example, you are:
– Defining the TweenService
– Specifying your target object
– Designating the tween parameters using TweenInfo.new and the desired amount of transition time (in this case, 5 seconds)
– Providing the property values that you’d like to change
– Directing the TweenService to create this tween
– Commanding the Tween to play with :Play()
Animating Multiple Properties
A single tween could make multiple property changes. For instance, an object could be moved, reoriented, and resized simultaneously:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(2) local properties = {Position = Vector3.new(10, 10, 10), Orientation = Vector3.new(90, 0, 0), Size = Vector3.new(1, 1, 1)} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play()
With the above script, the object moves to a new position, rotates 90 degrees around the x-axis, and changes its size to 1 stud in every direction.
Utilizing Easing Styles and Directions
Tween Service additionally gives control over the way these transitions happen. For example, animations can be ‘eased’ in different styles and directions:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(2, Enum.EasingStyle.Sine, Enum.EasingDirection.InOut) local properties = {Position = Vector3.new(10, 10, 10)} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play()
In this script, the object moves to a new position with a sine wave-shaped easing in and out, which forms a smoother transition.
Keep playing around with these examples and code snippets and discover the incredible possibilities that Tween Service offers for your Roblox games.
A Deep Dive into TweenInfo Parameters
Understanding the TweenInfo parameters gives more control over your animations. Here are the parameters broken down:
- Time: This is how long the tween will take to complete in seconds.
- EasingStyle: This parameter defines how the interpolation of the properties is done.
- EasingDirection: Direction of easing.
- RepeatCount: Specifies the number of times tween will repeat.
- Reverses: If set to true, the tween will reverse and play backwards once it finishes.
- DelayTime: Specifies the amount of delay before the function starts.
Here’s an example of the TweenInfo with all parameters:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(5, Enum.EasingStyle.Quart, Enum.EasingDirection.Out, 2, true, 1) local properties = {Position = Vector3.new(10, 10, 10)} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play()
With this set up, the part will move to (10, 10, 10) position in 5 seconds with a ‘Quart’ easing style and ‘Out’ direction. The tween animation will be repeated 2 times, play back in reverse after each run, and start only after a 1-second delay.
Chaining Tweens Together
Want to create a sequence of tweens? You can utilize the Completed event which fires after a tween finishes. Let’s see an example:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(2) local properties1 = {Position = Vector3.new(10, 10, 10)} local properties2 = {Position = Vector3.new(0, 0, 0)} local tween1 = TweenService:Create(Part, tweenInfo, properties1) local tween2 = TweenService:Create(Part, tweenInfo, properties2) tween1.Completed:Connect(function() tween2:Play() end) tween1:Play()
In this script, when ‘tween1’ finishes, ‘tween2’ fires immediately, creating a sequence of animations.
Wait for Tween Completion
There may be instances where you want to pause the script execution until a tween completes. This can be achieved with the :Wait() function. Here’s an example:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(2) local properties = {Position = Vector3.new(10, 10, 10)} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play() tween:Wait() print("Tween Completed!")
In this case, the ‘Tween Completed!’ message will only print after the tween finishes.
TweenService provides an extremely flexible system for managing animations in your Roblox games. It allows for a high degree of customization, giving you control over precisely how and when elements in your game move or change. Happy Tweening!
Adjusting Transparency with Tween
Tween Service can also be utilized to adjust the transparency of a part:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(5) local properties = {Transparency = 1} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play()
In this script, the part’s transparency changes gradually from its initial state to fully transparent over 5 seconds.
Color Change with Tween
Animating the color of an object can make your game visually engaging. Let’s illustrate how to transition an object’s color:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(5) local properties = {BrickColor = BrickColor.new("Bright red")} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play()
Over 5 seconds, the Part’s color will transition to bright red.
Tweening Multiple Objects
You can control multiple objects with Tween service:
local TweenService = game:GetService("TweenService") local Part1 = workspace.Part1 local Part2 = workspace.Part2 local tweenInfo = TweenInfo.new(5) local properties = {Position = Vector3.new(10, 10, 10)} local tween1 = TweenService:Create(Part1, tweenInfo, properties) local tween2 = TweenService:Create(Part2, tweenInfo, properties) tween1:Play() tween2:Play()
This will move both Part1 and Part2 to a new position simultaneously.
Handling Tween Status
Checking the status of tween animations can be crucial in some situations. The following script shows how to print the status of a tween:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(5) local properties = {Position = Vector3.new(10, 10, 10)} local tween = TweenService:Create(Part, tweenInfo, properties) tween.StatusChanged:Connect(function(Status) print("Tween Status: ".. Status) end) tween:Play()
Through this, you’d be able to track the different stages in tween’s lifecycle: ‘Playing’, ‘Paused’, and ‘Completed’.
Controlling Tween Playback
Tween Service offers commands to control the playback of a tween:
local TweenService = game:GetService("TweenService") local Part = workspace.Part local tweenInfo = TweenInfo.new(5) local properties = {Position = Vector3.new(10, 10, 10)} local tween = TweenService:Create(Part, tweenInfo, properties) tween:Play() wait(2) tween:Pause() wait(2) tween:Cancel() wait(2) tween:Play()
In this script, the tween plays for 2 seconds, then pauses for 2 seconds. After that, the tween gets canceled and then restarts from the beginning.
With Tween Service, you can create a unique experience tailored to your vision, whether it’s animating GUI elements, controlling in-game objects, or transitioning player characters. Whichever way you slice it, mastering Tween Service is an essential step on your journey as a Roblox game developer!
Moving Forward With Roblox Game Development
You’ve now taken your first steps into the exciting world of animation and game design in Roblox. We hope you can leverage these newfound skills to create engaging and immersive gameplay experiences.
Ready to continue your journey in Roblox game creation? We invite you to explore the Roblox Game Development Mini-Degree offered by us at Zenva Academy. Our comprehensive collection of courses will empower you with the necessary tools to make games with Roblox Studio and Lua. Covering topics such as basic level creation, scripting, melee battle games, and first-person shooter games, these courses cater to both beginners and experienced developers.
Moreover, we offer an extensive collection of Roblox coding and game development courses that cater to a variety of skill levels and learning objectives. Keep exploring and upgrading your skills. The potential for creation within the Roblox environment is vast and should inspire you to push your boundaries and create something truly unique. Happy coding!
Conclusion
In the realm of Roblox game development, skills such as mastering the Tween Service could greatly enhance the quality of your games. It provides a gateway to creating engaging visuals and more interactive gameplay. Here, at Zenva Academy, we believe in empowering learners with the necessary tools to step up their game development skills.
Whether you’re starting from scratch or looking to level up your existing skills, our range of Roblox Game Development courses can equip you with the skills you want. Why wait? Step into the exciting journey of game development with Zenva – and who knows, your game could be the next big hit on Roblox!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
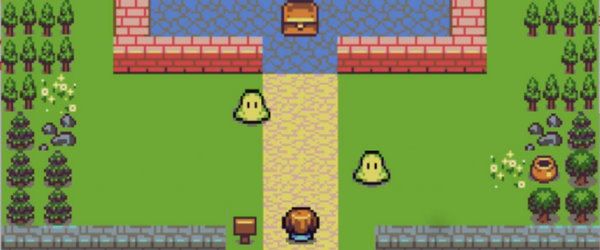
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.