Welcome to our comprehensive guide on Python error handling! This enthralling topic is one that all coders, beginner and experienced alike, encounter daily. It’s a fundamental part of programming and marks an important step forward in any coder’s journey. By understanding and learning to effectively handle Python errors, you move from merely writing code to becoming a real problem solver.
Table of contents
What is Python Error Handling?
Python Error Handling refers to how we address the inevitable ‘bugs’ or ‘errors’ that show up in our code. When an error occurs in Python, it can either be handled (caught) and dealt with, or it can remain unhandled, leading the program to stop working (crashing). A strategic handling of errors not only prevents your program from crashing but also affords the opportunity to provide useful feedback to the user.
Why Should I Learn It?
Errors are a part of coding. But it’s how we deal with these errors that separates good programmers from great ones. Learning to properly handle errors will grant your program stability and resilience it needs to perform in the real world.
– Enhances debugging: Error handling can provide precise feedback about what’s going wrong.
– Improves user experience: No user enjoys a crash! Graceful handling of errors gives a more professional outlook to your programs.
– Makes your code robust: In unexpected scenarios, your program will be able to react and continue running instead of just breaking down.
Stay tuned as we delve into the mechanics of Python error handling with engaging examples. Never again will a Python error daunt you!
Python Exception Handling – Basics
In Python, exceptions are the errors detected during execution, which are handled in Python by using ‘try’ and ‘except’ statements.
A Basic Example
try: print(5/0) except: print("An error occurred")
When run, instead of stopping and showing an error message, our program simply prints ‘An error occurred’.
Catching Specific Exceptions
We can catch specific exceptions by mentioning them in the except clause.
try: print(5/0) except ZeroDivisionError: print("You can't divide by zero")
This program will print “You can’t divide by zero”.
The else Clause
In Python, you can use the ‘else’ keyword to define a block of code to be executed if no errors were raised.
try: print("Hello") except: print("Something went wrong") else: print("Nothing went wrong")
Here, since there is no exception, ‘Nothing went wrong’ will be printed.
Using the finally Clause
try: print(x) except: print("Something went wrong") finally: print("The 'try except' is finished")
The ‘finally’ block lets you execute tasks that should always be run, regardless of the existence of an exception. It’s great for clean-up tasks.
Raising Exceptions
You can manually trigger (raise) an exception with the raise keyword.
x = -1 if x < 0: raise Exception("Sorry, no numbers below zero")
Here, since x is less than 0, the exception will be raised and the program will stop after printing the error message.
Conclusion
If you’ve made it this far, then congratulations! You now have a solid understanding of Python error handling basics. It won’t be a walk in the park from here, but understand that tackling and learning from errors is a big part of becoming an accomplished programmer. With time, handling errors will become second nature and you’ll find it a powerful tool in your coding arsenal. Happy coding!
Dealing with Multiple Exceptions
In Python, you can catch multiple exceptions by providing a tuple of error types.
try: # some code except (TypeError, ZeroDivisionError): print("Caught an exception")
Multiple except Blocks
You can also have multiple except blocks to handle different exceptions in different ways.
try: # some code except TypeError: print("TypeError occurred") except ZeroDivisionError: print("Divided by zero!")
Using the exception Instance
To access the error message associated with an exception, you can use an exception instance.
try: # some code except Exception as e: print("Caught an exception: " + str(e))
The exception instance e represents the exception that was raised.
Built-In Exception Hierarchy
Python exceptions are organized in a hierarchy, with all exceptions inheriting from the baseException class. You can even create your own custom exceptions by deriving from this base class.
Custom Exceptions
class CustomError(Exception): pass def raises_exception(): raise CustomError("This is a custom error") try: raises_exception() except CustomError as e: print("Caught a custom exception: " + str(e))
Using assert Statements
The ‘assert’ keyword is used when debugging code. The assert keyword lets you test if a condition in your code returns true, if not, the program will raise an AssertionError.
x = "hello" # if condition returns False, AssertionError is raised: assert x == "goodbye", "x should be 'goodbye'"
In this case, as the condition is false, an AssertionError ‘x should be ‘goodbye” will be raised.
Using try-except-else-finally
The full form of try-except-else-finally gives you robust control over your error handling.
try: # some code except Exception as e: print("Caught an exception: " + str(e)) else: print("No exceptions were raised!") finally: print("Exiting the program...")
The ‘finally’ clause is always executed no matter what.
Where to Go Next in Your Python Journey?
With your newfound knowledge in Python error handling, you may be wondering where to go next. Let us reassure you – there is plenty more to an explorer’s journey in the realm of Python. As you move forward, challenges may seem daunting, but remember, as a programmer, you’re a problem solver. And what would a problem solver be without problems to solve?
One of our highlights is the Python Mini-Degree– a fully-fledged guide for Python programming. Beyond coding basics, this range of courses aims to groom you to effectively utilize Python’s powerful capabilities – from creating games and apps to developing complex algorithms and diving into object-oriented programming. Why choose the Python Mini-Degree?
- It’s comprehensive. The mini-degree covers vast topics providing a broad understanding of Python.
- Taught by experienced instructors. Learn from experts certified by Unity Technologies and CompTIA.
- Suitable for all levels. Whether you’re a newbie or an experienced pro, the curriculum can be tailored to your skill level.
- Rich resources: Video lessons, interactive exercises, coding challenges and more.
- Get certified. On completion of the courses, earn certifications to add to your profile.
Check out our broad collection of Python courses for more. Your journey has only just begun; keep going and happy coding!
Conclusion
Python error handling is a crucial step in becoming a truly versatile coder. Mastering this subject imbues your code with resilience and aptitude, making your programs not only error-free but also user-friendly. Remember, every programmer faces errors, but only the best ones know how to handle them effectively.
Your Python journey doesn’t stop at error handling. Dive headfirst into the world of Python with our comprehensive Python Programming Mini-Degree. This extensive course offers a broad understanding of Python equipped to turn you into a bona fide Python programmer. Whatever your experience or goals may be, we at Zenva are excited to accompany you on your coding journey. Onward and upward—and happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
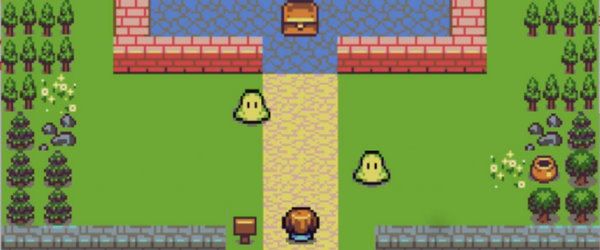
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.