Ever wondered how to establish order in your programming code when things become chaotic? Welcome to the fascinating world of clean-up actions in Python!
Performing clean-up actions in Python is crucial for writing sophisticated and efficient code, particularly when dealing with exceptional scenarios and maintaining resources.
Table of contents
What are Clean-Up Actions in Python?
Clean-up actions eliminate redundant or unneeded aspects of your code, helping you ensure that your programs run smoothly and efficiently. These clean-up actions can be anything from closing opened files, finalizing reopened ones, or properly shutting down services following their utilization.
Why Should I Learn Clean-Up Actions in Python?
Knowing how to perform clean-up actions is as essential as understanding how to drive a car. It goes beyond getting the car to start and move, it’s about ensuring that you parked correctly and turned off the engine when you’re finished. With the correct implementation of clean-up actions, you can:
- Reduce memory use.
- Improve system performance.
- Avoid the risk of data corruption.
- Control resource allocation better.
Let’s dive into the world of Python clean-up actions with two practical code examples tutorial. Learning applied is learning retained!
Python Clean-Up Actions: Code Examples
Let’s roll up our sleeves and examine a couple of examples where clean-up actions in Python can be employed.
Example 1: Using the try-finally Block
This is the most basic form of clean-up action, whereby a finally clause is implemented after a try block. Regardless of whether an exception has occurred within the try block, the statements within the finally block will always be executed.
try: file = open('example.txt', 'r') print(file.read()) finally: file.close()
In this code snippet, despite the possibility of a file not being found (causing an exception in the try block), the file.close() function in the finally block ensures the file is closed in any scenario.
Example 2: Using the with Statement
An alternative to the try-finally block is using the with statement. It provides a way for ensuring that clean-up code is executed.
with open('example.txt', 'r') as file: print(file.read())
In this example, the with statement makes certain the file is closed when the block of code is exited – irrespective of how the nested block exits – mitigating the need for a separate clean-up code.
Example 3: Cleaning Up in Functions
Sometimes, we need to perform clean-up actions in functions. They can also make use of the try-finally block or with statement.
def clean_receive(file_name): try: file = open(file_name, 'r') data = file.read() return data finally: file.close() print(clean_receive('example.txt'))
Just like in previous examples, this function ensures that the file is closed, regardless of whether it was successfully read or not.
Example 4: Cleaning Up in Class Code
Last but not least, clean-up actions can be used within class code too. Python offers the __del__() special method, which gets invoked when the object is about to be destroyed.
class CleanReceive: def __init__(self, file_name): self.file_name = file_name self.file = None def receive(self): self.file = open(self.file_name, 'r') data = self.file.read() return data def __del__(self): if self.file: self.file.close() clean = CleanReceive('example.txt') print(clean.receive())
Here our __del__() method is the clean-up action, which ensures that the file is properly closed when the object is about to be destroyed.
More Python Clean-Up Actions: In-depth Code Examples
Let’s move forward on our journey and examine a range of more intricate examples where clean-up actions in Python can be employed.
Example 5: Using Else Clause in the Try-Except Block
We can incorporate an else clause, which will be executed in scenarios where no exceptions are met within the try block of code.
try: file = open('example.txt', 'r') except IOError: print('An error occurred while opening the file.') else: try: print(file.read()) finally: file.close()
In this example, if the file example.txt fails to open, an exception is raised and the error message is printed. If the file opens successfully, the file’s content is printed, and the file is finally closed regardless of whether an exception occurs in the nested try block.
Example 6: Using Exit and Enter in a Class
In Python, class methods __enter__() and __exit__() can be used for controlling resources. This is typically utilized for file manipulation but can be adapted for any other resource that requires closing or clean-up.
class ManagedFile: def __init__(self, name): self.name = name def __enter__(self): self.file = open(self.name, 'r') return self.file def __exit__(self, exc_type, exc_val, exc_tb): if self.file: self.file.close() with ManagedFile('example.txt') as f: print(f.read())
This code creates a class ManagedFile that uses the special __enter__ and __exit__ methods. During the file manipulation process within the with block, if any exception is encountered, the __exit__ method ensures the file is closed, performing the necessary clean-up actions.
Example 7: Cleaning Up with the contextlib Module
The contextlib standard library in Python provides functionalities for resource management and helps in creating and managing the context in a more Pythonic way.
from contextlib import contextmanager @contextmanager def managed_file(name): try: f = open(name, 'r') yield f finally: f.close() with managed_file('example.txt') as f: print(f.read())
This snippet of code defines a generator function with a decorator contextmanager. This function yields the file object which has to be managed and makes sure the file is closed after the with block is exited, thus taking care of the clean-up actions.
Where To Go Next?
Having mastered the Python clean-up actions, where do you go next on your journey to becoming a Python programming guru? The answer is simple – continue exploring, practicing, and leveraging the numerous available resources to keep expanding your knowledge.
Check out our comprehensive Python Mini-Degree. Our Python Mini-Degree covers a wide range of subjects, taking you on a journey from the basics of coding, through algorithms, and all the way to game and app development using popular libraries and frameworks such as Pygame, Tkinter, and Kivy. You’ll even tackle engaging projects like creating games, building real-world applications, and developing AI chatbots.
The Python Mini-Degree is designed for both beginners and experienced programmers, with flexible learning options and access to live coding lessons and quizzes. Completing these courses not only helps learners build an impressive portfolio of Python projects but also equips them with in-demand skills for today’s job market.
Interested in more Python courses? Take a look at our wide collection of Python courses here.
Let’s continue pushing the boundaries of learning, innovating, and coding with Python!
Conclusion
Understanding clean-up actions in Python is crucial in your programming career, providing you with the skill to write efficient and error-free code. As you’ve seen from our examples, clean-up actions ensure resources are correctly managed and your programs are running as smoothly as they should be.
Ready to take your Python skills to the next level? Join us at Zenva, and gain access to an expansive library of courses, resources, and learning materials designed to empower you in your coding journey. Let’s master Python together, one lesson at a time!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
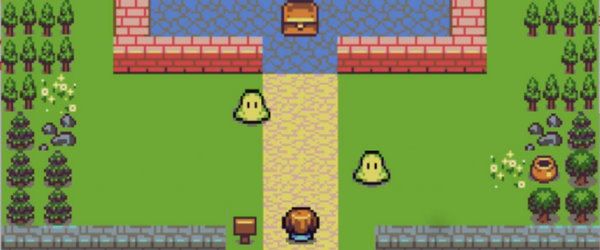
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.