Welcome to our thorough guide on Pygame Opacity. In this tutorial, we aim to demystify this essential concept in a simple, digestible manner to enrich your understanding of Pygame, a key library in Python game development.
Table of contents
What is Pygame Opacity?
Pygame Opacity refers to the feature of controlling transparency in Pygame’s Surface objects. Also known as alpha, opacity in Pygame offers developers a tool to create more dynamic visuals and effects in their games.
Why Learn About Pygame Opacity?
Learning about Pygame Opacity equips you with an essential tool in developing visually appealing games in Python. It is a versatile technique that can be applied in multiple ways to enhance your game visuals, such as creating fade-in or fade-out effects, or making objects semi-transparent.
What Is It Used For?
Opacity in Pygame is utilized in various ways, from creating layers of Surface objects with varying degrees of transparency to orchestrating compelling fade effects. Its possibilities in game development are bound only by a game developer’s creativity. With a strong grasp of Pygame Opacity, you can take your game visuals to a whole new level of sophistication.
Setting Up Pygame
Before we delve into Pygame Opacity, let’s set up a basic Pygame window. The following code will create a 600×400 window with a white background.
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) screen.fill((255,255,255)) pygame.display.flip() while pygame.event.wait().type != pygame.QUIT: pass pygame.quit()
With this foundation, we can proceed with exploring opacity.
Creating a Semi-Transparent Surface
To create a semi-transparent surface, you simply add an extra parameter to the color definition when using the ‘fill()’ command. We can use the following code to create a semi-transparent red surface over our existing white screen.
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) screen.fill((255,255,255)) surface = pygame.Surface((600,400), pygame.SRCALPHA) surface.fill((255,0,0,50)) screen.blit(surface, (0,0)) pygame.display.flip() while pygame.event.wait().type != pygame.QUIT: pass pygame.quit()
The fourth element of the tuple (255,0,0,50) represents the alpha value, or opacity. Here, we’ve set the opacity of the red surface to a low value of 50 (where 255 would be fully opaque), giving us a semi-transparent red overlay.
Creating an Opacity Gradient
By dynamically adjusting the alpha value, we can create interesting effects like opacity gradients. The following code creates a vertical gradient on our surface:
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) screen.fill((255,255,255)) surface = pygame.Surface((600,400), pygame.SRCALPHA) for i in range(600): pygame.draw.line(surface, (255,0,0,i//2.37), (i,0), (i,400)) screen.blit(surface, (0,0)) pygame.display.flip() while pygame.event.wait().type != pygame.QUIT: pass pygame.quit()
Here, we used a loop to draw thin vertical lines – each with a slightly different alpha value – across the surface. This resulted in a gradient effect where the left side is more transparent than the right.
Opacity in Game Sprites
Opacity can also be applied to in-game sprites to implement effects such as ghosting or flashing. Here’s how you would give a sprite a semi-transparent effect:
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) screen.fill((255,255,255)) sprite = pygame.image.load('sprite.png') sprite.fill((255, 255, 255, 128), None, pygame.BLEND_RGBA_MULT) screen.blit(sprite, (100,100)) pygame.display.flip() while pygame.event.wait().type != pygame.QUIT: pass pygame.quit()
In this code snippet, ‘sprite.png’ should be replaced with the file path to your sprite image. After loading the sprite, we modified its color to add a semi-transparent effect by using the ‘fill()’ function with alpha value set to 128.
Animating Surface Opacity
By continuously modifying the opacity value in your game loop, you can animate the opacity of your Surface objects and create fade-in and fade-out effects. Here’s an example of a simple fade-in effect:
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) surface = pygame.Surface((600,400), pygame.SRCALPHA) opacity = 0 clock = pygame.time.Clock() while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() screen.fill((255,255,255)) surface.fill((255,0,0,opacity)) screen.blit(surface, (0,0)) pygame.display.flip() clock.tick(60) if opacity < 255: opacity += 1
In this example, we’ve created a game loop where the opacity of the red surface increases by 1 every frame, creating a gradual fade-in effect. The clock.tick(60) ensures our game runs at a constant 60 frames per second, so the fade-in lasts around 4 seconds in total.
Conditional Opacity
Often, you’ll want to change an object’s opacity based on certain conditions in your game. For example, you might want a sprite to flash when it gets hit:
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) sprite = pygame.image.load('sprite.png') hit = False flash_timer = 0 while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() screen.fill((255,255,255)) if hit: if flash_timer % 2 == 0: sprite.set_alpha(128) else: sprite.set_alpha(255) flash_timer += 1 if flash_timer > 10: hit = False sprite.set_alpha(255) screen.blit(sprite, (100,100)) pygame.display.flip()
In this game loop, when the ‘hit’ variable is true, the sprite will flash for approximately 10 frames by alternating between semi-transparent and opaque states. After 10 flashes, it returns to the normal, fully opaque state.
Whether it’s to create more visually appealing graphics, to indicate a character’s invincibility or to punctuate a dramatic moment in your game, understanding Pygame Opacity pulls you a step closer to creating a more compelling gaming experience. At Zenva, we believe in empowering learners to be able to take their skills to the next level and bring their imaginations into reality.
Applying Opacity to Text
Similar to images and Surfaces, we can also apply various levels of transparency to text to enrich the visual experience. This can be especially useful when creating game menus or flying text effects.
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) font = pygame.font.Font(None, 72) text = font.render('Hello, Zenva!', True, (255,0,0)) surface = pygame.Surface(text.get_size(), pygame.SRCALPHA) surface.blit(text, (0,0)) opacity = 128 surface.fill((255,255,255,opacity), None, pygame.BLEND_RGBA_MULT) screen.blit(surface, (80,150)) pygame.display.flip() while pygame.event.wait().type != pygame.QUIT: pass pygame.quit()
In the above code, we’ve created a semi-transparent text effect by blitting the text onto a Surface, then modifying the alpha value of that surface.
Opacity for Layering
With Pygame opacity, one can also create layered effects by overlaying Surfaces with varying levels of transparency. Let’s consider an example where we overlay two squares of different colors and opacities:
import pygame pygame.init() screen = pygame.display.set_mode((200,200)) surface1 = pygame.Surface((100, 100), pygame.SRCALPHA) surface2 = pygame.Surface((100, 100), pygame.SRCALPHA) surface1.fill((255,0,0,180)) surface2.fill((0,255,0,100)) screen.blit(surface1, (50,50)) screen.blit(surface2, (70,70)) pygame.display.flip() while pygame.event.wait().type != pygame.QUIT: pass pygame.quit()
This creates an effect where the second (green) square is overlaid on the first (red) one, creating a blend of the two colors where they overlap.
Interactive Opacity
We can also make interactive opacity changes depending on user input or game state. As an example, let’s make a Surface that changes its opacity based on mouse clicks:
import pygame pygame.init() screen = pygame.display.set_mode((600,400)) surface = pygame.Surface((200, 200), pygame.SRCALPHA) opacity = 255 surface.fill((255,0,0,opacity)) while True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() if event.type == pygame.MOUSEBUTTONDOWN: if opacity > 0: opacity -= 20 surface.fill((255,0,0, opacity)) screen.fill((255,255,255)) screen.blit(surface, (200,100)) pygame.display.flip()
This snippet creates a red square that becomes less opaque with every mouse click, allowing players to interact directly with the game’s visuals.
Conclusion
The power of Pygame’s opacity capabilities truly adds an additional layer of depth (quite literally) to your game visuals. As with any skill, practice is important, and experimenting with your own opacity-based effects will inspire more creative ideas.
Perfecting your expertise in opacity manipulation is just one more step in your journey towards becoming an accomplished game developer. But as we often say at Zenva, a journey of a thousand miles begins with a single step. So, let’s keep moving forward and continue to discover new ways to bring our visions to life in the realm of game development.
Advance Your Python Skills with Zenva
If you have found this tutorial helpful and wish to further advance your Python development skills, we would recommend you to explore our Python Mini-Degree – an inclusive collection of courses aimed at teaching Python programming. Connecting coding basics with real-world projects, like creating games and apps, this degree focuses on libraries such as Pygame, Tkinter, and Kivy. This Mini-Degree offers flexibility for learners at all levels, from beginners to more experienced coders, and can be accessed at your own pace.
Besides, we also have a broad selection of Python courses on offer. It’s an exciting repository where you’d find courses based on your needs and interests. Embarking on these courses could potentially help learners transition into new careers, land jobs, and even start their own businesses.
With Zenva, you can truly pave your pathway from beginner to professional. Our courses are designed with an outcome-oriented approach, providing you with a diverse array of skills that can be put to practical use. So, don’t wait! Dive in now and continue on your exciting journey of code learning and game creation.
Conclusion
Mastering Pygame’s opacity can significantly enrich the visual aesthetics of your games, providing you with creative control to bring your vision to life. As you continue to explore and experiment with different levels of opacity, you’ll discover the vast possibilities this feature brings to your game development toolbox.
We hope you found this tutorial insightful. If you’re eager to learn more, we invite you to join us at Zenva. Here, you’ll find a comprehensive range of programming tutorials and courses, carefully curated to equip you with the skills necessary to turn your dreams into playable realities. The path to becoming a successful game developer is a thrilling one, and we’re excited to embark on that journey with you. Happy coding!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
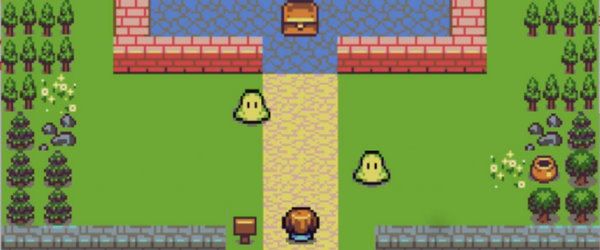
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.