Every good video game creates a seamless interaction between the player and their virtual environment. The bridge that allows this interaction to be smooth and intuitive is usually the game’s control mechanics. In Python, the Pygame library holds the key to unlocking a rich array of controls for your game characters, including, but not limited to, the mouse click feature. With Pygame mouse click, you can transform the gameplay experience, and this tutorial will show you how.
Table of contents
Understanding Pygame Mouse Click
The Pygame mouse click feature allows players to directly interact with game elements. This could be anything from clicking a button to start a game, selecting an item from the menu, to controlling a game character. Mouse controls are a fundamental part of many game genres and create an engaging and intuitive interface for users.
Why Learn About Pygame Mouse Click?
If you’re into game development, learning how to implement the Pygame mouse click feature is invaluable. It’s vital for creating games with complex interfaces or those that require precise point-and-click controls. Furthermore, mastering Pygame mouse click empowers you to leverage one of the most used peripherals, the computer mouse, effectively enhancing your game mechanics.
Detecting Mouse Click in Pygame
In Pygame, the mouse click event can be detected by using the event module specifically the MOUSEBUTTONDOWN function. This function will trigger when a mouse button is pressed.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: print('Mouse button pressed!') pygame.quit()
This code will print ‘Mouse button pressed!’ to your console every time a mouse button is clicked while the game window is active.
Differentiating Between Left & Right Clicks
Pygame allows you to distinguish between left and right mouse clicks. The mouse.get_pressed() method, when combined with an if statement, can be used to determine which mouse button was pressed. The mouse.get_pressed() method returns a tuple of three values. The first value indicates if the left button was pressed, the second for the middle, and the third for the right mouse button.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: if pygame.mouse.get_pressed()[0]: # Left click print('Left mouse button pressed!') elif pygame.mouse.get_pressed()[2]: # Right click print('Right mouse button pressed!') pygame.quit()
With this script, you can determine whether you’ve clicked the left or right mouse button. It enhances the interactivity of your game by enabling different reactions depending on whether the player uses the right or left mouse button.
Finding Mouse Click Coordinates
Apart from detecting mouse clicks, you might want to know the specific position the click occurred on your game screen. Pygame allows you to fetch the x and y coordinates of the mouse pointer at the time of a click using the mouse.get_pos() function.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: x, y = pygame.mouse.get_pos() print(f'Mouse clicked at {x}, {y}') pygame.quit()
Now every time you click within the game window, you will get the exact coordinates of where the click occurred. This can be particularly useful when developing point-and-click games where the position of the mouse click matters.
Interacting with Game Objects Using Mouse Clicks
Knowing which button was pressed or the position of the click is useful but still abstract. To add value to your game, you need to make use of this information to interact with game objects. Let’s explore how you can use the Pygame mouse click feature to interact with various objects in your game.
Creating Game Objects in Pygame
Before we interact with game objects, we need to create some. For simplicity, we will create a rectangle object using the pygame.draw.rect() function.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') rectangle_color = (255,0,0) # Red color rectangle_position = (400, 300) # Centre of screen rectangle_dimension = (100, 100) # Width and Height running = True while running: # Draw rectangle object pygame.draw.rect(gameScreen, rectangle_color, pygame.Rect(rectangle_position, rectangle_dimension)) pygame.display.update() # Update the display for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
This script would create a red square in the middle of your screen. However, it’s not interactive just yet.
Interacting with Game Objects Using Pygame Mouse Click
After creating our square object, we can now detect if it was clicked. Let’s enhance our script to accomplish this.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') rectangle_color = (255,0,0) # Red color rectangle_position = (400, 300) # Centre of screen rectangle_dimension = (100, 100) # Width and Height rectangle = pygame.Rect(rectangle_position, rectangle_dimension) running = True while running: pygame.draw.rect(gameScreen, rectangle_color, rectangle) pygame.display.update() for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: x, y = pygame.mouse.get_pos() # Get click position if rectangle.collidepoint(x, y): # Check if click is within rectangle print("Rectangle clicked!") pygame.quit()
Now, when you click on the square, your console will print ‘Rectangle clicked!’. This is a simple, yet powerful example of how you can combine the mouse click event and game objects to create interactive games.
Adding More Interaction: Changing Object Color on Click
To add more interaction and visual feedback, you could change the color of the square every time it is clicked. Let’s adjust our script one more time.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') rectangle_position = (400, 300) # Centre of screen rectangle_dimension = (100, 100) # Width and Height rectangle = pygame.Rect(rectangle_position, rectangle_dimension) rectangle_color = (255,0,0) # Red color running = True while running: gameScreen.fill((0,0,0)) # Erase previous drawings pygame.draw.rect(gameScreen, rectangle_color, rectangle) pygame.display.update() for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: x, y = pygame.mouse.get_pos() # Get click position if rectangle.collidepoint(x, y): # Check if click is within rectangle rectangle_color = (0,255,0) # Change color to green pygame.quit()
In this example, every time you click the square, it changes its color from red to green. Using these basics, you can expand the interaction to include a variety of changes or functions that create engaging and interactive gameplay.
Handling Multiple Game Object Interactions
Now that we’ve mastered single object interaction, let’s proceed with more complex scenarios. Suppose you have multiple objects on your screen, and you need to handle interactions for each object separately. Here’s how you get started.
Creating Multiple Game Objects
First, let’s add more squares to our game. Instead of one square, we will have three squares of different colors. Also, let’s store these squares in a list for easy access.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') # Define rectangles and their colors rectangles = [ {"rect": pygame.Rect((100, 100), (100, 100)), "color": (255,0,0)}, # Red rectangle {"rect": pygame.Rect((350, 275), (100, 100)), "color": (0,255,0)}, # Green rectangle {"rect": pygame.Rect((600, 450), (100, 100)), "color": (0,0,255)} # Blue rectangle ] running = True while running: gameScreen.fill((0,0,0)) # Clear the screen for r in rectangles: # Draw rectangles pygame.draw.rect(gameScreen, r["color"], r["rect"]) pygame.display.update() for event in pygame.event.get(): if event.type == pygame.QUIT: running = False pygame.quit()
This will create three squares on your screen in different positions and colors.
Distinguishing Object Interactions
Now that we have multiple squares on the screen, let’s work on distinguishing which square is clicked. Each square will react differently when clicked, offering a way to verify which game object was interacted with.
import pygame # Your game setup would go here gameScreen = pygame.display.set_mode((800,600)) pygame.display.set_caption('Pygame Mouse Click - Test Game') # Define rectangles and their colors rectangles = [ {"rect": pygame.Rect((100, 100), (100, 100)), "color": (255,0,0), "clicked_color": (255,255,0)}, # Red rectangle {"rect": pygame.Rect((350, 275), (100, 100)), "color": (0,255,0), "clicked_color": (255,0,255)}, # Green rectangle {"rect": pygame.Rect((600, 450), (100, 100)), "color": (0,0,255), "clicked_color": (0,255,255)} # Blue rectangle ] running = True while running: gameScreen.fill((0,0,0)) # Clear the screen for r in rectangles: # Draw rectangles pygame.draw.rect(gameScreen, r["color"], r["rect"]) pygame.display.update() for event in pygame.event.get(): if event.type == pygame.QUIT: running = False if event.type == pygame.MOUSEBUTTONDOWN: x, y = pygame.mouse.get_pos() # Get click position for r in rectangles: # Check each rectangle if r["rect"].collidepoint(x, y): # Check if click is within rectangle r["color"] = r["clicked_color"] # Change the clicked color pygame.quit()
When you run this script, each square changes color when clicked. This helps to isolate the interaction of each game object and to allow them to behave independently.
That covers some of the basics of using the Pygame mouse click feature in your game. It can provide a whole lot of interaction possibilities in your game and can improve the overall user experience.
There’s a lot more you can do with these tools, especially when you integrate them with other Pygame features. It’s like a key that opens tons of new opportunities once you fully grasp how to use it.
At Zenva, we are all about helping you unlock your potential as a developer. That’s why we offer comprehensive courses that take you from absolute beginner to confident programmer, at your own pace. Happy coding!
Continuing Your Journey in Game Development with Python
Learning how to use the Pygame mouse click feature is one of the many stepping stones in mastering game development with Python. With the Pygame library, the potential to create engaging and interactive games is vast and can open many opportunities for you as a developer. But don’t stop here! There is much more to learn and many more exciting concepts to master.
We encourage you to keep building and experimenting. Create more complex games, test new Pygame features, and continue exploring the world of game development. The more you practice, the better you will get!
If you want to extend your Python and game development skills, check out our Python Mini-Degree program. It is a comprehensive collection of courses covering everything from coding basics, algorithms, object-oriented programming to game, and app development. You’ll get the chance to build your own games, algorithms, and real-world apps, using Python – a popular and versatile programming language embraced across many industries.
And if you’re curious to learn more on a wider range of topics, we invite you to explore our broad collection of Python courses at Zenva Academy. Our aim at Zenva is to help you go from beginner to professional at your own pace, empowering you with the skills you need to boost your career in this ever-evolving field.
Conclusion
Mastering Pygame mouse click handling is a significant stride in your game development journey. It’s an essential feature that breathes life into your games, making them more engaging, interactive, and fun. The joy of seeing your game characters and elements respond to the user’s input, the sheer creativity of it all, is what makes game programming with Python and Pygame such an exciting endeavor.
At Zenva, we are all about helping you unlock your potential as a developer. Our Python Mini-Degree program offers a comprehensive curriculum to take you from beginner to confident programmer, so you can continue creating immersive experiences that thrill and entertain. Your dream to become a skillful game developer can become a reality, and we are here to help you achieve that. Keep coding, and watch your game development skills flourish!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
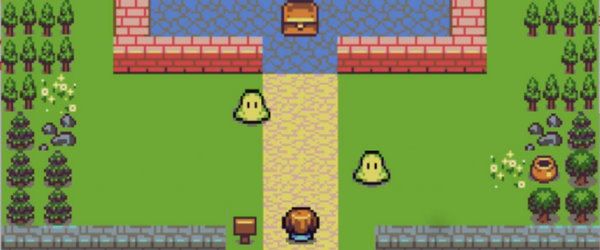
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.