Imagine you’re playing a video game, and mid-way through the game, your character acquires a new outfit. You’d expect to see that change appear on your screen instantly, right? That’s exactly what we’ll learn today. Within the friendly world of Pygame, ensuring your game’s display is updated smoothly and on time is as simple as using the pygame.display.flip() function. But what does this function do, and why is it so significant in the realm of game development?
Table of contents
What is pygame.display.flip()?
At its core, pygame.display.flip() is a Pygame function used to update the entire display. Whenever changes are made within your game frames, Pygame requires this function to reflect those changes on the display. Without it, your changes won’t be seen.
Why Should I learn pygame.display.flip()?
Understanding pygame.display.flip() is crucial to creating games with Pygame. It controls when and how changes made in your code will be visible on the screen. However, don’t be alarmed! It’s an accessible function to grasp and extremely valuable, not just for game development, but also in creating animations or interactive programs. Let’s dive into a practical example to better understand its power and simplicity.
Using pygame.display.flip() in Your Code
To begin with, let’s create a simple game that changes the display color when a key is pressed. Firstly, we need to import the Pygame module and initialize it:
import pygame pygame.init()
Next, we’ll create a basic window for our game:
screen = pygame.display.set_mode((800, 600))
This creates a display window with the size of 800×600 pixels. Now, we are prepared to utilize the pygame.display.flip() function. Let’s set our initial screen color to white:
screen.fill((255, 255, 255)) # fills the screen with a white color pygame.display.flip() # updates the entire display
Our game window should now be entirely white. Let’s move on to include some interaction. We will make our game change the screen’s color to black when a key is pressed:
running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: screen.fill((0, 0, 0)) # fills the screen with a black color pygame.display.flip() # updates the entire display pygame.quit()
Our while loop keeps the game running until we decide to quit. According to the logic, your Pygame window will initially be white, but it will turn black as soon as any key is pressed.
Imagine the pygame.display.flip() commands weren’t present above. The white and black fills would have stayed out of sight. Pygame would have never updated the display to reflect these changes. Hence, pygame.display.flip() is crucial in telling our game when to reflect the crucial modifications in our project.
Understanding the Update Rate
Now, it’s essential to comprehend that updating the game’s appearance too quickly can lead to an overwhelming visual experience. Let’s look at an example regarding the update rate:
clock = pygame.time.Clock() running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: screen.fill((0, 0, 0)) pygame.display.flip() clock.tick(60) # 60 frames per second pygame.quit()
In the above script, the pygame.time.Clock() function is used to control the frame rate, limiting it to the specified number of frames per second (in our case, it’s limited to 60 frames per second). Even if your hardware could execute more frames in a second, the clock.tick(60) will ensure that it doesn’t exceed that limit.
In conclusion, pygame.display.flip() and clock.tick() are fundamental to managing your Pygame’s display updates, offering you complete control over how your game evolves visually.
Enhancing Interaction with pygame.display.flip()
Let’s expand our game by adding more interactivity. We’ll change the color of the screen each time a key is pressed. To do this, we’ll need a way to store and change colors:
colors = [(255, 255, 255), (0, 0, 0), (255, 0, 0), (0, 255, 0), (0, 0, 255)] color_index = 0
Here, we’ve created a list of colors expressed in RGB format, and a color_index variable which will be used to pick a color from this list. Our initial color is white (255, 255, 255).
Let’s modify our event handling code to change the color each time a key is pressed:
while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: color_index = (color_index + 1) % len(colors) # cycling through the color list screen.fill(colors[color_index]) # fills the screen with the current color pygame.display.flip() # updates the entire display clock.tick(60) pygame.quit()
In this modified code, when any key is pressed, the color_index is incremented by 1 and then the modulo operation is performed to keep color_index within the bounds of the color list. The sequence of colors will keep repeating as long as you keep pressing a key.
Partial Screen Update with pygame.display.update()
It should be noted that there may occur situations when only a particular area of your screen needs to be updated rather than the whole screen. In such cases, Pygame provides a pygame.display.update() function, which allows to update a portion of the screen.
To implement this, the area to be updated needs to be passed in as a parameter to the function. This can help save resources as the entire screen isn’t being refreshed each time a change is made. Here is how it could look:
while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: color_index = (color_index + 1) % len(colors) # cycling through the color list screen.fill(colors[color_index]) # fills the screen with the current color pygame.display.update(pygame.Rect(0, 0, 400, 300)) # updates the specific area of the display clock.tick(60) pygame.quit()
In this case, only the portion of the screen specified by the coordinates (0, 0, 400, 300) will be updated to the new color when a key is pressed, while the rest of the screen will maintain its original color.
With these Pygame functions, you now have control over how and when your game display is updated. Whether to use pygame.display.flip() or pygame.display.update() largely depends on your game’s requirements and design choices, and both are significant tools in your game development arsenal.
Controlling Object Visibility with pygame.display.flip()
Pygame.display.flip() is also instrumental in controlling the visibility and disappearance of game objects like sprites. Let’s create an example of a game where a rectangle appears and disappears based on user input.
First, we’ll set up the Pygame environment and initialize a rectangle:
import pygame pygame.init() screen = pygame.display.set_mode((800, 600)) rect = pygame.Rect(50, 50, 200, 200)
We’ve set up a rectangle object that will be located at the (50,50) pixel mark on the screen and has a size of 200×200 pixels.
Now, let’s add a function that draws this rectangle only when the user presses a key:
running = True draw_rect = False while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.KEYDOWN: draw_rect = not draw_rect # toggling the drawing condition if draw_rect: pygame.draw.rect(screen, (255, 0, 0), rect) else: screen.fill((0, 0, 0)) pygame.display.flip() clock.tick(60) pygame.quit()
In the above example, when any key is pressed, the draw_rect condition will toggle between True and False. If draw_rect is True, the rectangle will be drawn; if False, the screen will be filled with black, essentially erasing the rectangle. Pygame.display.flip() here guarantees that the object appears and disappears based on user input.
Interacting with Multiple Objects
Let’s take the concepts we’ve learned and apply them to a more practical example where multiple objects are involved. In this example, we will create multiple rectangles that move around on the screen, and when clicked upon, they change color. To make things a bit easier, we are going to use Pygame’s built-in sprite system.
Firstly, let’s create a subclass of Pygame’s Sprite class for our rectangles:
class RectSprite(pygame.sprite.Sprite): def __init__(self, color, width, height, speed): super().__init__() self.image = pygame.Surface([width, height]) self.image.fill(color) self.rect = self.image.get_rect() self.speed = speed
The RectSprite class takes parameters for color, width, height, and speed. The image attribute gives a rectangular Surface, which is then filled with color. The rect attribute provides the dimensions and positioning of the sprite.
Next, let’s add an update() method that will allow these rectangles to move in random directions:
def update(self): self.rect.x += random.choice([-1, 1])*self.speed self.rect.y += random.choice([-1, 1])*self.speed
In our update() method, we’ve randomly incremented the x and y coordinates of our sprite. Now, whenever we call the update() method on a RectSprite instance, it will move in a random direction.
Now that we’ve finished our RectSprite class, let’s create a few instances, add them to a group, and make them interact with user clicks:
sprite_group = pygame.sprite.Group() for i in range(10): sprite = RectSprite((255, 0, 0), 50, 50, 5) sprite.rect.x = random.randint(0, screen.get_width()) sprite.rect.y = random.randint(0, screen.get_height()) sprite_group.add(sprite) running = True while running: for event in pygame.event.get(): if event.type == pygame.QUIT: running = False elif event.type == pygame.MOUSEBUTTONDOWN: pos = pygame.mouse.get_pos() clicked_sprites = [s for s in sprite_group if s.rect.collidepoint(pos)] for sprite in clicked_sprites: sprite.image.fill((0, 255, 0)) screen.fill((0, 0, 0)) sprite_group.update() sprite_group.draw(screen) pygame.display.flip() clock.tick(60) pygame.quit()
Our game manages 10 randomly moving rectangles that turn green when clicked on. Particularly, multiple sprites could be updated and redisplayed all at once with the sprite_group.update() and sprite_group.draw(screen) functions. Notice that all changes are reflected in the display with pygame.display.flip().
Remember, whether it’s an interaction as simple as changing a color, or one as complex as managing multiple moving and interacting sprites, pygame.display.flip() is the driving force behind promoting these changes onto your game screen.
Where to Go Next?
Congratulations! You’ve now grasped how to effectively manage and update your game displays using Pygame’s display functions. To continue on your journey with Python and broaden your skills further, we encourage you to check out our wide range of courses available on Zenva. We offer over 250 supported courses for all skill levels, ranging from beginner to professional.
We highly recommend our Python Mini-Degree. This comprehensive collection of Python courses will help you harness the versatility of Python, one of the most popular programming languages. The Mini-Degree covers essential topics, including coding basics, algorithms, object-oriented programming, and game and app development. Each concept is taught through step-by-step projects, enabling you to build a portfolio of Python projects along the way.
In addition to the Mini-Degree, you may also want to explore our broader collection of Python courses. These courses extend your Python journey into a multitude of fields, helping you build the skills you need to delve into various industries.
With Zenva, you can start your coding journey, create games, and earn certificates, paving your way from beginner to professional. Keep exploring, keep learning, and keep building!
Conclusion
The world of programming offers endless opportunities to build, innovate, and engage users. If you’ve made it this far, congrats! You have taken a significant stride in the fascinating universe of Python game development with Pygame. The command and control you now have over your game’s visuals with pygame.display.flip() truly opens up exciting potential for what you can create and display.
So, what are you waiting for? Amplify your skills and discover the heights you can reach in Python programming with our comprehensive Python Mini-Degree. We at Zenva are excited to accompany you on your journey towards mastering coding, fostering creativity, and turning your ideas into reality!
Did you come across any errors in this tutorial? Please let us know by completing this form and we’ll look into it!
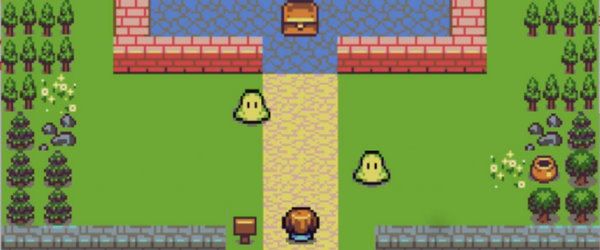
FINAL DAYS: Unlock coding courses in Unity, Godot, Unreal, Python and more.